mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
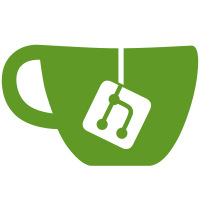
* Adding support for mass. * update grpc v1.69.2 * Upgrade protos using latest protoc * Use MassCommitment instead of Mass * Fix DomainTransaction clone, equal and tests --------- Co-authored-by: veronica <jimjouny@gmail.com> Co-authored-by: Ori Newman <orinewman1@gmail.com>
9246 lines
367 KiB
Go
9246 lines
367 KiB
Go
// RPC-related types. Request messages, response messages, and dependant types.
|
|
//
|
|
// Clients are expected to build RequestMessages and wrap them in KaspadMessage. (see messages.proto)
|
|
//
|
|
// Having received a RequestMessage, (wrapped in a KaspadMessage) the RPC server will respond with a
|
|
// ResponseMessage (likewise wrapped in a KaspadMessage) respective to the original RequestMessage.
|
|
//
|
|
// **IMPORTANT:** This API is a work in progress and is subject to break between versions.
|
|
//
|
|
|
|
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.36.2
|
|
// protoc v3.12.3
|
|
// source: rpc.proto
|
|
|
|
package protowire
|
|
|
|
import (
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
type SubmitBlockResponseMessage_RejectReason int32
|
|
|
|
const (
|
|
SubmitBlockResponseMessage_NONE SubmitBlockResponseMessage_RejectReason = 0
|
|
SubmitBlockResponseMessage_BLOCK_INVALID SubmitBlockResponseMessage_RejectReason = 1
|
|
SubmitBlockResponseMessage_IS_IN_IBD SubmitBlockResponseMessage_RejectReason = 2
|
|
)
|
|
|
|
// Enum value maps for SubmitBlockResponseMessage_RejectReason.
|
|
var (
|
|
SubmitBlockResponseMessage_RejectReason_name = map[int32]string{
|
|
0: "NONE",
|
|
1: "BLOCK_INVALID",
|
|
2: "IS_IN_IBD",
|
|
}
|
|
SubmitBlockResponseMessage_RejectReason_value = map[string]int32{
|
|
"NONE": 0,
|
|
"BLOCK_INVALID": 1,
|
|
"IS_IN_IBD": 2,
|
|
}
|
|
)
|
|
|
|
func (x SubmitBlockResponseMessage_RejectReason) Enum() *SubmitBlockResponseMessage_RejectReason {
|
|
p := new(SubmitBlockResponseMessage_RejectReason)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x SubmitBlockResponseMessage_RejectReason) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (SubmitBlockResponseMessage_RejectReason) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_rpc_proto_enumTypes[0].Descriptor()
|
|
}
|
|
|
|
func (SubmitBlockResponseMessage_RejectReason) Type() protoreflect.EnumType {
|
|
return &file_rpc_proto_enumTypes[0]
|
|
}
|
|
|
|
func (x SubmitBlockResponseMessage_RejectReason) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use SubmitBlockResponseMessage_RejectReason.Descriptor instead.
|
|
func (SubmitBlockResponseMessage_RejectReason) EnumDescriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{17, 0}
|
|
}
|
|
|
|
// RPCError represents a generic non-internal error.
|
|
//
|
|
// Receivers of any ResponseMessage are expected to check whether its error field is not null.
|
|
type RPCError struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Message string `protobuf:"bytes,1,opt,name=message,proto3" json:"message,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RPCError) Reset() {
|
|
*x = RPCError{}
|
|
mi := &file_rpc_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RPCError) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RPCError) ProtoMessage() {}
|
|
|
|
func (x *RPCError) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[0]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RPCError.ProtoReflect.Descriptor instead.
|
|
func (*RPCError) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *RPCError) GetMessage() string {
|
|
if x != nil {
|
|
return x.Message
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type RpcBlock struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Header *RpcBlockHeader `protobuf:"bytes,1,opt,name=header,proto3" json:"header,omitempty"`
|
|
Transactions []*RpcTransaction `protobuf:"bytes,2,rep,name=transactions,proto3" json:"transactions,omitempty"`
|
|
VerboseData *RpcBlockVerboseData `protobuf:"bytes,3,opt,name=verboseData,proto3" json:"verboseData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcBlock) Reset() {
|
|
*x = RpcBlock{}
|
|
mi := &file_rpc_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcBlock) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcBlock) ProtoMessage() {}
|
|
|
|
func (x *RpcBlock) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[1]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcBlock.ProtoReflect.Descriptor instead.
|
|
func (*RpcBlock) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *RpcBlock) GetHeader() *RpcBlockHeader {
|
|
if x != nil {
|
|
return x.Header
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcBlock) GetTransactions() []*RpcTransaction {
|
|
if x != nil {
|
|
return x.Transactions
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcBlock) GetVerboseData() *RpcBlockVerboseData {
|
|
if x != nil {
|
|
return x.VerboseData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RpcBlockHeader struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Version uint32 `protobuf:"varint,1,opt,name=version,proto3" json:"version,omitempty"`
|
|
Parents []*RpcBlockLevelParents `protobuf:"bytes,12,rep,name=parents,proto3" json:"parents,omitempty"`
|
|
HashMerkleRoot string `protobuf:"bytes,3,opt,name=hashMerkleRoot,proto3" json:"hashMerkleRoot,omitempty"`
|
|
AcceptedIdMerkleRoot string `protobuf:"bytes,4,opt,name=acceptedIdMerkleRoot,proto3" json:"acceptedIdMerkleRoot,omitempty"`
|
|
UtxoCommitment string `protobuf:"bytes,5,opt,name=utxoCommitment,proto3" json:"utxoCommitment,omitempty"`
|
|
Timestamp int64 `protobuf:"varint,6,opt,name=timestamp,proto3" json:"timestamp,omitempty"`
|
|
Bits uint32 `protobuf:"varint,7,opt,name=bits,proto3" json:"bits,omitempty"`
|
|
Nonce uint64 `protobuf:"varint,8,opt,name=nonce,proto3" json:"nonce,omitempty"`
|
|
DaaScore uint64 `protobuf:"varint,9,opt,name=daaScore,proto3" json:"daaScore,omitempty"`
|
|
BlueWork string `protobuf:"bytes,10,opt,name=blueWork,proto3" json:"blueWork,omitempty"`
|
|
PruningPoint string `protobuf:"bytes,14,opt,name=pruningPoint,proto3" json:"pruningPoint,omitempty"`
|
|
BlueScore uint64 `protobuf:"varint,13,opt,name=blueScore,proto3" json:"blueScore,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcBlockHeader) Reset() {
|
|
*x = RpcBlockHeader{}
|
|
mi := &file_rpc_proto_msgTypes[2]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcBlockHeader) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcBlockHeader) ProtoMessage() {}
|
|
|
|
func (x *RpcBlockHeader) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[2]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcBlockHeader.ProtoReflect.Descriptor instead.
|
|
func (*RpcBlockHeader) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetVersion() uint32 {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetParents() []*RpcBlockLevelParents {
|
|
if x != nil {
|
|
return x.Parents
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetHashMerkleRoot() string {
|
|
if x != nil {
|
|
return x.HashMerkleRoot
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetAcceptedIdMerkleRoot() string {
|
|
if x != nil {
|
|
return x.AcceptedIdMerkleRoot
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetUtxoCommitment() string {
|
|
if x != nil {
|
|
return x.UtxoCommitment
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetTimestamp() int64 {
|
|
if x != nil {
|
|
return x.Timestamp
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetBits() uint32 {
|
|
if x != nil {
|
|
return x.Bits
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetNonce() uint64 {
|
|
if x != nil {
|
|
return x.Nonce
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetDaaScore() uint64 {
|
|
if x != nil {
|
|
return x.DaaScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetBlueWork() string {
|
|
if x != nil {
|
|
return x.BlueWork
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetPruningPoint() string {
|
|
if x != nil {
|
|
return x.PruningPoint
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcBlockHeader) GetBlueScore() uint64 {
|
|
if x != nil {
|
|
return x.BlueScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type RpcBlockLevelParents struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
ParentHashes []string `protobuf:"bytes,1,rep,name=parentHashes,proto3" json:"parentHashes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcBlockLevelParents) Reset() {
|
|
*x = RpcBlockLevelParents{}
|
|
mi := &file_rpc_proto_msgTypes[3]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcBlockLevelParents) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcBlockLevelParents) ProtoMessage() {}
|
|
|
|
func (x *RpcBlockLevelParents) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[3]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcBlockLevelParents.ProtoReflect.Descriptor instead.
|
|
func (*RpcBlockLevelParents) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
func (x *RpcBlockLevelParents) GetParentHashes() []string {
|
|
if x != nil {
|
|
return x.ParentHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RpcBlockVerboseData struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Hash string `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
Difficulty float64 `protobuf:"fixed64,11,opt,name=difficulty,proto3" json:"difficulty,omitempty"`
|
|
SelectedParentHash string `protobuf:"bytes,13,opt,name=selectedParentHash,proto3" json:"selectedParentHash,omitempty"`
|
|
TransactionIds []string `protobuf:"bytes,14,rep,name=transactionIds,proto3" json:"transactionIds,omitempty"`
|
|
IsHeaderOnly bool `protobuf:"varint,15,opt,name=isHeaderOnly,proto3" json:"isHeaderOnly,omitempty"`
|
|
BlueScore uint64 `protobuf:"varint,16,opt,name=blueScore,proto3" json:"blueScore,omitempty"`
|
|
ChildrenHashes []string `protobuf:"bytes,17,rep,name=childrenHashes,proto3" json:"childrenHashes,omitempty"`
|
|
MergeSetBluesHashes []string `protobuf:"bytes,18,rep,name=mergeSetBluesHashes,proto3" json:"mergeSetBluesHashes,omitempty"`
|
|
MergeSetRedsHashes []string `protobuf:"bytes,19,rep,name=mergeSetRedsHashes,proto3" json:"mergeSetRedsHashes,omitempty"`
|
|
IsChainBlock bool `protobuf:"varint,20,opt,name=isChainBlock,proto3" json:"isChainBlock,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) Reset() {
|
|
*x = RpcBlockVerboseData{}
|
|
mi := &file_rpc_proto_msgTypes[4]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcBlockVerboseData) ProtoMessage() {}
|
|
|
|
func (x *RpcBlockVerboseData) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[4]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcBlockVerboseData.ProtoReflect.Descriptor instead.
|
|
func (*RpcBlockVerboseData) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetHash() string {
|
|
if x != nil {
|
|
return x.Hash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetDifficulty() float64 {
|
|
if x != nil {
|
|
return x.Difficulty
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetSelectedParentHash() string {
|
|
if x != nil {
|
|
return x.SelectedParentHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetTransactionIds() []string {
|
|
if x != nil {
|
|
return x.TransactionIds
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetIsHeaderOnly() bool {
|
|
if x != nil {
|
|
return x.IsHeaderOnly
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetBlueScore() uint64 {
|
|
if x != nil {
|
|
return x.BlueScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetChildrenHashes() []string {
|
|
if x != nil {
|
|
return x.ChildrenHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetMergeSetBluesHashes() []string {
|
|
if x != nil {
|
|
return x.MergeSetBluesHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetMergeSetRedsHashes() []string {
|
|
if x != nil {
|
|
return x.MergeSetRedsHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcBlockVerboseData) GetIsChainBlock() bool {
|
|
if x != nil {
|
|
return x.IsChainBlock
|
|
}
|
|
return false
|
|
}
|
|
|
|
type RpcTransaction struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Version uint32 `protobuf:"varint,1,opt,name=version,proto3" json:"version,omitempty"`
|
|
Inputs []*RpcTransactionInput `protobuf:"bytes,2,rep,name=inputs,proto3" json:"inputs,omitempty"`
|
|
Outputs []*RpcTransactionOutput `protobuf:"bytes,3,rep,name=outputs,proto3" json:"outputs,omitempty"`
|
|
LockTime uint64 `protobuf:"varint,4,opt,name=lockTime,proto3" json:"lockTime,omitempty"`
|
|
SubnetworkId string `protobuf:"bytes,5,opt,name=subnetworkId,proto3" json:"subnetworkId,omitempty"`
|
|
Gas uint64 `protobuf:"varint,6,opt,name=gas,proto3" json:"gas,omitempty"`
|
|
Payload string `protobuf:"bytes,8,opt,name=payload,proto3" json:"payload,omitempty"`
|
|
VerboseData *RpcTransactionVerboseData `protobuf:"bytes,9,opt,name=verboseData,proto3" json:"verboseData,omitempty"`
|
|
Mass uint64 `protobuf:"varint,10,opt,name=mass,proto3" json:"mass,omitempty"` // <<< BPS10 - Add mass to RpcTransaction
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcTransaction) Reset() {
|
|
*x = RpcTransaction{}
|
|
mi := &file_rpc_proto_msgTypes[5]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcTransaction) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcTransaction) ProtoMessage() {}
|
|
|
|
func (x *RpcTransaction) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[5]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcTransaction.ProtoReflect.Descriptor instead.
|
|
func (*RpcTransaction) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{5}
|
|
}
|
|
|
|
func (x *RpcTransaction) GetVersion() uint32 {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcTransaction) GetInputs() []*RpcTransactionInput {
|
|
if x != nil {
|
|
return x.Inputs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcTransaction) GetOutputs() []*RpcTransactionOutput {
|
|
if x != nil {
|
|
return x.Outputs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcTransaction) GetLockTime() uint64 {
|
|
if x != nil {
|
|
return x.LockTime
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcTransaction) GetSubnetworkId() string {
|
|
if x != nil {
|
|
return x.SubnetworkId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcTransaction) GetGas() uint64 {
|
|
if x != nil {
|
|
return x.Gas
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcTransaction) GetPayload() string {
|
|
if x != nil {
|
|
return x.Payload
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcTransaction) GetVerboseData() *RpcTransactionVerboseData {
|
|
if x != nil {
|
|
return x.VerboseData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcTransaction) GetMass() uint64 {
|
|
if x != nil {
|
|
return x.Mass
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type RpcTransactionInput struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
PreviousOutpoint *RpcOutpoint `protobuf:"bytes,1,opt,name=previousOutpoint,proto3" json:"previousOutpoint,omitempty"`
|
|
SignatureScript string `protobuf:"bytes,2,opt,name=signatureScript,proto3" json:"signatureScript,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,3,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
SigOpCount uint32 `protobuf:"varint,5,opt,name=sigOpCount,proto3" json:"sigOpCount,omitempty"`
|
|
VerboseData *RpcTransactionInputVerboseData `protobuf:"bytes,4,opt,name=verboseData,proto3" json:"verboseData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcTransactionInput) Reset() {
|
|
*x = RpcTransactionInput{}
|
|
mi := &file_rpc_proto_msgTypes[6]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcTransactionInput) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcTransactionInput) ProtoMessage() {}
|
|
|
|
func (x *RpcTransactionInput) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[6]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcTransactionInput.ProtoReflect.Descriptor instead.
