mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
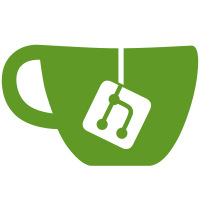
* Remove a random address from the address manager if it's full. * Implement TestOverfillAddressManager. * Add connectionFailedCount to addresses. * Mark connection failures. * Mark connection successes. * Implement removing by most connection failures. * Expand TestOverfillAddressManager. * Add comments. * Use a better method for finding the address with the greatest connectionFailedCount. * Fix a comment. * Compare addresses by IP in TestOverfillAddressManager. * Add a comment for updateNotBanned. Co-authored-by: Ori Newman <orinewman1@gmail.com>
48 lines
1.6 KiB
Go
48 lines
1.6 KiB
Go
package addressmanager
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
"github.com/kaspanet/kaspad/util/mstime"
|
|
"net"
|
|
"reflect"
|
|
"testing"
|
|
)
|
|
|
|
func TestAddressKeySerialization(t *testing.T) {
|
|
addressManager, teardown := newAddressManagerForTest(t, "TestAddressKeySerialization")
|
|
defer teardown()
|
|
addressStore := addressManager.store
|
|
|
|
testAddress := &appmessage.NetAddress{IP: net.ParseIP("2602:100:abcd::102"), Port: 12345}
|
|
testAddressKey := netAddressKey(testAddress)
|
|
|
|
serializedTestAddressKey := addressStore.serializeAddressKey(testAddressKey)
|
|
deserializedTestAddressKey := addressStore.deserializeAddressKey(serializedTestAddressKey)
|
|
if !reflect.DeepEqual(testAddressKey, deserializedTestAddressKey) {
|
|
t.Fatalf("testAddressKey and deserializedTestAddressKey are not equal\n"+
|
|
"testAddressKey:%+v\ndeserializedTestAddressKey:%+v", testAddressKey, deserializedTestAddressKey)
|
|
}
|
|
}
|
|
|
|
func TestAddressSerialization(t *testing.T) {
|
|
addressManager, teardown := newAddressManagerForTest(t, "TestAddressSerialization")
|
|
defer teardown()
|
|
addressStore := addressManager.store
|
|
|
|
testAddress := &address{
|
|
netAddress: &appmessage.NetAddress{
|
|
IP: net.ParseIP("2602:100:abcd::102"),
|
|
Port: 12345,
|
|
Timestamp: mstime.Now(),
|
|
},
|
|
connectionFailedCount: 98465,
|
|
}
|
|
|
|
serializedTestAddress := addressStore.serializeAddress(testAddress)
|
|
deserializedTestAddress := addressStore.deserializeAddress(serializedTestAddress)
|
|
if !reflect.DeepEqual(testAddress, deserializedTestAddress) {
|
|
t.Fatalf("testAddress and deserializedTestAddress are not equal\n"+
|
|
"testAddress:%+v\ndeserializedTestAddress:%+v", testAddress, deserializedTestAddress)
|
|
}
|
|
}
|