mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
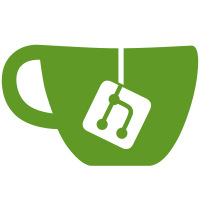
* [DEV-97] Moved github.com/daglabs/btcutil into github.com/daglabs/btcd/btcutil. * [DEV-97] Updated Gopkg.toml to no longer refer to btcutil. * [DEV-97] Renamed btcutil to util.
20 lines
588 B
Go
20 lines
588 B
Go
package util
|
|
|
|
// log2FloorMasks defines the masks to use when quickly calculating
|
|
// floor(log2(x)) in a constant log2(32) = 5 steps, where x is a uint32, using
|
|
// shifts. They are derived from (2^(2^x) - 1) * (2^(2^x)), for x in 4..0.
|
|
var log2FloorMasks = []uint32{0xffff0000, 0xff00, 0xf0, 0xc, 0x2}
|
|
|
|
// FastLog2Floor calculates and returns floor(log2(x)) in a constant 5 steps.
|
|
func FastLog2Floor(n uint32) uint8 {
|
|
rv := uint8(0)
|
|
exponent := uint8(16)
|
|
for i := 0; i < 5; i++ {
|
|
if n&log2FloorMasks[i] != 0 {
|
|
rv += exponent
|
|
n >>= exponent
|
|
}
|
|
exponent >>= 1
|
|
}
|
|
return rv
|
|
} |