mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-06-30 18:02:33 +00:00
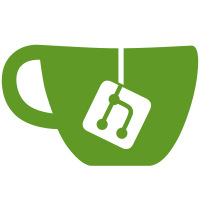
* Replace keccak with CSHAKE256 in oPoW * Add benchmarks to hash writers to compare blake2b to the CSHAKE * Update genesis blocks * Update tests * Define genesis's block level to be the maximal one * Add message to genesis coinbase * Add comments to genesis coinbase * Fix tests Co-authored-by: Ori Newman <orinewman1@gmail.com>
250 lines
10 KiB
Go
250 lines
10 KiB
Go
// Copyright (c) 2014-2016 The btcsuite developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package dagconfig
|
|
|
|
import (
|
|
"github.com/kaspanet/go-muhash"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/blockheader"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/subnetworks"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/transactionhelper"
|
|
"math/big"
|
|
)
|
|
|
|
var genesisTxOuts = []*externalapi.DomainTransactionOutput{}
|
|
|
|
var genesisTxPayload = []byte{
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // Blue score
|
|
0x00, 0xE1, 0xF5, 0x05, 0x00, 0x00, 0x00, 0x00, // Subsidy
|
|
0x00, 0x00, //script version
|
|
0x01, // Varint
|
|
0x00, // OP-FALSE
|
|
0xd7, 0x95, 0xd7, 0x9e, 0xd7, 0x94, 0x20, 0xd7, // ומה די עליך ועל אחיך ייטב בשאר כספא ודהבה למעבד כרעות אלהכם תעבדון
|
|
0x93, 0xd7, 0x99, 0x20, 0xd7, 0xa2, 0xd7, 0x9c,
|
|
0xd7, 0x99, 0xd7, 0x9a, 0x20, 0xd7, 0x95, 0xd7,
|
|
0xa2, 0xd7, 0x9c, 0x20, 0xd7, 0x90, 0xd7, 0x97,
|
|
0xd7, 0x99, 0xd7, 0x9a, 0x20, 0xd7, 0x99, 0xd7,
|
|
0x99, 0xd7, 0x98, 0xd7, 0x91, 0x20, 0xd7, 0x91,
|
|
0xd7, 0xa9, 0xd7, 0x90, 0xd7, 0xa8, 0x20, 0xd7,
|
|
0x9b, 0xd7, 0xa1, 0xd7, 0xa4, 0xd7, 0x90, 0x20,
|
|
0xd7, 0x95, 0xd7, 0x93, 0xd7, 0x94, 0xd7, 0x91,
|
|
0xd7, 0x94, 0x20, 0xd7, 0x9c, 0xd7, 0x9e, 0xd7,
|
|
0xa2, 0xd7, 0x91, 0xd7, 0x93, 0x20, 0xd7, 0x9b,
|
|
0xd7, 0xa8, 0xd7, 0xa2, 0xd7, 0x95, 0xd7, 0xaa,
|
|
0x20, 0xd7, 0x90, 0xd7, 0x9c, 0xd7, 0x94, 0xd7,
|
|
0x9b, 0xd7, 0x9d, 0x20, 0xd7, 0xaa, 0xd7, 0xa2,
|
|
0xd7, 0x91, 0xd7, 0x93, 0xd7, 0x95, 0xd7, 0x9f,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // Bitcoin block hash 00000000000000000001733c62adb19f1b77fa0735d0e11f25af36fc9ca908a5
|
|
0x00, 0x01, 0x73, 0x3c, 0x62, 0xad, 0xb1, 0x9f,
|
|
0x1b, 0x77, 0xfa, 0x07, 0x35, 0xd0, 0xe1, 0x1f,
|
|
0x25, 0xaf, 0x36, 0xfc, 0x9c, 0xa9, 0x08, 0xa5,
|
|
}
|
|
|
|
// genesisCoinbaseTx is the coinbase transaction for the genesis blocks for
|
|
// the main network.
|
|
var genesisCoinbaseTx = transactionhelper.NewSubnetworkTransaction(0, []*externalapi.DomainTransactionInput{}, genesisTxOuts,
|
|
&subnetworks.SubnetworkIDCoinbase, 0, genesisTxPayload)
|
|
|
|
// genesisHash is the hash of the first block in the block DAG for the main
|
|
// network (genesis block).
