mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
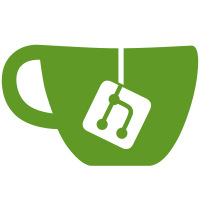
* Adding support for mass. * update grpc v1.69.2 * Upgrade protos using latest protoc * Use MassCommitment instead of Mass * Fix DomainTransaction clone, equal and tests --------- Co-authored-by: veronica <jimjouny@gmail.com> Co-authored-by: Ori Newman <orinewman1@gmail.com>
3616 lines
132 KiB
Go
3616 lines
132 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.36.2
|
|
// protoc v3.12.3
|
|
// source: p2p.proto
|
|
|
|
package protowire
|
|
|
|
import (
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
type RequestAddressesMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
IncludeAllSubnetworks bool `protobuf:"varint,1,opt,name=includeAllSubnetworks,proto3" json:"includeAllSubnetworks,omitempty"`
|
|
SubnetworkId *SubnetworkId `protobuf:"bytes,2,opt,name=subnetworkId,proto3" json:"subnetworkId,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestAddressesMessage) Reset() {
|
|
*x = RequestAddressesMessage{}
|
|
mi := &file_p2p_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestAddressesMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestAddressesMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestAddressesMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[0]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestAddressesMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestAddressesMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *RequestAddressesMessage) GetIncludeAllSubnetworks() bool {
|
|
if x != nil {
|
|
return x.IncludeAllSubnetworks
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *RequestAddressesMessage) GetSubnetworkId() *SubnetworkId {
|
|
if x != nil {
|
|
return x.SubnetworkId
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type AddressesMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
AddressList []*NetAddress `protobuf:"bytes,1,rep,name=addressList,proto3" json:"addressList,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *AddressesMessage) Reset() {
|
|
*x = AddressesMessage{}
|
|
mi := &file_p2p_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *AddressesMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AddressesMessage) ProtoMessage() {}
|
|
|
|
func (x *AddressesMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[1]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AddressesMessage.ProtoReflect.Descriptor instead.
|
|
func (*AddressesMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *AddressesMessage) GetAddressList() []*NetAddress {
|
|
if x != nil {
|
|
return x.AddressList
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type NetAddress struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Timestamp int64 `protobuf:"varint,1,opt,name=timestamp,proto3" json:"timestamp,omitempty"`
|
|
Ip []byte `protobuf:"bytes,3,opt,name=ip,proto3" json:"ip,omitempty"`
|
|
Port uint32 `protobuf:"varint,4,opt,name=port,proto3" json:"port,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NetAddress) Reset() {
|
|
*x = NetAddress{}
|
|
mi := &file_p2p_proto_msgTypes[2]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NetAddress) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NetAddress) ProtoMessage() {}
|
|
|
|
func (x *NetAddress) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[2]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NetAddress.ProtoReflect.Descriptor instead.
|
|
func (*NetAddress) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
func (x *NetAddress) GetTimestamp() int64 {
|
|
if x != nil {
|
|
return x.Timestamp
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *NetAddress) GetIp() []byte {
|
|
if x != nil {
|
|
return x.Ip
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *NetAddress) GetPort() uint32 {
|
|
if x != nil {
|
|
return x.Port
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type SubnetworkId struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Bytes []byte `protobuf:"bytes,1,opt,name=bytes,proto3" json:"bytes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *SubnetworkId) Reset() {
|
|
*x = SubnetworkId{}
|
|
mi := &file_p2p_proto_msgTypes[3]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *SubnetworkId) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubnetworkId) ProtoMessage() {}
|
|
|
|
func (x *SubnetworkId) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[3]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubnetworkId.ProtoReflect.Descriptor instead.
|
|
func (*SubnetworkId) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
func (x *SubnetworkId) GetBytes() []byte {
|
|
if x != nil {
|
|
return x.Bytes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type TransactionMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Version uint32 `protobuf:"varint,1,opt,name=version,proto3" json:"version,omitempty"`
|
|
Inputs []*TransactionInput `protobuf:"bytes,2,rep,name=inputs,proto3" json:"inputs,omitempty"`
|
|
Outputs []*TransactionOutput `protobuf:"bytes,3,rep,name=outputs,proto3" json:"outputs,omitempty"`
|
|
LockTime uint64 `protobuf:"varint,4,opt,name=lockTime,proto3" json:"lockTime,omitempty"`
|
|
SubnetworkId *SubnetworkId `protobuf:"bytes,5,opt,name=subnetworkId,proto3" json:"subnetworkId,omitempty"`
|
|
Gas uint64 `protobuf:"varint,6,opt,name=gas,proto3" json:"gas,omitempty"`
|
|
Payload []byte `protobuf:"bytes,8,opt,name=payload,proto3" json:"payload,omitempty"`
|
|
Mass uint64 `protobuf:"varint,9,opt,name=mass,proto3" json:"mass,omitempty"` // <<< BPS10 - Add mass to TransactionMessage
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *TransactionMessage) Reset() {
|
|
*x = TransactionMessage{}
|
|
mi := &file_p2p_proto_msgTypes[4]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *TransactionMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TransactionMessage) ProtoMessage() {}
|
|
|
|
func (x *TransactionMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[4]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TransactionMessage.ProtoReflect.Descriptor instead.
|
|
func (*TransactionMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
func (x *TransactionMessage) GetVersion() uint32 {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *TransactionMessage) GetInputs() []*TransactionInput {
|
|
if x != nil {
|
|
return x.Inputs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *TransactionMessage) GetOutputs() []*TransactionOutput {
|
|
if x != nil {
|
|
return x.Outputs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *TransactionMessage) GetLockTime() uint64 {
|
|
if x != nil {
|
|
return x.LockTime
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *TransactionMessage) GetSubnetworkId() *SubnetworkId {
|
|
if x != nil {
|
|
return x.SubnetworkId
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *TransactionMessage) GetGas() uint64 {
|
|
if x != nil {
|
|
return x.Gas
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *TransactionMessage) GetPayload() []byte {
|
|
if x != nil {
|
|
return x.Payload
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *TransactionMessage) GetMass() uint64 {
|
|
if x != nil {
|
|
return x.Mass
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type TransactionInput struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
PreviousOutpoint *Outpoint `protobuf:"bytes,1,opt,name=previousOutpoint,proto3" json:"previousOutpoint,omitempty"`
|
|
SignatureScript []byte `protobuf:"bytes,2,opt,name=signatureScript,proto3" json:"signatureScript,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,3,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
SigOpCount uint32 `protobuf:"varint,4,opt,name=sigOpCount,proto3" json:"sigOpCount,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *TransactionInput) Reset() {
|
|
*x = TransactionInput{}
|
|
mi := &file_p2p_proto_msgTypes[5]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *TransactionInput) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TransactionInput) ProtoMessage() {}
|
|
|
|
func (x *TransactionInput) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[5]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TransactionInput.ProtoReflect.Descriptor instead.
|
|
func (*TransactionInput) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{5}
|
|
}
|
|
|
|
func (x *TransactionInput) GetPreviousOutpoint() *Outpoint {
|
|
if x != nil {
|
|
return x.PreviousOutpoint
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *TransactionInput) GetSignatureScript() []byte {
|
|
if x != nil {
|
|
return x.SignatureScript
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *TransactionInput) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *TransactionInput) GetSigOpCount() uint32 {
|
|
if x != nil {
|
|
return x.SigOpCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type Outpoint struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
TransactionId *TransactionId `protobuf:"bytes,1,opt,name=transactionId,proto3" json:"transactionId,omitempty"`
|
|
Index uint32 `protobuf:"varint,2,opt,name=index,proto3" json:"index,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *Outpoint) Reset() {
|
|
*x = Outpoint{}
|
|
mi := &file_p2p_proto_msgTypes[6]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *Outpoint) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Outpoint) ProtoMessage() {}
|
|
|
|
func (x *Outpoint) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[6]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Outpoint.ProtoReflect.Descriptor instead.
|
|
func (*Outpoint) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{6}
|
|
}
|
|
|
|
func (x *Outpoint) GetTransactionId() *TransactionId {
|
|
if x != nil {
|
|
return x.TransactionId
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Outpoint) GetIndex() uint32 {
|
|
if x != nil {
|
|
return x.Index
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type TransactionId struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Bytes []byte `protobuf:"bytes,1,opt,name=bytes,proto3" json:"bytes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *TransactionId) Reset() {
|
|
*x = TransactionId{}
|
|
mi := &file_p2p_proto_msgTypes[7]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *TransactionId) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TransactionId) ProtoMessage() {}
|
|
|
|
func (x *TransactionId) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[7]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TransactionId.ProtoReflect.Descriptor instead.
|
|
func (*TransactionId) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{7}
|
|
}
|
|
|
|
func (x *TransactionId) GetBytes() []byte {
|
|
if x != nil {
|
|
return x.Bytes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ScriptPublicKey struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Script []byte `protobuf:"bytes,1,opt,name=script,proto3" json:"script,omitempty"`
|
|
Version uint32 `protobuf:"varint,2,opt,name=version,proto3" json:"version,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ScriptPublicKey) Reset() {
|
|
*x = ScriptPublicKey{}
|
|
mi := &file_p2p_proto_msgTypes[8]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ScriptPublicKey) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ScriptPublicKey) ProtoMessage() {}
|
|
|
|
func (x *ScriptPublicKey) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[8]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ScriptPublicKey.ProtoReflect.Descriptor instead.
|
|
func (*ScriptPublicKey) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{8}
|
|
}
|
|
|
|
func (x *ScriptPublicKey) GetScript() []byte {
|
|
if x != nil {
|
|
return x.Script
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *ScriptPublicKey) GetVersion() uint32 {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type TransactionOutput struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Value uint64 `protobuf:"varint,1,opt,name=value,proto3" json:"value,omitempty"`
|
|
ScriptPublicKey *ScriptPublicKey `protobuf:"bytes,2,opt,name=scriptPublicKey,proto3" json:"scriptPublicKey,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *TransactionOutput) Reset() {
|
|
*x = TransactionOutput{}
|
|
mi := &file_p2p_proto_msgTypes[9]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *TransactionOutput) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TransactionOutput) ProtoMessage() {}
|
|
|
|
func (x *TransactionOutput) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[9]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TransactionOutput.ProtoReflect.Descriptor instead.
|
|
func (*TransactionOutput) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{9}
|
|
}
|
|
|
|
func (x *TransactionOutput) GetValue() uint64 {
|
|
if x != nil {
|
|
return x.Value
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *TransactionOutput) GetScriptPublicKey() *ScriptPublicKey {
|
|
if x != nil {
|
|
return x.ScriptPublicKey
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BlockMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Header *BlockHeader `protobuf:"bytes,1,opt,name=header,proto3" json:"header,omitempty"`
|
|
Transactions []*TransactionMessage `protobuf:"bytes,2,rep,name=transactions,proto3" json:"transactions,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BlockMessage) Reset() {
|
|
*x = BlockMessage{}
|
|
mi := &file_p2p_proto_msgTypes[10]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BlockMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockMessage) ProtoMessage() {}
|
|
|
|
func (x *BlockMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[10]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockMessage.ProtoReflect.Descriptor instead.
|
|
func (*BlockMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{10}
|
|
}
|
|
|
|
func (x *BlockMessage) GetHeader() *BlockHeader {
|
|
if x != nil {
|
|
return x.Header
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockMessage) GetTransactions() []*TransactionMessage {
|
|
if x != nil {
|
|
return x.Transactions
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BlockHeader struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Version uint32 `protobuf:"varint,1,opt,name=version,proto3" json:"version,omitempty"`
|
|
Parents []*BlockLevelParents `protobuf:"bytes,12,rep,name=parents,proto3" json:"parents,omitempty"`
|
|
HashMerkleRoot *Hash `protobuf:"bytes,3,opt,name=hashMerkleRoot,proto3" json:"hashMerkleRoot,omitempty"`
|
|
AcceptedIdMerkleRoot *Hash `protobuf:"bytes,4,opt,name=acceptedIdMerkleRoot,proto3" json:"acceptedIdMerkleRoot,omitempty"`
|
|
UtxoCommitment *Hash `protobuf:"bytes,5,opt,name=utxoCommitment,proto3" json:"utxoCommitment,omitempty"`
|
|
Timestamp int64 `protobuf:"varint,6,opt,name=timestamp,proto3" json:"timestamp,omitempty"`
|
|
Bits uint32 `protobuf:"varint,7,opt,name=bits,proto3" json:"bits,omitempty"`
|
|
Nonce uint64 `protobuf:"varint,8,opt,name=nonce,proto3" json:"nonce,omitempty"`
|
|
DaaScore uint64 `protobuf:"varint,9,opt,name=daaScore,proto3" json:"daaScore,omitempty"`
|
|
BlueWork []byte `protobuf:"bytes,10,opt,name=blueWork,proto3" json:"blueWork,omitempty"`
|
|
PruningPoint *Hash `protobuf:"bytes,14,opt,name=pruningPoint,proto3" json:"pruningPoint,omitempty"`
|
|
BlueScore uint64 `protobuf:"varint,13,opt,name=blueScore,proto3" json:"blueScore,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BlockHeader) Reset() {
|
|
*x = BlockHeader{}
|
|
mi := &file_p2p_proto_msgTypes[11]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BlockHeader) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockHeader) ProtoMessage() {}
|
|
|
|
func (x *BlockHeader) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[11]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockHeader.ProtoReflect.Descriptor instead.
