mirror of
https://github.com/CommunitySolidServer/CommunitySolidServer.git
synced 2024-10-03 14:55:10 +00:00
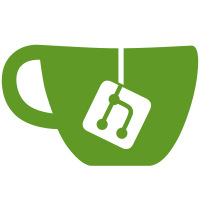
* feat: implemented SizeReporter and FileSizeReporter * test: FileSizeReporter tests * feat: added QuotedDataAccessor * test: added extra test to check recursiveness of filesizereporter * feat: added QuotaStrategy interface * feat: further progress in different files * feat: wrote doc, tests and improved code * feat: fixed bugs and code is now runnable and buildable * feat: finished implementation * fix: revert accidental chanegs * fix: fileSizeReported did not count container size * fix: bug calculating container sizes fixed * test: FileSizeReporter tests * test: QuotaDataValidator tests * test: QuotaError tests * fix: removed console.log * doc: added doc to several files * doc: changed doc for QuotaStrategy to new implementation * fix: improved content length regex * feat: improved GlobalQuotaStrategy code * fix: made FileSizeReported readonly * feat: added comments to quota-file.json * fix: changed default tempFilePath variable * test: included new tempFilePath variable in testing * chore: created seperate command for start:file:quota to pass tests * feat: removed all sync fs calls from FileSizeReporter * feat: minor changes in multple files * fix: changed function signatures to be in line with others * feat: optimized quota data validation * feat: improved FileSizeReporter code * fix: corrected calculation of containersizes and fixed erroring edgecase * feat: save content-length as number in metadata * feat: added comments and changed GlobalQuotaStrategy constructor * feat: changed file names and added small comment * test: AtomicFileDataAccessor tests * test: completed FileSizeReporter tests * fix: content-length is now saved correctly in RepresentationMetadata * feat: adapted content length metadata + tests * fix: removed tempFilePath variable * fix: reverted .gitignore * fix: forgot to remove tempFilePath variable from componentsjs config * test: GlobalQuotaStrategy tests * feat: replaced DataValidator with Validator * feat: reworked DataValidator * feat: added calcultateChunkSize() to SizeReporter * test: updated FileSizeReporter tests * fix: tempFile location now relative to rootFilePath * test: QuotaDataValidator tests * fix: corrected FileSizeReporter tests * fix: adapted FileSizeReporter tests * fix: FileSizeReporter bug on Windows * fix: regex linting error * feat: changed Validator class * feat: added PodQuotaStrategy to enable suota on a per pod basis * chore: bump context versions * fix: Capitalized comments in json file * chore: renamed ValidatorArgs to ValidatorInput * chore: order all exports * fix: made TODO comment clearer * chore: added seperated config files for global and pod based quota + fixed comments * chore: made minor changes to comments * feat: added PassthroughDataAccessor * feat: added PasstroughtDataAccessor + tests * fix: added invalid header check to ContentLengthParser * chore: improved mocks * chore: move quota limit higher up in config * fix: atomicity issue in AtomicFileDataAccessor * chore: moved .internal folder to config from FileSizeReporter * fix: improved algorithm to ignore folders while calculating file size in FileSizeReporter * fix: changes to support containers in the future * fix: added error handling to prevent reading of unexistent files * feat: added generic type to SizeReporter to calculate chunk sizes * test: use mocked DataAccessor * chore: added some comments to test and made minor improvement * fix: fs mock rename * chore: QuotaStrategy.estimateSize refactor * chore: move trackAvailableSpace to abstract class QuotaStrategy * fix: improved test case * test: quota integration tests * chore: edited some comments * chore: change lstat to stat * feat: moved estimateSize to SizeReporter to be consistent with calcultateChunkSize * test: finish up tests to reach coverage * fix: basic config * fix: minor changes to test CI run * fix: small fix for windows * fix: improved writing to file * chore: linting errors * chore: rename trackAvailableSpace * test: improved integration tests * test: logging info for test debugging * test: extra logging for debugging * test: logging for debugging * test: logging for debugging * test: logging for debugging * test: improved Quota integration test setup * test: improve quota tests for CI run * test: debugging Quota test * test: uncommented global quota test * test: changed global quota parameters * test: logging for debugging * test: logging cleanup * chore: minor changes, mostly typo fixes * chore: remove console.log * fix: getting inconsistent results * chore: try fix index.ts CI error * chore: try fix CI error * chore: try fix CI error * chore: revert last commits * chore: fix inconsistent files with origin * test: minor test improvements * chore: minor refactors and improvements * fix: added extra try catch for breaking bug * chore: improve config * chore: minor code improvements * test: use mockFs * feat: add extra check in podQuotaStrategy * chore: replace handle by handleSafe in ValidatingDataAccessor * chore: typo * test: improved Quota integration tests * test: made comment in test more correct * fix: rm -> rmdir for backwards compatibility * fix: fsPromises issue * chore: leave out irrelevant config * chore: removed start script from package.json * fix: Small fixes Co-authored-by: Joachim Van Herwegen <joachimvh@gmail.com>
