mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
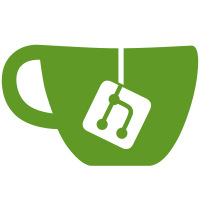
* '' * '' * '' * Changes genesis block version to 0. * a * a * All tests are done. * All tests passed for changed block version from int32 to uint16 * Adds validation of rejecting blocks with unknown versions. * Changes txn version from int32 to uint16. * . * Adds comments to exported functions. * Change functions name from ConvertFromRpcScriptPubKeyToRPCScriptPubKey to ConvertFromAppMsgRPCScriptPubKeyToRPCScriptPubKey and from ConvertFromRPCScriptPubKeyToRpcScriptPubKey to ConvertFromRPCScriptPubKeyToAppMsgRPCScriptPubKey * change comment to "ScriptPublicKey represents a Kaspad ScriptPublicKey" * delete part (tx.Version < 0) that cannot be exist on the if statement. * Revert protobuf version. * Fix a comment. * Fix a comment. * Rename a variable. * Rename a variable. * Remove a const. * Rename a type. * Rename a field. * Rename a field. * Remove commented-out code. * Remove dangerous nil case in DomainTransactionOutput.Clone(). * Remove a constant. * Fix a string. * Fix wrong totalScriptPubKeySize in transactionMassStandalonePart. * Remove a constant. * Remove an unused error. * Fix a serialization error. * Specify version types to be uint16 explicitly. * Use constants.ScriptPublicKeyVersion. * Fix a bad test. * Remove some whitespace. * Add a case to utxoEntry.Equal(). * Rename scriptPubKey to scriptPublicKey. * Remove a TODO. * Rename constants. * Rename a variable. * Add version to parseShortForm. Co-authored-by: tal <tal@daglabs.com> Co-authored-by: stasatdaglabs <stas@daglabs.com>
44 lines
1.6 KiB
Go
44 lines
1.6 KiB
Go
package utxoindex
|
|
|
|
import (
|
|
"encoding/binary"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
// ScriptPublicKeyString is a script public key represented as a string
|
|
// We use this type rather than just a byte slice because Go maps don't
|
|
// support slices as keys. See: UTXOChanges
|
|
type ScriptPublicKeyString string
|
|
|
|
// UTXOOutpointEntryPairs is a map between UTXO outpoints to UTXO entries
|
|
type UTXOOutpointEntryPairs map[externalapi.DomainOutpoint]externalapi.UTXOEntry
|
|
|
|
// UTXOOutpoints is a set of UTXO outpoints
|
|
type UTXOOutpoints map[externalapi.DomainOutpoint]interface{}
|
|
|
|
// UTXOChanges is the set of changes made to the UTXO index after
|
|
// a successful update
|
|
type UTXOChanges struct {
|
|
Added map[ScriptPublicKeyString]UTXOOutpointEntryPairs
|
|
Removed map[ScriptPublicKeyString]UTXOOutpoints
|
|
}
|
|
|
|
// ConvertScriptPublicKeyToString converts the given scriptPublicKey to a string
|
|
func ConvertScriptPublicKeyToString(scriptPublicKey *externalapi.ScriptPublicKey) ScriptPublicKeyString {
|
|
var versionBytes = make([]byte, 2) // uint16
|
|
binary.LittleEndian.PutUint16(versionBytes, scriptPublicKey.Version)
|
|
versionString := ScriptPublicKeyString(versionBytes)
|
|
scriptString := ScriptPublicKeyString(scriptPublicKey.Script)
|
|
return versionString + scriptString
|
|
|
|
}
|
|
|
|
// ConvertStringToScriptPublicKey converts the given string to a scriptPublicKey
|
|
func ConvertStringToScriptPublicKey(string ScriptPublicKeyString) *externalapi.ScriptPublicKey {
|
|
bytes := []byte(string)
|
|
version := binary.LittleEndian.Uint16(bytes[:2])
|
|
script := bytes[2:]
|
|
return &externalapi.ScriptPublicKey{Script: script, Version: version}
|
|
|
|
}
|