|
|
func (*RpcTransactionInput) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{6}
|
|
}
|
|
|
|
func (x *RpcTransactionInput) GetPreviousOutpoint() *RpcOutpoint {
|
|
if x != nil {
|
|
return x.PreviousOutpoint
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcTransactionInput) GetSignatureScript() string {
|
|
if x != nil {
|
|
return x.SignatureScript
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcTransactionInput) GetSequence() uint64 {
|
|
if x != nil {
|
|
return x.Sequence
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcTransactionInput) GetSigOpCount() uint32 {
|
|
if x != nil {
|
|
return x.SigOpCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcTransactionInput) GetVerboseData() *RpcTransactionInputVerboseData {
|
|
if x != nil {
|
|
return x.VerboseData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RpcScriptPublicKey struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Version uint32 `protobuf:"varint,1,opt,name=version,proto3" json:"version,omitempty"`
|
|
ScriptPublicKey string `protobuf:"bytes,2,opt,name=scriptPublicKey,proto3" json:"scriptPublicKey,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcScriptPublicKey) Reset() {
|
|
*x = RpcScriptPublicKey{}
|
|
mi := &file_rpc_proto_msgTypes[7]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcScriptPublicKey) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcScriptPublicKey) ProtoMessage() {}
|
|
|
|
func (x *RpcScriptPublicKey) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[7]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcScriptPublicKey.ProtoReflect.Descriptor instead.
|
|
func (*RpcScriptPublicKey) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{7}
|
|
}
|
|
|
|
func (x *RpcScriptPublicKey) GetVersion() uint32 {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcScriptPublicKey) GetScriptPublicKey() string {
|
|
if x != nil {
|
|
return x.ScriptPublicKey
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type RpcTransactionOutput struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Amount uint64 `protobuf:"varint,1,opt,name=amount,proto3" json:"amount,omitempty"`
|
|
ScriptPublicKey *RpcScriptPublicKey `protobuf:"bytes,2,opt,name=scriptPublicKey,proto3" json:"scriptPublicKey,omitempty"`
|
|
VerboseData *RpcTransactionOutputVerboseData `protobuf:"bytes,3,opt,name=verboseData,proto3" json:"verboseData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcTransactionOutput) Reset() {
|
|
*x = RpcTransactionOutput{}
|
|
mi := &file_rpc_proto_msgTypes[8]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcTransactionOutput) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcTransactionOutput) ProtoMessage() {}
|
|
|
|
func (x *RpcTransactionOutput) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[8]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcTransactionOutput.ProtoReflect.Descriptor instead.
|
|
func (*RpcTransactionOutput) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{8}
|
|
}
|
|
|
|
func (x *RpcTransactionOutput) GetAmount() uint64 {
|
|
if x != nil {
|
|
return x.Amount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcTransactionOutput) GetScriptPublicKey() *RpcScriptPublicKey {
|
|
if x != nil {
|
|
return x.ScriptPublicKey
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcTransactionOutput) GetVerboseData() *RpcTransactionOutputVerboseData {
|
|
if x != nil {
|
|
return x.VerboseData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RpcOutpoint struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
TransactionId string `protobuf:"bytes,1,opt,name=transactionId,proto3" json:"transactionId,omitempty"`
|
|
Index uint32 `protobuf:"varint,2,opt,name=index,proto3" json:"index,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcOutpoint) Reset() {
|
|
*x = RpcOutpoint{}
|
|
mi := &file_rpc_proto_msgTypes[9]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcOutpoint) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcOutpoint) ProtoMessage() {}
|
|
|
|
func (x *RpcOutpoint) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[9]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcOutpoint.ProtoReflect.Descriptor instead.
|
|
func (*RpcOutpoint) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{9}
|
|
}
|
|
|
|
func (x *RpcOutpoint) GetTransactionId() string {
|
|
if x != nil {
|
|
return x.TransactionId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcOutpoint) GetIndex() uint32 {
|
|
if x != nil {
|
|
return x.Index
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type RpcUtxoEntry struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Amount uint64 `protobuf:"varint,1,opt,name=amount,proto3" json:"amount,omitempty"`
|
|
ScriptPublicKey *RpcScriptPublicKey `protobuf:"bytes,2,opt,name=scriptPublicKey,proto3" json:"scriptPublicKey,omitempty"`
|
|
BlockDaaScore uint64 `protobuf:"varint,3,opt,name=blockDaaScore,proto3" json:"blockDaaScore,omitempty"`
|
|
IsCoinbase bool `protobuf:"varint,4,opt,name=isCoinbase,proto3" json:"isCoinbase,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcUtxoEntry) Reset() {
|
|
*x = RpcUtxoEntry{}
|
|
mi := &file_rpc_proto_msgTypes[10]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcUtxoEntry) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcUtxoEntry) ProtoMessage() {}
|
|
|
|
func (x *RpcUtxoEntry) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[10]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcUtxoEntry.ProtoReflect.Descriptor instead.
|
|
func (*RpcUtxoEntry) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{10}
|
|
}
|
|
|
|
func (x *RpcUtxoEntry) GetAmount() uint64 {
|
|
if x != nil {
|
|
return x.Amount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcUtxoEntry) GetScriptPublicKey() *RpcScriptPublicKey {
|
|
if x != nil {
|
|
return x.ScriptPublicKey
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcUtxoEntry) GetBlockDaaScore() uint64 {
|
|
if x != nil {
|
|
return x.BlockDaaScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcUtxoEntry) GetIsCoinbase() bool {
|
|
if x != nil {
|
|
return x.IsCoinbase
|
|
}
|
|
return false
|
|
}
|
|
|
|
type RpcTransactionVerboseData struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
TransactionId string `protobuf:"bytes,1,opt,name=transactionId,proto3" json:"transactionId,omitempty"`
|
|
Hash string `protobuf:"bytes,2,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
Mass uint64 `protobuf:"varint,4,opt,name=mass,proto3" json:"mass,omitempty"`
|
|
BlockHash string `protobuf:"bytes,12,opt,name=blockHash,proto3" json:"blockHash,omitempty"`
|
|
BlockTime uint64 `protobuf:"varint,14,opt,name=blockTime,proto3" json:"blockTime,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcTransactionVerboseData) Reset() {
|
|
*x = RpcTransactionVerboseData{}
|
|
mi := &file_rpc_proto_msgTypes[11]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcTransactionVerboseData) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcTransactionVerboseData) ProtoMessage() {}
|
|
|
|
func (x *RpcTransactionVerboseData) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[11]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcTransactionVerboseData.ProtoReflect.Descriptor instead.
|
|
func (*RpcTransactionVerboseData) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{11}
|
|
}
|
|
|
|
func (x *RpcTransactionVerboseData) GetTransactionId() string {
|
|
if x != nil {
|
|
return x.TransactionId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcTransactionVerboseData) GetHash() string {
|
|
if x != nil {
|
|
return x.Hash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcTransactionVerboseData) GetMass() uint64 {
|
|
if x != nil {
|
|
return x.Mass
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcTransactionVerboseData) GetBlockHash() string {
|
|
if x != nil {
|
|
return x.BlockHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcTransactionVerboseData) GetBlockTime() uint64 {
|
|
if x != nil {
|
|
return x.BlockTime
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type RpcTransactionInputVerboseData struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcTransactionInputVerboseData) Reset() {
|
|
*x = RpcTransactionInputVerboseData{}
|
|
mi := &file_rpc_proto_msgTypes[12]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcTransactionInputVerboseData) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcTransactionInputVerboseData) ProtoMessage() {}
|
|
|
|
func (x *RpcTransactionInputVerboseData) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[12]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcTransactionInputVerboseData.ProtoReflect.Descriptor instead.
|
|
func (*RpcTransactionInputVerboseData) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{12}
|
|
}
|
|
|
|
type RpcTransactionOutputVerboseData struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
ScriptPublicKeyType string `protobuf:"bytes,5,opt,name=scriptPublicKeyType,proto3" json:"scriptPublicKeyType,omitempty"`
|
|
ScriptPublicKeyAddress string `protobuf:"bytes,6,opt,name=scriptPublicKeyAddress,proto3" json:"scriptPublicKeyAddress,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcTransactionOutputVerboseData) Reset() {
|
|
*x = RpcTransactionOutputVerboseData{}
|
|
mi := &file_rpc_proto_msgTypes[13]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcTransactionOutputVerboseData) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcTransactionOutputVerboseData) ProtoMessage() {}
|
|
|
|
func (x *RpcTransactionOutputVerboseData) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[13]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcTransactionOutputVerboseData.ProtoReflect.Descriptor instead.
|
|
func (*RpcTransactionOutputVerboseData) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{13}
|
|
}
|
|
|
|
func (x *RpcTransactionOutputVerboseData) GetScriptPublicKeyType() string {
|
|
if x != nil {
|
|
return x.ScriptPublicKeyType
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *RpcTransactionOutputVerboseData) GetScriptPublicKeyAddress() string {
|
|
if x != nil {
|
|
return x.ScriptPublicKeyAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// GetCurrentNetworkRequestMessage requests the network kaspad is currently running against.
|
|
//
|
|
// Possible networks are: Mainnet, Testnet, Simnet, Devnet
|
|
type GetCurrentNetworkRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetCurrentNetworkRequestMessage) Reset() {
|
|
*x = GetCurrentNetworkRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[14]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetCurrentNetworkRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetCurrentNetworkRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetCurrentNetworkRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[14]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetCurrentNetworkRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetCurrentNetworkRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{14}
|
|
}
|
|
|
|
type GetCurrentNetworkResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
CurrentNetwork string `protobuf:"bytes,1,opt,name=currentNetwork,proto3" json:"currentNetwork,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetCurrentNetworkResponseMessage) Reset() {
|
|
*x = GetCurrentNetworkResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[15]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetCurrentNetworkResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetCurrentNetworkResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetCurrentNetworkResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[15]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetCurrentNetworkResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetCurrentNetworkResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{15}
|
|
}
|
|
|
|
func (x *GetCurrentNetworkResponseMessage) GetCurrentNetwork() string {
|
|
if x != nil {
|
|
return x.CurrentNetwork
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetCurrentNetworkResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// SubmitBlockRequestMessage requests to submit a block into the DAG.
|
|
// Blocks are generally expected to have been generated using the getBlockTemplate call.
|
|
//
|
|
// See: GetBlockTemplateRequestMessage
|
|
type SubmitBlockRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Block *RpcBlock `protobuf:"bytes,2,opt,name=block,proto3" json:"block,omitempty"`
|
|
AllowNonDAABlocks bool `protobuf:"varint,3,opt,name=allowNonDAABlocks,proto3" json:"allowNonDAABlocks,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *SubmitBlockRequestMessage) Reset() {
|
|
*x = SubmitBlockRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[16]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *SubmitBlockRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubmitBlockRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *SubmitBlockRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[16]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubmitBlockRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*SubmitBlockRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{16}
|
|
}
|
|
|
|
func (x *SubmitBlockRequestMessage) GetBlock() *RpcBlock {
|
|
if x != nil {
|
|
return x.Block
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SubmitBlockRequestMessage) GetAllowNonDAABlocks() bool {
|
|
if x != nil {
|
|
return x.AllowNonDAABlocks
|
|
}
|
|
return false
|
|
}
|
|
|
|
type SubmitBlockResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
RejectReason SubmitBlockResponseMessage_RejectReason `protobuf:"varint,1,opt,name=rejectReason,proto3,enum=protowire.SubmitBlockResponseMessage_RejectReason" json:"rejectReason,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *SubmitBlockResponseMessage) Reset() {
|
|
*x = SubmitBlockResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[17]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *SubmitBlockResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubmitBlockResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *SubmitBlockResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[17]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubmitBlockResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*SubmitBlockResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{17}
|
|
}
|
|
|
|
func (x *SubmitBlockResponseMessage) GetRejectReason() SubmitBlockResponseMessage_RejectReason {
|
|
if x != nil {
|
|
return x.RejectReason
|
|
}
|
|
return SubmitBlockResponseMessage_NONE
|
|
}
|
|
|
|
func (x *SubmitBlockResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetBlockTemplateRequestMessage requests a current block template.
|
|
// Callers are expected to solve the block template and submit it using the submitBlock call
|
|
//
|
|
// See: SubmitBlockRequestMessage
|
|
type GetBlockTemplateRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
// Which kaspa address should the coinbase block reward transaction pay into
|
|
PayAddress string `protobuf:"bytes,1,opt,name=payAddress,proto3" json:"payAddress,omitempty"`
|
|
ExtraData string `protobuf:"bytes,2,opt,name=extraData,proto3" json:"extraData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlockTemplateRequestMessage) Reset() {
|
|
*x = GetBlockTemplateRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[18]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlockTemplateRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlockTemplateRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlockTemplateRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[18]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlockTemplateRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlockTemplateRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{18}
|
|
}
|
|
|
|
func (x *GetBlockTemplateRequestMessage) GetPayAddress() string {
|
|
if x != nil {
|
|
return x.PayAddress
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetBlockTemplateRequestMessage) GetExtraData() string {
|
|
if x != nil {
|
|
return x.ExtraData
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type GetBlockTemplateResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Block *RpcBlock `protobuf:"bytes,3,opt,name=block,proto3" json:"block,omitempty"`
|
|
// Whether kaspad thinks that it's synced.
|
|
// Callers are discouraged (but not forbidden) from solving blocks when kaspad is not synced.
|
|
// That is because when kaspad isn't in sync with the rest of the network there's a high
|
|
// chance the block will never be accepted, thus the solving effort would have been wasted.
|
|
IsSynced bool `protobuf:"varint,2,opt,name=isSynced,proto3" json:"isSynced,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlockTemplateResponseMessage) Reset() {
|
|
*x = GetBlockTemplateResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[19]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlockTemplateResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlockTemplateResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlockTemplateResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[19]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlockTemplateResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlockTemplateResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{19}
|
|
}
|
|
|
|
func (x *GetBlockTemplateResponseMessage) GetBlock() *RpcBlock {
|
|
if x != nil {
|
|
return x.Block
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetBlockTemplateResponseMessage) GetIsSynced() bool {
|
|
if x != nil {
|
|
return x.IsSynced
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetBlockTemplateResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// NotifyBlockAddedRequestMessage registers this connection for blockAdded notifications.
|
|
//
|
|
// See: BlockAddedNotificationMessage
|
|
type NotifyBlockAddedRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyBlockAddedRequestMessage) Reset() {
|
|
*x = NotifyBlockAddedRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[20]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyBlockAddedRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyBlockAddedRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyBlockAddedRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[20]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyBlockAddedRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyBlockAddedRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{20}
|
|
}
|
|
|
|
type NotifyBlockAddedResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyBlockAddedResponseMessage) Reset() {
|
|
*x = NotifyBlockAddedResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[21]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyBlockAddedResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyBlockAddedResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyBlockAddedResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[21]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyBlockAddedResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyBlockAddedResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{21}
|
|
}
|
|
|
|
func (x *NotifyBlockAddedResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// BlockAddedNotificationMessage is sent whenever a blocks has been added (NOT accepted)
|
|
// into the DAG.
|
|
//
|
|
// See: NotifyBlockAddedRequestMessage
|
|
type BlockAddedNotificationMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Block *RpcBlock `protobuf:"bytes,3,opt,name=block,proto3" json:"block,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BlockAddedNotificationMessage) Reset() {
|
|
*x = BlockAddedNotificationMessage{}
|
|
mi := &file_rpc_proto_msgTypes[22]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BlockAddedNotificationMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockAddedNotificationMessage) ProtoMessage() {}
|
|
|
|
func (x *BlockAddedNotificationMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[22]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockAddedNotificationMessage.ProtoReflect.Descriptor instead.
|
|
func (*BlockAddedNotificationMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{22}
|
|
}
|
|
|
|
func (x *BlockAddedNotificationMessage) GetBlock() *RpcBlock {
|
|
if x != nil {
|
|
return x.Block
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetPeerAddressesRequestMessage requests the list of known kaspad addresses in the
|
|
// current network. (mainnet, testnet, etc.)
