mirror of
https://github.com/amark/gun.git
synced 2025-03-30 15:08:33 +00:00
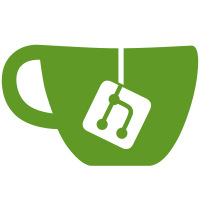
* feat: create pair with seed, content addressing with shorter hash * feat: create pair using priv/epriv * optimize SEA.pair * feat: globalThis along with window * white labeling * feat: add WebAuthn example and enhance SEA.sign, SEA.verify, SEA check.pub, for WebAuthn support * feat: enhance WebAuthn integration with new put options and improved signature handling * polish SEA.sign and SEA.verify * feat: localize options in SEA.check.pub to enhance security and prevent attacks * fix: correct destructuring of user object to enhance security in SEA * rebuild SEA * feat: support ArrayBuffer as seed for key pair generation in SEA * test: add unit test for hashing ArrayBuffer in SEA * fix: create deterministic key pair from seed * fix: add missing B parameter for ECC curve and implement point validation * feat: add ArrayBuffer support for hashing in SEA and implement corresponding unit test * fix: convert numeric salt to string in PBKDF2 implementation * fix: convert numeric salt option to string in PBKDF2 implementation * improve hashing tests * improve sea.work * rebuild SEA * improve SEA.work and rebuild SEA * enhance SEA encryption handling and improve test coverage for SEA functions --------- Co-authored-by: noname <x@null.com> Co-authored-by: x <x@mimiza.com> Co-authored-by: x <null> Co-authored-by: noname <no@name.com>
139 lines
3.1 KiB
JavaScript
139 lines
3.1 KiB
JavaScript
var fs = require('fs');
|
|
var nodePath = require('path');
|
|
|
|
var dir = __dirname + '/../';
|
|
|
|
function read(path) {
|
|
return fs.readFileSync(nodePath.join(dir, path)).toString();
|
|
}
|
|
|
|
function write(path, data) {
|
|
return fs.writeFileSync(nodePath.join(dir, path), data);
|
|
}
|
|
|
|
// The order of modules matters due to dependencies
|
|
const seaModules = [
|
|
'root',
|
|
'https',
|
|
'base64',
|
|
'array',
|
|
'buffer',
|
|
'shim',
|
|
'settings',
|
|
'sha256',
|
|
'sha1',
|
|
'work',
|
|
'pair',
|
|
'sign',
|
|
'verify',
|
|
'aeskey',
|
|
'encrypt',
|
|
'decrypt',
|
|
'secret',
|
|
'certify',
|
|
'sea',
|
|
'user',
|
|
'then',
|
|
'create',
|
|
'auth',
|
|
'recall',
|
|
'share',
|
|
'index'
|
|
];
|
|
|
|
function normalizeContent(code) {
|
|
// Remove IIFE wrapper if present
|
|
code = code.replace(/^\s*;?\s*\(\s*function\s*\(\s*\)\s*\{/, '');
|
|
code = code.replace(/\}\s*\(\s*\)\s*\)?\s*;?\s*$/, '');
|
|
|
|
// Split into lines and remove common indentation
|
|
const lines = code.split('\n');
|
|
let minIndent = Infinity;
|
|
|
|
// Find minimum indentation (ignoring empty lines)
|
|
lines.forEach(line => {
|
|
if (line.trim().length > 0) {
|
|
const indent = line.match(/^\s*/)[0].length;
|
|
minIndent = Math.min(minIndent, indent);
|
|
}
|
|
});
|
|
|
|
// Remove common indentation
|
|
const cleanedLines = lines.map(line => {
|
|
if (line.trim().length > 0) {
|
|
return line.slice(minIndent);
|
|
}
|
|
return '';
|
|
});
|
|
|
|
return cleanedLines.join('\n').trim();
|
|
}
|
|
|
|
function buildSea(arg) {
|
|
if (arg !== 'sea') {
|
|
console.error('Only "sea" argument is supported');
|
|
process.exit(1);
|
|
}
|
|
|
|
// Start with the USE function definition
|
|
let output = `;(function(){
|
|
|
|
/* UNBUILD */
|
|
function USE(arg, req){
|
|
return req? require(arg) : arg.slice? USE[R(arg)] : function(mod, path){
|
|
arg(mod = {exports: {}});
|
|
USE[R(path)] = mod.exports;
|
|
}
|
|
function R(p){
|
|
return p.split('/').slice(-1).toString().replace('.js','');
|
|
}
|
|
}
|
|
if(typeof module !== "undefined"){ var MODULE = module }
|
|
/* UNBUILD */\n\n`;
|
|
|
|
// Add each module wrapped in USE()
|
|
seaModules.forEach(name => {
|
|
try {
|
|
let code = read('sea/' + name + '.js');
|
|
|
|
// Clean up the code
|
|
code = normalizeContent(code);
|
|
|
|
// Replace require() with USE(), but skip any requires within UNBUILD comments
|
|
let inUnbuild = false;
|
|
const lines = code.split('\n').map(line => {
|
|
if (line.includes('/* UNBUILD */')) {
|
|
inUnbuild = !inUnbuild;
|
|
return line;
|
|
}
|
|
if (!inUnbuild) {
|
|
return line.replace(/require\(/g, 'USE(');
|
|
}
|
|
return line;
|
|
});
|
|
code = lines.join('\n');
|
|
|
|
// Add module with consistent indentation
|
|
output += ` ;USE(function(module){\n`;
|
|
output += code.split('\n').map(line => line.length ? ' ' + line : '').join('\n');
|
|
output += `\n })(USE, './${name}');\n\n`;
|
|
} catch(e) {
|
|
console.error('Error processing ' + name + '.js:', e);
|
|
}
|
|
});
|
|
|
|
// Close IIFE
|
|
output += '}());';
|
|
|
|
// Write output
|
|
write('sea.js', output);
|
|
console.log('Built sea.js');
|
|
}
|
|
|
|
if (require.main === module) {
|
|
const arg = process.argv[2];
|
|
buildSea(arg);
|
|
}
|
|
|
|
module.exports = buildSea;
|