mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
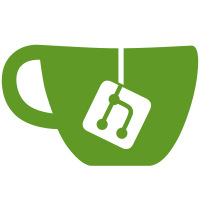
It's followup of #15667. This patch is to use zaptest/observer as base to provide a similar function to pkg/expect.Expect. The test env ```bash 11th Gen Intel(R) Core(TM) i5-1135G7 @ 2.40GHz mkdir /sys/fs/cgroup/etcd-followup-15667 echo 0-2 | tee /sys/fs/cgroup/etcd-followup-15667/cpuset.cpus # three cores ``` Before change: * memory.peak: ~ 681 MiB * Elapsed (wall clock) time (h:mm:ss or m:ss): 6:14.04 After change: * memory.peak: ~ 671 MiB * Elapsed (wall clock) time (h:mm:ss or m:ss): 6:13.07 Based on the test result, I think it's safe to be enabled by default. Signed-off-by: Wei Fu <fuweid89@gmail.com>
84 lines
2.1 KiB
Go
84 lines
2.1 KiB
Go
// Copyright 2023 The etcd Authors
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package testutils
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"fmt"
|
|
"strings"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
"go.uber.org/zap"
|
|
)
|
|
|
|
func TestLogObserver_Timeout(t *testing.T) {
|
|
logCore, logOb := NewLogObserver(zap.InfoLevel)
|
|
|
|
logger := zap.New(logCore)
|
|
logger.Info(t.Name())
|
|
|
|
ctx, cancel := context.WithTimeout(context.TODO(), 100*time.Millisecond)
|
|
_, err := logOb.Expect(ctx, "unknown", 1)
|
|
cancel()
|
|
assert.True(t, errors.Is(err, context.DeadlineExceeded))
|
|
|
|
assert.Equal(t, 1, len(logOb.entries))
|
|
}
|
|
|
|
func TestLogObserver_Expect(t *testing.T) {
|
|
logCore, logOb := NewLogObserver(zap.InfoLevel)
|
|
|
|
logger := zap.New(logCore)
|
|
|
|
ctx, cancel := context.WithCancel(context.TODO())
|
|
defer cancel()
|
|
|
|
resCh := make(chan []string, 1)
|
|
go func() {
|
|
defer close(resCh)
|
|
|
|
res, err := logOb.Expect(ctx, t.Name(), 2)
|
|
require.NoError(t, err)
|
|
resCh <- res
|
|
}()
|
|
|
|
msgs := []string{"Hello " + t.Name(), t.Name() + ", World"}
|
|
for _, msg := range msgs {
|
|
logger.Info(msg)
|
|
time.Sleep(40 * time.Millisecond)
|
|
}
|
|
|
|
res := <-resCh
|
|
assert.Equal(t, 2, len(res))
|
|
|
|
// The logged message should be like
|
|
//
|
|
// 2023-04-16T11:46:19.367+0800 INFO Hello TestLogObserver_Expect
|
|
// 2023-04-16T11:46:19.408+0800 INFO TestLogObserver_Expect, World
|
|
//
|
|
// The prefix timestamp is unpredictable so we should assert the suffix
|
|
// only.
|
|
for idx := range msgs {
|
|
expected := fmt.Sprintf("\tINFO\t%s\n", msgs[idx])
|
|
assert.True(t, strings.HasSuffix(res[idx], expected))
|
|
}
|
|
|
|
assert.Equal(t, 2, len(logOb.entries))
|
|
}
|