|
|
func (*BlockHeader) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{11}
|
|
}
|
|
|
|
func (x *BlockHeader) GetVersion() uint32 {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BlockHeader) GetParents() []*BlockLevelParents {
|
|
if x != nil {
|
|
return x.Parents
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockHeader) GetHashMerkleRoot() *Hash {
|
|
if x != nil {
|
|
return x.HashMerkleRoot
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockHeader) GetAcceptedIdMerkleRoot() *Hash {
|
|
if x != nil {
|
|
return x.AcceptedIdMerkleRoot
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockHeader) GetUtxoCommitment() *Hash {
|
|
if x != nil {
|
|
return x.UtxoCommitment
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockHeader) GetTimestamp() int64 {
|
|
if x != nil {
|
|
return x.Timestamp
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BlockHeader) GetBits() uint32 {
|
|
if x != nil {
|
|
return x.Bits
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BlockHeader) GetNonce() uint64 {
|
|
if x != nil {
|
|
return x.Nonce
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BlockHeader) GetDaaScore() uint64 {
|
|
if x != nil {
|
|
return x.DaaScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BlockHeader) GetBlueWork() []byte {
|
|
if x != nil {
|
|
return x.BlueWork
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockHeader) GetPruningPoint() *Hash {
|
|
if x != nil {
|
|
return x.PruningPoint
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockHeader) GetBlueScore() uint64 {
|
|
if x != nil {
|
|
return x.BlueScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type BlockLevelParents struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
ParentHashes []*Hash `protobuf:"bytes,1,rep,name=parentHashes,proto3" json:"parentHashes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BlockLevelParents) Reset() {
|
|
*x = BlockLevelParents{}
|
|
mi := &file_p2p_proto_msgTypes[12]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BlockLevelParents) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockLevelParents) ProtoMessage() {}
|
|
|
|
func (x *BlockLevelParents) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[12]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockLevelParents.ProtoReflect.Descriptor instead.
|
|
func (*BlockLevelParents) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{12}
|
|
}
|
|
|
|
func (x *BlockLevelParents) GetParentHashes() []*Hash {
|
|
if x != nil {
|
|
return x.ParentHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type Hash struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Bytes []byte `protobuf:"bytes,1,opt,name=bytes,proto3" json:"bytes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *Hash) Reset() {
|
|
*x = Hash{}
|
|
mi := &file_p2p_proto_msgTypes[13]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *Hash) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Hash) ProtoMessage() {}
|
|
|
|
func (x *Hash) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[13]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Hash.ProtoReflect.Descriptor instead.
|
|
func (*Hash) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{13}
|
|
}
|
|
|
|
func (x *Hash) GetBytes() []byte {
|
|
if x != nil {
|
|
return x.Bytes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RequestBlockLocatorMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
HighHash *Hash `protobuf:"bytes,1,opt,name=highHash,proto3" json:"highHash,omitempty"`
|
|
Limit uint32 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestBlockLocatorMessage) Reset() {
|
|
*x = RequestBlockLocatorMessage{}
|
|
mi := &file_p2p_proto_msgTypes[14]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestBlockLocatorMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestBlockLocatorMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestBlockLocatorMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[14]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestBlockLocatorMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestBlockLocatorMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{14}
|
|
}
|
|
|
|
func (x *RequestBlockLocatorMessage) GetHighHash() *Hash {
|
|
if x != nil {
|
|
return x.HighHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RequestBlockLocatorMessage) GetLimit() uint32 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type BlockLocatorMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Hashes []*Hash `protobuf:"bytes,1,rep,name=hashes,proto3" json:"hashes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BlockLocatorMessage) Reset() {
|
|
*x = BlockLocatorMessage{}
|
|
mi := &file_p2p_proto_msgTypes[15]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BlockLocatorMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockLocatorMessage) ProtoMessage() {}
|
|
|
|
func (x *BlockLocatorMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[15]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockLocatorMessage.ProtoReflect.Descriptor instead.
|
|
func (*BlockLocatorMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{15}
|
|
}
|
|
|
|
func (x *BlockLocatorMessage) GetHashes() []*Hash {
|
|
if x != nil {
|
|
return x.Hashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RequestHeadersMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
LowHash *Hash `protobuf:"bytes,1,opt,name=lowHash,proto3" json:"lowHash,omitempty"`
|
|
HighHash *Hash `protobuf:"bytes,2,opt,name=highHash,proto3" json:"highHash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestHeadersMessage) Reset() {
|
|
*x = RequestHeadersMessage{}
|
|
mi := &file_p2p_proto_msgTypes[16]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestHeadersMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestHeadersMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestHeadersMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[16]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestHeadersMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestHeadersMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{16}
|
|
}
|
|
|
|
func (x *RequestHeadersMessage) GetLowHash() *Hash {
|
|
if x != nil {
|
|
return x.LowHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RequestHeadersMessage) GetHighHash() *Hash {
|
|
if x != nil {
|
|
return x.HighHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RequestNextHeadersMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestNextHeadersMessage) Reset() {
|
|
*x = RequestNextHeadersMessage{}
|
|
mi := &file_p2p_proto_msgTypes[17]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestNextHeadersMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestNextHeadersMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestNextHeadersMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[17]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestNextHeadersMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestNextHeadersMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{17}
|
|
}
|
|
|
|
type DoneHeadersMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *DoneHeadersMessage) Reset() {
|
|
*x = DoneHeadersMessage{}
|
|
mi := &file_p2p_proto_msgTypes[18]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *DoneHeadersMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DoneHeadersMessage) ProtoMessage() {}
|
|
|
|
func (x *DoneHeadersMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[18]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DoneHeadersMessage.ProtoReflect.Descriptor instead.
|
|
func (*DoneHeadersMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{18}
|
|
}
|
|
|
|
type RequestRelayBlocksMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Hashes []*Hash `protobuf:"bytes,1,rep,name=hashes,proto3" json:"hashes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestRelayBlocksMessage) Reset() {
|
|
*x = RequestRelayBlocksMessage{}
|
|
mi := &file_p2p_proto_msgTypes[19]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestRelayBlocksMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestRelayBlocksMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestRelayBlocksMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[19]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestRelayBlocksMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestRelayBlocksMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{19}
|
|
}
|
|
|
|
func (x *RequestRelayBlocksMessage) GetHashes() []*Hash {
|
|
if x != nil {
|
|
return x.Hashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RequestTransactionsMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Ids []*TransactionId `protobuf:"bytes,1,rep,name=ids,proto3" json:"ids,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestTransactionsMessage) Reset() {
|
|
*x = RequestTransactionsMessage{}
|
|
mi := &file_p2p_proto_msgTypes[20]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestTransactionsMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestTransactionsMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestTransactionsMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[20]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestTransactionsMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestTransactionsMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{20}
|
|
}
|
|
|
|
func (x *RequestTransactionsMessage) GetIds() []*TransactionId {
|
|
if x != nil {
|
|
return x.Ids
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type TransactionNotFoundMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Id *TransactionId `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *TransactionNotFoundMessage) Reset() {
|
|
*x = TransactionNotFoundMessage{}
|
|
mi := &file_p2p_proto_msgTypes[21]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *TransactionNotFoundMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TransactionNotFoundMessage) ProtoMessage() {}
|
|
|
|
func (x *TransactionNotFoundMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[21]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TransactionNotFoundMessage.ProtoReflect.Descriptor instead.
|
|
func (*TransactionNotFoundMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{21}
|
|
}
|
|
|
|
func (x *TransactionNotFoundMessage) GetId() *TransactionId {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type InvRelayBlockMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Hash *Hash `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *InvRelayBlockMessage) Reset() {
|
|
*x = InvRelayBlockMessage{}
|
|
mi := &file_p2p_proto_msgTypes[22]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *InvRelayBlockMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InvRelayBlockMessage) ProtoMessage() {}
|
|
|
|
func (x *InvRelayBlockMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[22]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InvRelayBlockMessage.ProtoReflect.Descriptor instead.
|
|
func (*InvRelayBlockMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{22}
|
|
}
|
|
|
|
func (x *InvRelayBlockMessage) GetHash() *Hash {
|
|
if x != nil {
|
|
return x.Hash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type InvTransactionsMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Ids []*TransactionId `protobuf:"bytes,1,rep,name=ids,proto3" json:"ids,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *InvTransactionsMessage) Reset() {
|
|
*x = InvTransactionsMessage{}
|
|
mi := &file_p2p_proto_msgTypes[23]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *InvTransactionsMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InvTransactionsMessage) ProtoMessage() {}
|
|
|
|
func (x *InvTransactionsMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[23]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InvTransactionsMessage.ProtoReflect.Descriptor instead.
|
|
func (*InvTransactionsMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{23}
|
|
}
|
|
|
|
func (x *InvTransactionsMessage) GetIds() []*TransactionId {
|
|
if x != nil {
|
|
return x.Ids
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type PingMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Nonce uint64 `protobuf:"varint,1,opt,name=nonce,proto3" json:"nonce,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *PingMessage) Reset() {
|
|
*x = PingMessage{}
|
|
mi := &file_p2p_proto_msgTypes[24]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *PingMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PingMessage) ProtoMessage() {}
|
|
|
|
func (x *PingMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[24]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PingMessage.ProtoReflect.Descriptor instead.
|
|
func (*PingMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{24}
|
|
}
|
|
|
|
func (x *PingMessage) GetNonce() uint64 {
|
|
if x != nil {
|
|
return x.Nonce
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type PongMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Nonce uint64 `protobuf:"varint,1,opt,name=nonce,proto3" json:"nonce,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *PongMessage) Reset() {
|
|
*x = PongMessage{}
|
|
mi := &file_p2p_proto_msgTypes[25]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *PongMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PongMessage) ProtoMessage() {}
|
|
|
|
func (x *PongMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[25]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PongMessage.ProtoReflect.Descriptor instead.
|
|
func (*PongMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{25}
|
|
}
|
|
|
|
func (x *PongMessage) GetNonce() uint64 {
|
|
if x != nil {
|
|
return x.Nonce
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type VerackMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *VerackMessage) Reset() {
|
|
*x = VerackMessage{}
|
|
mi := &file_p2p_proto_msgTypes[26]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *VerackMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VerackMessage) ProtoMessage() {}
|
|
|
|
func (x *VerackMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[26]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VerackMessage.ProtoReflect.Descriptor instead.
|
|
func (*VerackMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{26}
|
|
}
|
|
|
|
type VersionMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
ProtocolVersion uint32 `protobuf:"varint,1,opt,name=protocolVersion,proto3" json:"protocolVersion,omitempty"`
|
|
Services uint64 `protobuf:"varint,2,opt,name=services,proto3" json:"services,omitempty"`
|
|
Timestamp int64 `protobuf:"varint,3,opt,name=timestamp,proto3" json:"timestamp,omitempty"`
|
|
Address *NetAddress `protobuf:"bytes,4,opt,name=address,proto3" json:"address,omitempty"`
|
|
Id []byte `protobuf:"bytes,5,opt,name=id,proto3" json:"id,omitempty"`
|
|
UserAgent string `protobuf:"bytes,6,opt,name=userAgent,proto3" json:"userAgent,omitempty"`
|
|
DisableRelayTx bool `protobuf:"varint,8,opt,name=disableRelayTx,proto3" json:"disableRelayTx,omitempty"`
|
|
SubnetworkId *SubnetworkId `protobuf:"bytes,9,opt,name=subnetworkId,proto3" json:"subnetworkId,omitempty"`
|
|
Network string `protobuf:"bytes,10,opt,name=network,proto3" json:"network,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *VersionMessage) Reset() {
|
|
*x = VersionMessage{}
|
|
mi := &file_p2p_proto_msgTypes[27]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *VersionMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VersionMessage) ProtoMessage() {}
|
|
|
|
func (x *VersionMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[27]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VersionMessage.ProtoReflect.Descriptor instead.
|
|
func (*VersionMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{27}
|
|
}
|
|
|
|
func (x *VersionMessage) GetProtocolVersion() uint32 {
|
|
if x != nil {
|
|
return x.ProtocolVersion
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *VersionMessage) GetServices() uint64 {
|
|
if x != nil {
|
|
return x.Services
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *VersionMessage) GetTimestamp() int64 {
|
|
if x != nil {
|
|
return x.Timestamp
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *VersionMessage) GetAddress() *NetAddress {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VersionMessage) GetId() []byte {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VersionMessage) GetUserAgent() string {
|
|
if x != nil {
|
|
return x.UserAgent
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *VersionMessage) GetDisableRelayTx() bool {
|
|
if x != nil {
|
|
return x.DisableRelayTx
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *VersionMessage) GetSubnetworkId() *SubnetworkId {
|
|
if x != nil {
|
|
return x.SubnetworkId
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VersionMessage) GetNetwork() string {
|
|
if x != nil {
|
|
return x.Network
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type RejectMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Reason string `protobuf:"bytes,1,opt,name=reason,proto3" json:"reason,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RejectMessage) Reset() {
|
|
*x = RejectMessage{}
|
|
mi := &file_p2p_proto_msgTypes[28]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RejectMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RejectMessage) ProtoMessage() {}
|
|
|
|
func (x *RejectMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[28]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RejectMessage.ProtoReflect.Descriptor instead.
