mirror of
https://github.com/CommunitySolidServer/CommunitySolidServer.git
synced 2024-10-03 14:55:10 +00:00
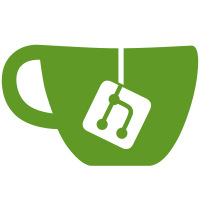
* chore: add the reference change to npm version * chore: remove unused require * chore: add conventional-changelog * chore: add git to pre-release config changes * style: formatting * chore: fix commit message * chore: add no-verify to commit of configs * chore: no more shellJs * chore: fixing async * chore: committing restored * refactor: move and rename * chore: remove shelljs devdep and old script * chore: change npm script ref after refactor * chore: upgrade-config code improvements * chore: edit package.json (not package-lock) * chore(changelog): use conventionalcommits preset * chore: add conventional changelog config * chore: use .versionrc directly * chore: update changelog config * chore: update .versionrc.json * chore: use standard-version * chore: change to standard version * styling(changelog): remove a tags + formatting * styling: conventiontal-changelog styling * chore: postformatting of changelog * chore: remove unnecessary dependencies * chore: add upgrade-config to version as backup * docs: update release.md * styling: order scripts alphabetically * docs: requested changes + dry-run explanation * chore: release script to TS * chore: use ts-node to execute the TS scripts * docs: add some documentation comments to script * docs: remove unnecessary newline * docs: fix comment linting * chore: add test/integration and templates configs * chore: correct automated commit message * chore: remove fdir dependency * chore: remove manual-git-changelog dependency * chore: impl requested changes * docs: update script comments * chore: ensure full cov * chore: review comments
151 lines
5.1 KiB
JavaScript
151 lines
5.1 KiB
JavaScript
/* eslint-disable @typescript-eslint/naming-convention */
|
|
module.exports = {
|
|
root: true,
|
|
parser: '@typescript-eslint/parser',
|
|
parserOptions: {
|
|
tsconfigRootDir: __dirname,
|
|
project: [ './tsconfig.json', './test/tsconfig.json', './scripts/tsconfig.json' ],
|
|
},
|
|
// Ignoring js files (such as this one) since they seem to conflict with rules that require typing info
|
|
ignorePatterns: [ '*.js' ],
|
|
globals: {
|
|
AsyncIterable: 'readonly',
|
|
NodeJS: 'readonly',
|
|
RequestInit: 'readonly',
|
|
},
|
|
plugins: [
|
|
'tsdoc',
|
|
'import',
|
|
'unused-imports',
|
|
],
|
|
extends: [
|
|
'es/node',
|
|
'eslint:recommended',
|
|
'plugin:import/errors',
|
|
'plugin:import/warnings',
|
|
'plugin:import/typescript',
|
|
],
|
|
settings: {
|
|
'import/resolver': {
|
|
typescript: {
|
|
// Always try to resolve types under `<root>@types` directory
|
|
// even it doesn't contain any source code, like `@types/rdf-js`
|
|
alwaysTryTypes: true,
|
|
},
|
|
},
|
|
},
|
|
rules: {
|
|
// There are valid typing reasons to have one or the other
|
|
'@typescript-eslint/consistent-type-definitions': 'off',
|
|
'@typescript-eslint/lines-between-class-members': [ 'error', { exceptAfterSingleLine: true }],
|
|
'@typescript-eslint/no-empty-interface': 'off',
|
|
// Breaks with default void in AsyncHandler 2nd generic
|
|
'@typescript-eslint/no-invalid-void-type': 'off',
|
|
// Problems with optional parameters
|
|
'@typescript-eslint/no-unnecessary-condition': 'off',
|
|
'@typescript-eslint/prefer-optional-chain': 'error',
|
|
'@typescript-eslint/promise-function-async': [ 'error', { checkArrowFunctions: false } ],
|
|
