mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-23 15:26:42 +00:00
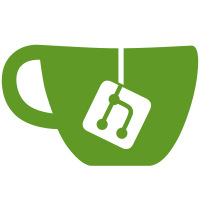
* Increase size of reachability cache * Change DomainHash to struct with unexported hashArray * Fixing compilation errors stemming from new DomainHash structure * Remove obsolete Read/WriteElement methods in appmessage * Fix all tests * Fix all tests * Add comments * A few renamings * go mod tidy
80 lines
2.3 KiB
Go
80 lines
2.3 KiB
Go
package externalapi
|
|
|
|
import (
|
|
"testing"
|
|
)
|
|
|
|
type testHashToCompare struct {
|
|
hash *DomainHash
|
|
expectedResult bool
|
|
}
|
|
|
|
type testHashStruct struct {
|
|
baseHash *DomainHash
|
|
hashesToCompareTo []testHashToCompare
|
|
}
|
|
|
|
func initTestDomainHashForEqual() []*testHashStruct {
|
|
tests := []*testHashStruct{
|
|
{
|
|
baseHash: nil,
|
|
hashesToCompareTo: []testHashToCompare{
|
|
{
|
|
hash: nil,
|
|
expectedResult: true,
|
|
}, {
|
|
hash: NewDomainHashFromByteArray(&[DomainHashSize]byte{
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}),
|
|
expectedResult: false,
|
|
},
|
|
},
|
|
}, {
|
|
baseHash: NewDomainHashFromByteArray(&[DomainHashSize]byte{
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF}),
|
|
hashesToCompareTo: []testHashToCompare{
|
|
{
|
|
hash: nil,
|
|
expectedResult: false,
|
|
}, {
|
|
hash: NewDomainHashFromByteArray(&[DomainHashSize]byte{
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}),
|
|
expectedResult: false,
|
|
}, {
|
|
hash: NewDomainHashFromByteArray(&[DomainHashSize]byte{
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
|
|
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF}),
|
|
expectedResult: true,
|
|
},
|
|
},
|
|
},
|
|
}
|
|
return tests
|
|
}
|
|
|
|
func TestDomainHash_Equal(t *testing.T) {
|
|
hashTests := initTestDomainHashForEqual()
|
|
for i, test := range hashTests {
|
|
for j, subTest := range test.hashesToCompareTo {
|
|
result1 := test.baseHash.Equal(subTest.hash)
|
|
if result1 != subTest.expectedResult {
|
|
t.Fatalf("Test #%d:%d: Expected %t but got %t", i, j, subTest.expectedResult, result1)
|
|
}
|
|
result2 := subTest.hash.Equal(test.baseHash)
|
|
if result2 != subTest.expectedResult {
|
|
t.Fatalf("Test #%d:%d: Expected %t but got %t", i, j, subTest.expectedResult, result2)
|
|
}
|
|
}
|
|
}
|
|
}
|