mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
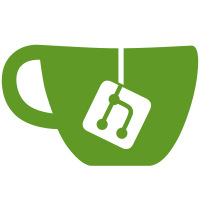
this separates out the listening IP from the advertised IP. This is necessary so that we can hit etcd on 127.0.0.1 but also advertise the right IP to the rest of the cluster.
42 lines
801 B
Go
42 lines
801 B
Go
package main
|
|
|
|
import (
|
|
"net/http"
|
|
)
|
|
|
|
type etcdServer struct {
|
|
http.Server
|
|
name string
|
|
url string
|
|
tlsConf *TLSConfig
|
|
tlsInfo *TLSInfo
|
|
}
|
|
|
|
var e *etcdServer
|
|
|
|
func newEtcdServer(name string, urlStr string, listenHost string, tlsConf *TLSConfig, tlsInfo *TLSInfo) *etcdServer {
|
|
return &etcdServer{
|
|
Server: http.Server{
|
|
Handler: NewEtcdMuxer(),
|
|
TLSConfig: &tlsConf.Server,
|
|
Addr: listenHost,
|
|
},
|
|
name: name,
|
|
url: urlStr,
|
|
tlsConf: tlsConf,
|
|
tlsInfo: tlsInfo,
|
|
}
|
|
}
|
|
|
|
// Start to listen and response etcd client command
|
|
func (e *etcdServer) ListenAndServe() {
|
|
|
|
infof("etcd server [%s:%s]", e.name, e.url)
|
|
|
|
if e.tlsConf.Scheme == "http" {
|
|
fatal(e.Server.ListenAndServe())
|
|
} else {
|
|
fatal(e.Server.ListenAndServeTLS(e.tlsInfo.CertFile, e.tlsInfo.KeyFile))
|
|
}
|
|
}
|