mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
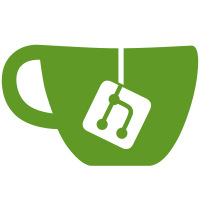
In d0c4916fe9b2afaa273a2a7bc9782321a866ab9f the TLS CA Certificate verification broke. This was bisected using the following basic test: ``` ./bin/etcd -f -name machine0 -data-dir machine0 -ca-file=/tmp/ca/ca.crt -cert-file=/tmp/ca/server.crt -key-file=/tmp/ca/server.key.insecure ``` And in another window doing ``` curl --key /tmp/ca/server2.key.insecure --cert /tmp/ca/server2.crt -k -L https://127.0.0.1:4001/v2/keys/foo -XPUT -d value=bar -v ``` Before merging this PR there are a few things that need to be fixed up: 1) Tests for client certs both positive and negative 2) Refactor (or at least documentation of) the TLSConfig types
44 lines
748 B
Go
44 lines
748 B
Go
package server
|
|
|
|
import (
|
|
"crypto/tls"
|
|
"net"
|
|
)
|
|
|
|
func NewListener(addr string) (net.Listener, error) {
|
|
if addr == "" {
|
|
addr = ":http"
|
|
}
|
|
l, e := net.Listen("tcp", addr)
|
|
if e != nil {
|
|
return nil, e
|
|
}
|
|
return l, nil
|
|
}
|
|
|
|
func NewTLSListener(config *tls.Config, addr, certFile, keyFile string) (net.Listener, error) {
|
|
if addr == "" {
|
|
addr = ":https"
|
|
}
|
|
|
|
if config == nil {
|
|
config = &tls.Config{}
|
|
}
|
|
|
|
config.NextProtos = []string{"http/1.1"}
|
|
|
|
var err error
|
|
config.Certificates = make([]tls.Certificate, 1)
|
|
config.Certificates[0], err = tls.LoadX509KeyPair(certFile, keyFile)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
conn, err := net.Listen("tcp", addr)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return tls.NewListener(conn, config), nil
|
|
}
|