mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
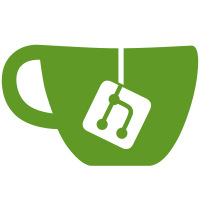
This is a simple solution to having the proxy keep up to date with the state of the cluster. Basically, it uses the cluster configuration provided at start up (i.e. with `-initial-cluster-state`) to determine where to reach peer(s) in the cluster, and then it will periodically hit the `/members` endpoint of those peer(s) (using the same mechanism that `-cluster-state=existing` does to initialise) to update the set of valid client URLs to proxy to. This does not address discovery (#1376), and it would probably be better to update the set of proxyURLs dynamically whenever we fetch the new state of the cluster; but it needs a bit more thinking to have this done in a clean way with the proxy interface. Example in Procfile works again.
93 lines
2.1 KiB
Go
93 lines
2.1 KiB
Go
/*
|
|
Copyright 2014 CoreOS, Inc.
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
|
|
package proxy
|
|
|
|
import (
|
|
"net/url"
|
|
"reflect"
|
|
"testing"
|
|
)
|
|
|
|
func TestNewDirectorScheme(t *testing.T) {
|
|
tests := []struct {
|
|
urls []string
|
|
want []string
|
|
}{
|
|
{
|
|
urls: []string{"http://192.0.2.8:4002", "http://example.com:8080"},
|
|
want: []string{"http://192.0.2.8:4002", "http://example.com:8080"},
|
|
},
|
|
{
|
|
urls: []string{"https://192.0.2.8:4002", "https://example.com:8080"},
|
|
want: []string{"https://192.0.2.8:4002", "https://example.com:8080"},
|
|
},
|
|
|
|
// accept urls without a port
|
|
{
|
|
urls: []string{"http://192.0.2.8"},
|
|
want: []string{"http://192.0.2.8"},
|
|
},
|
|
|
|
// accept urls even if they are garbage
|
|
{
|
|
urls: []string{"http://."},
|
|
want: []string{"http://."},
|
|
},
|
|
}
|
|
|
|
for i, tt := range tests {
|
|
uf := func() []string {
|
|
return tt.urls
|
|
}
|
|
got := newDirector(uf)
|
|
|
|
for ii, wep := range tt.want {
|
|
gep := got.ep[ii].URL.String()
|
|
if !reflect.DeepEqual(wep, gep) {
|
|
t.Errorf("#%d: want endpoints[%d] = %#v, got = %#v", i, ii, wep, gep)
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestDirectorEndpointsFiltering(t *testing.T) {
|
|
d := director{
|
|
ep: []*endpoint{
|
|
&endpoint{
|
|
URL: url.URL{Scheme: "http", Host: "192.0.2.5:5050"},
|
|
Available: false,
|
|
},
|
|
&endpoint{
|
|
URL: url.URL{Scheme: "http", Host: "192.0.2.4:4000"},
|
|
Available: true,
|
|
},
|
|
},
|
|
}
|
|
|
|
got := d.endpoints()
|
|
want := []*endpoint{
|
|
&endpoint{
|
|
URL: url.URL{Scheme: "http", Host: "192.0.2.4:4000"},
|
|
Available: true,
|
|
},
|
|
}
|
|
|
|
if !reflect.DeepEqual(want, got) {
|
|
t.Fatalf("directed to incorrect endpoint: want = %#v, got = %#v", want, got)
|
|
}
|
|
}
|