mirror of
https://github.com/CommunitySolidServer/CommunitySolidServer.git
synced 2024-10-03 14:55:10 +00:00
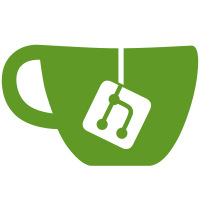
* feat: initial StremingHTTPChannel2023 notifications Co-authored-by: Maciej Samoraj <maciej.samoraj@gmail.com> * test: unit for StremingHTTPChannel2023 notifications Co-authored-by: Maciej Samoraj <maciej.samoraj@gmail.com> * test: integration for StremingHTTPChannel2023 notifications Co-authored-by: Maciej Samoraj <maciej.samoraj@gmail.com> * emit initial notification on streaming http channel * fix linting erros * ensure canceling fetch body in integration tests * extract defaultChannel for topic into util * add documentation * Apply suggestions from code review Co-authored-by: Ted Thibodeau Jr <tthibodeau@openlinksw.com> * only generate notifications when needed Co-authored-by: Maciej Samoraj <maciej.samoraj@gmail.com> * test: set body timeout to pass on node >21 Co-authored-by: Maciej Samoraj <maciej.samoraj@gmail.com> * address review feedback * remove node 21 workaround * add architecture documentation * Apply suggestions from code review Co-authored-by: Joachim Van Herwegen <joachimvh@gmail.com> --------- Co-authored-by: Maciej Samoraj <maciej.samoraj@gmail.com> Co-authored-by: Ted Thibodeau Jr <tthibodeau@openlinksw.com> Co-authored-by: Joachim Van Herwegen <joachimvh@gmail.com>
82 lines
3.1 KiB
TypeScript
82 lines
3.1 KiB
TypeScript
import type { WebSocket } from 'ws';
|
|
import { BasicRepresentation } from '../../../../../src/http/representation/BasicRepresentation';
|
|
import type { NotificationChannel } from '../../../../../src/server/notifications/NotificationChannel';
|
|
import {
|
|
WebSocket2023Emitter,
|
|
} from '../../../../../src/server/notifications/WebSocketChannel2023/WebSocket2023Emitter';
|
|
import type { SetMultiMap } from '../../../../../src/util/map/SetMultiMap';
|
|
import { WrappedSetMultiMap } from '../../../../../src/util/map/WrappedSetMultiMap';
|
|
|
|
describe('A WebSocket2023Emitter', (): void => {
|
|
const channel: NotificationChannel = {
|
|
id: 'id',
|
|
topic: 'http://example.com/foo',
|
|
type: 'type',
|
|
};
|
|
|
|
let webSocket: jest.Mocked<WebSocket>;
|
|
let socketMap: SetMultiMap<string, WebSocket>;
|
|
let emitter: WebSocket2023Emitter;
|
|
|
|
beforeEach(async(): Promise<void> => {
|
|
webSocket = {
|
|
send: jest.fn(),
|
|
} as any;
|
|
|
|
socketMap = new WrappedSetMultiMap();
|
|
|
|
emitter = new WebSocket2023Emitter(socketMap);
|
|
});
|
|
|
|
it('emits notifications to the stored WebSockets.', async(): Promise<void> => {
|
|
socketMap.add(channel.id, webSocket);
|
|
|
|
const representation = new BasicRepresentation('notification', 'text/plain');
|
|
await expect(emitter.handle({ channel, representation })).resolves.toBeUndefined();
|
|
expect(webSocket.send).toHaveBeenCalledTimes(1);
|
|
expect(webSocket.send).toHaveBeenLastCalledWith('notification');
|
|
});
|
|
|
|
it('destroys the representation if there is no matching WebSocket.', async(): Promise<void> => {
|
|
const representation = new BasicRepresentation('notification', 'text/plain');
|
|
await expect(emitter.handle({ channel, representation })).resolves.toBeUndefined();
|
|
expect(webSocket.send).toHaveBeenCalledTimes(0);
|
|
expect(representation.data.destroyed).toBe(true);
|
|
});
|
|
|
|
it('can send to multiple matching WebSockets.', async(): Promise<void> => {
|
|
const webSocket2: jest.Mocked<WebSocket> = {
|
|
send: jest.fn(),
|
|
} as any;
|
|
|
|
socketMap.add(channel.id, webSocket);
|
|
socketMap.add(channel.id, webSocket2);
|
|
|
|
const representation = new BasicRepresentation('notification', 'text/plain');
|
|
await expect(emitter.handle({ channel, representation })).resolves.toBeUndefined();
|
|
expect(webSocket.send).toHaveBeenCalledTimes(1);
|
|
expect(webSocket.send).toHaveBeenLastCalledWith('notification');
|
|
expect(webSocket2.send).toHaveBeenCalledTimes(1);
|
|
expect(webSocket2.send).toHaveBeenLastCalledWith('notification');
|
|
});
|
|
|
|
it('only sends to the matching WebSockets.', async(): Promise<void> => {
|
|
const webSocket2: jest.Mocked<WebSocket> = {
|
|
send: jest.fn(),
|
|
} as any;
|
|
const channel2: NotificationChannel = {
|
|
...channel,
|
|
id: 'other',
|
|
};
|
|
|
|
socketMap.add(channel.id, webSocket);
|
|
socketMap.add(channel2.id, webSocket2);
|
|
|
|
const representation = new BasicRepresentation('notification', 'text/plain');
|
|
await expect(emitter.handle({ channel, representation })).resolves.toBeUndefined();
|
|
expect(webSocket.send).toHaveBeenCalledTimes(1);
|
|
expect(webSocket.send).toHaveBeenLastCalledWith('notification');
|
|
expect(webSocket2.send).toHaveBeenCalledTimes(0);
|
|
});
|
|
});
|