'@typescript-eslint/space-before-function-paren': [ 'error', 'never' ],
|
|
'@typescript-eslint/unbound-method': 'off',
|
|
'@typescript-eslint/unified-signatures': 'off',
|
|
// Conflicts with functions from interfaces that sometimes don't require `this`
|
|
'class-methods-use-this': 'off',
|
|
'comma-dangle': [ 'error', 'always-multiline' ],
|
|
'dot-location': [ 'error', 'property' ],
|
|
'eslint-comments/disable-enable-pair': 'off',
|
|
// Allow declaring overloads in TypeScript (https://eslint.org/docs/rules/func-style)
|
|
'func-style': [ 'error', 'declaration' ],
|
|
'generator-star-spacing': [ 'error', 'after' ],
|
|
// Conflicts with padded-blocks
|
|
'lines-around-comment': 'off',
|
|
'lines-between-class-members': [ 'error', 'always', { exceptAfterSingleLine: true }],
|
|
'max-len': [ 'error', { code: 120, ignoreUrls: true }],
|
|
// Used for RDF constants
|
|
'new-cap': 'off',
|
|
// Necessary in constructor overloading
|
|
'no-param-reassign': 'off',
|
|
// Checked by @typescript-eslint/no-redeclare
|
|
'no-redeclare': 'off',
|
|
// Conflicts with external libraries
|
|
'no-underscore-dangle': 'off',
|
|
// Already checked by @typescript-eslint/no-unused-vars
|
|
'no-unused-vars': 'off',
|
|
'padding-line-between-statements': 'off',
|
|
'prefer-named-capture-group': 'off',
|
|
// Already generated by TypeScript
|
|
strict: 'off',
|
|
'tsdoc/syntax': 'error',
|
|
'unicorn/catch-error-name': 'off',
|
|
'unicorn/import-index': 'off',
|
|
'unicorn/import-style': 'off',
|
|
// The next 2 some functional programming paradigms
|
|
'unicorn/no-array-callback-reference': 'off',
|
|
'unicorn/no-fn-reference-in-iterator': 'off',
|
|
'unicorn/no-object-as-default-parameter': 'off',
|
|
'unicorn/numeric-separators-style': 'off',
|
|
// At function only supported in Node v16.6.0
|
|
'unicorn/prefer-at': 'off',
|
|
// Does not make sense for more complex cases
|
|
'unicorn/prefer-object-from-entries': 'off',
|
|
// Only supported in Node v15
|
|
'unicorn/prefer-string-replace-all' : 'off',
|
|
// Can get ugly with large single statements
|
|
'unicorn/prefer-ternary': 'off',
|
|
'yield-star-spacing': [ 'error', 'after' ],
|
|
|
|
// Need to use the typescript version of this rule to support overloading
|
|
"no-dupe-class-members": "off",
|
|
"@typescript-eslint/no-dupe-class-members": ["error"],
|
|
|
|
// Naming conventions
|
|
'@typescript-eslint/naming-convention': [
|
|
'error',
|
|
{
|
|
selector: 'default',
|
|
format: [ 'camelCase' ],
|
|
leadingUnderscore: 'forbid',
|
|
trailingUnderscore: 'forbid',
|
|
},
|
|
{
|
|
selector: 'variable',
|
|
format: [ 'camelCase', 'UPPER_CASE' ],
|
|
leadingUnderscore: 'forbid',
|
|
trailingUnderscore: 'forbid',
|
|
},
|
|
{
|
|
selector: 'typeLike',
|
|
format: [ 'PascalCase' ],
|
|
},
|
|
{
|
|
selector: [ 'typeParameter' ],
|
|
format: [ 'PascalCase' ],
|
|
prefix: [ 'T' ],
|
|
},
|
|
],
|
|
|
|
// Import
|
|
'@typescript-eslint/consistent-type-imports': [ 'error', { prefer: 'type-imports' }],
|
|
// Disabled in favor of eslint-plugin-import
|
|
'sort-imports': 'off',
|
|
'import/order': [ 'error', {
|
|
alphabetize: {
|
|
order: 'asc',
|
|
caseInsensitive: true,
|
|
},
|
|
}],
|
|
'import/no-duplicates': 'error',
|
|
'import/no-extraneous-dependencies': 'error',
|
|
'import/no-named-as-default': 'off',
|
|
// Doesn't work with type imports
|
|
'no-duplicate-imports': 'off',
|
|
'unused-imports/no-unused-imports-ts': 'error',
|
|
},
|
|
|
|
overrides: [
|
|
{
|
|
files: '*.js',
|
|
parser: 'espree',
|
|
},
|
|
],
|
|
};
|