|
|
func (*RejectMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{28}
|
|
}
|
|
|
|
func (x *RejectMessage) GetReason() string {
|
|
if x != nil {
|
|
return x.Reason
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type RequestPruningPointUTXOSetMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
PruningPointHash *Hash `protobuf:"bytes,1,opt,name=pruningPointHash,proto3" json:"pruningPointHash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestPruningPointUTXOSetMessage) Reset() {
|
|
*x = RequestPruningPointUTXOSetMessage{}
|
|
mi := &file_p2p_proto_msgTypes[29]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestPruningPointUTXOSetMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestPruningPointUTXOSetMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestPruningPointUTXOSetMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[29]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestPruningPointUTXOSetMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestPruningPointUTXOSetMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{29}
|
|
}
|
|
|
|
func (x *RequestPruningPointUTXOSetMessage) GetPruningPointHash() *Hash {
|
|
if x != nil {
|
|
return x.PruningPointHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type PruningPointUtxoSetChunkMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
OutpointAndUtxoEntryPairs []*OutpointAndUtxoEntryPair `protobuf:"bytes,1,rep,name=outpointAndUtxoEntryPairs,proto3" json:"outpointAndUtxoEntryPairs,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *PruningPointUtxoSetChunkMessage) Reset() {
|
|
*x = PruningPointUtxoSetChunkMessage{}
|
|
mi := &file_p2p_proto_msgTypes[30]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *PruningPointUtxoSetChunkMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PruningPointUtxoSetChunkMessage) ProtoMessage() {}
|
|
|
|
func (x *PruningPointUtxoSetChunkMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[30]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PruningPointUtxoSetChunkMessage.ProtoReflect.Descriptor instead.
|
|
func (*PruningPointUtxoSetChunkMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{30}
|
|
}
|
|
|
|
func (x *PruningPointUtxoSetChunkMessage) GetOutpointAndUtxoEntryPairs() []*OutpointAndUtxoEntryPair {
|
|
if x != nil {
|
|
return x.OutpointAndUtxoEntryPairs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type OutpointAndUtxoEntryPair struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Outpoint *Outpoint `protobuf:"bytes,1,opt,name=outpoint,proto3" json:"outpoint,omitempty"`
|
|
UtxoEntry *UtxoEntry `protobuf:"bytes,2,opt,name=utxoEntry,proto3" json:"utxoEntry,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *OutpointAndUtxoEntryPair) Reset() {
|
|
*x = OutpointAndUtxoEntryPair{}
|
|
mi := &file_p2p_proto_msgTypes[31]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *OutpointAndUtxoEntryPair) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*OutpointAndUtxoEntryPair) ProtoMessage() {}
|
|
|
|
func (x *OutpointAndUtxoEntryPair) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[31]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use OutpointAndUtxoEntryPair.ProtoReflect.Descriptor instead.
|
|
func (*OutpointAndUtxoEntryPair) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{31}
|
|
}
|
|
|
|
func (x *OutpointAndUtxoEntryPair) GetOutpoint() *Outpoint {
|
|
if x != nil {
|
|
return x.Outpoint
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *OutpointAndUtxoEntryPair) GetUtxoEntry() *UtxoEntry {
|
|
if x != nil {
|
|
return x.UtxoEntry
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UtxoEntry struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Amount uint64 `protobuf:"varint,1,opt,name=amount,proto3" json:"amount,omitempty"`
|
|
ScriptPublicKey *ScriptPublicKey `protobuf:"bytes,2,opt,name=scriptPublicKey,proto3" json:"scriptPublicKey,omitempty"`
|
|
BlockDaaScore uint64 `protobuf:"varint,3,opt,name=blockDaaScore,proto3" json:"blockDaaScore,omitempty"`
|
|
IsCoinbase bool `protobuf:"varint,4,opt,name=isCoinbase,proto3" json:"isCoinbase,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *UtxoEntry) Reset() {
|
|
*x = UtxoEntry{}
|
|
mi := &file_p2p_proto_msgTypes[32]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *UtxoEntry) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UtxoEntry) ProtoMessage() {}
|
|
|
|
func (x *UtxoEntry) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[32]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UtxoEntry.ProtoReflect.Descriptor instead.
|
|
func (*UtxoEntry) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{32}
|
|
}
|
|
|
|
func (x *UtxoEntry) GetAmount() uint64 {
|
|
if x != nil {
|
|
return x.Amount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UtxoEntry) GetScriptPublicKey() *ScriptPublicKey {
|
|
if x != nil {
|
|
return x.ScriptPublicKey
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UtxoEntry) GetBlockDaaScore() uint64 {
|
|
if x != nil {
|
|
return x.BlockDaaScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UtxoEntry) GetIsCoinbase() bool {
|
|
if x != nil {
|
|
return x.IsCoinbase
|
|
}
|
|
return false
|
|
}
|
|
|
|
type RequestNextPruningPointUtxoSetChunkMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestNextPruningPointUtxoSetChunkMessage) Reset() {
|
|
*x = RequestNextPruningPointUtxoSetChunkMessage{}
|
|
mi := &file_p2p_proto_msgTypes[33]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestNextPruningPointUtxoSetChunkMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestNextPruningPointUtxoSetChunkMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestNextPruningPointUtxoSetChunkMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[33]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestNextPruningPointUtxoSetChunkMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestNextPruningPointUtxoSetChunkMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{33}
|
|
}
|
|
|
|
type DonePruningPointUtxoSetChunksMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *DonePruningPointUtxoSetChunksMessage) Reset() {
|
|
*x = DonePruningPointUtxoSetChunksMessage{}
|
|
mi := &file_p2p_proto_msgTypes[34]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *DonePruningPointUtxoSetChunksMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DonePruningPointUtxoSetChunksMessage) ProtoMessage() {}
|
|
|
|
func (x *DonePruningPointUtxoSetChunksMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[34]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DonePruningPointUtxoSetChunksMessage.ProtoReflect.Descriptor instead.
|
|
func (*DonePruningPointUtxoSetChunksMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{34}
|
|
}
|
|
|
|
type RequestIBDBlocksMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Hashes []*Hash `protobuf:"bytes,1,rep,name=hashes,proto3" json:"hashes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestIBDBlocksMessage) Reset() {
|
|
*x = RequestIBDBlocksMessage{}
|
|
mi := &file_p2p_proto_msgTypes[35]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestIBDBlocksMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestIBDBlocksMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestIBDBlocksMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[35]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestIBDBlocksMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestIBDBlocksMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{35}
|
|
}
|
|
|
|
func (x *RequestIBDBlocksMessage) GetHashes() []*Hash {
|
|
if x != nil {
|
|
return x.Hashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UnexpectedPruningPointMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *UnexpectedPruningPointMessage) Reset() {
|
|
*x = UnexpectedPruningPointMessage{}
|
|
mi := &file_p2p_proto_msgTypes[36]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *UnexpectedPruningPointMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UnexpectedPruningPointMessage) ProtoMessage() {}
|
|
|
|
func (x *UnexpectedPruningPointMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[36]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UnexpectedPruningPointMessage.ProtoReflect.Descriptor instead.
|
|
func (*UnexpectedPruningPointMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{36}
|
|
}
|
|
|
|
type IbdBlockLocatorMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
TargetHash *Hash `protobuf:"bytes,1,opt,name=targetHash,proto3" json:"targetHash,omitempty"`
|
|
BlockLocatorHashes []*Hash `protobuf:"bytes,2,rep,name=blockLocatorHashes,proto3" json:"blockLocatorHashes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *IbdBlockLocatorMessage) Reset() {
|
|
*x = IbdBlockLocatorMessage{}
|
|
mi := &file_p2p_proto_msgTypes[37]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *IbdBlockLocatorMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*IbdBlockLocatorMessage) ProtoMessage() {}
|
|
|
|
func (x *IbdBlockLocatorMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[37]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use IbdBlockLocatorMessage.ProtoReflect.Descriptor instead.
|
|
func (*IbdBlockLocatorMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{37}
|
|
}
|
|
|
|
func (x *IbdBlockLocatorMessage) GetTargetHash() *Hash {
|
|
if x != nil {
|
|
return x.TargetHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *IbdBlockLocatorMessage) GetBlockLocatorHashes() []*Hash {
|
|
if x != nil {
|
|
return x.BlockLocatorHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RequestIBDChainBlockLocatorMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
LowHash *Hash `protobuf:"bytes,1,opt,name=lowHash,proto3" json:"lowHash,omitempty"`
|
|
HighHash *Hash `protobuf:"bytes,2,opt,name=highHash,proto3" json:"highHash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestIBDChainBlockLocatorMessage) Reset() {
|
|
*x = RequestIBDChainBlockLocatorMessage{}
|
|
mi := &file_p2p_proto_msgTypes[38]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestIBDChainBlockLocatorMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestIBDChainBlockLocatorMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestIBDChainBlockLocatorMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[38]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestIBDChainBlockLocatorMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestIBDChainBlockLocatorMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{38}
|
|
}
|
|
|
|
func (x *RequestIBDChainBlockLocatorMessage) GetLowHash() *Hash {
|
|
if x != nil {
|
|
return x.LowHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RequestIBDChainBlockLocatorMessage) GetHighHash() *Hash {
|
|
if x != nil {
|
|
return x.HighHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type IbdChainBlockLocatorMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BlockLocatorHashes []*Hash `protobuf:"bytes,1,rep,name=blockLocatorHashes,proto3" json:"blockLocatorHashes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *IbdChainBlockLocatorMessage) Reset() {
|
|
*x = IbdChainBlockLocatorMessage{}
|
|
mi := &file_p2p_proto_msgTypes[39]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *IbdChainBlockLocatorMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*IbdChainBlockLocatorMessage) ProtoMessage() {}
|
|
|
|
func (x *IbdChainBlockLocatorMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[39]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use IbdChainBlockLocatorMessage.ProtoReflect.Descriptor instead.
|
|
func (*IbdChainBlockLocatorMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{39}
|
|
}
|
|
|
|
func (x *IbdChainBlockLocatorMessage) GetBlockLocatorHashes() []*Hash {
|
|
if x != nil {
|
|
return x.BlockLocatorHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RequestAnticoneMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BlockHash *Hash `protobuf:"bytes,1,opt,name=blockHash,proto3" json:"blockHash,omitempty"`
|
|
ContextHash *Hash `protobuf:"bytes,2,opt,name=contextHash,proto3" json:"contextHash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestAnticoneMessage) Reset() {
|
|
*x = RequestAnticoneMessage{}
|
|
mi := &file_p2p_proto_msgTypes[40]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestAnticoneMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestAnticoneMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestAnticoneMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[40]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestAnticoneMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestAnticoneMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{40}
|
|
}
|
|
|
|
func (x *RequestAnticoneMessage) GetBlockHash() *Hash {
|
|
if x != nil {
|
|
return x.BlockHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RequestAnticoneMessage) GetContextHash() *Hash {
|
|
if x != nil {
|
|
return x.ContextHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type IbdBlockLocatorHighestHashMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
HighestHash *Hash `protobuf:"bytes,1,opt,name=highestHash,proto3" json:"highestHash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *IbdBlockLocatorHighestHashMessage) Reset() {
|
|
*x = IbdBlockLocatorHighestHashMessage{}
|
|
mi := &file_p2p_proto_msgTypes[41]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *IbdBlockLocatorHighestHashMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*IbdBlockLocatorHighestHashMessage) ProtoMessage() {}
|
|
|
|
func (x *IbdBlockLocatorHighestHashMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[41]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use IbdBlockLocatorHighestHashMessage.ProtoReflect.Descriptor instead.
|
|
func (*IbdBlockLocatorHighestHashMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{41}
|
|
}
|
|
|
|
func (x *IbdBlockLocatorHighestHashMessage) GetHighestHash() *Hash {
|
|
if x != nil {
|
|
return x.HighestHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type IbdBlockLocatorHighestHashNotFoundMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *IbdBlockLocatorHighestHashNotFoundMessage) Reset() {
|
|
*x = IbdBlockLocatorHighestHashNotFoundMessage{}
|
|
mi := &file_p2p_proto_msgTypes[42]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *IbdBlockLocatorHighestHashNotFoundMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*IbdBlockLocatorHighestHashNotFoundMessage) ProtoMessage() {}
|
|
|
|
func (x *IbdBlockLocatorHighestHashNotFoundMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[42]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use IbdBlockLocatorHighestHashNotFoundMessage.ProtoReflect.Descriptor instead.
|
|
func (*IbdBlockLocatorHighestHashNotFoundMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{42}
|
|
}
|
|
|
|
type BlockHeadersMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BlockHeaders []*BlockHeader `protobuf:"bytes,1,rep,name=blockHeaders,proto3" json:"blockHeaders,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BlockHeadersMessage) Reset() {
|
|
*x = BlockHeadersMessage{}
|
|
mi := &file_p2p_proto_msgTypes[43]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BlockHeadersMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockHeadersMessage) ProtoMessage() {}
|
|
|
|
func (x *BlockHeadersMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[43]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockHeadersMessage.ProtoReflect.Descriptor instead.
