mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
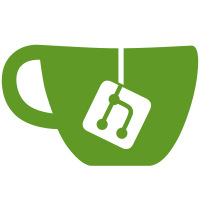
find -name '*.go' | xargs sed --follow-symlinks -i 's|go.etcd.io/etcd/v3/pkg/|go.etcd.io/etcd/pkg/v3/|g' go fmt ./...
106 lines
2.8 KiB
Go
106 lines
2.8 KiB
Go
// Copyright 2020 The etcd Authors
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package integration
|
|
|
|
import (
|
|
"log"
|
|
"net/http"
|
|
"sync"
|
|
"time"
|
|
|
|
"go.etcd.io/etcd/pkg/v3/transport"
|
|
)
|
|
|
|
// Infrastructure to provision a single shared cluster for tests - only
|
|
// when its needed.
|
|
//
|
|
// See ./tests/integration/clientv3/examples/main_test.go for canonical usage.
|
|
// Please notice that the shared (LazyCluster's) state is preserved between
|
|
// testcases, so left-over state might has cross-testcase effects.
|
|
// Prefer dedicated clusters for substancial test-cases.
|
|
|
|
type LazyCluster interface {
|
|
// EndpointsV2 - exposes connection points for client v2.
|
|
// Calls to this method might initialize the cluster.
|
|
EndpointsV2() []string
|
|
|
|
// EndpointsV3 - exposes connection points for client v3.
|
|
// Calls to this method might initialize the cluster.
|
|
EndpointsV3() []string
|
|
|
|
// Cluster - calls to this method might initialize the cluster.
|
|
Cluster() *ClusterV3
|
|
|
|
// Transport - call to this method might initialize the cluster.
|
|
Transport() *http.Transport
|
|
|
|
Terminate()
|
|
}
|
|
|
|
type lazyCluster struct {
|
|
cfg ClusterConfig
|
|
cluster *ClusterV3
|
|
transport *http.Transport
|
|
once sync.Once
|
|
}
|
|
|
|
// NewLazyCluster returns a new test cluster handler that gets created on the
|
|
// first call to GetEndpoints() or GetTransport()
|
|
func NewLazyCluster() LazyCluster {
|
|
return NewLazyClusterWithConfig(ClusterConfig{Size: 1})
|
|
}
|
|
|
|
// NewLazyClusterWithConfig returns a new test cluster handler that gets created
|
|
// on the first call to GetEndpoints() or GetTransport()
|
|
func NewLazyClusterWithConfig(cfg ClusterConfig) LazyCluster {
|
|
return &lazyCluster{cfg: cfg}
|
|
}
|
|
|
|
func (lc *lazyCluster) mustLazyInit() {
|
|
lc.once.Do(func() {
|
|
var err error
|
|
lc.transport, err = transport.NewTransport(transport.TLSInfo{}, time.Second)
|
|
if err != nil {
|
|
log.Fatal(err)
|
|
}
|
|
lc.cluster = NewClusterV3(nil, &lc.cfg)
|
|
})
|
|
}
|
|
|
|
func (lc *lazyCluster) Terminate() {
|
|
if lc != nil && lc.cluster != nil {
|
|
lc.cluster.Terminate(nil)
|
|
lc.cluster = nil
|
|
}
|
|
}
|
|
|
|
func (lc *lazyCluster) EndpointsV2() []string {
|
|
return []string{lc.Cluster().Members[0].URL()}
|
|
}
|
|
|
|
func (lc *lazyCluster) EndpointsV3() []string {
|
|
return lc.Cluster().Client(0).Endpoints()
|
|
}
|
|
|
|
func (lc *lazyCluster) Cluster() *ClusterV3 {
|
|
lc.mustLazyInit()
|
|
return lc.cluster
|
|
}
|
|
|
|
func (lc *lazyCluster) Transport() *http.Transport {
|
|
lc.mustLazyInit()
|
|
return lc.transport
|
|
}
|