|
|
type GetPeerAddressesRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetPeerAddressesRequestMessage) Reset() {
|
|
*x = GetPeerAddressesRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[23]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetPeerAddressesRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetPeerAddressesRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetPeerAddressesRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[23]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetPeerAddressesRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetPeerAddressesRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{23}
|
|
}
|
|
|
|
type GetPeerAddressesResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Addresses []*GetPeerAddressesKnownAddressMessage `protobuf:"bytes,1,rep,name=addresses,proto3" json:"addresses,omitempty"`
|
|
BannedAddresses []*GetPeerAddressesKnownAddressMessage `protobuf:"bytes,2,rep,name=bannedAddresses,proto3" json:"bannedAddresses,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetPeerAddressesResponseMessage) Reset() {
|
|
*x = GetPeerAddressesResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[24]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetPeerAddressesResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetPeerAddressesResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetPeerAddressesResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[24]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetPeerAddressesResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetPeerAddressesResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{24}
|
|
}
|
|
|
|
func (x *GetPeerAddressesResponseMessage) GetAddresses() []*GetPeerAddressesKnownAddressMessage {
|
|
if x != nil {
|
|
return x.Addresses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetPeerAddressesResponseMessage) GetBannedAddresses() []*GetPeerAddressesKnownAddressMessage {
|
|
if x != nil {
|
|
return x.BannedAddresses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetPeerAddressesResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetPeerAddressesKnownAddressMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Addr string `protobuf:"bytes,1,opt,name=Addr,proto3" json:"Addr,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetPeerAddressesKnownAddressMessage) Reset() {
|
|
*x = GetPeerAddressesKnownAddressMessage{}
|
|
mi := &file_rpc_proto_msgTypes[25]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetPeerAddressesKnownAddressMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetPeerAddressesKnownAddressMessage) ProtoMessage() {}
|
|
|
|
func (x *GetPeerAddressesKnownAddressMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[25]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetPeerAddressesKnownAddressMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetPeerAddressesKnownAddressMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{25}
|
|
}
|
|
|
|
func (x *GetPeerAddressesKnownAddressMessage) GetAddr() string {
|
|
if x != nil {
|
|
return x.Addr
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// GetSelectedTipHashRequestMessage requests the hash of the current virtual's
|
|
// selected parent.
|
|
type GetSelectedTipHashRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetSelectedTipHashRequestMessage) Reset() {
|
|
*x = GetSelectedTipHashRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[26]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetSelectedTipHashRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetSelectedTipHashRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetSelectedTipHashRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[26]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetSelectedTipHashRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetSelectedTipHashRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{26}
|
|
}
|
|
|
|
type GetSelectedTipHashResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
SelectedTipHash string `protobuf:"bytes,1,opt,name=selectedTipHash,proto3" json:"selectedTipHash,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetSelectedTipHashResponseMessage) Reset() {
|
|
*x = GetSelectedTipHashResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[27]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetSelectedTipHashResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetSelectedTipHashResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetSelectedTipHashResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[27]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetSelectedTipHashResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetSelectedTipHashResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{27}
|
|
}
|
|
|
|
func (x *GetSelectedTipHashResponseMessage) GetSelectedTipHash() string {
|
|
if x != nil {
|
|
return x.SelectedTipHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetSelectedTipHashResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetMempoolEntryRequestMessage requests information about a specific transaction
|
|
// in the mempool.
|
|
type GetMempoolEntryRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
// The transaction's TransactionID.
|
|
TxId string `protobuf:"bytes,1,opt,name=txId,proto3" json:"txId,omitempty"`
|
|
IncludeOrphanPool bool `protobuf:"varint,2,opt,name=includeOrphanPool,proto3" json:"includeOrphanPool,omitempty"`
|
|
FilterTransactionPool bool `protobuf:"varint,3,opt,name=filterTransactionPool,proto3" json:"filterTransactionPool,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetMempoolEntryRequestMessage) Reset() {
|
|
*x = GetMempoolEntryRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[28]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetMempoolEntryRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetMempoolEntryRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetMempoolEntryRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[28]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetMempoolEntryRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetMempoolEntryRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{28}
|
|
}
|
|
|
|
func (x *GetMempoolEntryRequestMessage) GetTxId() string {
|
|
if x != nil {
|
|
return x.TxId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetMempoolEntryRequestMessage) GetIncludeOrphanPool() bool {
|
|
if x != nil {
|
|
return x.IncludeOrphanPool
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetMempoolEntryRequestMessage) GetFilterTransactionPool() bool {
|
|
if x != nil {
|
|
return x.FilterTransactionPool
|
|
}
|
|
return false
|
|
}
|
|
|
|
type GetMempoolEntryResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Entry *MempoolEntry `protobuf:"bytes,1,opt,name=entry,proto3" json:"entry,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetMempoolEntryResponseMessage) Reset() {
|
|
*x = GetMempoolEntryResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[29]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetMempoolEntryResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetMempoolEntryResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetMempoolEntryResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[29]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetMempoolEntryResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetMempoolEntryResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{29}
|
|
}
|
|
|
|
func (x *GetMempoolEntryResponseMessage) GetEntry() *MempoolEntry {
|
|
if x != nil {
|
|
return x.Entry
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetMempoolEntryResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetMempoolEntriesRequestMessage requests information about all the transactions
|
|
// currently in the mempool.
|
|
type GetMempoolEntriesRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
IncludeOrphanPool bool `protobuf:"varint,1,opt,name=includeOrphanPool,proto3" json:"includeOrphanPool,omitempty"`
|
|
FilterTransactionPool bool `protobuf:"varint,2,opt,name=filterTransactionPool,proto3" json:"filterTransactionPool,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetMempoolEntriesRequestMessage) Reset() {
|
|
*x = GetMempoolEntriesRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[30]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetMempoolEntriesRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetMempoolEntriesRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetMempoolEntriesRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[30]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetMempoolEntriesRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetMempoolEntriesRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{30}
|
|
}
|
|
|
|
func (x *GetMempoolEntriesRequestMessage) GetIncludeOrphanPool() bool {
|
|
if x != nil {
|
|
return x.IncludeOrphanPool
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetMempoolEntriesRequestMessage) GetFilterTransactionPool() bool {
|
|
if x != nil {
|
|
return x.FilterTransactionPool
|
|
}
|
|
return false
|
|
}
|
|
|
|
type GetMempoolEntriesResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Entries []*MempoolEntry `protobuf:"bytes,1,rep,name=entries,proto3" json:"entries,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetMempoolEntriesResponseMessage) Reset() {
|
|
*x = GetMempoolEntriesResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[31]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetMempoolEntriesResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetMempoolEntriesResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetMempoolEntriesResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[31]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetMempoolEntriesResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetMempoolEntriesResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{31}
|
|
}
|
|
|
|
func (x *GetMempoolEntriesResponseMessage) GetEntries() []*MempoolEntry {
|
|
if x != nil {
|
|
return x.Entries
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetMempoolEntriesResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type MempoolEntry struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Fee uint64 `protobuf:"varint,1,opt,name=fee,proto3" json:"fee,omitempty"`
|
|
Transaction *RpcTransaction `protobuf:"bytes,3,opt,name=transaction,proto3" json:"transaction,omitempty"`
|
|
IsOrphan bool `protobuf:"varint,4,opt,name=isOrphan,proto3" json:"isOrphan,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *MempoolEntry) Reset() {
|
|
*x = MempoolEntry{}
|
|
mi := &file_rpc_proto_msgTypes[32]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *MempoolEntry) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MempoolEntry) ProtoMessage() {}
|
|
|
|
func (x *MempoolEntry) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[32]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MempoolEntry.ProtoReflect.Descriptor instead.
|
|
func (*MempoolEntry) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{32}
|
|
}
|
|
|
|
func (x *MempoolEntry) GetFee() uint64 {
|
|
if x != nil {
|
|
return x.Fee
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *MempoolEntry) GetTransaction() *RpcTransaction {
|
|
if x != nil {
|
|
return x.Transaction
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *MempoolEntry) GetIsOrphan() bool {
|
|
if x != nil {
|
|
return x.IsOrphan
|
|
}
|
|
return false
|
|
}
|
|
|
|
// GetConnectedPeerInfoRequestMessage requests information about all the p2p peers
|
|
// currently connected to this kaspad.
|
|
type GetConnectedPeerInfoRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoRequestMessage) Reset() {
|
|
*x = GetConnectedPeerInfoRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[33]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetConnectedPeerInfoRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetConnectedPeerInfoRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[33]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetConnectedPeerInfoRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetConnectedPeerInfoRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{33}
|
|
}
|
|
|
|
type GetConnectedPeerInfoResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Infos []*GetConnectedPeerInfoMessage `protobuf:"bytes,1,rep,name=infos,proto3" json:"infos,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoResponseMessage) Reset() {
|
|
*x = GetConnectedPeerInfoResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[34]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetConnectedPeerInfoResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetConnectedPeerInfoResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[34]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetConnectedPeerInfoResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetConnectedPeerInfoResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{34}
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoResponseMessage) GetInfos() []*GetConnectedPeerInfoMessage {
|
|
if x != nil {
|
|
return x.Infos
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetConnectedPeerInfoMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Id string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Address string `protobuf:"bytes,2,opt,name=address,proto3" json:"address,omitempty"`
|
|
// How long did the last ping/pong exchange take
|
|
LastPingDuration int64 `protobuf:"varint,3,opt,name=lastPingDuration,proto3" json:"lastPingDuration,omitempty"`
|
|
// Whether this kaspad initiated the connection
|
|
IsOutbound bool `protobuf:"varint,6,opt,name=isOutbound,proto3" json:"isOutbound,omitempty"`
|
|
TimeOffset int64 `protobuf:"varint,7,opt,name=timeOffset,proto3" json:"timeOffset,omitempty"`
|
|
UserAgent string `protobuf:"bytes,8,opt,name=userAgent,proto3" json:"userAgent,omitempty"`
|
|
// The protocol version that this peer claims to support
|
|
AdvertisedProtocolVersion uint32 `protobuf:"varint,9,opt,name=advertisedProtocolVersion,proto3" json:"advertisedProtocolVersion,omitempty"`
|
|
// The timestamp of when this peer connected to this kaspad
|
|
TimeConnected int64 `protobuf:"varint,10,opt,name=timeConnected,proto3" json:"timeConnected,omitempty"`
|
|
// Whether this peer is the IBD peer (if IBD is running)
|
|
IsIbdPeer bool `protobuf:"varint,11,opt,name=isIbdPeer,proto3" json:"isIbdPeer,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) Reset() {
|
|
*x = GetConnectedPeerInfoMessage{}
|
|
mi := &file_rpc_proto_msgTypes[35]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetConnectedPeerInfoMessage) ProtoMessage() {}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[35]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetConnectedPeerInfoMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetConnectedPeerInfoMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{35}
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) GetId() string {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) GetLastPingDuration() int64 {
|
|
if x != nil {
|
|
return x.LastPingDuration
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) GetIsOutbound() bool {
|
|
if x != nil {
|
|
return x.IsOutbound
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) GetTimeOffset() int64 {
|
|
if x != nil {
|
|
return x.TimeOffset
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) GetUserAgent() string {
|
|
if x != nil {
|
|
return x.UserAgent
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) GetAdvertisedProtocolVersion() uint32 {
|
|
if x != nil {
|
|
return x.AdvertisedProtocolVersion
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) GetTimeConnected() int64 {
|
|
if x != nil {
|
|
return x.TimeConnected
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetConnectedPeerInfoMessage) GetIsIbdPeer() bool {
|
|
if x != nil {
|
|
return x.IsIbdPeer
|
|
}
|
|
return false
|
|
}
|
|
|
|
// AddPeerRequestMessage adds a peer to kaspad's outgoing connection list.
|
|
// This will, in most cases, result in kaspad connecting to said peer.
|
|
type AddPeerRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Address string `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
// Whether to keep attempting to connect to this peer after disconnection
|
|
IsPermanent bool `protobuf:"varint,2,opt,name=isPermanent,proto3" json:"isPermanent,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *AddPeerRequestMessage) Reset() {
|
|
*x = AddPeerRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[36]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *AddPeerRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AddPeerRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *AddPeerRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[36]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AddPeerRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*AddPeerRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{36}
|
|
}
|
|
|
|
func (x *AddPeerRequestMessage) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *AddPeerRequestMessage) GetIsPermanent() bool {
|
|
if x != nil {
|
|
return x.IsPermanent
|
|
}
|
|
return false
|
|
}
|
|
|
|
type AddPeerResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *AddPeerResponseMessage) Reset() {
|
|
*x = AddPeerResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[37]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *AddPeerResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AddPeerResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *AddPeerResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[37]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AddPeerResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*AddPeerResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{37}
|
|
}
|
|
|
|
func (x *AddPeerResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// SubmitTransactionRequestMessage submits a transaction to the mempool
|
|
type SubmitTransactionRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Transaction *RpcTransaction `protobuf:"bytes,1,opt,name=transaction,proto3" json:"transaction,omitempty"`
|
|
AllowOrphan bool `protobuf:"varint,2,opt,name=allowOrphan,proto3" json:"allowOrphan,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *SubmitTransactionRequestMessage) Reset() {
|
|
*x = SubmitTransactionRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[38]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *SubmitTransactionRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubmitTransactionRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *SubmitTransactionRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[38]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubmitTransactionRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*SubmitTransactionRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{38}
|
|
}
|
|
|
|
func (x *SubmitTransactionRequestMessage) GetTransaction() *RpcTransaction {
|
|
if x != nil {
|
|
return x.Transaction
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SubmitTransactionRequestMessage) GetAllowOrphan() bool {
|
|
if x != nil {
|
|
return x.AllowOrphan
|
|
}
|
|
return false
|
|
}
|
|
|
|
type SubmitTransactionResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
// The transaction ID of the submitted transaction
|
|
TransactionId string `protobuf:"bytes,1,opt,name=transactionId,proto3" json:"transactionId,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *SubmitTransactionResponseMessage) Reset() {
|
|
*x = SubmitTransactionResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[39]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *SubmitTransactionResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubmitTransactionResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *SubmitTransactionResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[39]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubmitTransactionResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*SubmitTransactionResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{39}
|
|
}
|
|
|
|
func (x *SubmitTransactionResponseMessage) GetTransactionId() string {
|
|
if x != nil {
|
|
return x.TransactionId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *SubmitTransactionResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// NotifyVirtualSelectedParentChainChangedRequestMessage registers this connection for virtualSelectedParentChainChanged notifications.
|
|
//
|
|
// See: VirtualSelectedParentChainChangedNotificationMessage
|
|
type NotifyVirtualSelectedParentChainChangedRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
IncludeAcceptedTransactionIds bool `protobuf:"varint,1,opt,name=includeAcceptedTransactionIds,proto3" json:"includeAcceptedTransactionIds,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentChainChangedRequestMessage) Reset() {
|
|
*x = NotifyVirtualSelectedParentChainChangedRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[40]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentChainChangedRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyVirtualSelectedParentChainChangedRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyVirtualSelectedParentChainChangedRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[40]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyVirtualSelectedParentChainChangedRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyVirtualSelectedParentChainChangedRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{40}
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentChainChangedRequestMessage) GetIncludeAcceptedTransactionIds() bool {
|
|
if x != nil {
|
|
return x.IncludeAcceptedTransactionIds
|
|
}
|
|
return false
|
|
}
|
|
|
|
type NotifyVirtualSelectedParentChainChangedResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentChainChangedResponseMessage) Reset() {
|
|
*x = NotifyVirtualSelectedParentChainChangedResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[41]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentChainChangedResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyVirtualSelectedParentChainChangedResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyVirtualSelectedParentChainChangedResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[41]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyVirtualSelectedParentChainChangedResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyVirtualSelectedParentChainChangedResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{41}
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentChainChangedResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// VirtualSelectedParentChainChangedNotificationMessage is sent whenever the DAG's selected parent
|
|
// chain had changed.