|
|
func (*BlockHeadersMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{43}
|
|
}
|
|
|
|
func (x *BlockHeadersMessage) GetBlockHeaders() []*BlockHeader {
|
|
if x != nil {
|
|
return x.BlockHeaders
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RequestPruningPointAndItsAnticoneMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestPruningPointAndItsAnticoneMessage) Reset() {
|
|
*x = RequestPruningPointAndItsAnticoneMessage{}
|
|
mi := &file_p2p_proto_msgTypes[44]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestPruningPointAndItsAnticoneMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestPruningPointAndItsAnticoneMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestPruningPointAndItsAnticoneMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[44]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestPruningPointAndItsAnticoneMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestPruningPointAndItsAnticoneMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{44}
|
|
}
|
|
|
|
type RequestNextPruningPointAndItsAnticoneBlocksMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestNextPruningPointAndItsAnticoneBlocksMessage) Reset() {
|
|
*x = RequestNextPruningPointAndItsAnticoneBlocksMessage{}
|
|
mi := &file_p2p_proto_msgTypes[45]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestNextPruningPointAndItsAnticoneBlocksMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestNextPruningPointAndItsAnticoneBlocksMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestNextPruningPointAndItsAnticoneBlocksMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[45]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestNextPruningPointAndItsAnticoneBlocksMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestNextPruningPointAndItsAnticoneBlocksMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{45}
|
|
}
|
|
|
|
type BlockWithTrustedDataMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Block *BlockMessage `protobuf:"bytes,1,opt,name=block,proto3" json:"block,omitempty"`
|
|
DaaScore uint64 `protobuf:"varint,2,opt,name=daaScore,proto3" json:"daaScore,omitempty"`
|
|
DaaWindow []*DaaBlock `protobuf:"bytes,3,rep,name=daaWindow,proto3" json:"daaWindow,omitempty"`
|
|
GhostdagData []*BlockGhostdagDataHashPair `protobuf:"bytes,4,rep,name=ghostdagData,proto3" json:"ghostdagData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataMessage) Reset() {
|
|
*x = BlockWithTrustedDataMessage{}
|
|
mi := &file_p2p_proto_msgTypes[46]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockWithTrustedDataMessage) ProtoMessage() {}
|
|
|
|
func (x *BlockWithTrustedDataMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[46]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockWithTrustedDataMessage.ProtoReflect.Descriptor instead.
|
|
func (*BlockWithTrustedDataMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{46}
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataMessage) GetBlock() *BlockMessage {
|
|
if x != nil {
|
|
return x.Block
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataMessage) GetDaaScore() uint64 {
|
|
if x != nil {
|
|
return x.DaaScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataMessage) GetDaaWindow() []*DaaBlock {
|
|
if x != nil {
|
|
return x.DaaWindow
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataMessage) GetGhostdagData() []*BlockGhostdagDataHashPair {
|
|
if x != nil {
|
|
return x.GhostdagData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type DaaBlock struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Block *BlockMessage `protobuf:"bytes,3,opt,name=block,proto3" json:"block,omitempty"`
|
|
GhostdagData *GhostdagData `protobuf:"bytes,2,opt,name=ghostdagData,proto3" json:"ghostdagData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *DaaBlock) Reset() {
|
|
*x = DaaBlock{}
|
|
mi := &file_p2p_proto_msgTypes[47]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *DaaBlock) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DaaBlock) ProtoMessage() {}
|
|
|
|
func (x *DaaBlock) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[47]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DaaBlock.ProtoReflect.Descriptor instead.
|
|
func (*DaaBlock) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{47}
|
|
}
|
|
|
|
func (x *DaaBlock) GetBlock() *BlockMessage {
|
|
if x != nil {
|
|
return x.Block
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *DaaBlock) GetGhostdagData() *GhostdagData {
|
|
if x != nil {
|
|
return x.GhostdagData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type DaaBlockV4 struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Header *BlockHeader `protobuf:"bytes,1,opt,name=header,proto3" json:"header,omitempty"`
|
|
GhostdagData *GhostdagData `protobuf:"bytes,2,opt,name=ghostdagData,proto3" json:"ghostdagData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *DaaBlockV4) Reset() {
|
|
*x = DaaBlockV4{}
|
|
mi := &file_p2p_proto_msgTypes[48]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *DaaBlockV4) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DaaBlockV4) ProtoMessage() {}
|
|
|
|
func (x *DaaBlockV4) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[48]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DaaBlockV4.ProtoReflect.Descriptor instead.
|
|
func (*DaaBlockV4) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{48}
|
|
}
|
|
|
|
func (x *DaaBlockV4) GetHeader() *BlockHeader {
|
|
if x != nil {
|
|
return x.Header
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *DaaBlockV4) GetGhostdagData() *GhostdagData {
|
|
if x != nil {
|
|
return x.GhostdagData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BlockGhostdagDataHashPair struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Hash *Hash `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
GhostdagData *GhostdagData `protobuf:"bytes,2,opt,name=ghostdagData,proto3" json:"ghostdagData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BlockGhostdagDataHashPair) Reset() {
|
|
*x = BlockGhostdagDataHashPair{}
|
|
mi := &file_p2p_proto_msgTypes[49]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BlockGhostdagDataHashPair) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockGhostdagDataHashPair) ProtoMessage() {}
|
|
|
|
func (x *BlockGhostdagDataHashPair) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[49]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockGhostdagDataHashPair.ProtoReflect.Descriptor instead.
|
|
func (*BlockGhostdagDataHashPair) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{49}
|
|
}
|
|
|
|
func (x *BlockGhostdagDataHashPair) GetHash() *Hash {
|
|
if x != nil {
|
|
return x.Hash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockGhostdagDataHashPair) GetGhostdagData() *GhostdagData {
|
|
if x != nil {
|
|
return x.GhostdagData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GhostdagData struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BlueScore uint64 `protobuf:"varint,1,opt,name=blueScore,proto3" json:"blueScore,omitempty"`
|
|
BlueWork []byte `protobuf:"bytes,2,opt,name=blueWork,proto3" json:"blueWork,omitempty"`
|
|
SelectedParent *Hash `protobuf:"bytes,3,opt,name=selectedParent,proto3" json:"selectedParent,omitempty"`
|
|
MergeSetBlues []*Hash `protobuf:"bytes,4,rep,name=mergeSetBlues,proto3" json:"mergeSetBlues,omitempty"`
|
|
MergeSetReds []*Hash `protobuf:"bytes,5,rep,name=mergeSetReds,proto3" json:"mergeSetReds,omitempty"`
|
|
BluesAnticoneSizes []*BluesAnticoneSizes `protobuf:"bytes,6,rep,name=bluesAnticoneSizes,proto3" json:"bluesAnticoneSizes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GhostdagData) Reset() {
|
|
*x = GhostdagData{}
|
|
mi := &file_p2p_proto_msgTypes[50]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GhostdagData) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GhostdagData) ProtoMessage() {}
|
|
|
|
func (x *GhostdagData) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[50]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GhostdagData.ProtoReflect.Descriptor instead.
|
|
func (*GhostdagData) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{50}
|
|
}
|
|
|
|
func (x *GhostdagData) GetBlueScore() uint64 {
|
|
if x != nil {
|
|
return x.BlueScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GhostdagData) GetBlueWork() []byte {
|
|
if x != nil {
|
|
return x.BlueWork
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GhostdagData) GetSelectedParent() *Hash {
|
|
if x != nil {
|
|
return x.SelectedParent
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GhostdagData) GetMergeSetBlues() []*Hash {
|
|
if x != nil {
|
|
return x.MergeSetBlues
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GhostdagData) GetMergeSetReds() []*Hash {
|
|
if x != nil {
|
|
return x.MergeSetReds
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GhostdagData) GetBluesAnticoneSizes() []*BluesAnticoneSizes {
|
|
if x != nil {
|
|
return x.BluesAnticoneSizes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BluesAnticoneSizes struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BlueHash *Hash `protobuf:"bytes,1,opt,name=blueHash,proto3" json:"blueHash,omitempty"`
|
|
AnticoneSize uint32 `protobuf:"varint,2,opt,name=anticoneSize,proto3" json:"anticoneSize,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BluesAnticoneSizes) Reset() {
|
|
*x = BluesAnticoneSizes{}
|
|
mi := &file_p2p_proto_msgTypes[51]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BluesAnticoneSizes) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BluesAnticoneSizes) ProtoMessage() {}
|
|
|
|
func (x *BluesAnticoneSizes) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[51]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BluesAnticoneSizes.ProtoReflect.Descriptor instead.
|
|
func (*BluesAnticoneSizes) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{51}
|
|
}
|
|
|
|
func (x *BluesAnticoneSizes) GetBlueHash() *Hash {
|
|
if x != nil {
|
|
return x.BlueHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BluesAnticoneSizes) GetAnticoneSize() uint32 {
|
|
if x != nil {
|
|
return x.AnticoneSize
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type DoneBlocksWithTrustedDataMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *DoneBlocksWithTrustedDataMessage) Reset() {
|
|
*x = DoneBlocksWithTrustedDataMessage{}
|
|
mi := &file_p2p_proto_msgTypes[52]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *DoneBlocksWithTrustedDataMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DoneBlocksWithTrustedDataMessage) ProtoMessage() {}
|
|
|
|
func (x *DoneBlocksWithTrustedDataMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[52]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DoneBlocksWithTrustedDataMessage.ProtoReflect.Descriptor instead.
|
|
func (*DoneBlocksWithTrustedDataMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{52}
|
|
}
|
|
|
|
type PruningPointsMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Headers []*BlockHeader `protobuf:"bytes,1,rep,name=headers,proto3" json:"headers,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *PruningPointsMessage) Reset() {
|
|
*x = PruningPointsMessage{}
|
|
mi := &file_p2p_proto_msgTypes[53]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *PruningPointsMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PruningPointsMessage) ProtoMessage() {}
|
|
|
|
func (x *PruningPointsMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[53]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PruningPointsMessage.ProtoReflect.Descriptor instead.
|
|
func (*PruningPointsMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{53}
|
|
}
|
|
|
|
func (x *PruningPointsMessage) GetHeaders() []*BlockHeader {
|
|
if x != nil {
|
|
return x.Headers
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RequestPruningPointProofMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RequestPruningPointProofMessage) Reset() {
|
|
*x = RequestPruningPointProofMessage{}
|
|
mi := &file_p2p_proto_msgTypes[54]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RequestPruningPointProofMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RequestPruningPointProofMessage) ProtoMessage() {}
|
|
|
|
func (x *RequestPruningPointProofMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[54]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RequestPruningPointProofMessage.ProtoReflect.Descriptor instead.
|
|
func (*RequestPruningPointProofMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{54}
|
|
}
|
|
|
|
type PruningPointProofMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Headers []*PruningPointProofHeaderArray `protobuf:"bytes,1,rep,name=headers,proto3" json:"headers,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *PruningPointProofMessage) Reset() {
|
|
*x = PruningPointProofMessage{}
|
|
mi := &file_p2p_proto_msgTypes[55]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *PruningPointProofMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PruningPointProofMessage) ProtoMessage() {}
|
|
|
|
func (x *PruningPointProofMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[55]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PruningPointProofMessage.ProtoReflect.Descriptor instead.
|
|
func (*PruningPointProofMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{55}
|
|
}
|
|
|
|
func (x *PruningPointProofMessage) GetHeaders() []*PruningPointProofHeaderArray {
|
|
if x != nil {
|
|
return x.Headers
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type PruningPointProofHeaderArray struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Headers []*BlockHeader `protobuf:"bytes,1,rep,name=headers,proto3" json:"headers,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *PruningPointProofHeaderArray) Reset() {
|
|
*x = PruningPointProofHeaderArray{}
|
|
mi := &file_p2p_proto_msgTypes[56]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *PruningPointProofHeaderArray) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PruningPointProofHeaderArray) ProtoMessage() {}
|
|
|
|
func (x *PruningPointProofHeaderArray) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[56]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PruningPointProofHeaderArray.ProtoReflect.Descriptor instead.
|
|
func (*PruningPointProofHeaderArray) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{56}
|
|
}
|
|
|
|
func (x *PruningPointProofHeaderArray) GetHeaders() []*BlockHeader {
|
|
if x != nil {
|
|
return x.Headers
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ReadyMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ReadyMessage) Reset() {
|
|
*x = ReadyMessage{}
|
|
mi := &file_p2p_proto_msgTypes[57]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ReadyMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ReadyMessage) ProtoMessage() {}
|
|
|
|
func (x *ReadyMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[57]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ReadyMessage.ProtoReflect.Descriptor instead.
|
|
func (*ReadyMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{57}
|
|
}
|
|
|
|
type BlockWithTrustedDataV4Message struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Block *BlockMessage `protobuf:"bytes,1,opt,name=block,proto3" json:"block,omitempty"`
|
|
DaaWindowIndices []uint64 `protobuf:"varint,2,rep,packed,name=daaWindowIndices,proto3" json:"daaWindowIndices,omitempty"`
|
|
GhostdagDataIndices []uint64 `protobuf:"varint,3,rep,packed,name=ghostdagDataIndices,proto3" json:"ghostdagDataIndices,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataV4Message) Reset() {
|
|
*x = BlockWithTrustedDataV4Message{}
|
|
mi := &file_p2p_proto_msgTypes[58]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataV4Message) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockWithTrustedDataV4Message) ProtoMessage() {}
|
|
|
|
func (x *BlockWithTrustedDataV4Message) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[58]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockWithTrustedDataV4Message.ProtoReflect.Descriptor instead.
|
|
func (*BlockWithTrustedDataV4Message) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{58}
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataV4Message) GetBlock() *BlockMessage {
|
|
if x != nil {
|
|
return x.Block
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataV4Message) GetDaaWindowIndices() []uint64 {
|
|
if x != nil {
|
|
return x.DaaWindowIndices
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockWithTrustedDataV4Message) GetGhostdagDataIndices() []uint64 {
|
|
if x != nil {
|
|
return x.GhostdagDataIndices
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type TrustedDataMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
DaaWindow []*DaaBlockV4 `protobuf:"bytes,1,rep,name=daaWindow,proto3" json:"daaWindow,omitempty"`
|
|
GhostdagData []*BlockGhostdagDataHashPair `protobuf:"bytes,2,rep,name=ghostdagData,proto3" json:"ghostdagData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *TrustedDataMessage) Reset() {
|
|
*x = TrustedDataMessage{}
|
|
mi := &file_p2p_proto_msgTypes[59]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *TrustedDataMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TrustedDataMessage) ProtoMessage() {}
|
|
|
|
func (x *TrustedDataMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_p2p_proto_msgTypes[59]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TrustedDataMessage.ProtoReflect.Descriptor instead.
