mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-25 00:06:49 +00:00
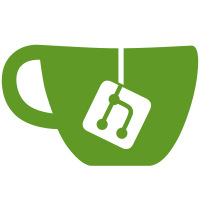
* Replaced the content of MsgIBDRootUTXOSetChunk with pairs of outpoint-utxo entry pairs. * Rename utxoIter to utxoIterator. * Add a big stinky TODO on an assert. * Replace pruningStore staging with a UTXO set iterator. * Reimplement receiveAndInsertIBDRootUTXOSet. * Extract OutpointAndUTXOEntryPairsToDomainOutpointAndUTXOEntryPairs into domainconverters.go. * Pass the outpoint and utxy entry pairs to the pruning store. * Implement InsertCandidatePruningPointUTXOs. * Implement ClearCandidatePruningPointUTXOs. * Implement UpdateCandidatePruningPointMultiset. * Use the candidate pruning point multiset in updatePruningPoint. * Implement CandidatePruningPointUTXOIterator. * Use the pruning point utxo set iterator for StageVirtualUTXOSet. * Defer ClearCandidatePruningPointUTXOs. * Implement OverwriteVirtualUTXOSet. * Implement CommitCandidatePruningPointUTXOSet. * Implement BeginOverwritingVirtualUTXOSet and FinishOverwritingVirtualUTXOSet. * Implement overwriteVirtualUTXOSetAndCommitPruningPointUTXOSet. * Rename ClearCandidatePruningPointUTXOs to ClearCandidatePruningPointData. * Add missing methods to dbManager. * Implement PruningPointUTXOs. * Implement RecoverUTXOIfRequired. * Delete the utxoserialization package. * Fix compilation errors in TestValidateAndInsertPruningPoint. * Switch order of operations in the if statements in PruningPointUTXOs so that Next() wouldn't be unnecessarily called. * Fix missing pruning point utxo set staging and bad slice length. * Fix no default multiset in InsertCandidatePruningPointUTXOs. * Make go vet happy. * Rename candidateXXX to importedXXX. * Do some more renaming. * Rename some more. * Fix bad MsgIBDRootNotFound logic. * Fix an error message. * Simplify receiveIBDRootBlock. * Fix error message in receiveAndInsertIBDRootUTXOSet. * Do some more renaming. * Fix merge errors. * Fix a bug caused by calling iterator.First() unnecessarily. * Remove databaseContext from stores and don't use a transaction in ClearXXX functions. * Simplify receiveAndInsertIBDRootUTXOSet. * Fix offset count in PruningPointUTXOs(). * Fix readOnlyUTXOIteratorWithDiff.First(). * Split handleRequestIBDRootUTXOSetAndBlockFlow into smaller methods. * Rename IbdRootNotFound to UnexpectedPruningPoint. * Rename requestIBDRootHash to requestPruningPointHash. * Rename IBDRootHash to PruningPointHash. * Rename RequestIBDRootUTXOSetAndBlock to RequestPruningPointUTXOSetAndBlock. * Rename IBDRootUTXOSetChunk to PruningPointUTXOSetChunk. * Rename RequestNextIBDRootUTXOSetChunk to RequestNextPruningPointUTXOSetChunk. * Rename DoneIBDRootUTXOSetChunks to DonePruningPointUTXOSetChunks. * Rename remaining references to IBD root. * Fix an error message. * Add a check for HadStartedImportingPruningPointUTXOSet in commitVirtualUTXODiff. * Add a check for HadStartedImportingPruningPointUTXOSet in ImportPruningPointUTXOSetIntoVirtualUTXOSet. * Move FinishImportingPruningPointUTXOSet closer to HadStartedImportingPruningPointUTXOSet. * Remove reference to pruningStore in utxoSetIterator. * Pointerify utxoSetIterator receivers. * Fix bad insert in CommitImportedPruningPointUTXOSet. * Rename commitImportedPruningPointUTXOSetAll to applyImportedPruningPointUTXOSet. * Simplify PruningPointUTXOs. * Add populateTransactionWithUTXOEntriesFromUTXOSet. * Fix a TODO comment. * Rename InsertImportedPruningPointUTXOs to AppendImportedPruningPointUTXOs. * Extract handleRequestPruningPointUTXOSetAndBlockMessage to a separate method. * Rename stuff in readOnlyUTXOIteratorWithDiff.First(). * Address toAddIterator in readOnlyUTXOIteratorWithDiff.First(). * Call First() before any full iteration on ReadOnlyUTXOSetIterator. * Call First() before any full iteration on a database Cursor. * Put StartImportingPruningPointUTXOSet inside the pruning point transaction. * Make serializeOutpoint and serializeUTXOEntry free functions in pruningStore. * Fix readOnlyUTXOIteratorWithDiff.First(). * Fix bad validations in importPruningPoint. * Remove superfluous call to validateBlockTransactionsAgainstPastUTXO.