|
|
//
|
|
// See: NotifyVirtualSelectedParentChainChangedRequestMessage
|
|
type VirtualSelectedParentChainChangedNotificationMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
// The chain blocks that were removed, in high-to-low order
|
|
RemovedChainBlockHashes []string `protobuf:"bytes,1,rep,name=removedChainBlockHashes,proto3" json:"removedChainBlockHashes,omitempty"`
|
|
// The chain blocks that were added, in low-to-high order
|
|
AddedChainBlockHashes []string `protobuf:"bytes,3,rep,name=addedChainBlockHashes,proto3" json:"addedChainBlockHashes,omitempty"`
|
|
// Will be filled only if `includeAcceptedTransactionIds = true` in the notify request.
|
|
AcceptedTransactionIds []*AcceptedTransactionIds `protobuf:"bytes,2,rep,name=acceptedTransactionIds,proto3" json:"acceptedTransactionIds,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *VirtualSelectedParentChainChangedNotificationMessage) Reset() {
|
|
*x = VirtualSelectedParentChainChangedNotificationMessage{}
|
|
mi := &file_rpc_proto_msgTypes[42]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *VirtualSelectedParentChainChangedNotificationMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VirtualSelectedParentChainChangedNotificationMessage) ProtoMessage() {}
|
|
|
|
func (x *VirtualSelectedParentChainChangedNotificationMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[42]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VirtualSelectedParentChainChangedNotificationMessage.ProtoReflect.Descriptor instead.
|
|
func (*VirtualSelectedParentChainChangedNotificationMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{42}
|
|
}
|
|
|
|
func (x *VirtualSelectedParentChainChangedNotificationMessage) GetRemovedChainBlockHashes() []string {
|
|
if x != nil {
|
|
return x.RemovedChainBlockHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VirtualSelectedParentChainChangedNotificationMessage) GetAddedChainBlockHashes() []string {
|
|
if x != nil {
|
|
return x.AddedChainBlockHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VirtualSelectedParentChainChangedNotificationMessage) GetAcceptedTransactionIds() []*AcceptedTransactionIds {
|
|
if x != nil {
|
|
return x.AcceptedTransactionIds
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetBlockRequestMessage requests information about a specific block
|
|
type GetBlockRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
// The hash of the requested block
|
|
Hash string `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
// Whether to include transaction data in the response
|
|
IncludeTransactions bool `protobuf:"varint,3,opt,name=includeTransactions,proto3" json:"includeTransactions,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlockRequestMessage) Reset() {
|
|
*x = GetBlockRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[43]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlockRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlockRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlockRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[43]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlockRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlockRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{43}
|
|
}
|
|
|
|
func (x *GetBlockRequestMessage) GetHash() string {
|
|
if x != nil {
|
|
return x.Hash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetBlockRequestMessage) GetIncludeTransactions() bool {
|
|
if x != nil {
|
|
return x.IncludeTransactions
|
|
}
|
|
return false
|
|
}
|
|
|
|
type GetBlockResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Block *RpcBlock `protobuf:"bytes,3,opt,name=block,proto3" json:"block,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlockResponseMessage) Reset() {
|
|
*x = GetBlockResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[44]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlockResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlockResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlockResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[44]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlockResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlockResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{44}
|
|
}
|
|
|
|
func (x *GetBlockResponseMessage) GetBlock() *RpcBlock {
|
|
if x != nil {
|
|
return x.Block
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetBlockResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetSubnetworkRequestMessage requests information about a specific subnetwork
|
|
//
|
|
// Currently unimplemented
|
|
type GetSubnetworkRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
SubnetworkId string `protobuf:"bytes,1,opt,name=subnetworkId,proto3" json:"subnetworkId,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetSubnetworkRequestMessage) Reset() {
|
|
*x = GetSubnetworkRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[45]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetSubnetworkRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetSubnetworkRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetSubnetworkRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[45]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetSubnetworkRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetSubnetworkRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{45}
|
|
}
|
|
|
|
func (x *GetSubnetworkRequestMessage) GetSubnetworkId() string {
|
|
if x != nil {
|
|
return x.SubnetworkId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type GetSubnetworkResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
GasLimit uint64 `protobuf:"varint,1,opt,name=gasLimit,proto3" json:"gasLimit,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetSubnetworkResponseMessage) Reset() {
|
|
*x = GetSubnetworkResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[46]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetSubnetworkResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetSubnetworkResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetSubnetworkResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[46]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetSubnetworkResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetSubnetworkResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{46}
|
|
}
|
|
|
|
func (x *GetSubnetworkResponseMessage) GetGasLimit() uint64 {
|
|
if x != nil {
|
|
return x.GasLimit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetSubnetworkResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetVirtualSelectedParentChainFromBlockRequestMessage requests the virtual selected
|
|
// parent chain from some startHash to this kaspad's current virtual
|
|
type GetVirtualSelectedParentChainFromBlockRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
StartHash string `protobuf:"bytes,1,opt,name=startHash,proto3" json:"startHash,omitempty"`
|
|
IncludeAcceptedTransactionIds bool `protobuf:"varint,2,opt,name=includeAcceptedTransactionIds,proto3" json:"includeAcceptedTransactionIds,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockRequestMessage) Reset() {
|
|
*x = GetVirtualSelectedParentChainFromBlockRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[47]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetVirtualSelectedParentChainFromBlockRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[47]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetVirtualSelectedParentChainFromBlockRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetVirtualSelectedParentChainFromBlockRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{47}
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockRequestMessage) GetStartHash() string {
|
|
if x != nil {
|
|
return x.StartHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockRequestMessage) GetIncludeAcceptedTransactionIds() bool {
|
|
if x != nil {
|
|
return x.IncludeAcceptedTransactionIds
|
|
}
|
|
return false
|
|
}
|
|
|
|
type AcceptedTransactionIds struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
AcceptingBlockHash string `protobuf:"bytes,1,opt,name=acceptingBlockHash,proto3" json:"acceptingBlockHash,omitempty"`
|
|
AcceptedTransactionIds []string `protobuf:"bytes,2,rep,name=acceptedTransactionIds,proto3" json:"acceptedTransactionIds,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *AcceptedTransactionIds) Reset() {
|
|
*x = AcceptedTransactionIds{}
|
|
mi := &file_rpc_proto_msgTypes[48]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *AcceptedTransactionIds) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AcceptedTransactionIds) ProtoMessage() {}
|
|
|
|
func (x *AcceptedTransactionIds) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[48]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AcceptedTransactionIds.ProtoReflect.Descriptor instead.
|
|
func (*AcceptedTransactionIds) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{48}
|
|
}
|
|
|
|
func (x *AcceptedTransactionIds) GetAcceptingBlockHash() string {
|
|
if x != nil {
|
|
return x.AcceptingBlockHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *AcceptedTransactionIds) GetAcceptedTransactionIds() []string {
|
|
if x != nil {
|
|
return x.AcceptedTransactionIds
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetVirtualSelectedParentChainFromBlockResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
// The chain blocks that were removed, in high-to-low order
|
|
RemovedChainBlockHashes []string `protobuf:"bytes,1,rep,name=removedChainBlockHashes,proto3" json:"removedChainBlockHashes,omitempty"`
|
|
// The chain blocks that were added, in low-to-high order
|
|
AddedChainBlockHashes []string `protobuf:"bytes,3,rep,name=addedChainBlockHashes,proto3" json:"addedChainBlockHashes,omitempty"`
|
|
// The transactions accepted by each block in addedChainBlockHashes.
|
|
// Will be filled only if `includeAcceptedTransactionIds = true` in the request.
|
|
AcceptedTransactionIds []*AcceptedTransactionIds `protobuf:"bytes,2,rep,name=acceptedTransactionIds,proto3" json:"acceptedTransactionIds,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockResponseMessage) Reset() {
|
|
*x = GetVirtualSelectedParentChainFromBlockResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[49]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetVirtualSelectedParentChainFromBlockResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[49]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetVirtualSelectedParentChainFromBlockResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetVirtualSelectedParentChainFromBlockResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{49}
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockResponseMessage) GetRemovedChainBlockHashes() []string {
|
|
if x != nil {
|
|
return x.RemovedChainBlockHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockResponseMessage) GetAddedChainBlockHashes() []string {
|
|
if x != nil {
|
|
return x.AddedChainBlockHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockResponseMessage) GetAcceptedTransactionIds() []*AcceptedTransactionIds {
|
|
if x != nil {
|
|
return x.AcceptedTransactionIds
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentChainFromBlockResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetBlocksRequestMessage requests blocks between a certain block lowHash up to this
|
|
// kaspad's current virtual.
|
|
type GetBlocksRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
LowHash string `protobuf:"bytes,1,opt,name=lowHash,proto3" json:"lowHash,omitempty"`
|
|
IncludeBlocks bool `protobuf:"varint,2,opt,name=includeBlocks,proto3" json:"includeBlocks,omitempty"`
|
|
IncludeTransactions bool `protobuf:"varint,3,opt,name=includeTransactions,proto3" json:"includeTransactions,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlocksRequestMessage) Reset() {
|
|
*x = GetBlocksRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[50]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlocksRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlocksRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlocksRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[50]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlocksRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlocksRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{50}
|
|
}
|
|
|
|
func (x *GetBlocksRequestMessage) GetLowHash() string {
|
|
if x != nil {
|
|
return x.LowHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetBlocksRequestMessage) GetIncludeBlocks() bool {
|
|
if x != nil {
|
|
return x.IncludeBlocks
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetBlocksRequestMessage) GetIncludeTransactions() bool {
|
|
if x != nil {
|
|
return x.IncludeTransactions
|
|
}
|
|
return false
|
|
}
|
|
|
|
type GetBlocksResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BlockHashes []string `protobuf:"bytes,4,rep,name=blockHashes,proto3" json:"blockHashes,omitempty"`
|
|
Blocks []*RpcBlock `protobuf:"bytes,3,rep,name=blocks,proto3" json:"blocks,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlocksResponseMessage) Reset() {
|
|
*x = GetBlocksResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[51]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlocksResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlocksResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlocksResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[51]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlocksResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlocksResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{51}
|
|
}
|
|
|
|
func (x *GetBlocksResponseMessage) GetBlockHashes() []string {
|
|
if x != nil {
|
|
return x.BlockHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetBlocksResponseMessage) GetBlocks() []*RpcBlock {
|
|
if x != nil {
|
|
return x.Blocks
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetBlocksResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetBlockCountRequestMessage requests the current number of blocks in this kaspad.
|
|
// Note that this number may decrease as pruning occurs.
|
|
type GetBlockCountRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlockCountRequestMessage) Reset() {
|
|
*x = GetBlockCountRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[52]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlockCountRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlockCountRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlockCountRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[52]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlockCountRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlockCountRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{52}
|
|
}
|
|
|
|
type GetBlockCountResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BlockCount uint64 `protobuf:"varint,1,opt,name=blockCount,proto3" json:"blockCount,omitempty"`
|
|
HeaderCount uint64 `protobuf:"varint,2,opt,name=headerCount,proto3" json:"headerCount,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlockCountResponseMessage) Reset() {
|
|
*x = GetBlockCountResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[53]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlockCountResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlockCountResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlockCountResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[53]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlockCountResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlockCountResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{53}
|
|
}
|
|
|
|
func (x *GetBlockCountResponseMessage) GetBlockCount() uint64 {
|
|
if x != nil {
|
|
return x.BlockCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetBlockCountResponseMessage) GetHeaderCount() uint64 {
|
|
if x != nil {
|
|
return x.HeaderCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetBlockCountResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetBlockDagInfoRequestMessage requests general information about the current state
|
|
// of this kaspad's DAG.
|
|
type GetBlockDagInfoRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlockDagInfoRequestMessage) Reset() {
|
|
*x = GetBlockDagInfoRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[54]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlockDagInfoRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlockDagInfoRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlockDagInfoRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[54]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlockDagInfoRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlockDagInfoRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{54}
|
|
}
|
|
|
|
type GetBlockDagInfoResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
NetworkName string `protobuf:"bytes,1,opt,name=networkName,proto3" json:"networkName,omitempty"`
|
|
BlockCount uint64 `protobuf:"varint,2,opt,name=blockCount,proto3" json:"blockCount,omitempty"`
|
|
HeaderCount uint64 `protobuf:"varint,3,opt,name=headerCount,proto3" json:"headerCount,omitempty"`
|
|
TipHashes []string `protobuf:"bytes,4,rep,name=tipHashes,proto3" json:"tipHashes,omitempty"`
|
|
Difficulty float64 `protobuf:"fixed64,5,opt,name=difficulty,proto3" json:"difficulty,omitempty"`
|
|
PastMedianTime int64 `protobuf:"varint,6,opt,name=pastMedianTime,proto3" json:"pastMedianTime,omitempty"`
|
|
VirtualParentHashes []string `protobuf:"bytes,7,rep,name=virtualParentHashes,proto3" json:"virtualParentHashes,omitempty"`
|
|
PruningPointHash string `protobuf:"bytes,8,opt,name=pruningPointHash,proto3" json:"pruningPointHash,omitempty"`
|
|
VirtualDaaScore uint64 `protobuf:"varint,9,opt,name=virtualDaaScore,proto3" json:"virtualDaaScore,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) Reset() {
|
|
*x = GetBlockDagInfoResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[55]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBlockDagInfoResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[55]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBlockDagInfoResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBlockDagInfoResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{55}
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetNetworkName() string {
|
|
if x != nil {
|
|
return x.NetworkName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetBlockCount() uint64 {
|
|
if x != nil {
|
|
return x.BlockCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetHeaderCount() uint64 {
|
|
if x != nil {
|
|
return x.HeaderCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetTipHashes() []string {
|
|
if x != nil {
|
|
return x.TipHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetDifficulty() float64 {
|
|
if x != nil {
|
|
return x.Difficulty
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetPastMedianTime() int64 {
|
|
if x != nil {
|
|
return x.PastMedianTime
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetVirtualParentHashes() []string {
|
|
if x != nil {
|
|
return x.VirtualParentHashes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetPruningPointHash() string {
|
|
if x != nil {
|
|
return x.PruningPointHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetVirtualDaaScore() uint64 {
|
|
if x != nil {
|
|
return x.VirtualDaaScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetBlockDagInfoResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ResolveFinalityConflictRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
FinalityBlockHash string `protobuf:"bytes,1,opt,name=finalityBlockHash,proto3" json:"finalityBlockHash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ResolveFinalityConflictRequestMessage) Reset() {
|
|
*x = ResolveFinalityConflictRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[56]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ResolveFinalityConflictRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ResolveFinalityConflictRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *ResolveFinalityConflictRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[56]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ResolveFinalityConflictRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*ResolveFinalityConflictRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{56}
|
|
}
|
|
|
|
func (x *ResolveFinalityConflictRequestMessage) GetFinalityBlockHash() string {
|
|
if x != nil {
|
|
return x.FinalityBlockHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type ResolveFinalityConflictResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ResolveFinalityConflictResponseMessage) Reset() {
|
|
*x = ResolveFinalityConflictResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[57]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ResolveFinalityConflictResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ResolveFinalityConflictResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *ResolveFinalityConflictResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[57]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ResolveFinalityConflictResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*ResolveFinalityConflictResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{57}
|
|
}
|
|
|
|
func (x *ResolveFinalityConflictResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type NotifyFinalityConflictsRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyFinalityConflictsRequestMessage) Reset() {
|
|
*x = NotifyFinalityConflictsRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[58]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyFinalityConflictsRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyFinalityConflictsRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyFinalityConflictsRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[58]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyFinalityConflictsRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyFinalityConflictsRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{58}
|
|
}
|
|
|
|
type NotifyFinalityConflictsResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyFinalityConflictsResponseMessage) Reset() {
|
|
*x = NotifyFinalityConflictsResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[59]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyFinalityConflictsResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyFinalityConflictsResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyFinalityConflictsResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[59]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyFinalityConflictsResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyFinalityConflictsResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{59}
|
|
}
|
|
|
|
func (x *NotifyFinalityConflictsResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type FinalityConflictNotificationMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
ViolatingBlockHash string `protobuf:"bytes,1,opt,name=violatingBlockHash,proto3" json:"violatingBlockHash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *FinalityConflictNotificationMessage) Reset() {
|
|
*x = FinalityConflictNotificationMessage{}
|
|
mi := &file_rpc_proto_msgTypes[60]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *FinalityConflictNotificationMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*FinalityConflictNotificationMessage) ProtoMessage() {}
|
|
|
|
func (x *FinalityConflictNotificationMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[60]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use FinalityConflictNotificationMessage.ProtoReflect.Descriptor instead.
