mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-09 06:12:32 +00:00
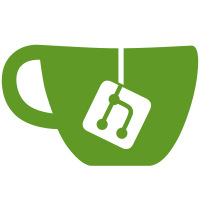
* Add fee estimation to wallet * Add fee rate to kaspawallet parse * Update go version * Get rid of golint * Add RBF support to wallet * Fix bump_fee UTXO lookup and fix wrong change address * impl storage mass as per KIP9 * Use CalculateTransactionOverallMass where needed * Some fixes * Minor typos * Fix test * update version * BroadcastRBF -> BroadcastReplacement * rc3 * align proto files to only use camel case (fixed on RK as well) * Rename to FeePolicy and add MaxFee option + todo * apply max fee constrains * increase minChangeTarget to 10kas * fmt * Some fixes * fix description: maximum -> minimum * put min feerate check in the correct location * Fix calculateFeeLimits nil handling * Add validations to CLI flags * Change to rc6 * Add checkTransactionFeeRate * Add failed broadcast transactions on send error` * Fix estimateFee change value * Estimate fee correctly for --send-all * On estimateFee always assume that the recipient has ECDSA address * remove patch version --------- Co-authored-by: Michael Sutton <msutton@cs.huji.ac.il>
172 lines
5.1 KiB
Go
172 lines
5.1 KiB
Go
package server
|
|
|
|
import (
|
|
"fmt"
|
|
"net"
|
|
"os"
|
|
"sync"
|
|
"sync/atomic"
|
|
"time"
|
|
|
|
"github.com/kaspanet/kaspad/version"
|
|
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
|
|
"github.com/kaspanet/kaspad/util/txmass"
|
|
|
|
"github.com/kaspanet/kaspad/util/profiling"
|
|
|
|
"github.com/kaspanet/kaspad/cmd/kaspawallet/daemon/pb"
|
|
"github.com/kaspanet/kaspad/cmd/kaspawallet/keys"
|
|
"github.com/kaspanet/kaspad/domain/dagconfig"
|
|
"github.com/kaspanet/kaspad/infrastructure/network/rpcclient"
|
|
"github.com/kaspanet/kaspad/infrastructure/os/signal"
|
|
"github.com/kaspanet/kaspad/util/panics"
|
|
"github.com/pkg/errors"
|
|
|
|
"google.golang.org/grpc"
|
|
)
|
|
|
|
type server struct {
|
|
pb.UnimplementedKaspawalletdServer
|
|
|
|
rpcClient *rpcclient.RPCClient // RPC client for ongoing user requests
|
|
backgroundRPCClient *rpcclient.RPCClient // RPC client dedicated for address and UTXO background fetching
|
|
params *dagconfig.Params
|
|
coinbaseMaturity uint64 // Is different from default if we use testnet-11
|
|
|
|
lock sync.RWMutex
|
|
utxosSortedByAmount []*walletUTXO
|
|
mempoolExcludedUTXOs map[externalapi.DomainOutpoint]*walletUTXO
|
|
nextSyncStartIndex uint32
|
|
keysFile *keys.File
|
|
shutdown chan struct{}
|
|
forceSyncChan chan struct{}
|
|
startTimeOfLastCompletedRefresh time.Time
|
|
addressSet walletAddressSet
|
|
txMassCalculator *txmass.Calculator
|
|
usedOutpoints map[externalapi.DomainOutpoint]time.Time
|
|
firstSyncDone atomic.Bool
|
|
|
|
isLogFinalProgressLineShown bool
|
|
maxUsedAddressesForLog uint32
|
|
maxProcessedAddressesForLog uint32
|
|
}
|
|
|
|
// MaxDaemonSendMsgSize is the max send message size used for the daemon server.