201 lines
3.4 KiB
Protocol Buffer
201 lines
3.4 KiB
Protocol Buffer
syntax = "proto3";
|
|
package protowire;
|
|
|
|
option go_package = "github.com/kaspanet/kaspad/protowire";
|
|
|
|
message RequestAddressesMessage{
|
|
bool includeAllSubnetworks = 1;
|
|
SubnetworkId subnetworkId = 2;
|
|
}
|
|
|
|
message AddressesMessage{
|
|
repeated NetAddress addressList = 1;
|
|
}
|
|
|
|
message NetAddress{
|
|
int64 timestamp = 1;
|
|
uint64 services = 2;
|
|
bytes ip = 3;
|
|
uint32 port = 4;
|
|
}
|
|
|
|
message SubnetworkId{
|
|
bytes bytes = 1;
|
|
}
|
|
|
|
message TransactionMessage{
|
|
uint32 version = 1;
|
|
repeated TransactionInput inputs = 2;
|
|
repeated TransactionOutput outputs = 3;
|
|
uint64 lockTime = 4;
|
|
SubnetworkId subnetworkId = 5;
|
|
uint64 gas = 6;
|
|
Hash payloadHash = 7;
|
|
bytes payload = 8;
|
|
}
|
|
|
|
message TransactionInput{
|
|
Outpoint previousOutpoint = 1;
|
|
bytes signatureScript = 2;
|
|
uint64 sequence = 3;
|
|
}
|
|
|
|
message Outpoint{
|
|
TransactionId transactionId = 1;
|
|
uint32 index = 2;
|
|
}
|
|
|
|
message TransactionId{
|
|
bytes bytes = 1;
|
|
}
|
|
message ScriptPublicKey {
|
|
bytes script = 1;
|
|
uint32 version = 2;
|
|
}
|
|
|
|
message TransactionOutput{
|
|
uint64 value = 1;
|
|
ScriptPublicKey scriptPublicKey = 2;
|
|
}
|
|
|
|
message BlockMessage{
|
|
BlockHeaderMessage header = 1;
|
|
repeated TransactionMessage transactions = 2;
|
|
}
|
|
|
|
message BlockHeaderMessage{
|
|
uint32 version = 1;
|
|
repeated Hash parentHashes = 2;
|
|
Hash hashMerkleRoot = 3;
|
|
Hash acceptedIdMerkleRoot = 4;
|
|
Hash utxoCommitment = 5;
|
|
int64 timestamp = 6;
|
|
uint32 bits = 7;
|
|
uint64 nonce = 8;
|
|
}
|
|
|
|
message Hash{
|
|
bytes bytes = 1;
|
|
}
|
|
|
|
message RequestBlockLocatorMessage{
|
|
Hash lowHash = 1;
|
|
Hash highHash = 2;
|
|
uint32 limit = 3;
|
|
}
|
|
|
|
message BlockLocatorMessage{
|
|
repeated Hash hashes = 1;
|
|
}
|
|
|
|
message RequestHeadersMessage{
|
|
Hash lowHash = 1;
|
|
Hash highHash = 2;
|
|
}
|
|
|
|
message RequestNextHeadersMessage{
|
|
}
|
|
|
|
message DoneHeadersMessage{
|
|
}
|
|
|
|
message RequestRelayBlocksMessage{
|
|
repeated Hash hashes = 1;
|
|
}
|
|
|
|
message RequestTransactionsMessage {
|
|
repeated TransactionId ids = 1;
|
|
}
|
|
|
|
message TransactionNotFoundMessage{
|
|
TransactionId id = 1;
|
|
}
|
|
|
|
message InvRelayBlockMessage{
|
|
Hash hash = 1;
|
|
}
|
|
|
|
message InvTransactionsMessage{
|
|
repeated TransactionId ids = 1;
|
|
}
|
|
|
|
message PingMessage{
|
|
uint64 nonce = 1;
|
|
}
|
|
|
|
message PongMessage{
|
|
uint64 nonce = 1;
|
|
}
|
|
|
|
message VerackMessage{
|
|
}
|
|
|
|
message VersionMessage{
|
|
uint32 protocolVersion = 1;
|
|
uint64 services = 2;
|
|
int64 timestamp = 3;
|
|
NetAddress address = 4;
|
|
bytes id = 5;
|
|
string userAgent = 6;
|
|
bool disableRelayTx = 8;
|
|
SubnetworkId subnetworkId = 9;
|
|
string network = 10;
|
|
}
|
|
|
|
message RejectMessage{
|
|
string reason = 1;
|
|
}
|
|
|
|
message RequestPruningPointUTXOSetAndBlockMessage{
|
|
Hash pruningPointHash = 1;
|
|
}
|
|
|
|
message PruningPointUtxoSetChunkMessage{
|
|
repeated OutpointAndUtxoEntryPair outpointAndUtxoEntryPairs = 1;
|
|
}
|
|
|
|
message OutpointAndUtxoEntryPair{
|
|
Outpoint outpoint = 1;
|
|
UtxoEntry utxoEntry = 2;
|
|
}
|
|
|
|
message UtxoEntry {
|
|
uint64 amount = 1;
|
|
ScriptPublicKey scriptPublicKey = 2;
|
|
uint64 blockBlueScore = 3;
|
|
bool isCoinbase = 4;
|
|
}
|
|
|
|
message RequestNextPruningPointUtxoSetChunkMessage {
|
|
}
|
|
|
|
message DonePruningPointUtxoSetChunksMessage {
|
|
}
|
|
|
|
message RequestIBDBlocksMessage{
|
|
repeated Hash hashes = 1;
|
|
}
|
|
|
|
message UnexpectedPruningPointMessage{
|
|
}
|
|
|
|
message RequestPruningPointHashMessage{
|
|
}
|
|
|
|
message PruningPointHashMessage{
|
|
Hash hash = 1;
|
|
}
|
|
|
|
message IbdBlockLocatorMessage {
|
|
Hash targetHash = 1;
|
|
repeated Hash blockLocatorHashes = 2;
|
|
}
|
|
|
|
message IbdBlockLocatorHighestHashMessage {
|
|
Hash highestHash = 1;
|
|
}
|
|
|
|
message BlockHeadersMessage {
|
|
repeated BlockHeaderMessage blockHeaders = 1;
|
|
}
|