|
|
var genesisHash = externalapi.NewDomainHashFromByteArray(&[externalapi.DomainHashSize]byte{
|
|
0xca, 0xeb, 0x97, 0x96, 0x0a, 0x16, 0x0c, 0x21,
|
|
0x1a, 0x6b, 0x21, 0x96, 0xbd, 0x78, 0x39, 0x9f,
|
|
0xd4, 0xc4, 0xcc, 0x5b, 0x50, 0x9f, 0x55, 0xc1,
|
|
0x2c, 0x8a, 0x7d, 0x81, 0x5f, 0x75, 0x36, 0xea,
|
|
})
|
|
|
|
// genesisMerkleRoot is the hash of the first transaction in the genesis block
|
|
// for the main network.
|
|
var genesisMerkleRoot = externalapi.NewDomainHashFromByteArray(&[externalapi.DomainHashSize]byte{
|
|
0xca, 0xed, 0xaf, 0x7d, 0x4a, 0x08, 0xbb, 0xe8,
|
|
0x90, 0x11, 0x64, 0x0c, 0x48, 0x41, 0xb6, 0x6d,
|
|
0x5b, 0xba, 0x67, 0xd7, 0x28, 0x8c, 0xe6, 0xd6,
|
|
0x72, 0x28, 0xdb, 0x00, 0x09, 0x66, 0xe9, 0x74,
|
|
})
|
|
|
|
// genesisBlock defines the genesis block of the block DAG which serves as the
|
|
// public transaction ledger for the main network.
|
|
var genesisBlock = externalapi.DomainBlock{
|
|
Header: blockheader.NewImmutableBlockHeader(
|
|
0,
|
|
[]externalapi.BlockLevelParents{},
|
|
genesisMerkleRoot,
|
|
&externalapi.DomainHash{},
|
|
externalapi.NewDomainHashFromByteArray(muhash.EmptyMuHashHash.AsArray()),
|
|
0x17cfb020c02,
|
|
0x1e7fffff,
|
|
0x3392c,
|
|
0,
|
|
0,
|
|
big.NewInt(0),
|
|
&externalapi.DomainHash{},
|
|
),
|
|
Transactions: []*externalapi.DomainTransaction{genesisCoinbaseTx},
|
|
}
|
|
|
|
var devnetGenesisTxOuts = []*externalapi.DomainTransactionOutput{}
|
|
|
|
var devnetGenesisTxPayload = []byte{
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // Blue score
|
|
0x00, 0xE1, 0xF5, 0x05, 0x00, 0x00, 0x00, 0x00, // Subsidy
|
|
0x00, 0x00, // Script version
|
|
0x01, // Varint
|
|
0x00, // OP-FALSE
|
|
0x6b, 0x61, 0x73, 0x70, 0x61, 0x2d, 0x64, 0x65, 0x76, 0x6e, 0x65, 0x74, // kaspa-devnet
|
|
}
|
|
|
|
// devnetGenesisCoinbaseTx is the coinbase transaction for the genesis blocks for
|
|
// the development network.
|
|
var devnetGenesisCoinbaseTx = transactionhelper.NewSubnetworkTransaction(0,
|
|
[]*externalapi.DomainTransactionInput{}, devnetGenesisTxOuts,
|
|
&subnetworks.SubnetworkIDCoinbase, 0, devnetGenesisTxPayload)
|
|
|
|
// devGenesisHash is the hash of the first block in the block DAG for the development
|
|
// network (genesis block).
|
|
var devnetGenesisHash = externalapi.NewDomainHashFromByteArray(&[externalapi.DomainHashSize]byte{
|
|
0x34, 0x3b, 0x53, 0xb7, 0xad, 0x82, 0x8a, 0x33,
|
|
0x4b, 0x03, 0xb1, 0xbb, 0x13, 0x15, 0xb0, 0x75,
|
|
0xf3, 0xf0, 0x40, 0xfb, 0x64, 0x0c, 0xca, 0x19,
|
|
0xc9, 0x61, 0xe7, 0x69, 0xdb, 0x98, 0x98, 0x3e,
|
|
})
|
|
|
|
// devnetGenesisMerkleRoot is the hash of the first transaction in the genesis block
|
|
// for the devopment network.
