mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
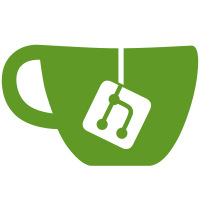
This commit adds --user for auth in benchmarks. Its purpose is measuring overhead of authentication of v3 API. Of course the given user must be granted permission of target keys before benchmarking. Example of a case with no authentication: % ./benchmark range k1 bench with linearizable range 10000 / 10000 Booooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooo! 100.00%2m10s Summary: Total: 130.1850 secs. Slowest: 0.4071 secs. Fastest: 0.0064 secs. Average: 0.0130 secs. Stddev: 0.0079 secs. Requests/sec: 76.8138 Response time histogram: 0.006 [1] | 0.046 [9990] |∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎ 0.087 [3] | 0.127 [0] | 0.167 [3] | 0.207 [2] | 0.247 [0] | 0.287 [0] | 0.327 [0] | 0.367 [0] | 0.407 [1] | Latency distribution: 10% in 0.0076 secs. 25% in 0.0086 secs. 50% in 0.0113 secs. 75% in 0.0146 secs. 90% in 0.0209 secs. 95% in 0.0272 secs. 99% in 0.0344 secs. Example of a case with authentication: % ./benchmark --user=u1:p range k1 bench with linearizable range 10000 / 10000 Booooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooo! 100.00%2m11s Summary: Total: 131.4923 secs. Slowest: 0.1637 secs. Fastest: 0.0065 secs. Average: 0.0131 secs. Stddev: 0.0070 secs. Requests/sec: 76.0501 Response time histogram: 0.006 [1] | 0.022 [9075] |∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎∎ 0.038 [875] |∎∎∎ 0.054 [36] | 0.069 [5] | 0.085 [1] | 0.101 [1] | 0.117 [0] | 0.132 [0] | 0.148 [5] | 0.164 [1] | Latency distribution: 10% in 0.0076 secs. 25% in 0.0087 secs. 50% in 0.0114 secs. 75% in 0.0150 secs. 90% in 0.0215 secs. 95% in 0.0272 secs. 99% in 0.0347 secs. It seems that current auth mechanism does not introduce visible overhead.
86 lines
2.0 KiB
Go
86 lines
2.0 KiB
Go
// Copyright 2015 The etcd Authors
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package cmd
|
|
|
|
import (
|
|
"crypto/rand"
|
|
"fmt"
|
|
"os"
|
|
"strings"
|
|
|
|
"github.com/coreos/etcd/clientv3"
|
|
)
|
|
|
|
var (
|
|
// dialTotal counts the number of mustCreateConn calls so that endpoint
|
|
// connections can be handed out in round-robin order
|
|
dialTotal int
|
|
)
|
|
|
|
func mustCreateConn() *clientv3.Client {
|
|
endpoint := endpoints[dialTotal%len(endpoints)]
|
|
dialTotal++
|
|
cfg := clientv3.Config{Endpoints: []string{endpoint}}
|
|
if !tls.Empty() {
|
|
cfgtls, err := tls.ClientConfig()
|
|
if err != nil {
|
|
fmt.Fprintf(os.Stderr, "bad tls config: %v\n", err)
|
|
os.Exit(1)
|
|
}
|
|
cfg.TLS = cfgtls
|
|
}
|
|
|
|
if len(user) != 0 {
|
|
splitted := strings.SplitN(user, ":", 2)
|
|
if len(splitted) != 2 {
|
|
fmt.Fprintf(os.Stderr, "bad user information: %s\n", user)
|
|
os.Exit(1)
|
|
}
|
|
|
|
cfg.Username = splitted[0]
|
|
cfg.Password = splitted[1]
|
|
}
|
|
|
|
client, err := clientv3.New(cfg)
|
|
if err != nil {
|
|
fmt.Fprintf(os.Stderr, "dial error: %v\n", err)
|
|
os.Exit(1)
|
|
}
|
|
return client
|
|
}
|
|
|
|
func mustCreateClients(totalClients, totalConns uint) []*clientv3.Client {
|
|
conns := make([]*clientv3.Client, totalConns)
|
|
for i := range conns {
|
|
conns[i] = mustCreateConn()
|
|
}
|
|
|
|
clients := make([]*clientv3.Client, totalClients)
|
|
for i := range clients {
|
|
clients[i] = conns[i%int(totalConns)]
|
|
}
|
|
return clients
|
|
}
|
|
|
|
func mustRandBytes(n int) []byte {
|
|
rb := make([]byte, n)
|
|
_, err := rand.Read(rb)
|
|
if err != nil {
|
|
fmt.Fprintf(os.Stderr, "failed to generate value: %v\n", err)
|
|
os.Exit(1)
|
|
}
|
|
return rb
|
|
}
|