|
|
func (*TrustedDataMessage) Descriptor() ([]byte, []int) {
|
|
return file_p2p_proto_rawDescGZIP(), []int{59}
|
|
}
|
|
|
|
func (x *TrustedDataMessage) GetDaaWindow() []*DaaBlockV4 {
|
|
if x != nil {
|
|
return x.DaaWindow
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *TrustedDataMessage) GetGhostdagData() []*BlockGhostdagDataHashPair {
|
|
if x != nil {
|
|
return x.GhostdagData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
var File_p2p_proto protoreflect.FileDescriptor
|
|
|
|
var file_p2p_proto_rawDesc = []byte{
|
|
0x0a, 0x09, 0x70, 0x32, 0x70, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x12, 0x09, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x22, 0x8c, 0x01, 0x0a, 0x17, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61,
|
|
0x67, 0x65, 0x12, 0x34, 0x0a, 0x15, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x41, 0x6c, 0x6c,
|
|
0x53, 0x75, 0x62, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x08, 0x52, 0x15, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x41, 0x6c, 0x6c, 0x53, 0x75, 0x62,
|
|
0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x73, 0x12, 0x3b, 0x0a, 0x0c, 0x73, 0x75, 0x62, 0x6e,
|
|
0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x17,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x53, 0x75, 0x62, 0x6e, 0x65,
|
|
0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x52, 0x0c, 0x73, 0x75, 0x62, 0x6e, 0x65, 0x74, 0x77,
|
|
0x6f, 0x72, 0x6b, 0x49, 0x64, 0x22, 0x4b, 0x0a, 0x10, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73,
|
|
0x65, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x37, 0x0a, 0x0b, 0x61, 0x64, 0x64,
|
|
0x72, 0x65, 0x73, 0x73, 0x4c, 0x69, 0x73, 0x74, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x15,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x4e, 0x65, 0x74, 0x41, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x52, 0x0b, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x4c, 0x69,
|
|
0x73, 0x74, 0x22, 0x4e, 0x0a, 0x0a, 0x4e, 0x65, 0x74, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73,
|
|
0x12, 0x1c, 0x0a, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x03, 0x52, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x12, 0x0e,
|
|
0x0a, 0x02, 0x69, 0x70, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x02, 0x69, 0x70, 0x12, 0x12,
|
|
0x0a, 0x04, 0x70, 0x6f, 0x72, 0x74, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x04, 0x70, 0x6f,
|
|
0x72, 0x74, 0x22, 0x24, 0x0a, 0x0c, 0x53, 0x75, 0x62, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b,
|
|
0x49, 0x64, 0x12, 0x14, 0x0a, 0x05, 0x62, 0x79, 0x74, 0x65, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x0c, 0x52, 0x05, 0x62, 0x79, 0x74, 0x65, 0x73, 0x22, 0xb4, 0x02, 0x0a, 0x12, 0x54, 0x72, 0x61,
|
|
0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x18, 0x0a, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d,
|
|
0x52, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x33, 0x0a, 0x06, 0x69, 0x6e, 0x70,
|
|
0x75, 0x74, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x52, 0x06, 0x69, 0x6e, 0x70, 0x75, 0x74, 0x73, 0x12, 0x36,
|
|
0x0a, 0x07, 0x6f, 0x75, 0x74, 0x70, 0x75, 0x74, 0x73, 0x18, 0x03, 0x20, 0x03, 0x28, 0x0b, 0x32,
|
|
0x1c, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x54, 0x72, 0x61, 0x6e,
|
|
0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4f, 0x75, 0x74, 0x70, 0x75, 0x74, 0x52, 0x07, 0x6f,
|
|
0x75, 0x74, 0x70, 0x75, 0x74, 0x73, 0x12, 0x1a, 0x0a, 0x08, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x69,
|
|
0x6d, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x69,
|
|
0x6d, 0x65, 0x12, 0x3b, 0x0a, 0x0c, 0x73, 0x75, 0x62, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b,
|
|
0x49, 0x64, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x77, 0x69, 0x72, 0x65, 0x2e, 0x53, 0x75, 0x62, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49,
|
|
0x64, 0x52, 0x0c, 0x73, 0x75, 0x62, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x12,
|
|
0x10, 0x0a, 0x03, 0x67, 0x61, 0x73, 0x18, 0x06, 0x20, 0x01, 0x28, 0x04, 0x52, 0x03, 0x67, 0x61,
|
|
0x73, 0x12, 0x18, 0x0a, 0x07, 0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x18, 0x08, 0x20, 0x01,
|
|
0x28, 0x0c, 0x52, 0x07, 0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x12, 0x12, 0x0a, 0x04, 0x6d,
|
|
0x61, 0x73, 0x73, 0x18, 0x09, 0x20, 0x01, 0x28, 0x04, 0x52, 0x04, 0x6d, 0x61, 0x73, 0x73, 0x22,
|
|
0xb9, 0x01, 0x0a, 0x10, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49,
|
|
0x6e, 0x70, 0x75, 0x74, 0x12, 0x3f, 0x0a, 0x10, 0x70, 0x72, 0x65, 0x76, 0x69, 0x6f, 0x75, 0x73,
|
|
0x4f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x4f, 0x75, 0x74, 0x70, 0x6f,
|
|
0x69, 0x6e, 0x74, 0x52, 0x10, 0x70, 0x72, 0x65, 0x76, 0x69, 0x6f, 0x75, 0x73, 0x4f, 0x75, 0x74,
|
|
0x70, 0x6f, 0x69, 0x6e, 0x74, 0x12, 0x28, 0x0a, 0x0f, 0x73, 0x69, 0x67, 0x6e, 0x61, 0x74, 0x75,
|
|
0x72, 0x65, 0x53, 0x63, 0x72, 0x69, 0x70, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x0f,
|
|
0x73, 0x69, 0x67, 0x6e, 0x61, 0x74, 0x75, 0x72, 0x65, 0x53, 0x63, 0x72, 0x69, 0x70, 0x74, 0x12,
|
|
0x1a, 0x0a, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x08, 0x73, 0x65, 0x71, 0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x73,
|
|
0x69, 0x67, 0x4f, 0x70, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0d, 0x52,
|
|
0x0a, 0x73, 0x69, 0x67, 0x4f, 0x70, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x22, 0x60, 0x0a, 0x08, 0x4f,
|
|
0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x12, 0x3e, 0x0a, 0x0d, 0x74, 0x72, 0x61, 0x6e, 0x73,
|
|
0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x18,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x54, 0x72, 0x61, 0x6e, 0x73,
|
|
0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x52, 0x0d, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61,
|
|
0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x12, 0x14, 0x0a, 0x05, 0x69, 0x6e, 0x64, 0x65, 0x78,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x05, 0x69, 0x6e, 0x64, 0x65, 0x78, 0x22, 0x25, 0x0a,
|
|
0x0d, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x12, 0x14,
|
|
0x0a, 0x05, 0x62, 0x79, 0x74, 0x65, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x05, 0x62,
|
|
0x79, 0x74, 0x65, 0x73, 0x22, 0x43, 0x0a, 0x0f, 0x53, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75,
|
|
0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x12, 0x16, 0x0a, 0x06, 0x73, 0x63, 0x72, 0x69, 0x70,
|
|
0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x06, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x12,
|
|
0x18, 0x0a, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0d,
|
|
0x52, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x22, 0x6f, 0x0a, 0x11, 0x54, 0x72, 0x61,
|
|
0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4f, 0x75, 0x74, 0x70, 0x75, 0x74, 0x12, 0x14,
|
|
0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x76,
|
|
0x61, 0x6c, 0x75, 0x65, 0x12, 0x44, 0x0a, 0x0f, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75,
|
|
0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x53, 0x63, 0x72, 0x69, 0x70, 0x74,
|
|
0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x52, 0x0f, 0x73, 0x63, 0x72, 0x69, 0x70,
|
|
0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x22, 0x81, 0x01, 0x0a, 0x0c, 0x42,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2e, 0x0a, 0x06, 0x68,
|
|
0x65, 0x61, 0x64, 0x65, 0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x16, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65, 0x61,
|
|
0x64, 0x65, 0x72, 0x52, 0x06, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x12, 0x41, 0x0a, 0x0c, 0x74,
|
|
0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28,
|
|
0x0b, 0x32, 0x1d, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x54, 0x72,
|
|
0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x52, 0x0c, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x22, 0xe9,
|
|
0x03, 0x0a, 0x0b, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x12, 0x18,
|
|
0x0a, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52,
|
|
0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x36, 0x0a, 0x07, 0x70, 0x61, 0x72, 0x65,
|
|
0x6e, 0x74, 0x73, 0x18, 0x0c, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4c, 0x65, 0x76, 0x65, 0x6c,
|
|
0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x73, 0x52, 0x07, 0x70, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x73,
|
|
0x12, 0x37, 0x0a, 0x0e, 0x68, 0x61, 0x73, 0x68, 0x4d, 0x65, 0x72, 0x6b, 0x6c, 0x65, 0x52, 0x6f,
|
|
0x6f, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x0e, 0x68, 0x61, 0x73, 0x68, 0x4d,
|
|
0x65, 0x72, 0x6b, 0x6c, 0x65, 0x52, 0x6f, 0x6f, 0x74, 0x12, 0x43, 0x0a, 0x14, 0x61, 0x63, 0x63,
|
|
0x65, 0x70, 0x74, 0x65, 0x64, 0x49, 0x64, 0x4d, 0x65, 0x72, 0x6b, 0x6c, 0x65, 0x52, 0x6f, 0x6f,
|
|
0x74, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x14, 0x61, 0x63, 0x63, 0x65, 0x70, 0x74,
|
|
0x65, 0x64, 0x49, 0x64, 0x4d, 0x65, 0x72, 0x6b, 0x6c, 0x65, 0x52, 0x6f, 0x6f, 0x74, 0x12, 0x37,
|
|
0x0a, 0x0e, 0x75, 0x74, 0x78, 0x6f, 0x43, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x6d, 0x65, 0x6e, 0x74,
|
|
0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x0e, 0x75, 0x74, 0x78, 0x6f, 0x43, 0x6f, 0x6d,
|
|
0x6d, 0x69, 0x74, 0x6d, 0x65, 0x6e, 0x74, 0x12, 0x1c, 0x0a, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73,
|
|
0x74, 0x61, 0x6d, 0x70, 0x18, 0x06, 0x20, 0x01, 0x28, 0x03, 0x52, 0x09, 0x74, 0x69, 0x6d, 0x65,
|
|
0x73, 0x74, 0x61, 0x6d, 0x70, 0x12, 0x12, 0x0a, 0x04, 0x62, 0x69, 0x74, 0x73, 0x18, 0x07, 0x20,
|
|
0x01, 0x28, 0x0d, 0x52, 0x04, 0x62, 0x69, 0x74, 0x73, 0x12, 0x14, 0x0a, 0x05, 0x6e, 0x6f, 0x6e,
|
|
0x63, 0x65, 0x18, 0x08, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x6e, 0x6f, 0x6e, 0x63, 0x65, 0x12,
|
|
0x1a, 0x0a, 0x08, 0x64, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x09, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x08, 0x64, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x1a, 0x0a, 0x08, 0x62,
|
|
0x6c, 0x75, 0x65, 0x57, 0x6f, 0x72, 0x6b, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x08, 0x62,
|
|
0x6c, 0x75, 0x65, 0x57, 0x6f, 0x72, 0x6b, 0x12, 0x33, 0x0a, 0x0c, 0x70, 0x72, 0x75, 0x6e, 0x69,
|
|
0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x18, 0x0e, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x0c,
|
|
0x70, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x12, 0x1c, 0x0a, 0x09,
|
|
0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x0d, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x09, 0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x22, 0x48, 0x0a, 0x11, 0x42, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x4c, 0x65, 0x76, 0x65, 0x6c, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x73, 0x12,
|
|
0x33, 0x0a, 0x0c, 0x70, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18,
|
|
0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x0c, 0x70, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x48, 0x61,
|
|
0x73, 0x68, 0x65, 0x73, 0x22, 0x1c, 0x0a, 0x04, 0x48, 0x61, 0x73, 0x68, 0x12, 0x14, 0x0a, 0x05,
|
|
0x62, 0x79, 0x74, 0x65, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x05, 0x62, 0x79, 0x74,
|
|
0x65, 0x73, 0x22, 0x5f, 0x0a, 0x1a, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x42, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x4c, 0x6f, 0x63, 0x61, 0x74, 0x6f, 0x72, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x12, 0x2b, 0x0a, 0x08, 0x68, 0x69, 0x67, 0x68, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48,
|
|
0x61, 0x73, 0x68, 0x52, 0x08, 0x68, 0x69, 0x67, 0x68, 0x48, 0x61, 0x73, 0x68, 0x12, 0x14, 0x0a,
|
|
0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x05, 0x6c, 0x69,
|
|
