mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-23 15:26:42 +00:00
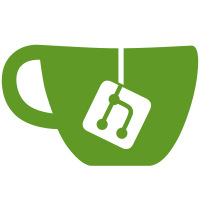
* [NOD-1551] Make UTXO-Diff implemented fully in utils/utxo * [NOD-1551] Fixes everywhere except database * [NOD-1551] Fix database * [NOD-1551] Add comments * [NOD-1551] Partial commit * [NOD-1551] Comlete making UTXOEntry immutable + don't clone it in UTXOCollectionClone * [NOD-1551] Rename ToUnmutable -> ToImmutable * [NOD-1551] Track immutable references generated from mutable UTXODiff, and invalidate them if the mutable one changed * [NOD-1551] Clone scriptPubKey in NewUTXOEntry * [NOD-1551] Remove redundant code * [NOD-1551] Remove redundant call for .CloneMutable and then .ToImmutable * [NOD-1551] Make utxoEntry pointert-receiver + clone ScriptPubKey in getter
41 lines
1.1 KiB
Go
41 lines
1.1 KiB
Go
package serialization
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/utxo"
|
|
)
|
|
|
|
func utxoCollectionToDBUTXOCollection(utxoCollection model.UTXOCollection) ([]*DbUtxoCollectionItem, error) {
|
|
items := make([]*DbUtxoCollectionItem, utxoCollection.Len())
|
|
i := 0
|
|
utxoIterator := utxoCollection.Iterator()
|
|
for utxoIterator.Next() {
|
|
outpoint, entry, err := utxoIterator.Get()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
items[i] = &DbUtxoCollectionItem{
|
|
Outpoint: DomainOutpointToDbOutpoint(outpoint),
|
|
UtxoEntry: UTXOEntryToDBUTXOEntry(entry),
|
|
}
|
|
i++
|
|
}
|
|
|
|
return items, nil
|
|
}
|
|
|
|
func dbUTXOCollectionToUTXOCollection(items []*DbUtxoCollectionItem) (model.UTXOCollection, error) {
|
|
utxoMap := make(map[externalapi.DomainOutpoint]externalapi.UTXOEntry, len(items))
|
|
for _, item := range items {
|
|
outpoint, err := DbOutpointToDomainOutpoint(item.Outpoint)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
utxoMap[*outpoint] = DBUTXOEntryToUTXOEntry(item.UtxoEntry)
|
|
}
|
|
return utxo.NewUTXOCollection(utxoMap), nil
|
|
}
|