mirror of
https://github.com/CommunitySolidServer/CommunitySolidServer.git
synced 2024-10-03 14:55:10 +00:00
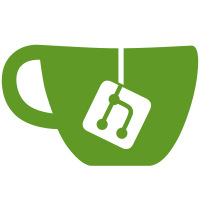
* feat(seeding): seed accounts and pods with seeded-pod-config.json * feat(seeding): dry up SeededPodInitializer by using RegistrationManager directly and make compatible with version/3.0.0 * feat(seeding): update seed config files to version 3.0.0 context * feat(seeding): simplify seeded-root config by importing pre-existing prefilled-root config * feat(seeding): Add seeding as a default initializer, update seeded pod copy and guide, change seeded pod config to array * feat(seeding): remove template info from seeded pod guide, use mockFs, code style nit, fix redlock test * feat(seeding): remove old config file
34 lines
1.4 KiB
TypeScript
34 lines
1.4 KiB
TypeScript
import { AssetPathExtractor } from '../../../../../src/init/variables/extractors/AssetPathExtractor';
|
|
import { joinFilePath } from '../../../../../src/util/PathUtil';
|
|
|
|
describe('An AssetPathExtractor', (): void => {
|
|
let resolver: AssetPathExtractor;
|
|
|
|
beforeEach(async(): Promise<void> => {
|
|
resolver = new AssetPathExtractor('path');
|
|
});
|
|
|
|
it('resolves the asset path.', async(): Promise<void> => {
|
|
await expect(resolver.handle({ path: '/var/data' })).resolves.toBe('/var/data');
|
|
});
|
|
|
|
it('errors if the path is not a string.', async(): Promise<void> => {
|
|
await expect(resolver.handle({ path: 1234 })).rejects.toThrow('Invalid path argument');
|
|
});
|
|
|
|
it('converts paths containing the module path placeholder.', async(): Promise<void> => {
|
|
await expect(resolver.handle({ path: '@css:config/file.json' }))
|
|
.resolves.toEqual(joinFilePath(__dirname, '../../../../../config/file.json'));
|
|
});
|
|
|
|
it('defaults to the given path if none is provided.', async(): Promise<void> => {
|
|
resolver = new AssetPathExtractor('path', '/root');
|
|
await expect(resolver.handle({ otherPath: '/var/data' })).resolves.toBe('/root');
|
|
});
|
|
|
|
it('returns null if not default value or default is provided.', async(): Promise<void> => {
|
|
resolver = new AssetPathExtractor('path');
|
|
await expect(resolver.handle({ otherPath: '/var/data' })).resolves.toBeNull();
|
|
});
|
|
});
|