mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
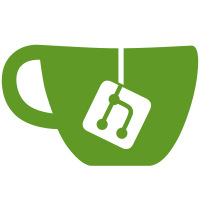
* [NOD-1500] Added Domain type and Constructor * [NOD-1500] Replaced dag+txpool with domain in flowContext * [NOD-1500] Replaced dag+txpool with domain in flowContext * [NOD-1500] Converters: domain objects from/to appmessage * [NOD-1500] Convert hashes to DomainHashes in appmessages * [NOD-1500] Remove references to daghash in dagconfig * [NOD-1500] Fixed all appmessage usages of hashes * [NOD-1500] Update all RPC to use domain * [NOD-1500] Big chunk of protocol flows re-wired to domain * [NOD-1500] Finished re-wiring all protocol flows to new Domain * [NOD-1500] Fix some mempool and kaspaminer compilation errors * [NOD-1500] Deleted util/{block,tx,daghash} and dbaccess * [NOD-1500] util.CoinbaseTransactionIndex -> transactionhelper.CoinbaseTransactionIndex * [NOD-1500] Fix txsigner * [NOD-1500] Removed all references to util/subnetworkid * [NOD-1500] Update RpcGetBlock related messages * [NOD-1500] Many more compilation fixes * [NOD-1500] Return full list of missing blocks for orphan resolution * [NOD-1500] Fixed handshake * [NOD-1500] Fixed flowcontext compilation * [NOD-1500] Update users of StartIBDIfRequired to handle error * [NOD-1500] Removed some more fields from RPC * [NOD-1500] Fix the getBlockTemplate flow * [NOD-1500] Fix HandleGetCurrentNetwork * [NOD-1500] Remove redundant code * [NOD-1500] Remove obsolete notifications * [NOD-1500] Split MiningManager and Consensus to separate fields in Domain * [NOD-1500] Update two wrong references to location of txscript * [NOD-1500] Added comments * [NOD-1500] Fix some tests * [NOD-1500] Removed serialization logic from appmessage * [NOD-1500] Rename database/serialization/messages.proto to dbobjects.proto * [NOD-1500] Delete integration tests * [NOD-1500] Remove txsort * [NOD-1500] Fix tiny bug * [NOD-1500] Remove rogue dependancy on bchd * [NOD-1500] Some stylistic fixes
111 lines
2.8 KiB
Go
111 lines
2.8 KiB
Go
package protowire
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/hashes"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/subnetworks"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/transactionid"
|
|
"math"
|
|
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
"github.com/kaspanet/kaspad/util/mstime"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
func (x *Hash) toDomain() (*externalapi.DomainHash, error) {
|
|
return hashes.FromBytes(x.Bytes)
|
|
}
|
|
|
|
func protoHashesToDomain(protoHashes []*Hash) ([]*externalapi.DomainHash, error) {
|
|
domainHashes := make([]*externalapi.DomainHash, len(protoHashes))
|
|
for i, protoHash := range protoHashes {
|
|
var err error
|
|
domainHashes[i], err = protoHash.toDomain()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
return domainHashes, nil
|
|
}
|
|
|
|
func domainHashToProto(hash *externalapi.DomainHash) *Hash {
|
|
return &Hash{
|
|
Bytes: hash[:],
|
|
}
|
|
}
|
|
|
|
func domainHashesToProto(hashes []*externalapi.DomainHash) []*Hash {
|
|
protoHashes := make([]*Hash, len(hashes))
|
|
for i, hash := range hashes {
|
|
protoHashes[i] = domainHashToProto(hash)
|
|
}
|
|
return protoHashes
|
|
}
|
|
|
|
func (x *TransactionID) toDomain() (*externalapi.DomainTransactionID, error) {
|
|
return transactionid.FromBytes(x.Bytes)
|
|
}
|
|
|
|
func protoTransactionIDsToDomain(protoIDs []*TransactionID) ([]*externalapi.DomainTransactionID, error) {
|
|
txIDs := make([]*externalapi.DomainTransactionID, len(protoIDs))
|
|
for i, protoID := range protoIDs {
|
|
var err error
|
|
txIDs[i], err = protoID.toDomain()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
}
|
|
return txIDs, nil
|
|
}
|
|
|
|
func domainTransactionIDToProto(id *externalapi.DomainTransactionID) *TransactionID {
|
|
return &TransactionID{
|
|
Bytes: id[:],
|
|
}
|
|
}
|
|
|
|
func wireTransactionIDsToProto(ids []*externalapi.DomainTransactionID) []*TransactionID {
|
|
protoIDs := make([]*TransactionID, len(ids))
|
|
for i, hash := range ids {
|
|
protoIDs[i] = domainTransactionIDToProto(hash)
|
|
}
|
|
return protoIDs
|
|
}
|
|
|
|
func (x *SubnetworkID) toDomain() (*externalapi.DomainSubnetworkID, error) {
|
|
if x == nil {
|
|
return nil, nil
|
|
}
|
|
return subnetworks.FromBytes(x.Bytes)
|
|
}
|
|
|
|
func domainSubnetworkIDToProto(id *externalapi.DomainSubnetworkID) *SubnetworkID {
|
|
if id == nil {
|
|
return nil
|
|
}
|
|
return &SubnetworkID{
|
|
Bytes: id[:],
|
|
}
|
|
}
|
|
|
|
func (x *NetAddress) toAppMessage() (*appmessage.NetAddress, error) {
|
|
if x.Port > math.MaxUint16 {
|
|
return nil, errors.Errorf("port number is larger than %d", math.MaxUint16)
|
|
}
|
|
return &appmessage.NetAddress{
|
|
Timestamp: mstime.UnixMilliseconds(x.Timestamp),
|
|
Services: appmessage.ServiceFlag(x.Services),
|
|
IP: x.Ip,
|
|
Port: uint16(x.Port),
|
|
}, nil
|
|
}
|
|
|
|
func appMessageNetAddressToProto(address *appmessage.NetAddress) *NetAddress {
|
|
return &NetAddress{
|
|
Timestamp: address.Timestamp.UnixMilliseconds(),
|
|
Services: uint64(address.Services),
|
|
Ip: address.IP,
|
|
Port: uint32(address.Port),
|
|
}
|
|
}
|