mirror of
https://github.com/etcd-io/etcd.git
synced 2024-09-27 06:25:44 +00:00
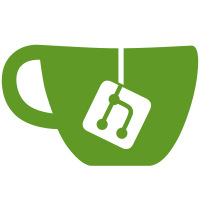
A call file.Sync on OSX doesn't guarantee actual persistence on physical drive media as the data can be cached in physical drive's buffers. Hence calls to file.Sync need to be replaced with fcntl(F_FULLFSYNC).
75 lines
1.8 KiB
Go
75 lines
1.8 KiB
Go
// Copyright 2015 CoreOS, Inc.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package snap
|
|
|
|
import (
|
|
"fmt"
|
|
"io"
|
|
"io/ioutil"
|
|
"os"
|
|
"path"
|
|
|
|
"github.com/coreos/etcd/pkg/fileutil"
|
|
)
|
|
|
|
// SaveDBFrom saves snapshot of the database from the given reader. It
|
|
// guarantees the save operation is atomic.
|
|
func (s *Snapshotter) SaveDBFrom(r io.Reader, id uint64) error {
|
|
f, err := ioutil.TempFile(s.dir, "tmp")
|
|
if err != nil {
|
|
return err
|
|
}
|
|
var n int64
|
|
n, err = io.Copy(f, r)
|
|
if err == nil {
|
|
err = fileutil.Fsync(f)
|
|
}
|
|
f.Close()
|
|
if err != nil {
|
|
os.Remove(f.Name())
|
|
return err
|
|
}
|
|
fn := path.Join(s.dir, fmt.Sprintf("%016x.snap.db", id))
|
|
if fileutil.Exist(fn) {
|
|
os.Remove(f.Name())
|
|
return nil
|
|
}
|
|
err = os.Rename(f.Name(), fn)
|
|
if err != nil {
|
|
os.Remove(f.Name())
|
|
return err
|
|
}
|
|
|
|
plog.Infof("saved database snapshot to disk [total bytes: %d]", n)
|
|
|
|
return nil
|
|
}
|
|
|
|
// DBFilePath returns the file path for the snapshot of the database with
|
|
// given id. If the snapshot does not exist, it returns error.
|
|
func (s *Snapshotter) DBFilePath(id uint64) (string, error) {
|
|
fns, err := fileutil.ReadDir(s.dir)
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
wfn := fmt.Sprintf("%016x.snap.db", id)
|
|
for _, fn := range fns {
|
|
if fn == wfn {
|
|
return path.Join(s.dir, fn), nil
|
|
}
|
|
}
|
|
return "", fmt.Errorf("snap: snapshot file doesn't exist")
|
|
}
|