0x6d, 0x69, 0x74, 0x22, 0x3e, 0x0a, 0x13, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4c, 0x6f, 0x63, 0x61,
|
|
0x74, 0x6f, 0x72, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x27, 0x0a, 0x06, 0x68, 0x61,
|
|
0x73, 0x68, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x06, 0x68, 0x61, 0x73,
|
|
0x68, 0x65, 0x73, 0x22, 0x6f, 0x0a, 0x15, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x48, 0x65,
|
|
0x61, 0x64, 0x65, 0x72, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x29, 0x0a, 0x07,
|
|
0x6c, 0x6f, 0x77, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x07,
|
|
0x6c, 0x6f, 0x77, 0x48, 0x61, 0x73, 0x68, 0x12, 0x2b, 0x0a, 0x08, 0x68, 0x69, 0x67, 0x68, 0x48,
|
|
0x61, 0x73, 0x68, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x08, 0x68, 0x69, 0x67, 0x68,
|
|
0x48, 0x61, 0x73, 0x68, 0x22, 0x1b, 0x0a, 0x19, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4e,
|
|
0x65, 0x78, 0x74, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x22, 0x14, 0x0a, 0x12, 0x44, 0x6f, 0x6e, 0x65, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x73,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x44, 0x0a, 0x19, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x52, 0x65, 0x6c, 0x61, 0x79, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x4d, 0x65, 0x73,
|
|
0x73, 0x61, 0x67, 0x65, 0x12, 0x27, 0x0a, 0x06, 0x68, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18, 0x01,
|
|
0x20, 0x03, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65,
|
|
0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x06, 0x68, 0x61, 0x73, 0x68, 0x65, 0x73, 0x22, 0x48, 0x0a,
|
|
0x1a, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x03, 0x69,
|
|
0x64, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x18, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x77, 0x69, 0x72, 0x65, 0x2e, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x49, 0x64, 0x52, 0x03, 0x69, 0x64, 0x73, 0x22, 0x46, 0x0a, 0x1a, 0x54, 0x72, 0x61, 0x6e, 0x73,
|
|
0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4e, 0x6f, 0x74, 0x46, 0x6f, 0x75, 0x6e, 0x64, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x28, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x18, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x54, 0x72,
|
|
0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x52, 0x02, 0x69, 0x64, 0x22,
|
|
0x3b, 0x0a, 0x14, 0x49, 0x6e, 0x76, 0x52, 0x65, 0x6c, 0x61, 0x79, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x23, 0x0a, 0x04, 0x68, 0x61, 0x73, 0x68, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x04, 0x68, 0x61, 0x73, 0x68, 0x22, 0x44, 0x0a, 0x16,
|
|
0x49, 0x6e, 0x76, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x03, 0x69, 0x64, 0x73, 0x18, 0x01, 0x20,
|
|
0x03, 0x28, 0x0b, 0x32, 0x18, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e,
|
|
0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x52, 0x03, 0x69,
|
|
0x64, 0x73, 0x22, 0x23, 0x0a, 0x0b, 0x50, 0x69, 0x6e, 0x67, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x14, 0x0a, 0x05, 0x6e, 0x6f, 0x6e, 0x63, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04,
|
|
0x52, 0x05, 0x6e, 0x6f, 0x6e, 0x63, 0x65, 0x22, 0x23, 0x0a, 0x0b, 0x50, 0x6f, 0x6e, 0x67, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x14, 0x0a, 0x05, 0x6e, 0x6f, 0x6e, 0x63, 0x65, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x6e, 0x6f, 0x6e, 0x63, 0x65, 0x22, 0x0f, 0x0a, 0x0d,
|
|
0x56, 0x65, 0x72, 0x61, 0x63, 0x6b, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0xd2, 0x02,
|
|
0x0a, 0x0e, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x12, 0x28, 0x0a, 0x0f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x56, 0x65, 0x72, 0x73,
|
|
0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0f, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x63, 0x6f, 0x6c, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65,
|
|
0x72, 0x76, 0x69, 0x63, 0x65, 0x73, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x73, 0x65,
|
|
0x72, 0x76, 0x69, 0x63, 0x65, 0x73, 0x12, 0x1c, 0x0a, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74,
|
|
0x61, 0x6d, 0x70, 0x18, 0x03, 0x20, 0x01, 0x28, 0x03, 0x52, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73,
|
|
0x74, 0x61, 0x6d, 0x70, 0x12, 0x2f, 0x0a, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18,
|
|
0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x15, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x4e, 0x65, 0x74, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x52, 0x07, 0x61, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x05, 0x20, 0x01, 0x28,
|
|
0x0c, 0x52, 0x02, 0x69, 0x64, 0x12, 0x1c, 0x0a, 0x09, 0x75, 0x73, 0x65, 0x72, 0x41, 0x67, 0x65,
|
|
0x6e, 0x74, 0x18, 0x06, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x75, 0x73, 0x65, 0x72, 0x41, 0x67,
|
|
0x65, 0x6e, 0x74, 0x12, 0x26, 0x0a, 0x0e, 0x64, 0x69, 0x73, 0x61, 0x62, 0x6c, 0x65, 0x52, 0x65,
|
|
0x6c, 0x61, 0x79, 0x54, 0x78, 0x18, 0x08, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0e, 0x64, 0x69, 0x73,
|
|
0x61, 0x62, 0x6c, 0x65, 0x52, 0x65, 0x6c, 0x61, 0x79, 0x54, 0x78, 0x12, 0x3b, 0x0a, 0x0c, 0x73,
|
|
0x75, 0x62, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x18, 0x09, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x53, 0x75,
|
|
0x62, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x52, 0x0c, 0x73, 0x75, 0x62, 0x6e,
|
|
0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x12, 0x18, 0x0a, 0x07, 0x6e, 0x65, 0x74, 0x77,
|
|
0x6f, 0x72, 0x6b, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x6e, 0x65, 0x74, 0x77, 0x6f,
|
|
0x72, 0x6b, 0x22, 0x27, 0x0a, 0x0d, 0x52, 0x65, 0x6a, 0x65, 0x63, 0x74, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x12, 0x16, 0x0a, 0x06, 0x72, 0x65, 0x61, 0x73, 0x6f, 0x6e, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x06, 0x72, 0x65, 0x61, 0x73, 0x6f, 0x6e, 0x22, 0x60, 0x0a, 0x21, 0x52,
|
|
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x50, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69,
|
|
0x6e, 0x74, 0x55, 0x54, 0x58, 0x4f, 0x53, 0x65, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x12, 0x3b, 0x0a, 0x10, 0x70, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74,
|
|
0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x10, 0x70, 0x72, 0x75,
|
|
0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x48, 0x61, 0x73, 0x68, 0x22, 0x84, 0x01,
|
|
0x0a, 0x1f, 0x50, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x55, 0x74,
|
|
0x78, 0x6f, 0x53, 0x65, 0x74, 0x43, 0x68, 0x75, 0x6e, 0x6b, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x61, 0x0a, 0x19, 0x6f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x41, 0x6e, 0x64,
|
|
0x55, 0x74, 0x78, 0x6f, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x50, 0x61, 0x69, 0x72, 0x73, 0x18, 0x01,
|
|
0x20, 0x03, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65,
|
|
0x2e, 0x4f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x41, 0x6e, 0x64, 0x55, 0x74, 0x78, 0x6f,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x50, 0x61, 0x69, 0x72, 0x52, 0x19, 0x6f, 0x75, 0x74, 0x70, 0x6f,
|
|
0x69, 0x6e, 0x74, 0x41, 0x6e, 0x64, 0x55, 0x74, 0x78, 0x6f, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x50,
|
|
0x61, 0x69, 0x72, 0x73, 0x22, 0x7f, 0x0a, 0x18, 0x4f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74,
|
|
0x41, 0x6e, 0x64, 0x55, 0x74, 0x78, 0x6f, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x50, 0x61, 0x69, 0x72,
|
|
0x12, 0x2f, 0x0a, 0x08, 0x6f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x4f,
|
|
0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x52, 0x08, 0x6f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e,
|
|
0x74, 0x12, 0x32, 0x0a, 0x09, 0x75, 0x74, 0x78, 0x6f, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x14, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65,
|
|
0x2e, 0x55, 0x74, 0x78, 0x6f, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x09, 0x75, 0x74, 0x78, 0x6f,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x22, 0xaf, 0x01, 0x0a, 0x09, 0x55, 0x74, 0x78, 0x6f, 0x45, 0x6e,
|
|
0x74, 0x72, 0x79, 0x12, 0x16, 0x0a, 0x06, 0x61, 0x6d, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x04, 0x52, 0x06, 0x61, 0x6d, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x44, 0x0a, 0x0f, 0x73,
|
|
0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65,
|
|
0x2e, 0x53, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79,
|
|
0x52, 0x0f, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65,
|
|
0x79, 0x12, 0x24, 0x0a, 0x0d, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f,
|
|
0x72, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0d, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x44,
|
|
0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x69, 0x73, 0x43, 0x6f, 0x69,
|
|
0x6e, 0x62, 0x61, 0x73, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0a, 0x69, 0x73, 0x43,
|
|
0x6f, 0x69, 0x6e, 0x62, 0x61, 0x73, 0x65, 0x22, 0x2c, 0x0a, 0x2a, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x4e, 0x65, 0x78, 0x74, 0x50, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69,
|
|
0x6e, 0x74, 0x55, 0x74, 0x78, 0x6f, 0x53, 0x65, 0x74, 0x43, 0x68, 0x75, 0x6e, 0x6b, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x26, 0x0a, 0x24, 0x44, 0x6f, 0x6e, 0x65, 0x50, 0x72, 0x75,
|
|
0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x55, 0x74, 0x78, 0x6f, 0x53, 0x65, 0x74,
|
|
0x43, 0x68, 0x75, 0x6e, 0x6b, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x42, 0x0a,
|
|
0x17, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x49, 0x42, 0x44, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x27, 0x0a, 0x06, 0x68, 0x61, 0x73, 0x68,
|
|
0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x06, 0x68, 0x61, 0x73, 0x68, 0x65,
|
|
0x73, 0x22, 0x1f, 0x0a, 0x1d, 0x55, 0x6e, 0x65, 0x78, 0x70, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50,
|
|
0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61,
|
|
0x67, 0x65, 0x22, 0x8a, 0x01, 0x0a, 0x16, 0x49, 0x62, 0x64, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4c,
|
|
0x6f, 0x63, 0x61, 0x74, 0x6f, 0x72, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2f, 0x0a,
|
|
0x0a, 0x74, 0x61, 0x72, 0x67, 0x65, 0x74, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61,
|
|
0x73, 0x68, 0x52, 0x0a, 0x74, 0x61, 0x72, 0x67, 0x65, 0x74, 0x48, 0x61, 0x73, 0x68, 0x12, 0x3f,
|
|
0x0a, 0x12, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x4c, 0x6f, 0x63, 0x61, 0x74, 0x6f, 0x72, 0x48, 0x61,
|
|
0x73, 0x68, 0x65, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x12, 0x62, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x4c, 0x6f, 0x63, 0x61, 0x74, 0x6f, 0x72, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x22,
|
|
0x7c, 0x0a, 0x22, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x49, 0x42, 0x44, 0x43, 0x68, 0x61,
|
|
0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4c, 0x6f, 0x63, 0x61, 0x74, 0x6f, 0x72, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x29, 0x0a, 0x07, 0x6c, 0x6f, 0x77, 0x48, 0x61, 0x73, 0x68,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x07, 0x6c, 0x6f, 0x77, 0x48, 0x61, 0x73, 0x68,
|
|
0x12, 0x2b, 0x0a, 0x08, 0x68, 0x69, 0x67, 0x68, 0x48, 0x61, 0x73, 0x68, 0x18, 0x02, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48,
|
|
0x61, 0x73, 0x68, 0x52, 0x08, 0x68, 0x69, 0x67, 0x68, 0x48, 0x61, 0x73, 0x68, 0x22, 0x5e, 0x0a,
|
|
0x1b, 0x49, 0x62, 0x64, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4c, 0x6f,
|
|
0x63, 0x61, 0x74, 0x6f, 0x72, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x3f, 0x0a, 0x12,
|
|
0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x4c, 0x6f, 0x63, 0x61, 0x74, 0x6f, 0x72, 0x48, 0x61, 0x73, 0x68,
|
|
