mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-23 15:26:42 +00:00
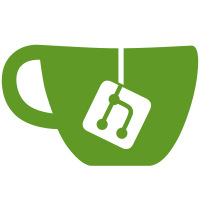
* [NOD-1551] Make UTXO-Diff implemented fully in utils/utxo * [NOD-1551] Fixes everywhere except database * [NOD-1551] Fix database * [NOD-1551] Add comments * [NOD-1551] Partial commit * [NOD-1551] Comlete making UTXOEntry immutable + don't clone it in UTXOCollectionClone * [NOD-1551] Rename ToUnmutable -> ToImmutable * [NOD-1551] Track immutable references generated from mutable UTXODiff, and invalidate them if the mutable one changed * [NOD-1551] Clone scriptPubKey in NewUTXOEntry * [NOD-1551] Remove redundant code * [NOD-1551] Remove redundant call for .CloneMutable and then .ToImmutable * [NOD-1551] Make utxoEntry pointert-receiver + clone ScriptPubKey in getter
36 lines
1.0 KiB
Go
36 lines
1.0 KiB
Go
package consensusstatestore
|
|
|
|
import (
|
|
"github.com/golang/protobuf/proto"
|
|
"github.com/kaspanet/kaspad/domain/consensus/database/serialization"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
func serializeOutpoint(outpoint *externalapi.DomainOutpoint) ([]byte, error) {
|
|
return proto.Marshal(serialization.DomainOutpointToDbOutpoint(outpoint))
|
|
}
|
|
|
|
func serializeUTXOEntry(entry externalapi.UTXOEntry) ([]byte, error) {
|
|
return proto.Marshal(serialization.UTXOEntryToDBUTXOEntry(entry))
|
|
}
|
|
|
|
func deserializeOutpoint(outpointBytes []byte) (*externalapi.DomainOutpoint, error) {
|
|
dbOutpoint := &serialization.DbOutpoint{}
|
|
err := proto.Unmarshal(outpointBytes, dbOutpoint)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return serialization.DbOutpointToDomainOutpoint(dbOutpoint)
|
|
}
|
|
|
|
func deserializeUTXOEntry(entryBytes []byte) (externalapi.UTXOEntry, error) {
|
|
dbEntry := &serialization.DbUtxoEntry{}
|
|
err := proto.Unmarshal(entryBytes, dbEntry)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return serialization.DBUTXOEntryToUTXOEntry(dbEntry), nil
|
|
}
|