mirror of
https://github.com/bigchaindb/bigchaindb.git
synced 2024-10-13 13:34:05 +00:00
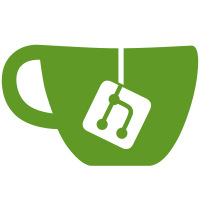
* Allow AssetLinks to be used in place of Assets in the Transaction Model and enforce `Transaction.transfer()` to only take an AssetLink * Remove AssetLink's inheritance from Asset * Remove id from the Asset model * Fix get_txids_by_asset_id query for rethinkdb after removing asset's uuid Because `CREATE` transactions don't have an asset that contains an id anymore, one way to find all the transactions related to an asset is to query the database twice: once for the `CREATE` transaction and another for the `TRANSFER` transactions. * Add TODO notice for vote test utils to be fixtures * Update asset model documentation to reflect usage of transaction id * Fix outdated asset description in transaction schema
90 lines
1.6 KiB
Python
90 lines
1.6 KiB
Python
""" Script to build http examples for http server api docs """
|
|
|
|
import json
|
|
import os
|
|
import os.path
|
|
|
|
from bigchaindb.common.transaction import Asset, Transaction
|
|
|
|
|
|
TPLS = {}
|
|
|
|
TPLS['post-tx-request'] = """\
|
|
POST /transactions/ HTTP/1.1
|
|
Host: example.com
|
|
Content-Type: application/json
|
|
|
|
%(tx)s
|
|
"""
|
|
|
|
|
|
TPLS['post-tx-response'] = """\
|
|
HTTP/1.1 201 Created
|
|
Content-Type: application/json
|
|
|
|
%(tx)s
|
|
"""
|
|
|
|
|
|
TPLS['get-tx-status-request'] = """\
|
|
GET /transactions/%(txid)s/status HTTP/1.1
|
|
Host: example.com
|
|
|
|
"""
|
|
|
|
|
|
TPLS['get-tx-status-response'] = """\
|
|
HTTP/1.1 200 OK
|
|
Content-Type: application/json
|
|
|
|
{
|
|
"status": "valid"
|
|
}
|
|
"""
|
|
|
|
|
|
TPLS['get-tx-request'] = """\
|
|
GET /transactions/%(txid)s HTTP/1.1
|
|
Host: example.com
|
|
|
|
"""
|
|
|
|
|
|
TPLS['get-tx-response'] = """\
|
|
HTTP/1.1 200 OK
|
|
Content-Type: application/json
|
|
|
|
%(tx)s
|
|
"""
|
|
|
|
|
|
def main():
|
|
""" Main function """
|
|
privkey = 'CfdqtD7sS7FgkMoGPXw55MVGGFwQLAoHYTcBhZDtF99Z'
|
|
pubkey = '4K9sWUMFwTgaDGPfdynrbxWqWS6sWmKbZoTjxLtVUibD'
|
|
asset = Asset(None)
|
|
tx = Transaction.create([pubkey], [([pubkey], 1)], asset=asset)
|
|
tx = tx.sign([privkey])
|
|
tx_json = json.dumps(tx.to_dict(), indent=2, sort_keys=True)
|
|
|
|
base_path = os.path.join(os.path.dirname(__file__),
|
|
'source/drivers-clients/samples')
|
|
|
|
if not os.path.exists(base_path):
|
|
os.makedirs(base_path)
|
|
|
|
for name, tpl in TPLS.items():
|
|
path = os.path.join(base_path, name + '.http')
|
|
code = tpl % {'tx': tx_json, 'txid': tx.id}
|
|
with open(path, 'w') as handle:
|
|
handle.write(code)
|
|
|
|
|
|
def setup(*_):
|
|
""" Fool sphinx into think it's an extension muahaha """
|
|
main()
|
|
|
|
|
|
if __name__ == '__main__':
|
|
main()
|