mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-06-30 18:02:33 +00:00
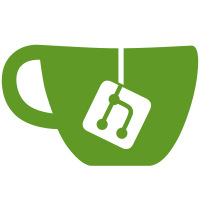
* Unite all reachability stores * Upgrade script * Fix tests * Add UpdateReindexRoot to RebuildReachability * Use dbTx when deleting reachability stores * Use ghostdagDataWithoutPrunedBlocks when rebuilding reachability * Use next tree ancestor wherever possible and avoid finality point search if the block is too close to pruning point * Address the boundary case where the pruning point becomes the finality point * some minor fixes * Remove RebuildReachability and use manual syncing between old and new consensus for migration * Remove sanity test (it failed when tips where not in the same order) Co-authored-by: msutton <mikisiton2@gmail.com>
153 lines
3.8 KiB
Go
153 lines
3.8 KiB
Go
package consensus
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/testapi"
|
|
"github.com/kaspanet/kaspad/infrastructure/db/database"
|
|
)
|
|
|
|
func (tc *testConsensus) DatabaseContext() model.DBManager {
|
|
return tc.databaseContext
|
|
}
|
|
|
|
func (tc *testConsensus) Database() database.Database {
|
|
return tc.database
|
|
}
|
|
|
|
func (tc *testConsensus) AcceptanceDataStore() model.AcceptanceDataStore {
|
|
return tc.acceptanceDataStore
|
|
}
|
|
|
|
func (tc *testConsensus) BlockHeaderStore() model.BlockHeaderStore {
|
|
return tc.blockHeaderStore
|
|
}
|
|
|
|
func (tc *testConsensus) BlockRelationStore() model.BlockRelationStore {
|
|
return tc.blockRelationStores[0]
|
|
}
|
|
|
|
func (tc *testConsensus) BlockStatusStore() model.BlockStatusStore {
|
|
return tc.blockStatusStore
|
|
}
|
|
|
|
func (tc *testConsensus) BlockStore() model.BlockStore {
|
|
return tc.blockStore
|
|
}
|
|
|
|
func (tc *testConsensus) ConsensusStateStore() model.ConsensusStateStore {
|
|
return tc.consensusStateStore
|
|
}
|
|
|
|
func (tc *testConsensus) GHOSTDAGDataStore() model.GHOSTDAGDataStore {
|
|
return tc.ghostdagDataStores[0]
|
|
}
|
|
|
|
func (tc *testConsensus) GHOSTDAGDataStores() []model.GHOSTDAGDataStore {
|
|
return tc.ghostdagDataStores
|
|
}
|
|
|
|
func (tc *testConsensus) HeaderTipsStore() model.HeaderSelectedTipStore {
|
|
return tc.headersSelectedTipStore
|
|
}
|
|
|
|
func (tc *testConsensus) MultisetStore() model.MultisetStore {
|
|
return tc.multisetStore
|
|
}
|
|
|
|
func (tc *testConsensus) PruningStore() model.PruningStore {
|
|
return tc.pruningStore
|
|
}
|
|
|
|
func (tc *testConsensus) ReachabilityDataStore() model.ReachabilityDataStore {
|
|
return tc.reachabilityDataStore
|
|
}
|
|
|
|
func (tc *testConsensus) UTXODiffStore() model.UTXODiffStore {
|
|
return tc.utxoDiffStore
|
|
}
|
|
|
|
func (tc *testConsensus) BlockBuilder() testapi.TestBlockBuilder {
|
|
return tc.testBlockBuilder
|
|
}
|
|
|
|
func (tc *testConsensus) BlockProcessor() model.BlockProcessor {
|
|
return tc.blockProcessor
|
|
}
|
|
|
|
func (tc *testConsensus) BlockValidator() model.BlockValidator {
|
|
return tc.blockValidator
|
|
}
|
|
|
|
func (tc *testConsensus) CoinbaseManager() model.CoinbaseManager {
|
|
return tc.coinbaseManager
|
|
}
|
|
|
|
func (tc *testConsensus) ConsensusStateManager() testapi.TestConsensusStateManager {
|
|
return tc.testConsensusStateManager
|
|
}
|
|
|
|
func (tc *testConsensus) DAGTopologyManager() model.DAGTopologyManager {
|
|
return tc.dagTopologyManagers[0]
|
|
}
|
|
|
|
func (tc *testConsensus) DAGTraversalManager() model.DAGTraversalManager {
|
|
return tc.dagTraversalManager
|
|
}
|
|
|
|
func (tc *testConsensus) DifficultyManager() model.DifficultyManager {
|
|
return tc.difficultyManager
|
|
}
|
|
|
|
func (tc *testConsensus) GHOSTDAGManager() model.GHOSTDAGManager {
|
|
return tc.ghostdagManagers[0]
|
|
}
|
|
|
|
func (tc *testConsensus) HeaderTipsManager() model.HeadersSelectedTipManager {
|
|
return tc.headerTipsManager
|
|
}
|
|
|
|
func (tc *testConsensus) MergeDepthManager() model.MergeDepthManager {
|
|
return tc.mergeDepthManager
|
|
}
|
|
|
|
func (tc *testConsensus) PastMedianTimeManager() model.PastMedianTimeManager {
|
|
return tc.pastMedianTimeManager
|
|
}
|
|
|
|
func (tc *testConsensus) PruningManager() model.PruningManager {
|
|
return tc.pruningManager
|
|
}
|
|
|
|
func (tc *testConsensus) ReachabilityManager() testapi.TestReachabilityManager {
|
|
return tc.testReachabilityManager
|
|
}
|
|
|
|
func (tc *testConsensus) SyncManager() model.SyncManager {
|
|
return tc.syncManager
|
|
}
|
|
|
|
func (tc *testConsensus) TransactionValidator() testapi.TestTransactionValidator {
|
|
return tc.testTransactionValidator
|
|
}
|
|
|
|
func (tc *testConsensus) FinalityManager() model.FinalityManager {
|
|
return tc.finalityManager
|
|
}
|
|
|
|
func (tc *testConsensus) FinalityStore() model.FinalityStore {
|
|
return tc.finalityStore
|
|
}
|
|
|
|
func (tc *testConsensus) HeadersSelectedChainStore() model.HeadersSelectedChainStore {
|
|
return tc.headersSelectedChainStore
|
|
}
|
|
|
|
func (tc *testConsensus) DAABlocksStore() model.DAABlocksStore {
|
|
return tc.daaBlocksStore
|
|
}
|
|
|
|
func (tc *testConsensus) Consensus() externalapi.Consensus {
|
|
return tc
|
|
}
|