mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-08 05:42:36 +00:00
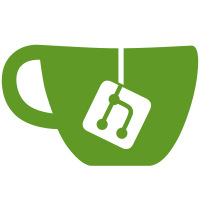
* fix mempool accessing, rewrite get_mempool_entries_by_addresses * fix counter, add verbose * fmt * addresses as string * Define error in case utxoEntry is missing. * fix error variable to string * stop tests from failing (see in code comment) * access both pools in the same state via parameters * get rid of todo message * fmt - very important! * perf: scriptpublickey in mempool, no txscript. * address reveiw * fmt fix * mixed up isorphan bool, pass tests now * do map preallocation in mempoolbyaddresses * no proallocation for orphanpool sending. Co-authored-by: Ori Newman <orinewman1@gmail.com>
59 lines
1.7 KiB
Go
59 lines
1.7 KiB
Go
package transactionrelay
|
|
|
|
import (
|
|
"github.com/kaspanet/kaspad/app/appmessage"
|
|
"github.com/kaspanet/kaspad/infrastructure/network/netadapter/router"
|
|
)
|
|
|
|
type handleRequestedTransactionsFlow struct {
|
|
TransactionsRelayContext
|
|
incomingRoute, outgoingRoute *router.Route
|
|
}
|
|
|
|
// HandleRequestedTransactions listens to appmessage.MsgRequestTransactions messages, responding with the requested
|
|
// transactions if those are in the mempool.
|
|
// Missing transactions would be ignored
|
|
func HandleRequestedTransactions(context TransactionsRelayContext, incomingRoute *router.Route, outgoingRoute *router.Route) error {
|
|
flow := &handleRequestedTransactionsFlow{
|
|
TransactionsRelayContext: context,
|
|
incomingRoute: incomingRoute,
|
|
outgoingRoute: outgoingRoute,
|
|
}
|
|
return flow.start()
|
|
}
|
|
|
|
func (flow *handleRequestedTransactionsFlow) start() error {
|
|
for {
|
|
msgRequestTransactions, err := flow.readRequestTransactions()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, transactionID := range msgRequestTransactions.IDs {
|
|
tx, _, ok := flow.Domain().MiningManager().GetTransaction(transactionID, true, false)
|
|
|
|
if !ok {
|
|
msgTransactionNotFound := appmessage.NewMsgTransactionNotFound(transactionID)
|
|
err := flow.outgoingRoute.Enqueue(msgTransactionNotFound)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
continue
|
|
}
|
|
err := flow.outgoingRoute.Enqueue(appmessage.DomainTransactionToMsgTx(tx))
|
|
if err != nil {
|
|
return err
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
func (flow *handleRequestedTransactionsFlow) readRequestTransactions() (*appmessage.MsgRequestTransactions, error) {
|
|
msg, err := flow.incomingRoute.Dequeue()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return msg.(*appmessage.MsgRequestTransactions), nil
|
|
}
|