|
|
func (*FinalityConflictNotificationMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{60}
|
|
}
|
|
|
|
func (x *FinalityConflictNotificationMessage) GetViolatingBlockHash() string {
|
|
if x != nil {
|
|
return x.ViolatingBlockHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type FinalityConflictResolvedNotificationMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
FinalityBlockHash string `protobuf:"bytes,1,opt,name=finalityBlockHash,proto3" json:"finalityBlockHash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *FinalityConflictResolvedNotificationMessage) Reset() {
|
|
*x = FinalityConflictResolvedNotificationMessage{}
|
|
mi := &file_rpc_proto_msgTypes[61]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *FinalityConflictResolvedNotificationMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*FinalityConflictResolvedNotificationMessage) ProtoMessage() {}
|
|
|
|
func (x *FinalityConflictResolvedNotificationMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[61]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use FinalityConflictResolvedNotificationMessage.ProtoReflect.Descriptor instead.
|
|
func (*FinalityConflictResolvedNotificationMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{61}
|
|
}
|
|
|
|
func (x *FinalityConflictResolvedNotificationMessage) GetFinalityBlockHash() string {
|
|
if x != nil {
|
|
return x.FinalityBlockHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
// ShutDownRequestMessage shuts down this kaspad.
|
|
type ShutDownRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ShutDownRequestMessage) Reset() {
|
|
*x = ShutDownRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[62]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ShutDownRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ShutDownRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *ShutDownRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[62]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ShutDownRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*ShutDownRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{62}
|
|
}
|
|
|
|
type ShutDownResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ShutDownResponseMessage) Reset() {
|
|
*x = ShutDownResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[63]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ShutDownResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ShutDownResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *ShutDownResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[63]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ShutDownResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*ShutDownResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{63}
|
|
}
|
|
|
|
func (x *ShutDownResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetHeadersRequestMessage requests headers between the given startHash and the
|
|
// current virtual, up to the given limit.
|
|
type GetHeadersRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
StartHash string `protobuf:"bytes,1,opt,name=startHash,proto3" json:"startHash,omitempty"`
|
|
Limit uint64 `protobuf:"varint,2,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
IsAscending bool `protobuf:"varint,3,opt,name=isAscending,proto3" json:"isAscending,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetHeadersRequestMessage) Reset() {
|
|
*x = GetHeadersRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[64]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetHeadersRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetHeadersRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetHeadersRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[64]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetHeadersRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetHeadersRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{64}
|
|
}
|
|
|
|
func (x *GetHeadersRequestMessage) GetStartHash() string {
|
|
if x != nil {
|
|
return x.StartHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetHeadersRequestMessage) GetLimit() uint64 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetHeadersRequestMessage) GetIsAscending() bool {
|
|
if x != nil {
|
|
return x.IsAscending
|
|
}
|
|
return false
|
|
}
|
|
|
|
type GetHeadersResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Headers []string `protobuf:"bytes,1,rep,name=headers,proto3" json:"headers,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetHeadersResponseMessage) Reset() {
|
|
*x = GetHeadersResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[65]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetHeadersResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetHeadersResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetHeadersResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[65]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetHeadersResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetHeadersResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{65}
|
|
}
|
|
|
|
func (x *GetHeadersResponseMessage) GetHeaders() []string {
|
|
if x != nil {
|
|
return x.Headers
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetHeadersResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// NotifyUtxosChangedRequestMessage registers this connection for utxoChanged notifications
|
|
// for the given addresses.
|
|
//
|
|
// This call is only available when this kaspad was started with `--utxoindex`
|
|
//
|
|
// See: UtxosChangedNotificationMessage
|
|
type NotifyUtxosChangedRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Addresses []string `protobuf:"bytes,1,rep,name=addresses,proto3" json:"addresses,omitempty"` // Leave empty to get all updates
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyUtxosChangedRequestMessage) Reset() {
|
|
*x = NotifyUtxosChangedRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[66]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyUtxosChangedRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyUtxosChangedRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyUtxosChangedRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[66]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyUtxosChangedRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyUtxosChangedRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{66}
|
|
}
|
|
|
|
func (x *NotifyUtxosChangedRequestMessage) GetAddresses() []string {
|
|
if x != nil {
|
|
return x.Addresses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type NotifyUtxosChangedResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyUtxosChangedResponseMessage) Reset() {
|
|
*x = NotifyUtxosChangedResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[67]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyUtxosChangedResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyUtxosChangedResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyUtxosChangedResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[67]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyUtxosChangedResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyUtxosChangedResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{67}
|
|
}
|
|
|
|
func (x *NotifyUtxosChangedResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// UtxosChangedNotificationMessage is sent whenever the UTXO index had been updated.
|
|
//
|
|
// See: NotifyUtxosChangedRequestMessage
|
|
type UtxosChangedNotificationMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Added []*UtxosByAddressesEntry `protobuf:"bytes,1,rep,name=added,proto3" json:"added,omitempty"`
|
|
Removed []*UtxosByAddressesEntry `protobuf:"bytes,2,rep,name=removed,proto3" json:"removed,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *UtxosChangedNotificationMessage) Reset() {
|
|
*x = UtxosChangedNotificationMessage{}
|
|
mi := &file_rpc_proto_msgTypes[68]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *UtxosChangedNotificationMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UtxosChangedNotificationMessage) ProtoMessage() {}
|
|
|
|
func (x *UtxosChangedNotificationMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[68]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UtxosChangedNotificationMessage.ProtoReflect.Descriptor instead.
|
|
func (*UtxosChangedNotificationMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{68}
|
|
}
|
|
|
|
func (x *UtxosChangedNotificationMessage) GetAdded() []*UtxosByAddressesEntry {
|
|
if x != nil {
|
|
return x.Added
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UtxosChangedNotificationMessage) GetRemoved() []*UtxosByAddressesEntry {
|
|
if x != nil {
|
|
return x.Removed
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UtxosByAddressesEntry struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Address string `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
Outpoint *RpcOutpoint `protobuf:"bytes,2,opt,name=outpoint,proto3" json:"outpoint,omitempty"`
|
|
UtxoEntry *RpcUtxoEntry `protobuf:"bytes,3,opt,name=utxoEntry,proto3" json:"utxoEntry,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *UtxosByAddressesEntry) Reset() {
|
|
*x = UtxosByAddressesEntry{}
|
|
mi := &file_rpc_proto_msgTypes[69]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *UtxosByAddressesEntry) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UtxosByAddressesEntry) ProtoMessage() {}
|
|
|
|
func (x *UtxosByAddressesEntry) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[69]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UtxosByAddressesEntry.ProtoReflect.Descriptor instead.
|
|
func (*UtxosByAddressesEntry) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{69}
|
|
}
|
|
|
|
func (x *UtxosByAddressesEntry) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UtxosByAddressesEntry) GetOutpoint() *RpcOutpoint {
|
|
if x != nil {
|
|
return x.Outpoint
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UtxosByAddressesEntry) GetUtxoEntry() *RpcUtxoEntry {
|
|
if x != nil {
|
|
return x.UtxoEntry
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// StopNotifyingUtxosChangedRequestMessage unregisters this connection for utxoChanged notifications
|
|
// for the given addresses.
|
|
//
|
|
// This call is only available when this kaspad was started with `--utxoindex`
|
|
//
|
|
// See: UtxosChangedNotificationMessage
|
|
type StopNotifyingUtxosChangedRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Addresses []string `protobuf:"bytes,1,rep,name=addresses,proto3" json:"addresses,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *StopNotifyingUtxosChangedRequestMessage) Reset() {
|
|
*x = StopNotifyingUtxosChangedRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[70]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *StopNotifyingUtxosChangedRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StopNotifyingUtxosChangedRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *StopNotifyingUtxosChangedRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[70]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StopNotifyingUtxosChangedRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*StopNotifyingUtxosChangedRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{70}
|
|
}
|
|
|
|
func (x *StopNotifyingUtxosChangedRequestMessage) GetAddresses() []string {
|
|
if x != nil {
|
|
return x.Addresses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type StopNotifyingUtxosChangedResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *StopNotifyingUtxosChangedResponseMessage) Reset() {
|
|
*x = StopNotifyingUtxosChangedResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[71]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *StopNotifyingUtxosChangedResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StopNotifyingUtxosChangedResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *StopNotifyingUtxosChangedResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[71]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StopNotifyingUtxosChangedResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*StopNotifyingUtxosChangedResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{71}
|
|
}
|
|
|
|
func (x *StopNotifyingUtxosChangedResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetUtxosByAddressesRequestMessage requests all current UTXOs for the given kaspad addresses
|
|
//
|
|
// This call is only available when this kaspad was started with `--utxoindex`
|
|
type GetUtxosByAddressesRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Addresses []string `protobuf:"bytes,1,rep,name=addresses,proto3" json:"addresses,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetUtxosByAddressesRequestMessage) Reset() {
|
|
*x = GetUtxosByAddressesRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[72]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetUtxosByAddressesRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetUtxosByAddressesRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetUtxosByAddressesRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[72]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetUtxosByAddressesRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetUtxosByAddressesRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{72}
|
|
}
|
|
|
|
func (x *GetUtxosByAddressesRequestMessage) GetAddresses() []string {
|
|
if x != nil {
|
|
return x.Addresses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetUtxosByAddressesResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Entries []*UtxosByAddressesEntry `protobuf:"bytes,1,rep,name=entries,proto3" json:"entries,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetUtxosByAddressesResponseMessage) Reset() {
|
|
*x = GetUtxosByAddressesResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[73]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetUtxosByAddressesResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetUtxosByAddressesResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetUtxosByAddressesResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[73]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetUtxosByAddressesResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetUtxosByAddressesResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{73}
|
|
}
|
|
|
|
func (x *GetUtxosByAddressesResponseMessage) GetEntries() []*UtxosByAddressesEntry {
|
|
if x != nil {
|
|
return x.Entries
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetUtxosByAddressesResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetBalanceByAddressRequest returns the total balance in unspent transactions towards a given address
|
|
//
|
|
// This call is only available when this kaspad was started with `--utxoindex`
|
|
type GetBalanceByAddressRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Address string `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBalanceByAddressRequestMessage) Reset() {
|
|
*x = GetBalanceByAddressRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[74]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBalanceByAddressRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBalanceByAddressRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBalanceByAddressRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[74]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBalanceByAddressRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBalanceByAddressRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{74}
|
|
}
|
|
|
|
func (x *GetBalanceByAddressRequestMessage) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type GetBalanceByAddressResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Balance uint64 `protobuf:"varint,1,opt,name=balance,proto3" json:"balance,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBalanceByAddressResponseMessage) Reset() {
|
|
*x = GetBalanceByAddressResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[75]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBalanceByAddressResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBalanceByAddressResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBalanceByAddressResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[75]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBalanceByAddressResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBalanceByAddressResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{75}
|
|
}
|
|
|
|
func (x *GetBalanceByAddressResponseMessage) GetBalance() uint64 {
|
|
if x != nil {
|
|
return x.Balance
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetBalanceByAddressResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetBalancesByAddressesRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Addresses []string `protobuf:"bytes,1,rep,name=addresses,proto3" json:"addresses,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBalancesByAddressesRequestMessage) Reset() {
|
|
*x = GetBalancesByAddressesRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[76]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBalancesByAddressesRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBalancesByAddressesRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBalancesByAddressesRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[76]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBalancesByAddressesRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBalancesByAddressesRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{76}
|
|
}
|
|
|
|
func (x *GetBalancesByAddressesRequestMessage) GetAddresses() []string {
|
|
if x != nil {
|
|
return x.Addresses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BalancesByAddressEntry struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Address string `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
Balance uint64 `protobuf:"varint,2,opt,name=balance,proto3" json:"balance,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BalancesByAddressEntry) Reset() {
|
|
*x = BalancesByAddressEntry{}
|
|
mi := &file_rpc_proto_msgTypes[77]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BalancesByAddressEntry) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BalancesByAddressEntry) ProtoMessage() {}
|
|
|
|
func (x *BalancesByAddressEntry) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[77]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BalancesByAddressEntry.ProtoReflect.Descriptor instead.
|
|
func (*BalancesByAddressEntry) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{77}
|
|
}
|
|
|
|
func (x *BalancesByAddressEntry) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BalancesByAddressEntry) GetBalance() uint64 {
|
|
if x != nil {
|
|
return x.Balance
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BalancesByAddressEntry) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetBalancesByAddressesResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Entries []*BalancesByAddressEntry `protobuf:"bytes,1,rep,name=entries,proto3" json:"entries,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetBalancesByAddressesResponseMessage) Reset() {
|
|
*x = GetBalancesByAddressesResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[78]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetBalancesByAddressesResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetBalancesByAddressesResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetBalancesByAddressesResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[78]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetBalancesByAddressesResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetBalancesByAddressesResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{78}
|
|
}
|
|
|
|
func (x *GetBalancesByAddressesResponseMessage) GetEntries() []*BalancesByAddressEntry {
|
|
if x != nil {
|
|
return x.Entries
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetBalancesByAddressesResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetVirtualSelectedParentBlueScoreRequestMessage requests the blue score of the current selected parent
|
|
// of the virtual block.
