mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-09 14:22:33 +00:00
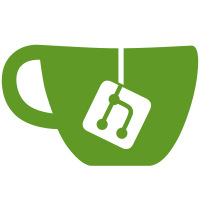
* Replace the old blockSubsidy parameters with the new ones. * Return subsidyGenesisReward if blockHash is the genesis hash. * Traverse a block's past for the subsidy calculation. * Partially implement SubsidyStore. * Refer to SubsidyStore from CoinbaseManager. * Wrap calcBlockSubsidy in getBlockSubsidy, which first checks the database. * Fix finalityStore not calling GenerateShardingID. * Implement calculateAveragePastSubsidy. * Implement calculateMergeSetSubsidySum. * Implement calculateSubsidyRandomVariable. * Implement calcBlockSubsidy. * Add a TODO about floats. * Update the calcBlockSubsidy TODO. * Use binary.LittleEndian in calculateSubsidyRandomVariable. * Fix bad range in calculateSubsidyRandomVariable. * Replace float64 with big.Rat everywhere except for subsidyRandomVariable. * Fix a nil dereference. * Use a random walk to approximate the normal distribution. * In order to avoid unsupported fractional results from powInt64, flip the numerator and the denominator manually. * Set standardDeviation to 0.25, MaxSompi to 10_000_000_000 * SompiPerKaspa and defaultSubsidyGenesisReward to 1_000. * Set the standard deviation to 0.2. * Use a binomial distribution instead of trying to estimate the normal distribution. * Change some values around. * Clamp the block subsidy. * Remove the fake duplicate constants in the util package. * Reduce MaxSompi to only 100m Kaspa to avoid hitting the uint64 ceiling. * Lower MaxSompi further to avoid new and exciting ways for the uint64 ceiling to be hit. * Remove debug logs. * Fix a couple of failing tests. * Fix TestBlockWindow. * Fix limitTransactionCount sometimes crashing on index-out-of-bounds. * In TrustedDataDataDAABlock, replace BlockHeader with DomainBlock * In calculateAveragePastSubsidy, use blockWindow instead of doing a BFS manually. * Remove the reference to DAGTopologyManager in coinbaseManager. * Add subsidy to the coinbase payload. * Get rid of the subsidy store and extract subsidies out of coinbase transactions. * Keep a blockWindow amount of blocks under the virtual for IBD purposes. * Manually remove the virtual genesis from the merge set. * Fix simnet genesis. * Fix TestPruning. * Fix TestCheckBlockIsNotPruned. * Fix TestBlockWindow. * Fix TestCalculateSignatureHashSchnorr. * Fix TestCalculateSignatureHashECDSA. * Fix serializing the wrong value into the coinbase payload. * Rename coinbaseOutputForBlueBlock to coinbaseOutputAndSubsidyForBlueBlock. * Add a TODO about optimizing trusted data DAA window blocks. * Expand on a comment in TestCheckBlockIsNotPruned. * In calcBlockSubsidy, divide the big.Int numerator by the big.Int denominator instead of converting to float64. * Clarify a comment. * Rename SubsidyMinGenesisReward to MinSubsidy. * Properly handle trusted data blocks in calculateMergeSetSubsidySum. * Use