mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
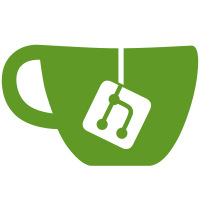
Comments fixed as per goword in go test files that shell function go_srcs_in_module lists as per changes on #14827 Helps in #14827 Signed-off-by: Bhargav Ravuri <bhargav.ravuri@infracloud.io>
94 lines
2.8 KiB
Go
94 lines
2.8 KiB
Go
// Copyright 2022 The etcd Authors
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package e2e
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
|
|
"go.etcd.io/etcd/tests/v3/framework/config"
|
|
"go.etcd.io/etcd/tests/v3/framework/e2e"
|
|
)
|
|
|
|
func TestWarningApplyDuration(t *testing.T) {
|
|
e2e.BeforeTest(t)
|
|
|
|
epc, err := e2e.NewEtcdProcessCluster(context.TODO(), t,
|
|
e2e.WithClusterSize(1),
|
|
e2e.WithWarningUnaryRequestDuration(time.Microsecond),
|
|
)
|
|
if err != nil {
|
|
t.Fatalf("could not start etcd process cluster (%v)", err)
|
|
}
|
|
t.Cleanup(func() {
|
|
if errC := epc.Close(); errC != nil {
|
|
t.Fatalf("error closing etcd processes (%v)", errC)
|
|
}
|
|
})
|
|
|
|
cc, err := e2e.NewEtcdctl(epc.Cfg.Client, epc.EndpointsV3())
|
|
require.NoError(t, err)
|
|
err = cc.Put(context.TODO(), "foo", "bar", config.PutOptions{})
|
|
assert.NoError(t, err, "error on put")
|
|
|
|
// verify warning
|
|
e2e.AssertProcessLogs(t, epc.Procs[0], "request stats")
|
|
}
|
|
|
|
// TestExperimentalWarningApplyDuration tests the experimental warning apply duration
|
|
// TODO: this test is a duplicate of TestWarningApplyDuration except it uses --experimental-warning-unary-request-duration
|
|
// Remove this test after --experimental-warning-unary-request-duration flag is removed.
|
|
func TestExperimentalWarningApplyDuration(t *testing.T) {
|
|
e2e.BeforeTest(t)
|
|
|
|
epc, err := e2e.NewEtcdProcessCluster(context.TODO(), t,
|
|
e2e.WithClusterSize(1),
|
|
e2e.WithExperimentalWarningUnaryRequestDuration(time.Microsecond),
|
|
)
|
|
if err != nil {
|
|
t.Fatalf("could not start etcd process cluster (%v)", err)
|
|
}
|
|
t.Cleanup(func() {
|
|
if errC := epc.Close(); errC != nil {
|
|
t.Fatalf("error closing etcd processes (%v)", errC)
|
|
}
|
|
})
|
|
|
|
cc, err := e2e.NewEtcdctl(epc.Cfg.Client, epc.EndpointsV3())
|
|
require.NoError(t, err)
|
|
err = cc.Put(context.TODO(), "foo", "bar", config.PutOptions{})
|
|
assert.NoError(t, err, "error on put")
|
|
|
|
// verify warning
|
|
e2e.AssertProcessLogs(t, epc.Procs[0], "request stats")
|
|
}
|
|
|
|
func TestBothWarningApplyDurationFlagsFail(t *testing.T) {
|
|
e2e.BeforeTest(t)
|
|
|
|
_, err := e2e.NewEtcdProcessCluster(context.TODO(), t,
|
|
e2e.WithClusterSize(1),
|
|
e2e.WithWarningUnaryRequestDuration(time.Second),
|
|
e2e.WithExperimentalWarningUnaryRequestDuration(time.Second),
|
|
)
|
|
if err == nil {
|
|
t.Fatal("Expected process to fail")
|
|
}
|
|
}
|