|
|
var devnetGenesisMerkleRoot = externalapi.NewDomainHashFromByteArray(&[externalapi.DomainHashSize]byte{
|
|
0x58, 0xab, 0xf2, 0x03, 0x21, 0xd7, 0x07, 0x16,
|
|
0x16, 0x2b, 0x6b, 0xf8, 0xd9, 0xf5, 0x89, 0xca,
|
|
0x33, 0xae, 0x6e, 0x32, 0xb3, 0xb1, 0x9a, 0xbb,
|
|
0x7f, 0xa6, 0x5d, 0x11, 0x41, 0xa3, 0xf9, 0x4d,
|
|
})
|
|
|
|
// devnetGenesisBlock defines the genesis block of the block DAG which serves as the
|
|
// public transaction ledger for the development network.
|
|
var devnetGenesisBlock = externalapi.DomainBlock{
|
|
Header: blockheader.NewImmutableBlockHeader(
|
|
0,
|
|
[]externalapi.BlockLevelParents{},
|
|
devnetGenesisMerkleRoot,
|
|
&externalapi.DomainHash{},
|
|
externalapi.NewDomainHashFromByteArray(muhash.EmptyMuHashHash.AsArray()),
|
|
0x11e9db49828,
|
|
0x1e7fffff,
|
|
0x48e5e,
|
|
0,
|
|
0,
|
|
big.NewInt(0),
|
|
&externalapi.DomainHash{},
|
|
),
|
|
Transactions: []*externalapi.DomainTransaction{devnetGenesisCoinbaseTx},
|
|
}
|
|
|
|
var simnetGenesisTxOuts = []*externalapi.DomainTransactionOutput{}
|
|
|
|
var simnetGenesisTxPayload = []byte{
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // Blue score
|
|
0x00, 0xE1, 0xF5, 0x05, 0x00, 0x00, 0x00, 0x00, // Subsidy
|
|
0x00, 0x00, // Script version
|
|
0x01, // Varint
|
|
0x00, // OP-FALSE
|
|
0x6b, 0x61, 0x73, 0x70, 0x61, 0x2d, 0x73, 0x69, 0x6d, 0x6e, 0x65, 0x74, // kaspa-simnet
|
|
}
|
|
|
|
// simnetGenesisCoinbaseTx is the coinbase transaction for the simnet genesis block.
|
|
var simnetGenesisCoinbaseTx = transactionhelper.NewSubnetworkTransaction(0,
|
|
[]*externalapi.DomainTransactionInput{}, simnetGenesisTxOuts,
|
|
&subnetworks.SubnetworkIDCoinbase, 0, simnetGenesisTxPayload)
|
|
|
|
// simnetGenesisHash is the hash of the first block in the block DAG for
|
|
// the simnet (genesis block).
|
|
var simnetGenesisHash = externalapi.NewDomainHashFromByteArray(&[externalapi.DomainHashSize]byte{
|
|
0x41, 0x1f, 0x8c, 0xd2, 0x6f, 0x3d, 0x41, 0xae,
|
|
0xa3, 0x9e, 0x78, 0x57, 0x39, 0x27, 0xda, 0x24,
|
|
0xd2, 0x39, 0x95, 0x70, 0x5b, 0x57, 0x9f, 0x30,
|
|
0x95, 0x9b, 0x91, 0x27, 0xe9, 0x6b, 0x79, 0xe3,
|
|
})
|
|
|
|
// simnetGenesisMerkleRoot is the hash of the first transaction in the genesis block
|
|
// for the devopment network.
|
|
var simnetGenesisMerkleRoot = externalapi.NewDomainHashFromByteArray(&[externalapi.DomainHashSize]byte{
|
|
0x19, 0x46, 0xd6, 0x29, 0xf7, 0xe9, 0x22, 0xa7,
|
|
0xbc, 0xed, 0x59, 0x19, 0x05, 0x21, 0xc3, 0x77,
|
|
0x1f, 0x73, 0xd3, 0x52, 0xdd, 0xbb, 0xb6, 0x86,
|
|
0x56, 0x4a, 0xd7, 0xfd, 0x56, 0x85, 0x7c, 0x1b,
|
|
})
|
|
|
|
// simnetGenesisBlock defines the genesis block of the block DAG which serves as the
|
|
// public transaction ledger for the development network.