|
|
type GetVirtualSelectedParentBlueScoreRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentBlueScoreRequestMessage) Reset() {
|
|
*x = GetVirtualSelectedParentBlueScoreRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[79]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentBlueScoreRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetVirtualSelectedParentBlueScoreRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetVirtualSelectedParentBlueScoreRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[79]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetVirtualSelectedParentBlueScoreRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetVirtualSelectedParentBlueScoreRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{79}
|
|
}
|
|
|
|
type GetVirtualSelectedParentBlueScoreResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BlueScore uint64 `protobuf:"varint,1,opt,name=blueScore,proto3" json:"blueScore,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentBlueScoreResponseMessage) Reset() {
|
|
*x = GetVirtualSelectedParentBlueScoreResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[80]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentBlueScoreResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetVirtualSelectedParentBlueScoreResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetVirtualSelectedParentBlueScoreResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[80]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetVirtualSelectedParentBlueScoreResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetVirtualSelectedParentBlueScoreResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{80}
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentBlueScoreResponseMessage) GetBlueScore() uint64 {
|
|
if x != nil {
|
|
return x.BlueScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetVirtualSelectedParentBlueScoreResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// NotifyVirtualSelectedParentBlueScoreChangedRequestMessage registers this connection for
|
|
// virtualSelectedParentBlueScoreChanged notifications.
|
|
//
|
|
// See: VirtualSelectedParentBlueScoreChangedNotificationMessage
|
|
type NotifyVirtualSelectedParentBlueScoreChangedRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentBlueScoreChangedRequestMessage) Reset() {
|
|
*x = NotifyVirtualSelectedParentBlueScoreChangedRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[81]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentBlueScoreChangedRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyVirtualSelectedParentBlueScoreChangedRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyVirtualSelectedParentBlueScoreChangedRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[81]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyVirtualSelectedParentBlueScoreChangedRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyVirtualSelectedParentBlueScoreChangedRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{81}
|
|
}
|
|
|
|
type NotifyVirtualSelectedParentBlueScoreChangedResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentBlueScoreChangedResponseMessage) Reset() {
|
|
*x = NotifyVirtualSelectedParentBlueScoreChangedResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[82]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentBlueScoreChangedResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyVirtualSelectedParentBlueScoreChangedResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyVirtualSelectedParentBlueScoreChangedResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[82]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyVirtualSelectedParentBlueScoreChangedResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyVirtualSelectedParentBlueScoreChangedResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{82}
|
|
}
|
|
|
|
func (x *NotifyVirtualSelectedParentBlueScoreChangedResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// VirtualSelectedParentBlueScoreChangedNotificationMessage is sent whenever the blue score
|
|
// of the virtual's selected parent changes.
|
|
//
|
|
// See NotifyVirtualSelectedParentBlueScoreChangedRequestMessage
|
|
type VirtualSelectedParentBlueScoreChangedNotificationMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
VirtualSelectedParentBlueScore uint64 `protobuf:"varint,1,opt,name=virtualSelectedParentBlueScore,proto3" json:"virtualSelectedParentBlueScore,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *VirtualSelectedParentBlueScoreChangedNotificationMessage) Reset() {
|
|
*x = VirtualSelectedParentBlueScoreChangedNotificationMessage{}
|
|
mi := &file_rpc_proto_msgTypes[83]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *VirtualSelectedParentBlueScoreChangedNotificationMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VirtualSelectedParentBlueScoreChangedNotificationMessage) ProtoMessage() {}
|
|
|
|
func (x *VirtualSelectedParentBlueScoreChangedNotificationMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[83]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VirtualSelectedParentBlueScoreChangedNotificationMessage.ProtoReflect.Descriptor instead.
|
|
func (*VirtualSelectedParentBlueScoreChangedNotificationMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{83}
|
|
}
|
|
|
|
func (x *VirtualSelectedParentBlueScoreChangedNotificationMessage) GetVirtualSelectedParentBlueScore() uint64 {
|
|
if x != nil {
|
|
return x.VirtualSelectedParentBlueScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
// NotifyVirtualDaaScoreChangedRequestMessage registers this connection for
|
|
// virtualDaaScoreChanged notifications.
|
|
//
|
|
// See: VirtualDaaScoreChangedNotificationMessage
|
|
type NotifyVirtualDaaScoreChangedRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyVirtualDaaScoreChangedRequestMessage) Reset() {
|
|
*x = NotifyVirtualDaaScoreChangedRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[84]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyVirtualDaaScoreChangedRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyVirtualDaaScoreChangedRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyVirtualDaaScoreChangedRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[84]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyVirtualDaaScoreChangedRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyVirtualDaaScoreChangedRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{84}
|
|
}
|
|
|
|
type NotifyVirtualDaaScoreChangedResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyVirtualDaaScoreChangedResponseMessage) Reset() {
|
|
*x = NotifyVirtualDaaScoreChangedResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[85]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyVirtualDaaScoreChangedResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyVirtualDaaScoreChangedResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyVirtualDaaScoreChangedResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[85]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyVirtualDaaScoreChangedResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyVirtualDaaScoreChangedResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{85}
|
|
}
|
|
|
|
func (x *NotifyVirtualDaaScoreChangedResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// VirtualDaaScoreChangedNotificationMessage is sent whenever the DAA score
|
|
// of the virtual changes.
|
|
//
|
|
// See NotifyVirtualDaaScoreChangedRequestMessage
|
|
type VirtualDaaScoreChangedNotificationMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
VirtualDaaScore uint64 `protobuf:"varint,1,opt,name=virtualDaaScore,proto3" json:"virtualDaaScore,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *VirtualDaaScoreChangedNotificationMessage) Reset() {
|
|
*x = VirtualDaaScoreChangedNotificationMessage{}
|
|
mi := &file_rpc_proto_msgTypes[86]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *VirtualDaaScoreChangedNotificationMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VirtualDaaScoreChangedNotificationMessage) ProtoMessage() {}
|
|
|
|
func (x *VirtualDaaScoreChangedNotificationMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[86]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VirtualDaaScoreChangedNotificationMessage.ProtoReflect.Descriptor instead.
|
|
func (*VirtualDaaScoreChangedNotificationMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{86}
|
|
}
|
|
|
|
func (x *VirtualDaaScoreChangedNotificationMessage) GetVirtualDaaScore() uint64 {
|
|
if x != nil {
|
|
return x.VirtualDaaScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
// NotifyPruningPointUTXOSetOverrideRequestMessage registers this connection for
|
|
// pruning point UTXO set override notifications.
|
|
//
|
|
// This call is only available when this kaspad was started with `--utxoindex`
|
|
//
|
|
// See: NotifyPruningPointUTXOSetOverrideResponseMessage
|
|
type NotifyPruningPointUTXOSetOverrideRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyPruningPointUTXOSetOverrideRequestMessage) Reset() {
|
|
*x = NotifyPruningPointUTXOSetOverrideRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[87]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyPruningPointUTXOSetOverrideRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyPruningPointUTXOSetOverrideRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyPruningPointUTXOSetOverrideRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[87]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyPruningPointUTXOSetOverrideRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyPruningPointUTXOSetOverrideRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{87}
|
|
}
|
|
|
|
type NotifyPruningPointUTXOSetOverrideResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyPruningPointUTXOSetOverrideResponseMessage) Reset() {
|
|
*x = NotifyPruningPointUTXOSetOverrideResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[88]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyPruningPointUTXOSetOverrideResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyPruningPointUTXOSetOverrideResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyPruningPointUTXOSetOverrideResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[88]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyPruningPointUTXOSetOverrideResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyPruningPointUTXOSetOverrideResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{88}
|
|
}
|
|
|
|
func (x *NotifyPruningPointUTXOSetOverrideResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// PruningPointUTXOSetOverrideNotificationMessage is sent whenever the UTXO index
|
|
// resets due to pruning point change via IBD.
|
|
//
|
|
// See NotifyPruningPointUTXOSetOverrideRequestMessage
|
|
type PruningPointUTXOSetOverrideNotificationMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *PruningPointUTXOSetOverrideNotificationMessage) Reset() {
|
|
*x = PruningPointUTXOSetOverrideNotificationMessage{}
|
|
mi := &file_rpc_proto_msgTypes[89]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *PruningPointUTXOSetOverrideNotificationMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PruningPointUTXOSetOverrideNotificationMessage) ProtoMessage() {}
|
|
|
|
func (x *PruningPointUTXOSetOverrideNotificationMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[89]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PruningPointUTXOSetOverrideNotificationMessage.ProtoReflect.Descriptor instead.
|
|
func (*PruningPointUTXOSetOverrideNotificationMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{89}
|
|
}
|
|
|
|
// StopNotifyingPruningPointUTXOSetOverrideRequestMessage unregisters this connection for
|
|
// pruning point UTXO set override notifications.
|
|
//
|
|
// This call is only available when this kaspad was started with `--utxoindex`
|
|
//
|
|
// See: PruningPointUTXOSetOverrideNotificationMessage
|
|
type StopNotifyingPruningPointUTXOSetOverrideRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *StopNotifyingPruningPointUTXOSetOverrideRequestMessage) Reset() {
|
|
*x = StopNotifyingPruningPointUTXOSetOverrideRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[90]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *StopNotifyingPruningPointUTXOSetOverrideRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StopNotifyingPruningPointUTXOSetOverrideRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *StopNotifyingPruningPointUTXOSetOverrideRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[90]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StopNotifyingPruningPointUTXOSetOverrideRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*StopNotifyingPruningPointUTXOSetOverrideRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{90}
|
|
}
|
|
|
|
type StopNotifyingPruningPointUTXOSetOverrideResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *StopNotifyingPruningPointUTXOSetOverrideResponseMessage) Reset() {
|
|
*x = StopNotifyingPruningPointUTXOSetOverrideResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[91]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *StopNotifyingPruningPointUTXOSetOverrideResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StopNotifyingPruningPointUTXOSetOverrideResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *StopNotifyingPruningPointUTXOSetOverrideResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[91]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StopNotifyingPruningPointUTXOSetOverrideResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*StopNotifyingPruningPointUTXOSetOverrideResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{91}
|
|
}
|
|
|
|
func (x *StopNotifyingPruningPointUTXOSetOverrideResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// BanRequestMessage bans the given ip.
|
|
type BanRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Ip string `protobuf:"bytes,1,opt,name=ip,proto3" json:"ip,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BanRequestMessage) Reset() {
|
|
*x = BanRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[92]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BanRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BanRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *BanRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[92]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BanRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*BanRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{92}
|
|
}
|
|
|
|
func (x *BanRequestMessage) GetIp() string {
|
|
if x != nil {
|
|
return x.Ip
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type BanResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BanResponseMessage) Reset() {
|
|
*x = BanResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[93]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BanResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BanResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *BanResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[93]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BanResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*BanResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{93}
|
|
}
|
|
|
|
func (x *BanResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// UnbanRequestMessage unbans the given ip.
|
|
type UnbanRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Ip string `protobuf:"bytes,1,opt,name=ip,proto3" json:"ip,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *UnbanRequestMessage) Reset() {
|
|
*x = UnbanRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[94]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *UnbanRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UnbanRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *UnbanRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[94]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UnbanRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*UnbanRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{94}
|
|
}
|
|
|
|
func (x *UnbanRequestMessage) GetIp() string {
|
|
if x != nil {
|
|
return x.Ip
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UnbanResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *UnbanResponseMessage) Reset() {
|
|
*x = UnbanResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[95]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *UnbanResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UnbanResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *UnbanResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[95]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UnbanResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*UnbanResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{95}
|
|
}
|
|
|
|
func (x *UnbanResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GetInfoRequestMessage returns info about the node.
|
|
type GetInfoRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetInfoRequestMessage) Reset() {
|
|
*x = GetInfoRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[96]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetInfoRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetInfoRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetInfoRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[96]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetInfoRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetInfoRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{96}
|
|
}
|
|
|
|
type GetInfoResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
P2PId string `protobuf:"bytes,1,opt,name=p2pId,proto3" json:"p2pId,omitempty"`
|
|
MempoolSize uint64 `protobuf:"varint,2,opt,name=mempoolSize,proto3" json:"mempoolSize,omitempty"`
|
|
ServerVersion string `protobuf:"bytes,3,opt,name=serverVersion,proto3" json:"serverVersion,omitempty"`
|
|
IsUtxoIndexed bool `protobuf:"varint,4,opt,name=isUtxoIndexed,proto3" json:"isUtxoIndexed,omitempty"`
|
|
IsSynced bool `protobuf:"varint,5,opt,name=isSynced,proto3" json:"isSynced,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetInfoResponseMessage) Reset() {
|
|
*x = GetInfoResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[97]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetInfoResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetInfoResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetInfoResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[97]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetInfoResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetInfoResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{97}
|
|
}
|
|
|
|
func (x *GetInfoResponseMessage) GetP2PId() string {
|
|
if x != nil {
|
|
return x.P2PId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetInfoResponseMessage) GetMempoolSize() uint64 {
|
|
if x != nil {
|
|
return x.MempoolSize
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetInfoResponseMessage) GetServerVersion() string {
|
|
if x != nil {
|
|
return x.ServerVersion
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetInfoResponseMessage) GetIsUtxoIndexed() bool {
|
|
if x != nil {
|
|
return x.IsUtxoIndexed
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetInfoResponseMessage) GetIsSynced() bool {
|
|
if x != nil {
|
|
return x.IsSynced
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetInfoResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type EstimateNetworkHashesPerSecondRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
WindowSize uint32 `protobuf:"varint,1,opt,name=windowSize,proto3" json:"windowSize,omitempty"`
|
|
StartHash string `protobuf:"bytes,2,opt,name=startHash,proto3" json:"startHash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondRequestMessage) Reset() {
|
|
*x = EstimateNetworkHashesPerSecondRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[98]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EstimateNetworkHashesPerSecondRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[98]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EstimateNetworkHashesPerSecondRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*EstimateNetworkHashesPerSecondRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{98}
|
|
}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondRequestMessage) GetWindowSize() uint32 {
|
|
if x != nil {
|
|
return x.WindowSize
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondRequestMessage) GetStartHash() string {
|
|
if x != nil {
|
|
return x.StartHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type EstimateNetworkHashesPerSecondResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
NetworkHashesPerSecond uint64 `protobuf:"varint,1,opt,name=networkHashesPerSecond,proto3" json:"networkHashesPerSecond,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondResponseMessage) Reset() {
|
|
*x = EstimateNetworkHashesPerSecondResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[99]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EstimateNetworkHashesPerSecondResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[99]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EstimateNetworkHashesPerSecondResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*EstimateNetworkHashesPerSecondResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{99}
|
|
}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondResponseMessage) GetNetworkHashesPerSecond() uint64 {
|
|
if x != nil {
|
|
return x.NetworkHashesPerSecond
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *EstimateNetworkHashesPerSecondResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// NotifyNewBlockTemplateRequestMessage registers this connection for
|
|
// NewBlockTemplate notifications.
|
|
//
|
|
// See: NewBlockTemplateNotificationMessage
|
|
type NotifyNewBlockTemplateRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyNewBlockTemplateRequestMessage) Reset() {
|
|
*x = NotifyNewBlockTemplateRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[100]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyNewBlockTemplateRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyNewBlockTemplateRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyNewBlockTemplateRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[100]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyNewBlockTemplateRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyNewBlockTemplateRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{100}
|
|
}
|
|
|
|
type NotifyNewBlockTemplateResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NotifyNewBlockTemplateResponseMessage) Reset() {
|
|
*x = NotifyNewBlockTemplateResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[101]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NotifyNewBlockTemplateResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NotifyNewBlockTemplateResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *NotifyNewBlockTemplateResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[101]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NotifyNewBlockTemplateResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*NotifyNewBlockTemplateResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{101}
|
|
}
|
|
|
|
func (x *NotifyNewBlockTemplateResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// NewBlockTemplateNotificationMessage is sent whenever a new updated block template is
|
|
// available for miners.
