mirror of
https://github.com/CommunitySolidServer/CommunitySolidServer.git
synced 2024-10-03 14:55:10 +00:00
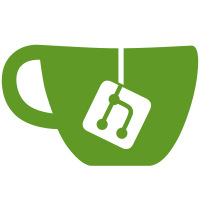
* chore: add the reference change to npm version * chore: remove unused require * chore: add conventional-changelog * chore: add git to pre-release config changes * style: formatting * chore: fix commit message * chore: add no-verify to commit of configs * chore: no more shellJs * chore: fixing async * chore: committing restored * refactor: move and rename * chore: remove shelljs devdep and old script * chore: change npm script ref after refactor * chore: upgrade-config code improvements * chore: edit package.json (not package-lock) * chore(changelog): use conventionalcommits preset * chore: add conventional changelog config * chore: use .versionrc directly * chore: update changelog config * chore: update .versionrc.json * chore: use standard-version * chore: change to standard version * styling(changelog): remove a tags + formatting * styling: conventiontal-changelog styling * chore: postformatting of changelog * chore: remove unnecessary dependencies * chore: add upgrade-config to version as backup * docs: update release.md * styling: order scripts alphabetically * docs: requested changes + dry-run explanation * chore: release script to TS * chore: use ts-node to execute the TS scripts * docs: add some documentation comments to script * docs: remove unnecessary newline * docs: fix comment linting * chore: add test/integration and templates configs * chore: correct automated commit message * chore: remove fdir dependency * chore: remove manual-git-changelog dependency * chore: impl requested changes * docs: update script comments * chore: ensure full cov * chore: review comments
92 lines
3.2 KiB
TypeScript
92 lines
3.2 KiB
TypeScript
#!/usr/bin/env ts-node
|
|
/* eslint-disable no-console */
|
|
import escapeStringRegexp from 'escape-string-regexp';
|
|
import { readdir, readFile, writeFile } from 'fs-extra';
|
|
import simpleGit from 'simple-git';
|
|
import { joinFilePath, readPackageJson } from '../src/util/PathUtil';
|
|
|
|
/**
|
|
* Script: upgradeConfigs.ts
|
|
* Run with: ts-node scripts/upgradeConfig.ts
|
|
* ------------------------------------------
|
|
* Upgrades the lsd:module references to CSS in package.json
|
|
* and all JSON-LD config files.
|
|
* This script is run alongside standard-version after the
|
|
* version bump is done in package.json but before the
|
|
* release has been committed.
|
|
*/
|
|
|
|
/**
|
|
* Search and replace the version of a component with given name
|
|
* @param filePath - File to search/replace
|
|
* @param regex - RegExp matching the component reference
|
|
* @param version - Semantic version to change to
|
|
*/
|
|
async function replaceComponentVersion(filePath: string, regex: RegExp, version: string): Promise<void> {
|
|
console.log(`Replacing version in ${filePath}`);
|
|
const data = await readFile(filePath, 'utf8');
|
|
const result = data.replace(regex, `$1^${version}`);
|
|
return writeFile(filePath, result, 'utf8');
|
|
}
|
|
|
|
/**
|
|
* Recursive search for files that match a given Regex
|
|
* @param path - Path of folder to start search in
|
|
* @param regex - A regular expression to which file names will be matched
|
|
* @returns Promise with all file pathss
|
|
*/
|
|
async function getFilePaths(path: string, regex: RegExp): Promise<string[]> {
|
|
const entries = await readdir(path, { withFileTypes: true });
|
|
|
|
const files = entries
|
|
.filter((file): boolean => !file.isDirectory())
|
|
.filter((file): boolean => regex.test(file.name))
|
|
.map((file): string => joinFilePath(path, file.name));
|
|
|
|
const folders = entries.filter((folder): boolean => folder.isDirectory());
|
|
|
|
for (const folder of folders) {
|
|
files.push(...await getFilePaths(joinFilePath(path, folder.name), regex));
|
|
}
|
|
|
|
return files;
|
|
}
|
|
|
|
/**
|
|
* Changes version of Component references in package.json and
|
|
* JSON-LD config files (config/) to the current major version of
|
|
* the NPM package.
|
|
* Commits changes to config files (not package.json, changes to
|
|
* that file are included in the release commit).
|
|
*/
|
|
async function upgradeConfig(): Promise<void> {
|
|
const pkg = await readPackageJson();
|
|
const major = pkg.version.split('.')[0];
|
|
|
|
console.log(`Changing ${pkg['lsd:module']} references to ${major}.0.0\n`);
|
|
|
|
const configs = await getFilePaths('config/', /.+\.json/u);
|
|
configs.push(...await getFilePaths('test/integration/config/', /.+\.json/u));
|
|
configs.push(...await getFilePaths('templates/config/', /.+\.json/u));
|
|
|
|
const escapedName = escapeStringRegexp(pkg['lsd:module']);
|
|
const regex = new RegExp(`(${escapedName}/)${/\^\d+\.\d+\.\d+/u.source}`, 'gmu');
|
|
|
|
for (const config of configs) {
|
|
await replaceComponentVersion(config, regex, `${major}.0.0`);
|
|
}
|
|
await replaceComponentVersion('package.json', regex, `${major}.0.0`);
|
|
|
|
await simpleGit().commit(`chore(release): Update configs to v${major}.0.0`, configs, { '--no-verify': null });
|
|
}
|
|
|
|
/**
|
|
* Ends the process and writes out an error in case something goes wrong.
|
|
*/
|
|
function endProcess(error: Error): never {
|
|
console.error(error);
|
|
process.exit(1);
|
|
}
|
|
|
|
upgradeConfig().catch(endProcess);
|