mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
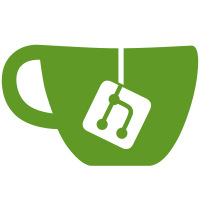
* Add fee estimation to wallet * Add fee rate to kaspawallet parse * Update go version * Get rid of golint * Add RBF support to wallet * Fix bump_fee UTXO lookup and fix wrong change address * impl storage mass as per KIP9 * Use CalculateTransactionOverallMass where needed * Some fixes * Minor typos * Fix test * update version * BroadcastRBF -> BroadcastReplacement * rc3 * align proto files to only use camel case (fixed on RK as well) * Rename to FeePolicy and add MaxFee option + todo * apply max fee constrains * increase minChangeTarget to 10kas * fmt * Some fixes * fix description: maximum -> minimum * put min feerate check in the correct location * Fix calculateFeeLimits nil handling * Add validations to CLI flags * Change to rc6 * Add checkTransactionFeeRate * Add failed broadcast transactions on send error` * Fix estimateFee change value * Estimate fee correctly for --send-all * On estimateFee always assume that the recipient has ECDSA address * remove patch version --------- Co-authored-by: Michael Sutton <msutton@cs.huji.ac.il>
43 lines
1.5 KiB
Go
43 lines
1.5 KiB
Go
package appmessage
|
|
|
|
// SubmitTransactionReplacementRequestMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type SubmitTransactionReplacementRequestMessage struct {
|
|
baseMessage
|
|
Transaction *RPCTransaction
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *SubmitTransactionReplacementRequestMessage) Command() MessageCommand {
|
|
return CmdSubmitTransactionReplacementRequestMessage
|
|
}
|
|
|
|
// NewSubmitTransactionReplacementRequestMessage returns a instance of the message
|
|
func NewSubmitTransactionReplacementRequestMessage(transaction *RPCTransaction) *SubmitTransactionReplacementRequestMessage {
|
|
return &SubmitTransactionReplacementRequestMessage{
|
|
Transaction: transaction,
|
|
}
|
|
}
|
|
|
|
// SubmitTransactionReplacementResponseMessage is an appmessage corresponding to
|
|
// its respective RPC message
|
|
type SubmitTransactionReplacementResponseMessage struct {
|
|
baseMessage
|
|
TransactionID string
|
|
ReplacedTransaction *RPCTransaction
|
|
|
|
Error *RPCError
|
|
}
|
|
|
|
// Command returns the protocol command string for the message
|
|
func (msg *SubmitTransactionReplacementResponseMessage) Command() MessageCommand {
|
|
return CmdSubmitTransactionReplacementResponseMessage
|
|
}
|
|
|
|
// NewSubmitTransactionReplacementResponseMessage returns a instance of the message
|
|
func NewSubmitTransactionReplacementResponseMessage(transactionID string) *SubmitTransactionReplacementResponseMessage {
|
|
return &SubmitTransactionReplacementResponseMessage{
|
|
TransactionID: transactionID,
|
|
}
|
|
}
|