|
|
//
|
|
// See NotifyNewBlockTemplateRequestMessage
|
|
type NewBlockTemplateNotificationMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *NewBlockTemplateNotificationMessage) Reset() {
|
|
*x = NewBlockTemplateNotificationMessage{}
|
|
mi := &file_rpc_proto_msgTypes[102]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *NewBlockTemplateNotificationMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NewBlockTemplateNotificationMessage) ProtoMessage() {}
|
|
|
|
func (x *NewBlockTemplateNotificationMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[102]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NewBlockTemplateNotificationMessage.ProtoReflect.Descriptor instead.
|
|
func (*NewBlockTemplateNotificationMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{102}
|
|
}
|
|
|
|
type MempoolEntryByAddress struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Address string `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
Sending []*MempoolEntry `protobuf:"bytes,2,rep,name=sending,proto3" json:"sending,omitempty"`
|
|
Receiving []*MempoolEntry `protobuf:"bytes,3,rep,name=receiving,proto3" json:"receiving,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *MempoolEntryByAddress) Reset() {
|
|
*x = MempoolEntryByAddress{}
|
|
mi := &file_rpc_proto_msgTypes[103]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *MempoolEntryByAddress) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*MempoolEntryByAddress) ProtoMessage() {}
|
|
|
|
func (x *MempoolEntryByAddress) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[103]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use MempoolEntryByAddress.ProtoReflect.Descriptor instead.
|
|
func (*MempoolEntryByAddress) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{103}
|
|
}
|
|
|
|
func (x *MempoolEntryByAddress) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *MempoolEntryByAddress) GetSending() []*MempoolEntry {
|
|
if x != nil {
|
|
return x.Sending
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *MempoolEntryByAddress) GetReceiving() []*MempoolEntry {
|
|
if x != nil {
|
|
return x.Receiving
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetMempoolEntriesByAddressesRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Addresses []string `protobuf:"bytes,1,rep,name=addresses,proto3" json:"addresses,omitempty"`
|
|
IncludeOrphanPool bool `protobuf:"varint,2,opt,name=includeOrphanPool,proto3" json:"includeOrphanPool,omitempty"`
|
|
FilterTransactionPool bool `protobuf:"varint,3,opt,name=filterTransactionPool,proto3" json:"filterTransactionPool,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetMempoolEntriesByAddressesRequestMessage) Reset() {
|
|
*x = GetMempoolEntriesByAddressesRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[104]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetMempoolEntriesByAddressesRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetMempoolEntriesByAddressesRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetMempoolEntriesByAddressesRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[104]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetMempoolEntriesByAddressesRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetMempoolEntriesByAddressesRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{104}
|
|
}
|
|
|
|
func (x *GetMempoolEntriesByAddressesRequestMessage) GetAddresses() []string {
|
|
if x != nil {
|
|
return x.Addresses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetMempoolEntriesByAddressesRequestMessage) GetIncludeOrphanPool() bool {
|
|
if x != nil {
|
|
return x.IncludeOrphanPool
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetMempoolEntriesByAddressesRequestMessage) GetFilterTransactionPool() bool {
|
|
if x != nil {
|
|
return x.FilterTransactionPool
|
|
}
|
|
return false
|
|
}
|
|
|
|
type GetMempoolEntriesByAddressesResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Entries []*MempoolEntryByAddress `protobuf:"bytes,1,rep,name=entries,proto3" json:"entries,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetMempoolEntriesByAddressesResponseMessage) Reset() {
|
|
*x = GetMempoolEntriesByAddressesResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[105]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetMempoolEntriesByAddressesResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetMempoolEntriesByAddressesResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetMempoolEntriesByAddressesResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[105]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetMempoolEntriesByAddressesResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetMempoolEntriesByAddressesResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{105}
|
|
}
|
|
|
|
func (x *GetMempoolEntriesByAddressesResponseMessage) GetEntries() []*MempoolEntryByAddress {
|
|
if x != nil {
|
|
return x.Entries
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetMempoolEntriesByAddressesResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetCoinSupplyRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetCoinSupplyRequestMessage) Reset() {
|
|
*x = GetCoinSupplyRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[106]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetCoinSupplyRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetCoinSupplyRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetCoinSupplyRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[106]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetCoinSupplyRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetCoinSupplyRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{106}
|
|
}
|
|
|
|
type GetCoinSupplyResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
MaxSompi uint64 `protobuf:"varint,1,opt,name=maxSompi,proto3" json:"maxSompi,omitempty"` // note: this is a hard coded maxSupply, actual maxSupply is expected to deviate by upto -5%, but cannot be measured exactly.
|
|
CirculatingSompi uint64 `protobuf:"varint,2,opt,name=circulatingSompi,proto3" json:"circulatingSompi,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetCoinSupplyResponseMessage) Reset() {
|
|
*x = GetCoinSupplyResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[107]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetCoinSupplyResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetCoinSupplyResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetCoinSupplyResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[107]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetCoinSupplyResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetCoinSupplyResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{107}
|
|
}
|
|
|
|
func (x *GetCoinSupplyResponseMessage) GetMaxSompi() uint64 {
|
|
if x != nil {
|
|
return x.MaxSompi
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetCoinSupplyResponseMessage) GetCirculatingSompi() uint64 {
|
|
if x != nil {
|
|
return x.CirculatingSompi
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetCoinSupplyResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type PingRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *PingRequestMessage) Reset() {
|
|
*x = PingRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[108]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *PingRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PingRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *PingRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[108]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PingRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*PingRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{108}
|
|
}
|
|
|
|
type PingResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *PingResponseMessage) Reset() {
|
|
*x = PingResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[109]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *PingResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PingResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *PingResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[109]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PingResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*PingResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{109}
|
|
}
|
|
|
|
func (x *PingResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ProcessMetrics struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
ResidentSetSize uint64 `protobuf:"varint,1,opt,name=residentSetSize,proto3" json:"residentSetSize,omitempty"`
|
|
VirtualMemorySize uint64 `protobuf:"varint,2,opt,name=virtualMemorySize,proto3" json:"virtualMemorySize,omitempty"`
|
|
CoreNum uint32 `protobuf:"varint,3,opt,name=coreNum,proto3" json:"coreNum,omitempty"`
|
|
CpuUsage float32 `protobuf:"fixed32,4,opt,name=cpuUsage,proto3" json:"cpuUsage,omitempty"`
|
|
FdNum uint32 `protobuf:"varint,5,opt,name=fdNum,proto3" json:"fdNum,omitempty"`
|
|
DiskIoReadBytes uint64 `protobuf:"varint,6,opt,name=diskIoReadBytes,proto3" json:"diskIoReadBytes,omitempty"`
|
|
DiskIoWriteBytes uint64 `protobuf:"varint,7,opt,name=diskIoWriteBytes,proto3" json:"diskIoWriteBytes,omitempty"`
|
|
DiskIoReadPerSec float32 `protobuf:"fixed32,8,opt,name=diskIoReadPerSec,proto3" json:"diskIoReadPerSec,omitempty"`
|
|
DiskIoWritePerSec float32 `protobuf:"fixed32,9,opt,name=diskIoWritePerSec,proto3" json:"diskIoWritePerSec,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ProcessMetrics) Reset() {
|
|
*x = ProcessMetrics{}
|
|
mi := &file_rpc_proto_msgTypes[110]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ProcessMetrics) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ProcessMetrics) ProtoMessage() {}
|
|
|
|
func (x *ProcessMetrics) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[110]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ProcessMetrics.ProtoReflect.Descriptor instead.
|
|
func (*ProcessMetrics) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{110}
|
|
}
|
|
|
|
func (x *ProcessMetrics) GetResidentSetSize() uint64 {
|
|
if x != nil {
|
|
return x.ResidentSetSize
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ProcessMetrics) GetVirtualMemorySize() uint64 {
|
|
if x != nil {
|
|
return x.VirtualMemorySize
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ProcessMetrics) GetCoreNum() uint32 {
|
|
if x != nil {
|
|
return x.CoreNum
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ProcessMetrics) GetCpuUsage() float32 {
|
|
if x != nil {
|
|
return x.CpuUsage
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ProcessMetrics) GetFdNum() uint32 {
|
|
if x != nil {
|
|
return x.FdNum
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ProcessMetrics) GetDiskIoReadBytes() uint64 {
|
|
if x != nil {
|
|
return x.DiskIoReadBytes
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ProcessMetrics) GetDiskIoWriteBytes() uint64 {
|
|
if x != nil {
|
|
return x.DiskIoWriteBytes
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ProcessMetrics) GetDiskIoReadPerSec() float32 {
|
|
if x != nil {
|
|
return x.DiskIoReadPerSec
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ProcessMetrics) GetDiskIoWritePerSec() float32 {
|
|
if x != nil {
|
|
return x.DiskIoWritePerSec
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type ConnectionMetrics struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BorshLiveConnections uint32 `protobuf:"varint,31,opt,name=borshLiveConnections,proto3" json:"borshLiveConnections,omitempty"`
|
|
BorshConnectionAttempts uint64 `protobuf:"varint,32,opt,name=borshConnectionAttempts,proto3" json:"borshConnectionAttempts,omitempty"`
|
|
BorshHandshakeFailures uint64 `protobuf:"varint,33,opt,name=borshHandshakeFailures,proto3" json:"borshHandshakeFailures,omitempty"`
|
|
JsonLiveConnections uint32 `protobuf:"varint,41,opt,name=jsonLiveConnections,proto3" json:"jsonLiveConnections,omitempty"`
|
|
JsonConnectionAttempts uint64 `protobuf:"varint,42,opt,name=jsonConnectionAttempts,proto3" json:"jsonConnectionAttempts,omitempty"`
|
|
JsonHandshakeFailures uint64 `protobuf:"varint,43,opt,name=jsonHandshakeFailures,proto3" json:"jsonHandshakeFailures,omitempty"`
|
|
ActivePeers uint32 `protobuf:"varint,51,opt,name=activePeers,proto3" json:"activePeers,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ConnectionMetrics) Reset() {
|
|
*x = ConnectionMetrics{}
|
|
mi := &file_rpc_proto_msgTypes[111]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ConnectionMetrics) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ConnectionMetrics) ProtoMessage() {}
|
|
|
|
func (x *ConnectionMetrics) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[111]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ConnectionMetrics.ProtoReflect.Descriptor instead.
|
|
func (*ConnectionMetrics) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{111}
|
|
}
|
|
|
|
func (x *ConnectionMetrics) GetBorshLiveConnections() uint32 {
|
|
if x != nil {
|
|
return x.BorshLiveConnections
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConnectionMetrics) GetBorshConnectionAttempts() uint64 {
|
|
if x != nil {
|
|
return x.BorshConnectionAttempts
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConnectionMetrics) GetBorshHandshakeFailures() uint64 {
|
|
if x != nil {
|
|
return x.BorshHandshakeFailures
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConnectionMetrics) GetJsonLiveConnections() uint32 {
|
|
if x != nil {
|
|
return x.JsonLiveConnections
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConnectionMetrics) GetJsonConnectionAttempts() uint64 {
|
|
if x != nil {
|
|
return x.JsonConnectionAttempts
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConnectionMetrics) GetJsonHandshakeFailures() uint64 {
|
|
if x != nil {
|
|
return x.JsonHandshakeFailures
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConnectionMetrics) GetActivePeers() uint32 {
|
|
if x != nil {
|
|
return x.ActivePeers
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type BandwidthMetrics struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BorshBytesTx uint64 `protobuf:"varint,61,opt,name=borshBytesTx,proto3" json:"borshBytesTx,omitempty"`
|
|
BorshBytesRx uint64 `protobuf:"varint,62,opt,name=borshBytesRx,proto3" json:"borshBytesRx,omitempty"`
|
|
JsonBytesTx uint64 `protobuf:"varint,63,opt,name=jsonBytesTx,proto3" json:"jsonBytesTx,omitempty"`
|
|
JsonBytesRx uint64 `protobuf:"varint,64,opt,name=jsonBytesRx,proto3" json:"jsonBytesRx,omitempty"`
|
|
GrpcP2PBytesTx uint64 `protobuf:"varint,65,opt,name=grpcP2pBytesTx,proto3" json:"grpcP2pBytesTx,omitempty"`
|
|
GrpcP2PBytesRx uint64 `protobuf:"varint,66,opt,name=grpcP2pBytesRx,proto3" json:"grpcP2pBytesRx,omitempty"`
|
|
GrpcUserBytesTx uint64 `protobuf:"varint,67,opt,name=grpcUserBytesTx,proto3" json:"grpcUserBytesTx,omitempty"`
|
|
GrpcUserBytesRx uint64 `protobuf:"varint,68,opt,name=grpcUserBytesRx,proto3" json:"grpcUserBytesRx,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *BandwidthMetrics) Reset() {
|
|
*x = BandwidthMetrics{}
|
|
mi := &file_rpc_proto_msgTypes[112]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *BandwidthMetrics) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BandwidthMetrics) ProtoMessage() {}
|
|
|
|
func (x *BandwidthMetrics) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[112]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BandwidthMetrics.ProtoReflect.Descriptor instead.
|
|
func (*BandwidthMetrics) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{112}
|
|
}
|
|
|
|
func (x *BandwidthMetrics) GetBorshBytesTx() uint64 {
|
|
if x != nil {
|
|
return x.BorshBytesTx
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BandwidthMetrics) GetBorshBytesRx() uint64 {
|
|
if x != nil {
|
|
return x.BorshBytesRx
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BandwidthMetrics) GetJsonBytesTx() uint64 {
|
|
if x != nil {
|
|
return x.JsonBytesTx
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BandwidthMetrics) GetJsonBytesRx() uint64 {
|
|
if x != nil {
|
|
return x.JsonBytesRx
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BandwidthMetrics) GetGrpcP2PBytesTx() uint64 {
|
|
if x != nil {
|
|
return x.GrpcP2PBytesTx
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BandwidthMetrics) GetGrpcP2PBytesRx() uint64 {
|
|
if x != nil {
|
|
return x.GrpcP2PBytesRx
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BandwidthMetrics) GetGrpcUserBytesTx() uint64 {
|
|
if x != nil {
|
|
return x.GrpcUserBytesTx
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BandwidthMetrics) GetGrpcUserBytesRx() uint64 {
|
|
if x != nil {
|
|
return x.GrpcUserBytesRx
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type ConsensusMetrics struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
BlocksSubmitted uint64 `protobuf:"varint,1,opt,name=blocksSubmitted,proto3" json:"blocksSubmitted,omitempty"`
|
|
HeaderCounts uint64 `protobuf:"varint,2,opt,name=headerCounts,proto3" json:"headerCounts,omitempty"`
|
|
DepCounts uint64 `protobuf:"varint,3,opt,name=depCounts,proto3" json:"depCounts,omitempty"`
|
|
BodyCounts uint64 `protobuf:"varint,4,opt,name=bodyCounts,proto3" json:"bodyCounts,omitempty"`
|
|
TxsCounts uint64 `protobuf:"varint,5,opt,name=txsCounts,proto3" json:"txsCounts,omitempty"`
|
|
ChainBlockCounts uint64 `protobuf:"varint,6,opt,name=chainBlockCounts,proto3" json:"chainBlockCounts,omitempty"`
|
|
MassCounts uint64 `protobuf:"varint,7,opt,name=massCounts,proto3" json:"massCounts,omitempty"`
|
|
BlockCount uint64 `protobuf:"varint,11,opt,name=blockCount,proto3" json:"blockCount,omitempty"`
|
|
HeaderCount uint64 `protobuf:"varint,12,opt,name=headerCount,proto3" json:"headerCount,omitempty"`
|
|
MempoolSize uint64 `protobuf:"varint,13,opt,name=mempoolSize,proto3" json:"mempoolSize,omitempty"`
|
|
TipHashesCount uint32 `protobuf:"varint,14,opt,name=tipHashesCount,proto3" json:"tipHashesCount,omitempty"`
|
|
Difficulty float64 `protobuf:"fixed64,15,opt,name=difficulty,proto3" json:"difficulty,omitempty"`
|
|
PastMedianTime uint64 `protobuf:"varint,16,opt,name=pastMedianTime,proto3" json:"pastMedianTime,omitempty"`
|
|
VirtualParentHashesCount uint32 `protobuf:"varint,17,opt,name=virtualParentHashesCount,proto3" json:"virtualParentHashesCount,omitempty"`
|
|
VirtualDaaScore uint64 `protobuf:"varint,18,opt,name=virtualDaaScore,proto3" json:"virtualDaaScore,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ConsensusMetrics) Reset() {
|
|
*x = ConsensusMetrics{}
|
|
mi := &file_rpc_proto_msgTypes[113]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ConsensusMetrics) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ConsensusMetrics) ProtoMessage() {}
|
|
|
|
func (x *ConsensusMetrics) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[113]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ConsensusMetrics.ProtoReflect.Descriptor instead.
