mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
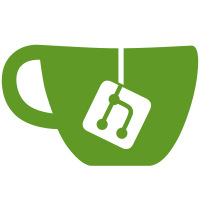
A MsgAppResp with Reject set should be sent back to the leader as soon as possible instead of batching.
42 lines
676 B
Go
42 lines
676 B
Go
package rafthttp
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/coreos/etcd/raft/raftpb"
|
|
)
|
|
|
|
type Batcher struct {
|
|
batchedN int
|
|
batchedT time.Time
|
|
batchN int
|
|
batchD time.Duration
|
|
}
|
|
|
|
func NewBatcher(n int, d time.Duration) *Batcher {
|
|
return &Batcher{
|
|
batchN: n,
|
|
batchD: d,
|
|
batchedT: time.Now(),
|
|
}
|
|
}
|
|
|
|
func (b *Batcher) ShouldBatch(now time.Time) bool {
|
|
b.batchedN++
|
|
batchedD := now.Sub(b.batchedT)
|
|
if b.batchedN >= b.batchN || batchedD >= b.batchD {
|
|
b.Reset(now)
|
|
return false
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (b *Batcher) Reset(t time.Time) {
|
|
b.batchedN = 0
|
|
b.batchedT = t
|
|
}
|
|
|
|
func canBatch(m raftpb.Message) bool {
|
|
return m.Type == raftpb.MsgAppResp && m.Reject == false
|
|
}
|