mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
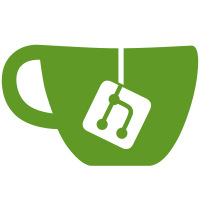
for i in github.com/BurntSushi/toml github.com/coreos/go-etcd/etcd github.com/coreos/go-log/log github.com/gorilla/context github.com/rcrowley/go-metrics bitbucket.org/kardianos/osext github.com/coreos/go-systemd/journal github.com/coreos/raft code.google.com/p/goprotobuf/proto ; do goven -copy -rewrite $i; done
65 lines
1.2 KiB
Go
65 lines
1.2 KiB
Go
package test
|
|
|
|
import (
|
|
"os"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/coreos/etcd/third_party/github.com/coreos/go-etcd/etcd"
|
|
)
|
|
|
|
func TestSimpleMultiNode(t *testing.T) {
|
|
templateTestSimpleMultiNode(t, false)
|
|
}
|
|
|
|
func TestSimpleMultiNodeTls(t *testing.T) {
|
|
templateTestSimpleMultiNode(t, true)
|
|
}
|
|
|
|
// Create a three nodes and try to set value
|
|
func templateTestSimpleMultiNode(t *testing.T, tls bool) {
|
|
procAttr := new(os.ProcAttr)
|
|
procAttr.Files = []*os.File{nil, os.Stdout, os.Stderr}
|
|
|
|
clusterSize := 3
|
|
|
|
_, etcds, err := CreateCluster(clusterSize, procAttr, tls)
|
|
|
|
if err != nil {
|
|
t.Fatal("cannot create cluster")
|
|
}
|
|
|
|
defer DestroyCluster(etcds)
|
|
|
|
time.Sleep(time.Second)
|
|
|
|
c := etcd.NewClient(nil)
|
|
|
|
c.SyncCluster()
|
|
|
|
// Test Set
|
|
result, err := c.Set("foo", "bar", 100)
|
|
node := result.Node
|
|
|
|
if err != nil || node.Key != "/foo" || node.Value != "bar" || node.TTL < 95 {
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
t.Fatalf("Set 1 failed with %s %s %v", node.Key, node.Value, node.TTL)
|
|
}
|
|
|
|
time.Sleep(time.Second)
|
|
|
|
result, err = c.Set("foo", "bar", 100)
|
|
node = result.Node
|
|
|
|
if err != nil || node.Key != "/foo" || node.Value != "bar" || node.TTL < 95 {
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
t.Fatalf("Set 2 failed with %s %s %v", node.Key, node.Value, node.TTL)
|
|
}
|
|
|
|
}
|