mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-02 10:52:32 +00:00
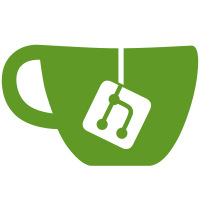
* Remove BlockHexes from GetBlocks request and response * Add GetBlocks RPC * Include the selectedTip's anticone in GetBlocks * Add Anticone to fakeRelayInvsContext * Include verbose data only if it was requested + Add comments to HandleGetBlocks * Allow antiPastHashesBetween to receive unrelated low and high hashes * Convert to/from protowire GetBlocksResponse with no verbose data correctly * Removed NextLowHash * Update GetBlocks in rpc_client * Validate in consensus.Anticone that blockHash exists Co-authored-by: stasatdaglabs <39559713+stasatdaglabs@users.noreply.github.com>
51 lines
1.5 KiB
Go
51 lines
1.5 KiB
Go
package appmessage
|
|
|
|
// GetBlocksRequestMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type GetBlocksRequestMessage struct {
|
|
baseMessage
|
|
LowHash string
|
|
IncludeBlockVerboseData bool
|
|
IncludeTransactionVerboseData bool
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *GetBlocksRequestMessage) Command() MessageCommand {
|
|
return CmdGetBlocksRequestMessage
|
|
}
|
|
|
|
// NewGetBlocksRequestMessage returns a instance of the message
|
|
func NewGetBlocksRequestMessage(lowHash string, includeBlockVerboseData bool,
|
|
includeTransactionVerboseData bool) *GetBlocksRequestMessage {
|
|
return &GetBlocksRequestMessage{
|
|
LowHash: lowHash,
|
|
IncludeBlockVerboseData: includeBlockVerboseData,
|
|
IncludeTransactionVerboseData: includeTransactionVerboseData,
|
|
}
|
|
}
|
|
|
|
// GetBlocksResponseMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type GetBlocksResponseMessage struct {
|
|
baseMessage
|
|
BlockHashes []string
|
|
BlockVerboseData []*BlockVerboseData
|
|
|
|
Error *RPCError
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *GetBlocksResponseMessage) Command() MessageCommand {
|
|
return CmdGetBlocksResponseMessage
|
|
}
|
|
|
|
// NewGetBlocksResponseMessage returns a instance of the message
|
|
func NewGetBlocksResponseMessage(blockHashes []string, blockHexes []string,
|
|
blockVerboseData []*BlockVerboseData) *GetBlocksResponseMessage {
|
|
|
|
return &GetBlocksResponseMessage{
|
|
BlockHashes: blockHashes,
|
|
BlockVerboseData: blockVerboseData,
|
|
}
|
|
}
|