mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
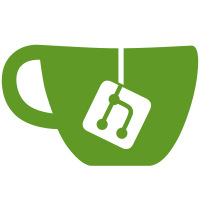
Following command was failing because the parser incorrectly picks up the second "watch" string in exec command, thus passing wrong exec commands. ``` ETCDCTL_API=3 ./bin/etcdctl watch aaa -- echo watch event received panic: runtime error: slice bounds out of range goroutine 1 [running]: github.com/coreos/etcd/etcdctl/ctlv3/command.parseWatchArgs(0xc42002e080, 0x8, 0x8, 0xc420206a20, 0x5, 0x6, 0x0, 0x0, 0x0, 0x0, ...) /home/gyuho/go/src/github.com/coreos/etcd/etcdctl/ctlv3/command/watch_command.go:303 +0xbed github.com/coreos/etcd/etcdctl/ctlv3/command.watchCommandFunc(0xc4202a7180, 0xc420206a20, 0x5, 0x6) /home/gyuho/go/src/github.com/coreos/etcd/etcdctl/ctlv3/command/watch_command.go:73 +0x11d github.com/coreos/etcd/vendor/github.com/spf13/cobra.(*Command).execute(0xc4202a7180, 0xc420206960, 0x6, 0x6, 0xc4202a7180, 0xc420206960) /home/gyuho/go/src/github.com/coreos/etcd/vendor/github.com/spf13/cobra/command.go:766 +0x2c1 github.com/coreos/etcd/vendor/github.com/spf13/cobra.(*Command).ExecuteC(0x1363de0, 0xc420128638, 0xc420185e01, 0xc420185ee8) /home/gyuho/go/src/github.com/coreos/etcd/vendor/github.com/spf13/cobra/command.go:852 +0x30a github.com/coreos/etcd/vendor/github.com/spf13/cobra.(*Command).Execute(0x1363de0, 0x0, 0x0) /home/gyuho/go/src/github.com/coreos/etcd/vendor/github.com/spf13/cobra/command.go:800 +0x2b github.com/coreos/etcd/etcdctl/ctlv3.Start() /home/gyuho/go/src/github.com/coreos/etcd/etcdctl/ctlv3/ctl_nocov.go:25 +0x8e main.main() /home/gyuho/go/src/github.com/coreos/etcd/etcdctl/main.go:40 +0x17b ``` Signed-off-by: Gyuho Lee <gyuhox@gmail.com>
559 lines
20 KiB
Go
559 lines
20 KiB
Go
// Copyright 2017 The etcd Authors
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package command
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
)
|
|
|
|
func Test_parseWatchArgs(t *testing.T) {
|
|
tt := []struct {
|
|
osArgs []string // raw arguments to "watch" command
|
|
commandArgs []string // arguments after "spf13/cobra" preprocessing
|
|
envKey, envRange string
|
|
interactive bool
|
|
|
|
interactiveWatchPrefix bool
|
|
interactiveWatchRev int64
|
|
interactiveWatchPrevKey bool
|
|
|
|
watchArgs []string
|
|
execArgs []string
|
|
err error
|
|
}{
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "bar"},
|
|
commandArgs: []string{"foo", "bar"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "bar", "--"},
|
|
commandArgs: []string{"foo", "bar"},
|
|
interactive: false,
|
|
watchArgs: nil,
|
|
execArgs: nil,
|
|
err: errBadArgsNumSeparator,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch"},
|
|
commandArgs: nil,
|
|
envKey: "foo",
|
|
envRange: "bar",
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo"},
|
|
commandArgs: []string{"foo"},
|
|
envKey: "foo",
|
|
envRange: "",
|
|
interactive: false,
|
|
watchArgs: nil,
|
|
execArgs: nil,
|
|
err: errBadArgsNumConflictEnv,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "bar"},
|
|
commandArgs: []string{"foo", "bar"},
|
|
envKey: "foo",
|
|
envRange: "",
|
|
interactive: false,
|
|
watchArgs: nil,
|
|
execArgs: nil,
|
|
err: errBadArgsNumConflictEnv,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "bar"},
|
|
commandArgs: []string{"foo", "bar"},
|
|
envKey: "foo",
|
|
envRange: "bar",
|
|
interactive: false,
|
|
watchArgs: nil,
|
|
execArgs: nil,
|
|
err: errBadArgsNumConflictEnv,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo"},
|
|
commandArgs: []string{"foo"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch"},
|
|
commandArgs: nil,
|
|
envKey: "foo",
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "--rev", "1", "foo"},
|
|
commandArgs: []string{"foo"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "--rev", "1", "foo"},
|
|
commandArgs: []string{"foo"},
|
|
envKey: "foo",
|
|
interactive: false,
|
|
watchArgs: nil,
|
|
execArgs: nil,
|
|
err: errBadArgsNumConflictEnv,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "--rev", "1"},
|
|
commandArgs: nil,
|
|
envKey: "foo",
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "--rev", "1"},
|
|
commandArgs: []string{"foo"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"foo", "echo", "Hello", "World"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "--", "echo", "watch", "event", "received"},
|
|
commandArgs: []string{"foo", "echo", "watch", "event", "received"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "watch", "event", "received"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "--rev", "1", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"foo", "echo", "Hello", "World"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "--rev", "1", "--", "echo", "watch", "event", "received"},
|
|
commandArgs: []string{"foo", "echo", "watch", "event", "received"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "watch", "event", "received"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "--rev", "1", "foo", "--", "echo", "watch", "event", "received"},
|
|
commandArgs: []string{"foo", "echo", "watch", "event", "received"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "watch", "event", "received"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "bar", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"foo", "bar", "echo", "Hello", "World"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "--rev", "1", "foo", "bar", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"foo", "bar", "echo", "Hello", "World"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "--rev", "1", "bar", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"foo", "bar", "echo", "Hello", "World"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "bar", "--rev", "1", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"foo", "bar", "echo", "Hello", "World"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "bar", "--rev", "1", "--", "echo", "watch", "event", "received"},
|
|
