mirror of
https://github.com/CommunitySolidServer/CommunitySolidServer.git
synced 2024-10-03 14:55:10 +00:00
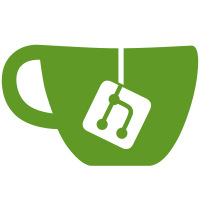
This allows PermissionReaders to potentially only check the necessary access modes for potential performance optimization.
36 lines
1.3 KiB
TypeScript
36 lines
1.3 KiB
TypeScript
import { CredentialGroup } from '../../../src/authentication/Credentials';
|
|
import { AllStaticReader } from '../../../src/authorization/AllStaticReader';
|
|
import type { Permission } from '../../../src/authorization/permissions/Permissions';
|
|
|
|
function getPermissions(allow: boolean): Permission {
|
|
return {
|
|
read: allow,
|
|
write: allow,
|
|
append: allow,
|
|
create: allow,
|
|
delete: allow,
|
|
};
|
|
}
|
|
|
|
describe('An AllStaticReader', (): void => {
|
|
const credentials = { [CredentialGroup.agent]: {}, [CredentialGroup.public]: undefined };
|
|
const identifier = { path: 'http://test.com/resource' };
|
|
|
|
it('can handle everything.', async(): Promise<void> => {
|
|
const authorizer = new AllStaticReader(true);
|
|
await expect(authorizer.canHandle({} as any)).resolves.toBeUndefined();
|
|
});
|
|
|
|
it('always returns permissions matching the given allow parameter.', async(): Promise<void> => {
|
|
let authorizer = new AllStaticReader(true);
|
|
await expect(authorizer.handle({ credentials, identifier, modes: new Set() })).resolves.toEqual({
|
|
[CredentialGroup.agent]: getPermissions(true),
|
|
});
|
|
|
|
authorizer = new AllStaticReader(false);
|
|
await expect(authorizer.handle({ credentials, identifier, modes: new Set() })).resolves.toEqual({
|
|
[CredentialGroup.agent]: getPermissions(false),
|
|
});
|
|
});
|
|
});
|