|
|
var simnetGenesisBlock = externalapi.DomainBlock{
|
|
Header: blockheader.NewImmutableBlockHeader(
|
|
0,
|
|
[]externalapi.BlockLevelParents{},
|
|
simnetGenesisMerkleRoot,
|
|
&externalapi.DomainHash{},
|
|
externalapi.NewDomainHashFromByteArray(muhash.EmptyMuHashHash.AsArray()),
|
|
0x17c5f62fbb6,
|
|
0x207fffff,
|
|
0x2,
|
|
0,
|
|
0,
|
|
big.NewInt(0),
|
|
&externalapi.DomainHash{},
|
|
),
|
|
Transactions: []*externalapi.DomainTransaction{simnetGenesisCoinbaseTx},
|
|
}
|
|
|
|
var testnetGenesisTxOuts = []*externalapi.DomainTransactionOutput{}
|
|
|
|
var testnetGenesisTxPayload = []byte{
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // Blue score
|
|
0x00, 0xE1, 0xF5, 0x05, 0x00, 0x00, 0x00, 0x00, // Subsidy
|
|
0x00, 0x00, // Script version
|
|
0x01, // Varint
|
|
0x00, // OP-FALSE
|
|
0x6b, 0x61, 0x73, 0x70, 0x61, 0x2d, 0x74, 0x65, 0x73, 0x74, 0x6e, 0x65, 0x74, // kaspa-testnet
|
|
}
|
|
|
|
// testnetGenesisCoinbaseTx is the coinbase transaction for the testnet genesis block.
|
|
var testnetGenesisCoinbaseTx = transactionhelper.NewSubnetworkTransaction(0,
|
|
[]*externalapi.DomainTransactionInput{}, testnetGenesisTxOuts,
|
|
&subnetworks.SubnetworkIDCoinbase, 0, testnetGenesisTxPayload)
|
|
|
|
// testnetGenesisHash is the hash of the first block in the block DAG for the test
|
|
// network (genesis block).
|
|
var testnetGenesisHash = externalapi.NewDomainHashFromByteArray(&[externalapi.DomainHashSize]byte{
|
|
0xf8, 0x96, 0xa3, 0x03, 0x48, 0x73, 0xbe, 0x17,
|
|
0x39, 0xfc, 0x43, 0x59, 0x23, 0x68, 0x99, 0xfd,
|
|
0x3d, 0x65, 0xd2, 0xbc, 0x94, 0xf9, 0x78, 0x0d,
|
|
0xf0, 0xd0, 0xda, 0x3e, 0xb1, 0xcc, 0x43, 0x70,
|
|
})
|
|
|
|
// testnetGenesisMerkleRoot is the hash of the first transaction in the genesis block
|
|
// for testnet.
|
|
var testnetGenesisMerkleRoot = externalapi.NewDomainHashFromByteArray(&[externalapi.DomainHashSize]byte{
|
|
0x17, 0x34, 0x14, 0x08, 0xa5, 0x72, 0x45, 0x56,
|
|
0x50, 0x4d, 0xf4, 0xd6, 0xcf, 0x51, 0x5c, 0xbf,
|
|
0xbb, 0x22, 0x04, 0x30, 0xdc, 0x45, 0x1c, 0x74,
|
|
0x3c, 0x22, 0xd5, 0xe9, 0x11, 0x72, 0x0c, 0x2a,
|
|
})
|
|
|
|
// testnetGenesisBlock defines the genesis block of the block DAG which serves as the
|
|
// public transaction ledger for testnet.
|
|
var testnetGenesisBlock = externalapi.DomainBlock{
|
|
Header: blockheader.NewImmutableBlockHeader(
|
|
0,
|
|
[]externalapi.BlockLevelParents{},
|
|
testnetGenesisMerkleRoot,
|
|
&externalapi.DomainHash{},
|
|
externalapi.NewDomainHashFromByteArray(muhash.EmptyMuHashHash.AsArray()),
|
|
0x17c5f62fbb6,
|
|
0x1e7fffff,
|
|
0x14582,
|
|
0,
|
|
0,
|
|
big.NewInt(0),
|
|
&externalapi.DomainHash{},
|
|
),
|
|
Transactions: []*externalapi.DomainTransaction{testnetGenesisCoinbaseTx},
|
|
}
|