mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-08 22:02:34 +00:00
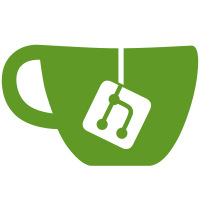
* Fix RPC connections counting * show incomming connections count * Use the flag RPCMaxClients instead of the const RPCMaxInboundConnections * Add grpc server name to log message Co-authored-by: Michael Sutton <mikisiton2@gmail.com>
30 lines
1.0 KiB
Go
30 lines
1.0 KiB
Go
package grpcserver
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/infrastructure/network/netadapter/server"
|
|
"github.com/kaspanet/kaspad/infrastructure/network/netadapter/server/grpcserver/protowire"
|
|
"github.com/kaspanet/kaspad/util/panics"
|
|
)
|
|
|
|
type rpcServer struct {
|
|
protowire.UnimplementedRPCServer
|
|
gRPCServer
|
|
}
|
|
|
|
// RPCMaxMessageSize is the max message size for the RPC server to send and receive
|
|
const RPCMaxMessageSize = 1024 * 1024 * 1024 // 1 GB
|
|
|
|
// NewRPCServer creates a new RPCServer
|
|
func NewRPCServer(listeningAddresses []string, rpcMaxInboundConnections int) (server.Server, error) {
|
|
gRPCServer := newGRPCServer(listeningAddresses, RPCMaxMessageSize, rpcMaxInboundConnections, "RPC")
|
|
rpcServer := &rpcServer{gRPCServer: *gRPCServer}
|
|
protowire.RegisterRPCServer(gRPCServer.server, rpcServer)
|
|
return rpcServer, nil
|
|
}
|
|
|
|
func (r *rpcServer) MessageStream(stream protowire.RPC_MessageStreamServer) error {
|
|
defer panics.HandlePanic(log, "rpcServer.MessageStream", nil)
|
|
|
|
return r.handleInboundConnection(stream.Context(), stream)
|
|
}
|