the first two bytes of the selected parent's hash for randomness instead of math/rand. * Restore maxSompi to what it used to be. * Fix TestPruning. * Fix TestAmountCreation. * Fix TestBlockWindow. * Fix TestAmountUnitConversions. * Increase the timeout in many-tips to 30 minutes. * Check coinbase subsidy for every block * Re-rename functions * Use shift instead of powInt64 to determine subsidyRandom Co-authored-by: Ori Newman <orinewman1@gmail.com>
255 lines
4.4 KiB
Protocol Buffer
255 lines
4.4 KiB
Protocol Buffer
syntax = "proto3";
|
|
package protowire;
|
|
|
|
option go_package = "github.com/kaspanet/kaspad/protowire";
|
|
|
|
message RequestAddressesMessage{
|
|
bool includeAllSubnetworks = 1;
|
|
SubnetworkId subnetworkId = 2;
|
|
}
|
|
|
|
message AddressesMessage{
|
|
repeated NetAddress addressList = 1;
|
|
}
|
|
|
|
message NetAddress{
|
|
int64 timestamp = 1;
|
|
bytes ip = 3;
|
|
uint32 port = 4;
|
|
}
|
|
|
|
message SubnetworkId{
|
|
bytes bytes = 1;
|
|
}
|
|
|
|
message TransactionMessage{
|
|
uint32 version = 1;
|
|
repeated TransactionInput inputs = 2;
|
|
repeated TransactionOutput outputs = 3;
|
|
uint64 lockTime = 4;
|
|
SubnetworkId subnetworkId = 5;
|
|
uint64 gas = 6;
|
|
bytes payload = 8;
|
|
}
|
|
|
|
message TransactionInput{
|
|
Outpoint previousOutpoint = 1;
|
|
bytes signatureScript = 2;
|
|
uint64 sequence = 3;
|
|
uint32 sigOpCount = 4;
|
|
}
|
|
|
|
message Outpoint{
|
|
TransactionId transactionId = 1;
|
|
uint32 index = 2;
|
|
}
|
|
|
|
message TransactionId{
|
|
bytes bytes = 1;
|
|
}
|
|
message ScriptPublicKey {
|
|
bytes script = 1;
|
|
uint32 version = 2;
|
|
}
|
|
|
|
message TransactionOutput{
|
|
uint64 value = 1;
|
|
ScriptPublicKey scriptPublicKey = 2;
|
|
}
|
|
|
|
message BlockMessage{
|
|
BlockHeader header = 1;
|
|
repeated TransactionMessage transactions = 2;
|
|
}
|
|
|
|
message BlockHeader{
|
|
uint32 version = 1;
|
|
repeated BlockLevelParents parents = 12;
|
|
Hash hashMerkleRoot = 3;
|
|
Hash acceptedIdMerkleRoot = 4;
|
|
Hash utxoCommitment = 5;
|
|
int64 timestamp = 6;
|
|
uint32 bits = 7;
|
|
uint64 nonce = 8;
|
|
uint64 daaScore = 9;
|
|
bytes blueWork = 10;
|
|
Hash pruningPoint = 14;
|
|
uint64 blueScore = 13;
|
|
}
|
|
|
|
message BlockLevelParents {
|
|
repeated Hash parentHashes = 1;
|
|
}
|
|
|
|
message Hash{
|
|
bytes bytes = 1;
|
|
}
|
|
|
|
message RequestBlockLocatorMessage{
|
|
Hash highHash = 1;
|
|
uint32 limit = 2;
|
|
}
|
|
|
|
message BlockLocatorMessage{
|
|
repeated Hash hashes = 1;
|
|
}
|
|
|
|
message RequestHeadersMessage{
|
|
Hash lowHash = 1;
|
|
Hash highHash = 2;
|
|
}
|
|
|
|
message RequestNextHeadersMessage{
|
|
}
|
|
|
|
message DoneHeadersMessage{
|
|
}
|
|
|
|
message RequestRelayBlocksMessage{
|
|
repeated Hash hashes = 1;
|
|
}
|
|
|
|
message RequestTransactionsMessage {
|
|
repeated TransactionId ids = 1;
|
|
}
|
|
|
|
message TransactionNotFoundMessage{
|
|
TransactionId id = 1;
