mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
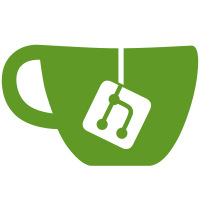
* [DEV-242] Modified some help functionality to convert to lowercase camel case instead of just lowercase. * [DEV-242] Corrected help functionality for struct field names. * [DEV-242] Corrected help functionality for struct names. * [DEV-242] Cleaned up toLowercaseCamelCase. * [DEV-242] Renamed toLowercaseCamelCase to toCamelCase. * [DEV-242] Converted the rest of the stuff in rpcserverhelp.go to camelCase. Fixed a bug in the camelCase converter. * [DEV-242] camelCase-ified the last few RPC parameter names. * [DEV-242] Fixed an off-by-one bug in toCamelCase. * [DEV-242] Changed back from "jsonRpc" to "jsonrpc". * [DEV-242] Moved toCamelCase into utils, wrote unit tests for it, and fixed an off-by-one bug. * [DEV-242] Re-exported DefaultHomeDir because it's required in windows_service.go. * [DEV-242] Added a comment above DefaultHomeDir to satisfy golint. * [DEV-242] Formatted config/config.go.
62 lines
1.5 KiB
Go
62 lines
1.5 KiB
Go
package util
|
|
|
|
import "testing"
|
|
|
|
// TestToCamelCase tests whether ToCamelCase correctly converts camelCase-ish strings to camelCase.
|
|
func TestToCamelCase(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
input string
|
|
expectedResult string
|
|
}{
|
|
{
|
|
name: "single word that's already in camelCase",
|
|
input: "abc",
|
|
expectedResult: "abc",
|
|
},
|
|
{
|
|
name: "single word in PascalCase",
|
|
input: "Abc",
|
|
expectedResult: "abc",
|
|
},
|
|
{
|
|
name: "single word in all caps",
|
|
input: "ABC",
|
|
expectedResult: "abc",
|
|
},
|
|
{
|
|
name: "multiple words that are already in camelCase",
|
|
input: "aaaBbbCcc",
|
|
expectedResult: "aaaBbbCcc",
|
|
},
|
|
{
|
|
name: "multiple words in PascalCase",
|
|
input: "AaaBbbCcc",
|
|
expectedResult: "aaaBbbCcc",
|
|
},
|
|
{
|
|
name: "acronym in start position",
|
|
input: "AAABbbCcc",
|
|
expectedResult: "aaaBbbCcc",
|
|
},
|
|
{
|
|
name: "acronym in middle position",
|
|
input: "aaaBBBCcc",
|
|
expectedResult: "aaaBbbCcc",
|
|
},
|
|
{
|
|
name: "acronym in end position",
|
|
input: "aaaBbbCCC",
|
|
expectedResult: "aaaBbbCcc",
|
|
},
|
|
}
|
|
|
|
for _, test := range tests {
|
|
result := ToCamelCase(test.input)
|
|
if result != test.expectedResult {
|
|
t.Errorf("ToCamelCase for test \"%s\" returned an unexpected result. "+
|
|
"Expected: \"%s\", got: \"%s\"", test.name, test.expectedResult, result)
|
|
}
|
|
}
|
|
}
|