85 lines
3.9 KiB
TypeScript
85 lines
3.9 KiB
TypeScript
import type { AuxiliaryIdentifierStrategy } from '../../../../src/http/auxiliary/AuxiliaryIdentifierStrategy';
|
|
import { ComposedAuxiliaryStrategy } from '../../../../src/http/auxiliary/ComposedAuxiliaryStrategy';
|
|
import type { MetadataGenerator } from '../../../../src/http/auxiliary/MetadataGenerator';
|
|
import type { Validator } from '../../../../src/http/auxiliary/Validator';
|
|
import { RepresentationMetadata } from '../../../../src/http/representation/RepresentationMetadata';
|
|
|
|
describe('A ComposedAuxiliaryStrategy', (): void => {
|
|
const identifier = { path: 'http://test.com/foo' };
|
|
let identifierStrategy: AuxiliaryIdentifierStrategy;
|
|
let metadataGenerator: MetadataGenerator;
|
|
let validator: Validator;
|
|
let strategy: ComposedAuxiliaryStrategy;
|
|
|
|
beforeEach(async(): Promise<void> => {
|
|
identifierStrategy = {
|
|
getAuxiliaryIdentifier: jest.fn(),
|
|
getAuxiliaryIdentifiers: jest.fn(),
|
|
getSubjectIdentifier: jest.fn(),
|
|
isAuxiliaryIdentifier: jest.fn(),
|
|
};
|
|
metadataGenerator = {
|
|
handleSafe: jest.fn(),
|
|
} as any;
|
|
validator = {
|
|
handleSafe: jest.fn(),
|
|
} as any;
|
|
strategy = new ComposedAuxiliaryStrategy(identifierStrategy, metadataGenerator, validator, false, true);
|
|
});
|
|
|
|
it('calls the AuxiliaryIdentifierStrategy for related calls.', async(): Promise<void> => {
|
|
strategy.getAuxiliaryIdentifier(identifier);
|
|
expect(identifierStrategy.getAuxiliaryIdentifier).toHaveBeenCalledTimes(1);
|
|
expect(identifierStrategy.getAuxiliaryIdentifier).toHaveBeenLastCalledWith(identifier);
|
|
|
|
strategy.getAuxiliaryIdentifiers(identifier);
|
|
expect(identifierStrategy.getAuxiliaryIdentifiers).toHaveBeenCalledTimes(1);
|
|
expect(identifierStrategy.getAuxiliaryIdentifiers).toHaveBeenLastCalledWith(identifier);
|
|
|
|
strategy.getSubjectIdentifier(identifier);
|
|
expect(identifierStrategy.getSubjectIdentifier).toHaveBeenCalledTimes(1);
|
|
expect(identifierStrategy.getSubjectIdentifier).toHaveBeenLastCalledWith(identifier);
|
|
|
|
strategy.isAuxiliaryIdentifier(identifier);
|
|
expect(identifierStrategy.isAuxiliaryIdentifier).toHaveBeenCalledTimes(1);
|
|
expect(identifierStrategy.isAuxiliaryIdentifier).toHaveBeenLastCalledWith(identifier);
|
|
});
|
|
|
|
it('returns the injected value for usesOwnAuthorization.', async(): Promise<void> => {
|
|
expect(strategy.usesOwnAuthorization()).toBe(false);
|
|
});
|
|
|
|
it('returns the injected value for isRequiredInRoot.', async(): Promise<void> => {
|
|
expect(strategy.isRequiredInRoot()).toBe(true);
|
|
});
|
|
|
|
it('adds metadata through the MetadataGenerator.', async(): Promise<void> => {
|
|
const metadata = new RepresentationMetadata();
|
|
await expect(strategy.addMetadata(metadata)).resolves.toBeUndefined();
|
|
expect(metadataGenerator.handleSafe).toHaveBeenCalledTimes(1);
|
|
expect(metadataGenerator.handleSafe).toHaveBeenLastCalledWith(metadata);
|
|
});
|
|
|
|
it('validates data through the Validator.', async(): Promise<void> => {
|
|
const representation = { data: 'data!', metadata: { identifier: { value: 'any' }}} as any;
|
|
await expect(strategy.validate(representation)).resolves.toBeUndefined();
|
|
expect(validator.handleSafe).toHaveBeenCalledTimes(1);
|
|
expect(validator.handleSafe).toHaveBeenLastCalledWith({ representation, identifier: { path: 'any' }});
|
|
});
|
|
|
|
it('defaults isRequiredInRoot to false.', async(): Promise<void> => {
|
|
strategy = new ComposedAuxiliaryStrategy(identifierStrategy, metadataGenerator, validator);
|
|
expect(strategy.isRequiredInRoot()).toBe(false);
|
|
});
|
|
|
|
it('does not add metadata or validate if the corresponding classes are not injected.', async(): Promise<void> => {
|
|
strategy = new ComposedAuxiliaryStrategy(identifierStrategy);
|
|
|
|
const metadata = new RepresentationMetadata();
|
|
await expect(strategy.addMetadata(metadata)).resolves.toBeUndefined();
|
|
|
|
const representation = { data: 'data!' } as any;
|
|
await expect(strategy.validate(representation)).resolves.toBeUndefined();
|
|
});
|
|
});
|