mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
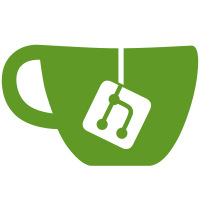
* Implement GHOST. * Implement TestGHOST. * Make GHOST() take arbitrary subDAGs. * Hold RootHashes in SubDAG rather than one GenesisHash. * Select which root the GHOST chain starts with instead of passing a lowHash. * If two child hashes have the same future size, decide which one is larger using the block hash. * Extract blockHashWithLargestFutureSize to a separate function. * Calculate future size for each block individually. * Make TestGHOST deterministic. * Increase the timeout for connecting 128 connections in TestRPCMaxInboundConnections. * Implement BenchmarkGHOST. * Fix an infinite loop. * Use much larger benchmark data. * Optimize `futureSizes` using reverse merge sets. * Temporarily make the benchmark data smaller while GHOST is being optimized. * Fix a bug in futureSizes. * Fix a bug in populateReverseMergeSet. * Choose a selectedChild at random instead of the one with the largest reverse merge set size. * Rename populateReverseMergeSet to calculateReverseMergeSet. * Use reachability to resolve isDescendantOf. * Extract heightMaps to a separate object. * Iterate using height maps in futureSizes. * Don't store reverse merge sets in memory. * Change calculateReverseMergeSet to calculateReverseMergeSetSize. * Fix bad initial reverseMergeSetSize. * Optimize calculateReverseMergeSetSize. * Enlarge the benchmark data to 86k blocks.
84 lines
2.1 KiB
Go
84 lines
2.1 KiB
Go
package integration
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/infrastructure/network/netadapter/server/grpcserver"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/kaspanet/kaspad/infrastructure/network/rpcclient"
|
|
)
|
|
|
|
const rpcTimeout = 10 * time.Second
|
|
|
|
type testRPCClient struct {
|
|
*rpcclient.RPCClient
|
|
}
|
|
|
|
func newTestRPCClient(rpcAddress string) (*testRPCClient, error) {
|
|
rpcClient, err := rpcclient.NewRPCClient(rpcAddress)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
rpcClient.SetTimeout(rpcTimeout)
|
|
|
|
return &testRPCClient{
|
|
RPCClient: rpcClient,
|
|
}, nil
|
|
}
|
|
|
|
func TestRPCMaxInboundConnections(t *testing.T) {
|
|
harness, teardown := setupHarness(t, &harnessParams{
|
|
p2pAddress: p2pAddress1,
|
|
rpcAddress: rpcAddress1,
|
|
miningAddress: miningAddress1,
|
|
miningAddressPrivateKey: miningAddress1PrivateKey,
|
|
})
|
|
defer teardown()
|
|
|
|
// Close the default RPC client so that it won't interfere with the test
|
|
err := harness.rpcClient.Close()
|
|
if err != nil {
|
|
t.Fatalf("Failed to close the default harness RPCClient: %s", err)
|
|
}
|
|
|
|
// Connect `RPCMaxInboundConnections` clients. We expect this to succeed immediately
|
|
rpcClients := []*testRPCClient{}
|
|
doneChan := make(chan error)
|
|
go func() {
|
|
for i := 0; i < grpcserver.RPCMaxInboundConnections; i++ {
|
|
rpcClient, err := newTestRPCClient(harness.rpcAddress)
|
|
if err != nil {
|
|
doneChan <- err
|
|
}
|
|
rpcClients = append(rpcClients, rpcClient)
|
|
}
|
|
doneChan <- nil
|
|
}()
|
|
select {
|
|
case err = <-doneChan:
|
|
if err != nil {
|
|
t.Fatalf("newTestRPCClient: %s", err)
|
|
}
|
|
case <-time.After(time.Second * 5):
|
|
t.Fatalf("Timeout for connecting %d RPC connections elapsed", grpcserver.RPCMaxInboundConnections)
|
|
}
|
|
|
|
// Try to connect another client. We expect this to fail
|
|
// We set a timeout to account for reconnection mechanisms
|
|
go func() {
|
|
rpcClient, err := newTestRPCClient(harness.rpcAddress)
|
|
if err != nil {
|
|
doneChan <- err
|
|
}
|
|
rpcClients = append(rpcClients, rpcClient)
|
|
doneChan <- nil
|
|
}()
|
|
select {
|
|
case err = <-doneChan:
|
|
if err == nil {
|
|
t.Fatalf("newTestRPCClient unexpectedly succeeded")
|
|
}
|
|
case <-time.After(time.Second * 15):
|
|
}
|
|
}
|