commandArgs: []string{"foo", "bar", "echo", "watch", "event", "received"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "watch", "event", "received"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "--rev", "1", "bar", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"foo", "bar", "echo", "Hello", "World"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "--rev", "1", "foo", "bar", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"foo", "bar", "echo", "Hello", "World"},
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "--rev", "1", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"echo", "Hello", "World"},
|
|
envKey: "foo",
|
|
envRange: "",
|
|
interactive: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "--rev", "1", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"echo", "Hello", "World"},
|
|
envKey: "foo",
|
|
envRange: "bar",
|
|
interactive: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "foo", "bar", "--rev", "1", "--", "echo", "Hello", "World"},
|
|
commandArgs: []string{"foo", "bar", "echo", "Hello", "World"},
|
|
envKey: "foo",
|
|
interactive: false,
|
|
watchArgs: nil,
|
|
execArgs: nil,
|
|
err: errBadArgsNumConflictEnv,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"foo", "bar", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: nil,
|
|
execArgs: nil,
|
|
err: errBadArgsInteractiveWatch,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "foo"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "foo", "bar"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch"},
|
|
envKey: "foo",
|
|
envRange: "bar",
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch"},
|
|
envKey: "hello world!",
|
|
envRange: "bar",
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"hello world!", "bar"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "foo", "--rev", "1"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 1,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "foo", "--rev", "1", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 1,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--rev", "1", "foo", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 1,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--rev", "5", "--prev-kv", "foo", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 5,
|
|
interactiveWatchPrevKey: true,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--rev", "1"},
|
|
envKey: "foo",
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 1,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--rev", "1"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: nil,
|
|
execArgs: nil,
|
|
err: errBadArgsNum,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--rev", "1", "--prefix"},
|
|
envKey: "foo",
|
|
interactive: true,
|
|
interactiveWatchPrefix: true,
|
|
interactiveWatchRev: 1,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--rev", "100", "--prefix", "--prev-kv"},
|
|
envKey: "foo",
|
|
interactive: true,
|
|
interactiveWatchPrefix: true,
|
|
interactiveWatchRev: 100,
|
|
interactiveWatchPrevKey: true,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: nil,
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--rev", "1", "--prefix"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: nil,
|
|
execArgs: nil,
|
|
err: errBadArgsNum,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--", "echo", "Hello", "World"},
|
|
envKey: "foo",
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--", "echo", "Hello", "World"},
|
|
envKey: "foo",
|
|
envRange: "bar",
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "foo", "bar", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 0,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--rev", "1", "foo", "bar", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 1,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "--rev", "1", "--", "echo", "Hello", "World"},
|
|
envKey: "foo",
|
|
envRange: "bar",
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 1,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "foo", "--rev", "1", "bar", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 1,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "foo", "bar", "--rev", "1", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: false,
|
|
interactiveWatchRev: 1,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "foo", "bar", "--rev", "7", "--prefix", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: true,
|
|
interactiveWatchRev: 7,
|
|
interactiveWatchPrevKey: false,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
{
|
|
osArgs: []string{"./bin/etcdctl", "watch", "-i"},
|
|
commandArgs: []string{"watch", "foo", "bar", "--rev", "7", "--prefix", "--prev-kv", "--", "echo", "Hello", "World"},
|
|
interactive: true,
|
|
interactiveWatchPrefix: true,
|
|
interactiveWatchRev: 7,
|
|
interactiveWatchPrevKey: true,
|
|
watchArgs: []string{"foo", "bar"},
|
|
execArgs: []string{"echo", "Hello", "World"},
|
|
err: nil,
|
|
},
|
|
}
|
|
for i, ts := range tt {
|
|
watchArgs, execArgs, err := parseWatchArgs(ts.osArgs, ts.commandArgs, ts.envKey, ts.envRange, ts.interactive)
|
|
if err != ts.err {
|
|
t.Fatalf("#%d: error expected %v, got %v", i, ts.err, err)
|
|
}
|
|
if !reflect.DeepEqual(watchArgs, ts.watchArgs) {
|
|
t.Fatalf("#%d: watchArgs expected %q, got %v", i, ts.watchArgs, watchArgs)
|
|
}
|
|
if !reflect.DeepEqual(execArgs, ts.execArgs) {
|
|
t.Fatalf("#%d: execArgs expected %q, got %v", i, ts.execArgs, execArgs)
|
|
}
|
|
if ts.interactive {
|
|
if ts.interactiveWatchPrefix != watchPrefix {
|
|
t.Fatalf("#%d: interactive watchPrefix expected %v, got %v", i, ts.interactiveWatchPrefix, watchPrefix)
|
|
}
|
|
if ts.interactiveWatchRev != watchRev {
|
|
t.Fatalf("#%d: interactive watchRev expected %d, got %d", i, ts.interactiveWatchRev, watchRev)
|
|
}
|
|
if ts.interactiveWatchPrevKey != watchPrevKey {
|
|
t.Fatalf("#%d: interactive watchPrevKey expected %v, got %v", i, ts.interactiveWatchPrevKey, watchPrevKey)
|
|
}
|
|
}
|
|
}
|
|
}
|