mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
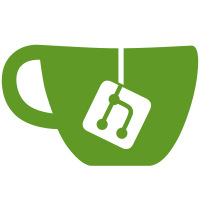
* Add boilerplate for the `parse` sub command. * Deserialize the given transaction hax. * Implement the rest of the wallet parse command. * Hide transaction inputs behind a `verbose` flag. * Indicate that we aren't able to extract an address out of a nonstandard transaction. Co-authored-by: Ori Newman <orinewman1@gmail.com>
40 lines
1.0 KiB
Go
40 lines
1.0 KiB
Go
package main
|
|
|
|
import "github.com/pkg/errors"
|
|
|
|
func main() {
|
|
subCmd, config := parseCommandLine()
|
|
|
|
var err error
|
|
switch subCmd {
|
|
case createSubCmd:
|
|
err = create(config.(*createConfig))
|
|
case balanceSubCmd:
|
|
err = balance(config.(*balanceConfig))
|
|
case sendSubCmd:
|
|
err = send(config.(*sendConfig))
|
|
case createUnsignedTransactionSubCmd:
|
|
err = createUnsignedTransaction(config.(*createUnsignedTransactionConfig))
|
|
case signSubCmd:
|
|
err = sign(config.(*signConfig))
|
|
case broadcastSubCmd:
|
|
err = broadcast(config.(*broadcastConfig))
|
|
case parseSubCmd:
|
|
err = parse(config.(*parseConfig))
|
|
case showAddressesSubCmd:
|
|
err = showAddresses(config.(*showAddressesConfig))
|
|
case newAddressSubCmd:
|
|
err = newAddress(config.(*newAddressConfig))
|
|
case dumpUnencryptedDataSubCmd:
|
|
err = dumpUnencryptedData(config.(*dumpUnencryptedDataConfig))
|
|
case startDaemonSubCmd:
|
|
err = startDaemon(config.(*startDaemonConfig))
|
|
default:
|
|
err = errors.Errorf("Unknown sub-command '%s'\n", subCmd)
|
|
}
|
|
|
|
if err != nil {
|
|
printErrorAndExit(err)
|
|
}
|
|
}
|