mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
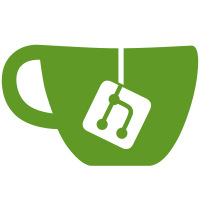
* Remove Subsystems map and replace with RegisterSubSystem * Clean up the logger * Fix LOGFLAGS and make LongFile work correctly * Parallelize the logger backend * More logger cleanup * Initialize and close the logger backend wherever it's needed * Move the location where the backend is closed, also print the log if it panics while writing * Add TestMain to reachability manager tests to preserve the same log level * Fix review comments Co-authored-by: Svarog <feanorr@gmail.com>
55 lines
1.3 KiB
Go
55 lines
1.3 KiB
Go
package logger
|
|
|
|
import "strings"
|
|
|
|
// Level is the level at which a logger is configured. All messages sent
|
|
// to a level which is below the current level are filtered.
|
|
type Level uint32
|
|
|
|
// Level constants.
|
|
const (
|
|
LevelTrace Level = iota
|
|
LevelDebug
|
|
LevelInfo
|
|
LevelWarn
|
|
LevelError
|
|
LevelCritical
|
|
LevelOff
|
|
)
|
|
|
|
// levelStrs defines the human-readable names for each logging level.
|
|
var levelStrs = [...]string{"TRC", "DBG", "INF", "WRN", "ERR", "CRT", "OFF"}
|
|
|
|
// LevelFromString returns a level based on the input string s. If the input
|
|
// can't be interpreted as a valid log level, the info level and false is
|
|
// returned.
|
|
func LevelFromString(s string) (l Level, ok bool) {
|
|
switch strings.ToLower(s) {
|
|
case "trace", "trc":
|
|
return LevelTrace, true
|
|
case "debug", "dbg":
|
|
return LevelDebug, true
|
|
case "info", "inf":
|
|
return LevelInfo, true
|
|
case "warn", "wrn":
|
|
return LevelWarn, true
|
|
case "error", "err":
|
|
return LevelError, true
|
|
case "critical", "crt":
|
|
return LevelCritical, true
|
|
case "off":
|
|
return LevelOff, true
|
|
default:
|
|
return LevelInfo, false
|
|
}
|
|
}
|
|
|
|
// String returns the tag of the logger used in log messages, or "OFF" if
|
|
// the level will not produce any log output.
|
|
func (l Level) String() string {
|
|
if l >= LevelOff {
|
|
return "OFF"
|
|
}
|
|
return levelStrs[l]
|
|
}
|