mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
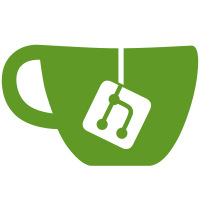
* [NOD-1575] Implement Clone and Equal for all model types * [NOD-1575] Add assertion for transaction ID equality * [NOD-1575] Use DomainTransaction.Equal to compare to expected coinbase transaction * [NOD-1575] Add TestDomainBlockHeader_Clone * [NOD-1575] Don't clone nil values * [NOD-1575] Add type assertions * [NOD-1575] Don't clone nil values * [NOD-1575] Add missing Equals * [NOD-1575] Add length checks * [NOD-1575] Update comment * [NOD-1575] Check length for TransactionAcceptanceData * [NOD-1575] Explicitly clone nils where needed * [NOD-1575] Clone tx id * [NOD-1575] Flip condition * Nod 1576 make coverage tests for equal clone inside model externalapi (#1177) * [NOD-1576] Make coverage tests for equal and clone inside model and externalapi * Some formatting and naming fixes * Made transactionToCompare type exported * Added some tests and made some changes to the tests code * No changes made * Some formatting and naming changes made * Made better test coverage for externalapi clone and equal functions * Changed expected result for two cases * Added equal and clone functions tests for ghostdag and utxodiff * Added tests * [NOD-1576] Implement reachabilitydata equal/clone unit tests * [NOD-1576] Full coverage of reachabilitydata equal/clone unit tests * Made changes and handling panic to transaction_equal_clone_test.go and formating of utxodiff_equal_clone_test.go * Added recoverForEqual2 for handling panic to transaction_equal_clone_test.go * [NOD-1576] Full coverage of transaction equal unit test * [NOD-1576] Add expects panic * [NOD-1576] Allow composites in go vet * [NOD-1576] Code review fixes (#1223) * [NOD-1576] Code review fixes * [NOD-1576] Code review fixes part 2 * [NOD-1576] Fix wrong name Co-authored-by: karim1king <karimkaspersky@yahoo.com> Co-authored-by: Ori Newman <orinewman1@gmail.com> Co-authored-by: Karim <karim1king@users.noreply.github.com> * Fix merge errors * Use Equal where possible * Use Equal where possible * Use Equal where possible Co-authored-by: andrey-hash <74914043+andrey-hash@users.noreply.github.com> Co-authored-by: karim1king <karimkaspersky@yahoo.com> Co-authored-by: Karim <karim1king@users.noreply.github.com>
50 lines
1.6 KiB
Go
50 lines
1.6 KiB
Go
package externalapi
|
|
|
|
// BlockStatus represents the validation state of the block.
|
|
type BlockStatus byte
|
|
|
|
// Clone returns a clone of BlockStatus
|
|
func (bs BlockStatus) Clone() BlockStatus {
|
|
return bs
|
|
}
|
|
|
|
// If this doesn't compile, it means the type definition has been changed, so it's
|
|
// an indication to update Equal and Clone accordingly.
|
|
var _ BlockStatus = 0
|
|
|
|
// Equal returns whether bs equals to other
|
|
func (bs BlockStatus) Equal(other BlockStatus) bool {
|
|
return bs == other
|
|
}
|
|
|
|
const (
|
|
// StatusInvalid indicates that the block is invalid.
|
|
StatusInvalid BlockStatus = iota
|
|
|
|
// StatusUTXOValid indicates the block is valid from any UTXO related aspects and has passed all the other validations as well.
|
|
StatusUTXOValid
|
|
|
|
// StatusUTXOPendingVerification indicates that the block is pending verification against its past UTXO-Set, either
|
|
// because it was not yet verified since the block was never in the selected parent chain, or if the
|
|
// block violates finality.
|
|
StatusUTXOPendingVerification
|
|
|
|
// StatusDisqualifiedFromChain indicates that the block is not eligible to be a selected parent.
|
|
StatusDisqualifiedFromChain
|
|
|
|
// StatusHeaderOnly indicates that the block transactions are not held (pruned or wasn't added yet)
|
|
StatusHeaderOnly
|
|
)
|
|
|
|
var blockStatusStrings = map[BlockStatus]string{
|
|
StatusInvalid: "Invalid",
|
|
StatusUTXOValid: "Valid",
|
|
StatusUTXOPendingVerification: "UTXOPendingVerification",
|
|
StatusDisqualifiedFromChain: "DisqualifiedFromChain",
|
|
StatusHeaderOnly: "HeaderOnly",
|
|
}
|
|
|
|
func (bs BlockStatus) String() string {
|
|
return blockStatusStrings[bs]
|
|
}
|