0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x12, 0x62, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x4c, 0x6f, 0x63, 0x61, 0x74, 0x6f, 0x72, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x22, 0x7a, 0x0a,
|
|
0x16, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x41, 0x6e, 0x74, 0x69, 0x63, 0x6f, 0x6e, 0x65,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2d, 0x0a, 0x09, 0x62, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x09, 0x62, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x12, 0x31, 0x0a, 0x0b, 0x63, 0x6f, 0x6e, 0x74, 0x65, 0x78,
|
|
0x74, 0x48, 0x61, 0x73, 0x68, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x0b, 0x63, 0x6f,
|
|
0x6e, 0x74, 0x65, 0x78, 0x74, 0x48, 0x61, 0x73, 0x68, 0x22, 0x56, 0x0a, 0x21, 0x49, 0x62, 0x64,
|
|
0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4c, 0x6f, 0x63, 0x61, 0x74, 0x6f, 0x72, 0x48, 0x69, 0x67, 0x68,
|
|
0x65, 0x73, 0x74, 0x48, 0x61, 0x73, 0x68, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x31,
|
|
0x0a, 0x0b, 0x68, 0x69, 0x67, 0x68, 0x65, 0x73, 0x74, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e,
|
|
0x48, 0x61, 0x73, 0x68, 0x52, 0x0b, 0x68, 0x69, 0x67, 0x68, 0x65, 0x73, 0x74, 0x48, 0x61, 0x73,
|
|
0x68, 0x22, 0x2b, 0x0a, 0x29, 0x49, 0x62, 0x64, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4c, 0x6f, 0x63,
|
|
0x61, 0x74, 0x6f, 0x72, 0x48, 0x69, 0x67, 0x68, 0x65, 0x73, 0x74, 0x48, 0x61, 0x73, 0x68, 0x4e,
|
|
0x6f, 0x74, 0x46, 0x6f, 0x75, 0x6e, 0x64, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x51,
|
|
0x0a, 0x13, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x73, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x3a, 0x0a, 0x0c, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65,
|
|
0x61, 0x64, 0x65, 0x72, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x16, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65, 0x61,
|
|
0x64, 0x65, 0x72, 0x52, 0x0c, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72,
|
|
0x73, 0x22, 0x2a, 0x0a, 0x28, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x50, 0x72, 0x75, 0x6e,
|
|
0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x41, 0x6e, 0x64, 0x49, 0x74, 0x73, 0x41, 0x6e,
|
|
0x74, 0x69, 0x63, 0x6f, 0x6e, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x34, 0x0a,
|
|
0x32, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4e, 0x65, 0x78, 0x74, 0x50, 0x72, 0x75, 0x6e,
|
|
0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x41, 0x6e, 0x64, 0x49, 0x74, 0x73, 0x41, 0x6e,
|
|
0x74, 0x69, 0x63, 0x6f, 0x6e, 0x65, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x22, 0xe5, 0x01, 0x0a, 0x1b, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x57, 0x69, 0x74,
|
|
0x68, 0x54, 0x72, 0x75, 0x73, 0x74, 0x65, 0x64, 0x44, 0x61, 0x74, 0x61, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x12, 0x2d, 0x0a, 0x05, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x42,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x52, 0x05, 0x62, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x12, 0x1a, 0x0a, 0x08, 0x64, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x64, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x31,
|
|
0x0a, 0x09, 0x64, 0x61, 0x61, 0x57, 0x69, 0x6e, 0x64, 0x6f, 0x77, 0x18, 0x03, 0x20, 0x03, 0x28,
|
|
0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x44, 0x61,
|
|
0x61, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x09, 0x64, 0x61, 0x61, 0x57, 0x69, 0x6e, 0x64, 0x6f,
|
|
0x77, 0x12, 0x48, 0x0a, 0x0c, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74,
|
|
0x61, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x24, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x47, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61,
|
|
0x67, 0x44, 0x61, 0x74, 0x61, 0x48, 0x61, 0x73, 0x68, 0x50, 0x61, 0x69, 0x72, 0x52, 0x0c, 0x67,
|
|
0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61, 0x22, 0x76, 0x0a, 0x08, 0x44,
|
|
0x61, 0x61, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x12, 0x2d, 0x0a, 0x05, 0x62, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x52,
|
|
0x05, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x12, 0x3b, 0x0a, 0x0c, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64,
|
|
0x61, 0x67, 0x44, 0x61, 0x74, 0x61, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x47, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61,
|
|
0x67, 0x44, 0x61, 0x74, 0x61, 0x52, 0x0c, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44,
|
|
0x61, 0x74, 0x61, 0x22, 0x79, 0x0a, 0x0a, 0x44, 0x61, 0x61, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x56,
|
|
0x34, 0x12, 0x2e, 0x0a, 0x06, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x16, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x42, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x52, 0x06, 0x68, 0x65, 0x61, 0x64, 0x65,
|
|
0x72, 0x12, 0x3b, 0x0a, 0x0c, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74,
|
|
0x61, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x47, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61,
|
|
0x52, 0x0c, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61, 0x22, 0x7d,
|
|
0x0a, 0x19, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x47, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44,
|
|
0x61, 0x74, 0x61, 0x48, 0x61, 0x73, 0x68, 0x50, 0x61, 0x69, 0x72, 0x12, 0x23, 0x0a, 0x04, 0x68,
|
|
0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x04, 0x68, 0x61, 0x73, 0x68,
|
|
0x12, 0x3b, 0x0a, 0x0c, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x47, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61, 0x52,
|
|
0x0c, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61, 0x22, 0xbc, 0x02,
|
|
0x0a, 0x0c, 0x47, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61, 0x12, 0x1c,
|
|
0x0a, 0x09, 0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x09, 0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x1a, 0x0a, 0x08,
|
|
0x62, 0x6c, 0x75, 0x65, 0x57, 0x6f, 0x72, 0x6b, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x08,
|
|
0x62, 0x6c, 0x75, 0x65, 0x57, 0x6f, 0x72, 0x6b, 0x12, 0x37, 0x0a, 0x0e, 0x73, 0x65, 0x6c, 0x65,
|
|
0x63, 0x74, 0x65, 0x64, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73,
|
|
0x68, 0x52, 0x0e, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x61, 0x72, 0x65, 0x6e,
|
|
0x74, 0x12, 0x35, 0x0a, 0x0d, 0x6d, 0x65, 0x72, 0x67, 0x65, 0x53, 0x65, 0x74, 0x42, 0x6c, 0x75,
|
|
0x65, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x0d, 0x6d, 0x65, 0x72, 0x67, 0x65,
|
|
0x53, 0x65, 0x74, 0x42, 0x6c, 0x75, 0x65, 0x73, 0x12, 0x33, 0x0a, 0x0c, 0x6d, 0x65, 0x72, 0x67,
|
|
0x65, 0x53, 0x65, 0x74, 0x52, 0x65, 0x64, 0x73, 0x18, 0x05, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0f,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x48, 0x61, 0x73, 0x68, 0x52,
|
|
0x0c, 0x6d, 0x65, 0x72, 0x67, 0x65, 0x53, 0x65, 0x74, 0x52, 0x65, 0x64, 0x73, 0x12, 0x4d, 0x0a,
|
|
0x12, 0x62, 0x6c, 0x75, 0x65, 0x73, 0x41, 0x6e, 0x74, 0x69, 0x63, 0x6f, 0x6e, 0x65, 0x53, 0x69,
|
|
0x7a, 0x65, 0x73, 0x18, 0x06, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1d, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x42, 0x6c, 0x75, 0x65, 0x73, 0x41, 0x6e, 0x74, 0x69, 0x63,
|
|
0x6f, 0x6e, 0x65, 0x53, 0x69, 0x7a, 0x65, 0x73, 0x52, 0x12, 0x62, 0x6c, 0x75, 0x65, 0x73, 0x41,
|
|
0x6e, 0x74, 0x69, 0x63, 0x6f, 0x6e, 0x65, 0x53, 0x69, 0x7a, 0x65, 0x73, 0x22, 0x65, 0x0a, 0x12,
|
|
0x42, 0x6c, 0x75, 0x65, 0x73, 0x41, 0x6e, 0x74, 0x69, 0x63, 0x6f, 0x6e, 0x65, 0x53, 0x69, 0x7a,
|
|
0x65, 0x73, 0x12, 0x2b, 0x0a, 0x08, 0x62, 0x6c, 0x75, 0x65, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65,
|
|
0x2e, 0x48, 0x61, 0x73, 0x68, 0x52, 0x08, 0x62, 0x6c, 0x75, 0x65, 0x48, 0x61, 0x73, 0x68, 0x12,
|
|
0x22, 0x0a, 0x0c, 0x61, 0x6e, 0x74, 0x69, 0x63, 0x6f, 0x6e, 0x65, 0x53, 0x69, 0x7a, 0x65, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0c, 0x61, 0x6e, 0x74, 0x69, 0x63, 0x6f, 0x6e, 0x65, 0x53,
|
|
0x69, 0x7a, 0x65, 0x22, 0x22, 0x0a, 0x20, 0x44, 0x6f, 0x6e, 0x65, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x73, 0x57, 0x69, 0x74, 0x68, 0x54, 0x72, 0x75, 0x73, 0x74, 0x65, 0x64, 0x44, 0x61, 0x74, 0x61,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x48, 0x0a, 0x14, 0x50, 0x72, 0x75, 0x6e, 0x69,
|
|
0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x30, 0x0a, 0x07, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b,
|
|
0x32, 0x16, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x42, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x52, 0x07, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72,
|
|
0x73, 0x22, 0x21, 0x0a, 0x1f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x50, 0x72, 0x75, 0x6e,
|
|
0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x50, 0x72, 0x6f, 0x6f, 0x66, 0x4d, 0x65, 0x73,
|
|
0x73, 0x61, 0x67, 0x65, 0x22, 0x5d, 0x0a, 0x18, 0x50, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50,
|
|
0x6f, 0x69, 0x6e, 0x74, 0x50, 0x72, 0x6f, 0x6f, 0x66, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x12, 0x41, 0x0a, 0x07, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28,
|
|
0x0b, 0x32, 0x27, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x50, 0x72,
|
|
0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x50, 0x72, 0x6f, 0x6f, 0x66, 0x48,
|
|
0x65, 0x61, 0x64, 0x65, 0x72, 0x41, 0x72, 0x72, 0x61, 0x79, 0x52, 0x07, 0x68, 0x65, 0x61, 0x64,
|
|
0x65, 0x72, 0x73, 0x22, 0x50, 0x0a, 0x1c, 0x50, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f,
|
|
0x69, 0x6e, 0x74, 0x50, 0x72, 0x6f, 0x6f, 0x66, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x41, 0x72,
|
|
0x72, 0x61, 0x79, 0x12, 0x30, 0x0a, 0x07, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x73, 0x18, 0x01,
|
|
0x20, 0x03, 0x28, 0x0b, 0x32, 0x16, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65,
|
|
0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x52, 0x07, 0x68, 0x65,
|
|
0x61, 0x64, 0x65, 0x72, 0x73, 0x22, 0x0e, 0x0a, 0x0c, 0x52, 0x65, 0x61, 0x64, 0x79, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0xac, 0x01, 0x0a, 0x1d, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x57,
|
|
0x69, 0x74, 0x68, 0x54, 0x72, 0x75, 0x73, 0x74, 0x65, 0x64, 0x44, 0x61, 0x74, 0x61, 0x56, 0x34,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2d, 0x0a, 0x05, 0x62, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x52,
|
|
0x05, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x12, 0x2a, 0x0a, 0x10, 0x64, 0x61, 0x61, 0x57, 0x69, 0x6e,
|
|
0x64, 0x6f, 0x77, 0x49, 0x6e, 0x64, 0x69, 0x63, 0x65, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x04,
|
|
0x52, 0x10, 0x64, 0x61, 0x61, 0x57, 0x69, 0x6e, 0x64, 0x6f, 0x77, 0x49, 0x6e, 0x64, 0x69, 0x63,
|
|
0x65, 0x73, 0x12, 0x30, 0x0a, 0x13, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61,
|
|
0x74, 0x61, 0x49, 0x6e, 0x64, 0x69, 0x63, 0x65, 0x73, 0x18, 0x03, 0x20, 0x03, 0x28, 0x04, 0x52,
|
|
0x13, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61, 0x49, 0x6e, 0x64,
|
|
0x69, 0x63, 0x65, 0x73, 0x22, 0x93, 0x01, 0x0a, 0x12, 0x54, 0x72, 0x75, 0x73, 0x74, 0x65, 0x64,
|
|
0x44, 0x61, 0x74, 0x61, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x33, 0x0a, 0x09, 0x64,
|
|
0x61, 0x61, 0x57, 0x69, 0x6e, 0x64, 0x6f, 0x77, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x15,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x44, 0x61, 0x61, 0x42, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x56, 0x34, 0x52, 0x09, 0x64, 0x61, 0x61, 0x57, 0x69, 0x6e, 0x64, 0x6f, 0x77,
|
|
0x12, 0x48, 0x0a, 0x0c, 0x67, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61,
|
|
0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x24, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x47, 0x68, 0x6f, 0x73, 0x74, 0x64, 0x61, 0x67,
|
|
0x44, 0x61, 0x74, 0x61, 0x48, 0x61, 0x73, 0x68, 0x50, 0x61, 0x69, 0x72, 0x52, 0x0c, 0x67, 0x68,
