mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-05 20:32:31 +00:00
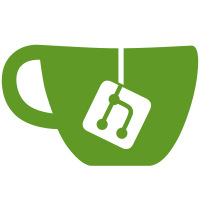
* Add a reconnect mechanism to RPCClient. * Fix Reconnect(). * Connect the internal reconnection logic to the miner reconnection logic. * Rename shouldReconnect to isClosed. * Move safe reconnection logic from the miner to rpcclient. * Remove sleep from HandleSubmitBlock. * Properly handle client errors and only disconnect if we're already connected. * Make go lint happy. Co-authored-by: Ori Newman <orinewman1@gmail.com>
61 lines
1.3 KiB
Go
61 lines
1.3 KiB
Go
package main
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
"github.com/kaspanet/kaspad/infrastructure/logger"
|
|
"github.com/kaspanet/kaspad/infrastructure/network/rpcclient"
|
|
"github.com/pkg/errors"
|
|
"time"
|
|
)
|
|
|
|
const minerTimeout = 10 * time.Second
|
|
|
|
type minerClient struct {
|
|
*rpcclient.RPCClient
|
|
|
|
cfg *configFlags
|
|
blockAddedNotificationChan chan struct{}
|
|
}
|
|
|
|
func (mc *minerClient) connect() error {
|
|
rpcAddress, err := mc.cfg.NetParams().NormalizeRPCServerAddress(mc.cfg.RPCServer)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
rpcClient, err := rpcclient.NewRPCClient(rpcAddress)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
mc.RPCClient = rpcClient
|
|
mc.SetTimeout(minerTimeout)
|
|
mc.SetLogger(backendLog, logger.LevelTrace)
|
|
|
|
err = mc.RegisterForBlockAddedNotifications(func(_ *appmessage.BlockAddedNotificationMessage) {
|
|
select {
|
|
case mc.blockAddedNotificationChan <- struct{}{}:
|
|
default:
|
|
}
|
|
})
|
|
if err != nil {
|
|
return errors.Wrapf(err, "error requesting block-added notifications")
|
|
}
|
|
|
|
log.Infof("Connected to %s", rpcAddress)
|
|
|
|
return nil
|
|
}
|
|
|
|
func newMinerClient(cfg *configFlags) (*minerClient, error) {
|
|
minerClient := &minerClient{
|
|
cfg: cfg,
|
|
blockAddedNotificationChan: make(chan struct{}),
|
|
}
|
|
|
|
err := minerClient.connect()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return minerClient, nil
|
|
}
|