|
|
}
|
|
|
|
message InvRelayBlockMessage{
|
|
Hash hash = 1;
|
|
}
|
|
|
|
message InvTransactionsMessage{
|
|
repeated TransactionId ids = 1;
|
|
}
|
|
|
|
message PingMessage{
|
|
uint64 nonce = 1;
|
|
}
|
|
|
|
message PongMessage{
|
|
uint64 nonce = 1;
|
|
}
|
|
|
|
message VerackMessage{
|
|
}
|
|
|
|
message VersionMessage{
|
|
uint32 protocolVersion = 1;
|
|
uint64 services = 2;
|
|
int64 timestamp = 3;
|
|
NetAddress address = 4;
|
|
bytes id = 5;
|
|
string userAgent = 6;
|
|
bool disableRelayTx = 8;
|
|
SubnetworkId subnetworkId = 9;
|
|
string network = 10;
|
|
}
|
|
|
|
message RejectMessage{
|
|
string reason = 1;
|
|
}
|
|
|
|
message RequestPruningPointUTXOSetMessage{
|
|
Hash pruningPointHash = 1;
|
|
}
|
|
|
|
message PruningPointUtxoSetChunkMessage{
|
|
repeated OutpointAndUtxoEntryPair outpointAndUtxoEntryPairs = 1;
|
|
}
|
|
|
|
message OutpointAndUtxoEntryPair{
|
|
Outpoint outpoint = 1;
|
|
UtxoEntry utxoEntry = 2;
|
|
}
|
|
|
|
message UtxoEntry {
|
|
uint64 amount = 1;
|
|
ScriptPublicKey scriptPublicKey = 2;
|
|
uint64 blockDaaScore = 3;
|
|
bool isCoinbase = 4;
|
|
}
|
|
|
|
message RequestNextPruningPointUtxoSetChunkMessage {
|
|
}
|
|
|
|
message DonePruningPointUtxoSetChunksMessage {
|
|
}
|
|
|
|
message RequestIBDBlocksMessage{
|
|
repeated Hash hashes = 1;
|
|
}
|
|
|
|
message UnexpectedPruningPointMessage{
|
|
}
|
|
|
|
message IbdBlockLocatorMessage {
|
|
Hash targetHash = 1;
|
|
repeated Hash blockLocatorHashes = 2;
|
|
}
|
|
|
|
message IbdBlockLocatorHighestHashMessage {
|
|
Hash highestHash = 1;
|
|
}
|
|
|
|
message IbdBlockLocatorHighestHashNotFoundMessage {
|
|
}
|
|
|
|
message BlockHeadersMessage {
|
|
repeated BlockHeader blockHeaders = 1;
|
|
}
|
|
|
|
message RequestPruningPointAndItsAnticoneMessage {
|
|
}
|
|
|
|
message BlockWithTrustedDataMessage {
|
|
BlockMessage block = 1;
|
|
uint64 daaScore = 2;
|
|
repeated DaaBlock daaWindow = 3;
|
|
repeated BlockGhostdagDataHashPair ghostdagData = 4;
|
|
}
|
|
|
|
message DaaBlock {
|
|
BlockMessage block = 3;
|
|
GhostdagData ghostdagData = 2;
|
|
}
|
|
|
|
message BlockGhostdagDataHashPair {
|
|
Hash hash = 1;
|
|
GhostdagData ghostdagData = 2;
|
|
}
|
|
|
|
message GhostdagData {
|
|
uint64 blueScore = 1;
|
|
bytes blueWork = 2;
|
|
Hash selectedParent = 3;
|
|
repeated Hash mergeSetBlues = 4;
|
|
repeated Hash mergeSetReds = 5;
|
|
repeated BluesAnticoneSizes bluesAnticoneSizes = 6;
|
|
}
|
|
|
|
message BluesAnticoneSizes {
|
|
Hash blueHash = 1;
|
|
uint32 anticoneSize = 2;
|
|
}
|
|
|
|
message DoneBlocksWithTrustedDataMessage {
|
|
}
|
|
|
|
message PruningPointsMessage {
|
|
repeated BlockHeader headers = 1;
|
|
}
|
|
|
|
message RequestPruningPointProofMessage {
|
|
}
|
|
|
|
message PruningPointProofMessage {
|
|
repeated PruningPointProofHeaderArray headers = 1;
|
|
}
|
|
|
|
message PruningPointProofHeaderArray {
|
|
repeated BlockHeader headers = 1;
|
|
}
|