mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-25 00:06:49 +00:00
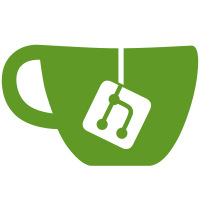
* Increase size of reachability cache * Change DomainHash to struct with unexported hashArray * Fixing compilation errors stemming from new DomainHash structure * Remove obsolete Read/WriteElement methods in appmessage * Fix all tests * Fix all tests * Add comments * A few renamings * go mod tidy
32 lines
673 B
Go
32 lines
673 B
Go
package hashes
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
)
|
|
|
|
// cmp compares two hashes and returns:
|
|
//
|
|
// -1 if a < b
|
|
// 0 if a == b
|
|
// +1 if a > b
|
|
//
|
|
func cmp(a, b *externalapi.DomainHash) int {
|
|
aBytes := a.ByteArray()
|
|
bBytes := b.ByteArray()
|
|
// We compare the hashes backwards because Hash is stored as a little endian byte array.
|
|
for i := externalapi.DomainHashSize - 1; i >= 0; i-- {
|
|
switch {
|
|
case aBytes[i] < bBytes[i]:
|
|
return -1
|
|
case aBytes[i] > bBytes[i]:
|
|
return 1
|
|
}
|
|
}
|
|
return 0
|
|
}
|
|
|
|
// Less returns true iff hash a is less than hash b
|
|
func Less(a, b *externalapi.DomainHash) bool {
|
|
return cmp(a, b) < 0
|
|
}
|