|
|
0x6f, 0x73, 0x74, 0x64, 0x61, 0x67, 0x44, 0x61, 0x74, 0x61, 0x42, 0x26, 0x5a, 0x24, 0x67, 0x69,
|
|
0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6b, 0x61, 0x73, 0x70, 0x61, 0x6e, 0x65,
|
|
0x74, 0x2f, 0x6b, 0x61, 0x73, 0x70, 0x61, 0x64, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
|
|
}
|
|
|
|
var (
|
|
file_p2p_proto_rawDescOnce sync.Once
|
|
file_p2p_proto_rawDescData = file_p2p_proto_rawDesc
|
|
)
|
|
|
|
func file_p2p_proto_rawDescGZIP() []byte {
|
|
file_p2p_proto_rawDescOnce.Do(func() {
|
|
file_p2p_proto_rawDescData = protoimpl.X.CompressGZIP(file_p2p_proto_rawDescData)
|
|
})
|
|
return file_p2p_proto_rawDescData
|
|
}
|
|
|
|
var file_p2p_proto_msgTypes = make([]protoimpl.MessageInfo, 60)
|
|
var file_p2p_proto_goTypes = []any{
|
|
(*RequestAddressesMessage)(nil), // 0: protowire.RequestAddressesMessage
|
|
(*AddressesMessage)(nil), // 1: protowire.AddressesMessage
|
|
(*NetAddress)(nil), // 2: protowire.NetAddress
|
|
(*SubnetworkId)(nil), // 3: protowire.SubnetworkId
|
|
(*TransactionMessage)(nil), // 4: protowire.TransactionMessage
|
|
(*TransactionInput)(nil), // 5: protowire.TransactionInput
|
|
(*Outpoint)(nil), // 6: protowire.Outpoint
|
|
(*TransactionId)(nil), // 7: protowire.TransactionId
|
|
(*ScriptPublicKey)(nil), // 8: protowire.ScriptPublicKey
|
|
(*TransactionOutput)(nil), // 9: protowire.TransactionOutput
|
|
(*BlockMessage)(nil), // 10: protowire.BlockMessage
|
|
(*BlockHeader)(nil), // 11: protowire.BlockHeader
|
|
(*BlockLevelParents)(nil), // 12: protowire.BlockLevelParents
|
|
(*Hash)(nil), // 13: protowire.Hash
|
|
(*RequestBlockLocatorMessage)(nil), // 14: protowire.RequestBlockLocatorMessage
|
|
(*BlockLocatorMessage)(nil), // 15: protowire.BlockLocatorMessage
|
|
(*RequestHeadersMessage)(nil), // 16: protowire.RequestHeadersMessage
|
|
(*RequestNextHeadersMessage)(nil), // 17: protowire.RequestNextHeadersMessage
|
|
(*DoneHeadersMessage)(nil), // 18: protowire.DoneHeadersMessage
|
|
(*RequestRelayBlocksMessage)(nil), // 19: protowire.RequestRelayBlocksMessage
|
|
(*RequestTransactionsMessage)(nil), // 20: protowire.RequestTransactionsMessage
|
|
(*TransactionNotFoundMessage)(nil), // 21: protowire.TransactionNotFoundMessage
|
|
(*InvRelayBlockMessage)(nil), // 22: protowire.InvRelayBlockMessage
|
|
(*InvTransactionsMessage)(nil), // 23: protowire.InvTransactionsMessage
|
|
(*PingMessage)(nil), // 24: protowire.PingMessage
|
|
(*PongMessage)(nil), // 25: protowire.PongMessage
|
|
(*VerackMessage)(nil), // 26: protowire.VerackMessage
|
|
(*VersionMessage)(nil), // 27: protowire.VersionMessage
|
|
(*RejectMessage)(nil), // 28: protowire.RejectMessage
|
|
(*RequestPruningPointUTXOSetMessage)(nil), // 29: protowire.RequestPruningPointUTXOSetMessage
|
|
(*PruningPointUtxoSetChunkMessage)(nil), // 30: protowire.PruningPointUtxoSetChunkMessage
|
|
(*OutpointAndUtxoEntryPair)(nil), // 31: protowire.OutpointAndUtxoEntryPair
|
|
(*UtxoEntry)(nil), // 32: protowire.UtxoEntry
|
|
(*RequestNextPruningPointUtxoSetChunkMessage)(nil), // 33: protowire.RequestNextPruningPointUtxoSetChunkMessage
|
|
(*DonePruningPointUtxoSetChunksMessage)(nil), // 34: protowire.DonePruningPointUtxoSetChunksMessage
|
|
(*RequestIBDBlocksMessage)(nil), // 35: protowire.RequestIBDBlocksMessage
|
|
(*UnexpectedPruningPointMessage)(nil), // 36: protowire.UnexpectedPruningPointMessage
|
|
(*IbdBlockLocatorMessage)(nil), // 37: protowire.IbdBlockLocatorMessage
|
|
(*RequestIBDChainBlockLocatorMessage)(nil), // 38: protowire.RequestIBDChainBlockLocatorMessage
|
|
(*IbdChainBlockLocatorMessage)(nil), // 39: protowire.IbdChainBlockLocatorMessage
|
|
(*RequestAnticoneMessage)(nil), // 40: protowire.RequestAnticoneMessage
|
|
(*IbdBlockLocatorHighestHashMessage)(nil), // 41: protowire.IbdBlockLocatorHighestHashMessage
|
|
(*IbdBlockLocatorHighestHashNotFoundMessage)(nil), // 42: protowire.IbdBlockLocatorHighestHashNotFoundMessage
|
|
(*BlockHeadersMessage)(nil), // 43: protowire.BlockHeadersMessage
|
|
(*RequestPruningPointAndItsAnticoneMessage)(nil), // 44: protowire.RequestPruningPointAndItsAnticoneMessage
|
|
(*RequestNextPruningPointAndItsAnticoneBlocksMessage)(nil), // 45: protowire.RequestNextPruningPointAndItsAnticoneBlocksMessage
|
|
(*BlockWithTrustedDataMessage)(nil), // 46: protowire.BlockWithTrustedDataMessage
|
|
(*DaaBlock)(nil), // 47: protowire.DaaBlock
|
|
(*DaaBlockV4)(nil), // 48: protowire.DaaBlockV4
|
|
(*BlockGhostdagDataHashPair)(nil), // 49: protowire.BlockGhostdagDataHashPair
|
|
(*GhostdagData)(nil), // 50: protowire.GhostdagData
|
|
(*BluesAnticoneSizes)(nil), // 51: protowire.BluesAnticoneSizes
|
|
(*DoneBlocksWithTrustedDataMessage)(nil), // 52: protowire.DoneBlocksWithTrustedDataMessage
|
|
(*PruningPointsMessage)(nil), // 53: protowire.PruningPointsMessage
|
|
(*RequestPruningPointProofMessage)(nil), // 54: protowire.RequestPruningPointProofMessage
|
|
(*PruningPointProofMessage)(nil), // 55: protowire.PruningPointProofMessage
|
|
(*PruningPointProofHeaderArray)(nil), // 56: protowire.PruningPointProofHeaderArray
|
|
(*ReadyMessage)(nil), // 57: protowire.ReadyMessage
|
|
(*BlockWithTrustedDataV4Message)(nil), // 58: protowire.BlockWithTrustedDataV4Message
|
|
(*TrustedDataMessage)(nil), // 59: protowire.TrustedDataMessage
|
|
}
|
|
var file_p2p_proto_depIdxs = []int32{
|
|
3, // 0: protowire.RequestAddressesMessage.subnetworkId:type_name -> protowire.SubnetworkId
|
|
2, // 1: protowire.AddressesMessage.addressList:type_name -> protowire.NetAddress
|
|
5, // 2: protowire.TransactionMessage.inputs:type_name -> protowire.TransactionInput
|
|
9, // 3: protowire.TransactionMessage.outputs:type_name -> protowire.TransactionOutput
|
|
3, // 4: protowire.TransactionMessage.subnetworkId:type_name -> protowire.SubnetworkId
|
|
6, // 5: protowire.TransactionInput.previousOutpoint:type_name -> protowire.Outpoint
|
|
7, // 6: protowire.Outpoint.transactionId:type_name -> protowire.TransactionId
|
|
8, // 7: protowire.TransactionOutput.scriptPublicKey:type_name -> protowire.ScriptPublicKey
|
|
11, // 8: protowire.BlockMessage.header:type_name -> protowire.BlockHeader
|
|
4, // 9: protowire.BlockMessage.transactions:type_name -> protowire.TransactionMessage
|
|
12, // 10: protowire.BlockHeader.parents:type_name -> protowire.BlockLevelParents
|
|
13, // 11: protowire.BlockHeader.hashMerkleRoot:type_name -> protowire.Hash
|
|
13, // 12: protowire.BlockHeader.acceptedIdMerkleRoot:type_name -> protowire.Hash
|
|
13, // 13: protowire.BlockHeader.utxoCommitment:type_name -> protowire.Hash
|
|
13, // 14: protowire.BlockHeader.pruningPoint:type_name -> protowire.Hash
|
|
13, // 15: protowire.BlockLevelParents.parentHashes:type_name -> protowire.Hash
|
|
13, // 16: protowire.RequestBlockLocatorMessage.highHash:type_name -> protowire.Hash
|
|
13, // 17: protowire.BlockLocatorMessage.hashes:type_name -> protowire.Hash
|
|
13, // 18: protowire.RequestHeadersMessage.lowHash:type_name -> protowire.Hash
|
|
13, // 19: protowire.RequestHeadersMessage.highHash:type_name -> protowire.Hash
|
|
13, // 20: protowire.RequestRelayBlocksMessage.hashes:type_name -> protowire.Hash
|
|
7, // 21: protowire.RequestTransactionsMessage.ids:type_name -> protowire.TransactionId
|
|
7, // 22: protowire.TransactionNotFoundMessage.id:type_name -> protowire.TransactionId
|
|
13, // 23: protowire.InvRelayBlockMessage.hash:type_name -> protowire.Hash
|
|
7, // 24: protowire.InvTransactionsMessage.ids:type_name -> protowire.TransactionId
|
|
2, // 25: protowire.VersionMessage.address:type_name -> protowire.NetAddress
|
|
3, // 26: protowire.VersionMessage.subnetworkId:type_name -> protowire.SubnetworkId
|
|
13, // 27: protowire.RequestPruningPointUTXOSetMessage.pruningPointHash:type_name -> protowire.Hash
|
|
31, // 28: protowire.PruningPointUtxoSetChunkMessage.outpointAndUtxoEntryPairs:type_name -> protowire.OutpointAndUtxoEntryPair
|
|
6, // 29: protowire.OutpointAndUtxoEntryPair.outpoint:type_name -> protowire.Outpoint
|
|
32, // 30: protowire.OutpointAndUtxoEntryPair.utxoEntry:type_name -> protowire.UtxoEntry
|
|
8, // 31: protowire.UtxoEntry.scriptPublicKey:type_name -> protowire.ScriptPublicKey
|
|
13, // 32: protowire.RequestIBDBlocksMessage.hashes:type_name -> protowire.Hash
|
|
13, // 33: protowire.IbdBlockLocatorMessage.targetHash:type_name -> protowire.Hash
|
|
13, // 34: protowire.IbdBlockLocatorMessage.blockLocatorHashes:type_name -> protowire.Hash
|
|
13, // 35: protowire.RequestIBDChainBlockLocatorMessage.lowHash:type_name -> protowire.Hash
|
|
13, // 36: protowire.RequestIBDChainBlockLocatorMessage.highHash:type_name -> protowire.Hash
|
|
13, // 37: protowire.IbdChainBlockLocatorMessage.blockLocatorHashes:type_name -> protowire.Hash
|
|
13, // 38: protowire.RequestAnticoneMessage.blockHash:type_name -> protowire.Hash
|
|
13, // 39: protowire.RequestAnticoneMessage.contextHash:type_name -> protowire.Hash
|
|
13, // 40: protowire.IbdBlockLocatorHighestHashMessage.highestHash:type_name -> protowire.Hash
|
|
11, // 41: protowire.BlockHeadersMessage.blockHeaders:type_name -> protowire.BlockHeader
|
|
10, // 42: protowire.BlockWithTrustedDataMessage.block:type_name -> protowire.BlockMessage
|
|
47, // 43: protowire.BlockWithTrustedDataMessage.daaWindow:type_name -> protowire.DaaBlock
|
|
49, // 44: protowire.BlockWithTrustedDataMessage.ghostdagData:type_name -> protowire.BlockGhostdagDataHashPair
|
|
10, // 45: protowire.DaaBlock.block:type_name -> protowire.BlockMessage
|
|
50, // 46: protowire.DaaBlock.ghostdagData:type_name -> protowire.GhostdagData
|
|
11, // 47: protowire.DaaBlockV4.header:type_name -> protowire.BlockHeader
|
|
50, // 48: protowire.DaaBlockV4.ghostdagData:type_name -> protowire.GhostdagData
|
|
13, // 49: protowire.BlockGhostdagDataHashPair.hash:type_name -> protowire.Hash
|
|
50, // 50: protowire.BlockGhostdagDataHashPair.ghostdagData:type_name -> protowire.GhostdagData
|
|
13, // 51: protowire.GhostdagData.selectedParent:type_name -> protowire.Hash
|
|
13, // 52: protowire.GhostdagData.mergeSetBlues:type_name -> protowire.Hash
|
|
13, // 53: protowire.GhostdagData.mergeSetReds:type_name -> protowire.Hash
|
|
51, // 54: protowire.GhostdagData.bluesAnticoneSizes:type_name -> protowire.BluesAnticoneSizes
|
|
13, // 55: protowire.BluesAnticoneSizes.blueHash:type_name -> protowire.Hash
|
|
11, // 56: protowire.PruningPointsMessage.headers:type_name -> protowire.BlockHeader
|
|
56, // 57: protowire.PruningPointProofMessage.headers:type_name -> protowire.PruningPointProofHeaderArray
|
|
11, // 58: protowire.PruningPointProofHeaderArray.headers:type_name -> protowire.BlockHeader
|
|
10, // 59: protowire.BlockWithTrustedDataV4Message.block:type_name -> protowire.BlockMessage
|
|
48, // 60: protowire.TrustedDataMessage.daaWindow:type_name -> protowire.DaaBlockV4
|
|
49, // 61: protowire.TrustedDataMessage.ghostdagData:type_name -> protowire.BlockGhostdagDataHashPair
|
|
62, // [62:62] is the sub-list for method output_type
|
|
62, // [62:62] is the sub-list for method input_type
|
|
62, // [62:62] is the sub-list for extension type_name
|
|
62, // [62:62] is the sub-list for extension extendee
|
|
0, // [0:62] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_p2p_proto_init() }
|
|
func file_p2p_proto_init() {
|
|
if File_p2p_proto != nil {
|
|
return
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_p2p_proto_rawDesc,
|
|
NumEnums: 0,
|
|
NumMessages: 60,
|
|
NumExtensions: 0,
|
|
NumServices: 0,
|
|
},
|
|
GoTypes: file_p2p_proto_goTypes,
|
|
DependencyIndexes: file_p2p_proto_depIdxs,
|
|
MessageInfos: file_p2p_proto_msgTypes,
|
|
}.Build()
|
|
File_p2p_proto = out.File
|
|
file_p2p_proto_rawDesc = nil
|
|
file_p2p_proto_goTypes = nil
|
|
file_p2p_proto_depIdxs = nil
|
|
}
|