|
|
// Currently, set to 100MB
|
|
const MaxDaemonSendMsgSize = 100_000_000
|
|
|
|
// Start starts the kaspawalletd server
|
|
func Start(params *dagconfig.Params, listen, rpcServer string, keysFilePath string, profile string, timeout uint32) error {
|
|
initLog(defaultLogFile, defaultErrLogFile)
|
|
|
|
defer panics.HandlePanic(log, "MAIN", nil)
|
|
interrupt := signal.InterruptListener()
|
|
|
|
if profile != "" {
|
|
profiling.Start(profile, log)
|
|
}
|
|
|
|
log.Infof("Version %s", version.Version())
|
|
listener, err := net.Listen("tcp", listen)
|
|
if err != nil {
|
|
return (errors.Wrapf(err, "Error listening to TCP on %s", listen))
|
|
}
|
|
log.Infof("Listening to TCP on %s", listen)
|
|
|
|
log.Infof("Connecting to a node at %s...", rpcServer)
|
|
rpcClient, err := connectToRPC(params, rpcServer, timeout)
|
|
if err != nil {
|
|
return (errors.Wrapf(err, "Error connecting to RPC server %s", rpcServer))
|
|
}
|
|
backgroundRPCClient, err := connectToRPC(params, rpcServer, timeout)
|
|
if err != nil {
|
|
return (errors.Wrapf(err, "Error making a second connection to RPC server %s", rpcServer))
|
|
}
|
|
|
|
log.Infof("Connected, reading keys file %s...", keysFilePath)
|
|
keysFile, err := keys.ReadKeysFile(params, keysFilePath)
|
|
if err != nil {
|
|
return (errors.Wrapf(err, "Error reading keys file %s", keysFilePath))
|
|
}
|
|
|
|
err = keysFile.TryLock()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
dagInfo, err := rpcClient.GetBlockDAGInfo()
|
|
if err != nil {
|
|
return nil
|
|
}
|
|
|
|
coinbaseMaturity := params.BlockCoinbaseMaturity
|
|
if dagInfo.NetworkName == "kaspa-testnet-11" {
|
|
coinbaseMaturity = 1000
|
|
}
|
|
|
|
serverInstance := &server{
|
|
rpcClient: rpcClient,
|
|
backgroundRPCClient: backgroundRPCClient,
|
|
params: params,
|
|
coinbaseMaturity: coinbaseMaturity,
|
|
utxosSortedByAmount: []*walletUTXO{},
|
|
mempoolExcludedUTXOs: map[externalapi.DomainOutpoint]*walletUTXO{},
|
|
nextSyncStartIndex: 0,
|
|
keysFile: keysFile,
|
|
shutdown: make(chan struct{}),
|
|
forceSyncChan: make(chan struct{}),
|
|
addressSet: make(walletAddressSet),
|
|
txMassCalculator: txmass.NewCalculator(params.MassPerTxByte, params.MassPerScriptPubKeyByte, params.MassPerSigOp),
|
|
usedOutpoints: map[externalapi.DomainOutpoint]time.Time{},
|
|
isLogFinalProgressLineShown: false,
|
|
maxUsedAddressesForLog: 0,
|
|
maxProcessedAddressesForLog: 0,
|
|
}
|
|
|
|
log.Infof("Read, syncing the wallet...")
|
|
spawn("serverInstance.syncLoop", func() {
|
|
err := serverInstance.syncLoop()
|
|
if err != nil {
|
|
printErrorAndExit(errors.Wrap(err, "error syncing the wallet"))
|
|
}
|
|
})
|
|
|
|
grpcServer := grpc.NewServer(grpc.MaxSendMsgSize(MaxDaemonSendMsgSize))
|
|
pb.RegisterKaspawalletdServer(grpcServer, serverInstance)
|
|
|
|
spawn("grpcServer.Serve", func() {
|
|
err := grpcServer.Serve(listener)
|
|
if err != nil {
|
|
printErrorAndExit(errors.Wrap(err, "Error serving gRPC"))
|
|
}
|
|
})
|
|
|
|
select {
|
|
case <-serverInstance.shutdown:
|
|
case <-interrupt:
|
|
const stopTimeout = 2 * time.Second
|
|
|
|
stopChan := make(chan interface{})
|
|
spawn("gRPCServer.Stop", func() {
|
|
grpcServer.GracefulStop()
|
|
close(stopChan)
|
|
})
|
|
|
|
select {
|
|
case <-stopChan:
|
|
case <-time.After(stopTimeout):
|
|
log.Warnf("Could not gracefully stop: timed out after %s", stopTimeout)
|
|
grpcServer.Stop()
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func printErrorAndExit(err error) {
|
|
fmt.Fprintf(os.Stderr, "%+v\n", err)
|
|
os.Exit(1)
|
|
}
|