|
|
func (*ConsensusMetrics) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{113}
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetBlocksSubmitted() uint64 {
|
|
if x != nil {
|
|
return x.BlocksSubmitted
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetHeaderCounts() uint64 {
|
|
if x != nil {
|
|
return x.HeaderCounts
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetDepCounts() uint64 {
|
|
if x != nil {
|
|
return x.DepCounts
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetBodyCounts() uint64 {
|
|
if x != nil {
|
|
return x.BodyCounts
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetTxsCounts() uint64 {
|
|
if x != nil {
|
|
return x.TxsCounts
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetChainBlockCounts() uint64 {
|
|
if x != nil {
|
|
return x.ChainBlockCounts
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetMassCounts() uint64 {
|
|
if x != nil {
|
|
return x.MassCounts
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetBlockCount() uint64 {
|
|
if x != nil {
|
|
return x.BlockCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetHeaderCount() uint64 {
|
|
if x != nil {
|
|
return x.HeaderCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetMempoolSize() uint64 {
|
|
if x != nil {
|
|
return x.MempoolSize
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetTipHashesCount() uint32 {
|
|
if x != nil {
|
|
return x.TipHashesCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetDifficulty() float64 {
|
|
if x != nil {
|
|
return x.Difficulty
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetPastMedianTime() uint64 {
|
|
if x != nil {
|
|
return x.PastMedianTime
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetVirtualParentHashesCount() uint32 {
|
|
if x != nil {
|
|
return x.VirtualParentHashesCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConsensusMetrics) GetVirtualDaaScore() uint64 {
|
|
if x != nil {
|
|
return x.VirtualDaaScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type StorageMetrics struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
StorageSizeBytes uint64 `protobuf:"varint,1,opt,name=storageSizeBytes,proto3" json:"storageSizeBytes,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *StorageMetrics) Reset() {
|
|
*x = StorageMetrics{}
|
|
mi := &file_rpc_proto_msgTypes[114]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *StorageMetrics) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StorageMetrics) ProtoMessage() {}
|
|
|
|
func (x *StorageMetrics) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[114]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StorageMetrics.ProtoReflect.Descriptor instead.
|
|
func (*StorageMetrics) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{114}
|
|
}
|
|
|
|
func (x *StorageMetrics) GetStorageSizeBytes() uint64 {
|
|
if x != nil {
|
|
return x.StorageSizeBytes
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type GetConnectionsRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
IncludeProfileData bool `protobuf:"varint,1,opt,name=includeProfileData,proto3" json:"includeProfileData,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetConnectionsRequestMessage) Reset() {
|
|
*x = GetConnectionsRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[115]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetConnectionsRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetConnectionsRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetConnectionsRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[115]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetConnectionsRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetConnectionsRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{115}
|
|
}
|
|
|
|
func (x *GetConnectionsRequestMessage) GetIncludeProfileData() bool {
|
|
if x != nil {
|
|
return x.IncludeProfileData
|
|
}
|
|
return false
|
|
}
|
|
|
|
type ConnectionsProfileData struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
CpuUsage float64 `protobuf:"fixed64,1,opt,name=cpuUsage,proto3" json:"cpuUsage,omitempty"`
|
|
MemoryUsage uint64 `protobuf:"varint,2,opt,name=memoryUsage,proto3" json:"memoryUsage,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *ConnectionsProfileData) Reset() {
|
|
*x = ConnectionsProfileData{}
|
|
mi := &file_rpc_proto_msgTypes[116]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *ConnectionsProfileData) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ConnectionsProfileData) ProtoMessage() {}
|
|
|
|
func (x *ConnectionsProfileData) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[116]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ConnectionsProfileData.ProtoReflect.Descriptor instead.
|
|
func (*ConnectionsProfileData) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{116}
|
|
}
|
|
|
|
func (x *ConnectionsProfileData) GetCpuUsage() float64 {
|
|
if x != nil {
|
|
return x.CpuUsage
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ConnectionsProfileData) GetMemoryUsage() uint64 {
|
|
if x != nil {
|
|
return x.MemoryUsage
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type GetConnectionsResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Clients uint32 `protobuf:"varint,1,opt,name=clients,proto3" json:"clients,omitempty"`
|
|
Peers uint32 `protobuf:"varint,2,opt,name=peers,proto3" json:"peers,omitempty"`
|
|
ProfileData *ConnectionsProfileData `protobuf:"bytes,3,opt,name=profileData,proto3" json:"profileData,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetConnectionsResponseMessage) Reset() {
|
|
*x = GetConnectionsResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[117]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetConnectionsResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetConnectionsResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetConnectionsResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[117]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetConnectionsResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetConnectionsResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{117}
|
|
}
|
|
|
|
func (x *GetConnectionsResponseMessage) GetClients() uint32 {
|
|
if x != nil {
|
|
return x.Clients
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetConnectionsResponseMessage) GetPeers() uint32 {
|
|
if x != nil {
|
|
return x.Peers
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetConnectionsResponseMessage) GetProfileData() *ConnectionsProfileData {
|
|
if x != nil {
|
|
return x.ProfileData
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetConnectionsResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetSystemInfoRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetSystemInfoRequestMessage) Reset() {
|
|
*x = GetSystemInfoRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[118]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetSystemInfoRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetSystemInfoRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetSystemInfoRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[118]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetSystemInfoRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetSystemInfoRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{118}
|
|
}
|
|
|
|
type GetSystemInfoResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Version string `protobuf:"bytes,1,opt,name=version,proto3" json:"version,omitempty"`
|
|
SystemId string `protobuf:"bytes,2,opt,name=systemId,proto3" json:"systemId,omitempty"`
|
|
GitHash string `protobuf:"bytes,3,opt,name=gitHash,proto3" json:"gitHash,omitempty"`
|
|
CoreNum uint32 `protobuf:"varint,4,opt,name=coreNum,proto3" json:"coreNum,omitempty"`
|
|
TotalMemory uint64 `protobuf:"varint,5,opt,name=totalMemory,proto3" json:"totalMemory,omitempty"`
|
|
FdLimit uint32 `protobuf:"varint,6,opt,name=fdLimit,proto3" json:"fdLimit,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetSystemInfoResponseMessage) Reset() {
|
|
*x = GetSystemInfoResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[119]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetSystemInfoResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetSystemInfoResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetSystemInfoResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[119]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetSystemInfoResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetSystemInfoResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{119}
|
|
}
|
|
|
|
func (x *GetSystemInfoResponseMessage) GetVersion() string {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetSystemInfoResponseMessage) GetSystemId() string {
|
|
if x != nil {
|
|
return x.SystemId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetSystemInfoResponseMessage) GetGitHash() string {
|
|
if x != nil {
|
|
return x.GitHash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetSystemInfoResponseMessage) GetCoreNum() uint32 {
|
|
if x != nil {
|
|
return x.CoreNum
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetSystemInfoResponseMessage) GetTotalMemory() uint64 {
|
|
if x != nil {
|
|
return x.TotalMemory
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetSystemInfoResponseMessage) GetFdLimit() uint32 {
|
|
if x != nil {
|
|
return x.FdLimit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetSystemInfoResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetMetricsRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
ProcessMetrics bool `protobuf:"varint,1,opt,name=processMetrics,proto3" json:"processMetrics,omitempty"`
|
|
ConnectionMetrics bool `protobuf:"varint,2,opt,name=connectionMetrics,proto3" json:"connectionMetrics,omitempty"`
|
|
BandwidthMetrics bool `protobuf:"varint,3,opt,name=bandwidthMetrics,proto3" json:"bandwidthMetrics,omitempty"`
|
|
ConsensusMetrics bool `protobuf:"varint,4,opt,name=consensusMetrics,proto3" json:"consensusMetrics,omitempty"`
|
|
StorageMetrics bool `protobuf:"varint,5,opt,name=storageMetrics,proto3" json:"storageMetrics,omitempty"`
|
|
CustomMetrics bool `protobuf:"varint,6,opt,name=customMetrics,proto3" json:"customMetrics,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetMetricsRequestMessage) Reset() {
|
|
*x = GetMetricsRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[120]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetMetricsRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetMetricsRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetMetricsRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[120]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetMetricsRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetMetricsRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{120}
|
|
}
|
|
|
|
func (x *GetMetricsRequestMessage) GetProcessMetrics() bool {
|
|
if x != nil {
|
|
return x.ProcessMetrics
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetMetricsRequestMessage) GetConnectionMetrics() bool {
|
|
if x != nil {
|
|
return x.ConnectionMetrics
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetMetricsRequestMessage) GetBandwidthMetrics() bool {
|
|
if x != nil {
|
|
return x.BandwidthMetrics
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetMetricsRequestMessage) GetConsensusMetrics() bool {
|
|
if x != nil {
|
|
return x.ConsensusMetrics
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetMetricsRequestMessage) GetStorageMetrics() bool {
|
|
if x != nil {
|
|
return x.StorageMetrics
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetMetricsRequestMessage) GetCustomMetrics() bool {
|
|
if x != nil {
|
|
return x.CustomMetrics
|
|
}
|
|
return false
|
|
}
|
|
|
|
type GetMetricsResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
ServerTime uint64 `protobuf:"varint,1,opt,name=serverTime,proto3" json:"serverTime,omitempty"`
|
|
ProcessMetrics *ProcessMetrics `protobuf:"bytes,11,opt,name=processMetrics,proto3" json:"processMetrics,omitempty"`
|
|
ConnectionMetrics *ConnectionMetrics `protobuf:"bytes,12,opt,name=connectionMetrics,proto3" json:"connectionMetrics,omitempty"`
|
|
BandwidthMetrics *BandwidthMetrics `protobuf:"bytes,13,opt,name=bandwidthMetrics,proto3" json:"bandwidthMetrics,omitempty"`
|
|
ConsensusMetrics *ConsensusMetrics `protobuf:"bytes,14,opt,name=consensusMetrics,proto3" json:"consensusMetrics,omitempty"`
|
|
StorageMetrics *StorageMetrics `protobuf:"bytes,15,opt,name=storageMetrics,proto3" json:"storageMetrics,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetMetricsResponseMessage) Reset() {
|
|
*x = GetMetricsResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[121]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetMetricsResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetMetricsResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetMetricsResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[121]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetMetricsResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetMetricsResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{121}
|
|
}
|
|
|
|
func (x *GetMetricsResponseMessage) GetServerTime() uint64 {
|
|
if x != nil {
|
|
return x.ServerTime
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetMetricsResponseMessage) GetProcessMetrics() *ProcessMetrics {
|
|
if x != nil {
|
|
return x.ProcessMetrics
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetMetricsResponseMessage) GetConnectionMetrics() *ConnectionMetrics {
|
|
if x != nil {
|
|
return x.ConnectionMetrics
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetMetricsResponseMessage) GetBandwidthMetrics() *BandwidthMetrics {
|
|
if x != nil {
|
|
return x.BandwidthMetrics
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetMetricsResponseMessage) GetConsensusMetrics() *ConsensusMetrics {
|
|
if x != nil {
|
|
return x.ConsensusMetrics
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetMetricsResponseMessage) GetStorageMetrics() *StorageMetrics {
|
|
if x != nil {
|
|
return x.StorageMetrics
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetMetricsResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetServerInfoRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetServerInfoRequestMessage) Reset() {
|
|
*x = GetServerInfoRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[122]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetServerInfoRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetServerInfoRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetServerInfoRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[122]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetServerInfoRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetServerInfoRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{122}
|
|
}
|
|
|
|
type GetServerInfoResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
RpcApiVersion uint32 `protobuf:"varint,1,opt,name=rpcApiVersion,proto3" json:"rpcApiVersion,omitempty"`
|
|
RpcApiRevision uint32 `protobuf:"varint,2,opt,name=rpcApiRevision,proto3" json:"rpcApiRevision,omitempty"`
|
|
ServerVersion string `protobuf:"bytes,3,opt,name=serverVersion,proto3" json:"serverVersion,omitempty"`
|
|
NetworkId string `protobuf:"bytes,4,opt,name=networkId,proto3" json:"networkId,omitempty"`
|
|
HasUtxoIndex bool `protobuf:"varint,5,opt,name=hasUtxoIndex,proto3" json:"hasUtxoIndex,omitempty"`
|
|
IsSynced bool `protobuf:"varint,6,opt,name=isSynced,proto3" json:"isSynced,omitempty"`
|
|
VirtualDaaScore uint64 `protobuf:"varint,7,opt,name=virtualDaaScore,proto3" json:"virtualDaaScore,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) Reset() {
|
|
*x = GetServerInfoResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[123]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetServerInfoResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetServerInfoResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[123]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetServerInfoResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetServerInfoResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{123}
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) GetRpcApiVersion() uint32 {
|
|
if x != nil {
|
|
return x.RpcApiVersion
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) GetRpcApiRevision() uint32 {
|
|
if x != nil {
|
|
return x.RpcApiRevision
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) GetServerVersion() string {
|
|
if x != nil {
|
|
return x.ServerVersion
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) GetNetworkId() string {
|
|
if x != nil {
|
|
return x.NetworkId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) GetHasUtxoIndex() bool {
|
|
if x != nil {
|
|
return x.HasUtxoIndex
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) GetIsSynced() bool {
|
|
if x != nil {
|
|
return x.IsSynced
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) GetVirtualDaaScore() uint64 {
|
|
if x != nil {
|
|
return x.VirtualDaaScore
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *GetServerInfoResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetSyncStatusRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetSyncStatusRequestMessage) Reset() {
|
|
*x = GetSyncStatusRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[124]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetSyncStatusRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetSyncStatusRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetSyncStatusRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[124]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetSyncStatusRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetSyncStatusRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{124}
|
|
}
|
|
|
|
type GetSyncStatusResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
IsSynced bool `protobuf:"varint,1,opt,name=isSynced,proto3" json:"isSynced,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetSyncStatusResponseMessage) Reset() {
|
|
*x = GetSyncStatusResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[125]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetSyncStatusResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetSyncStatusResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetSyncStatusResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[125]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetSyncStatusResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetSyncStatusResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{125}
|
|
}
|
|
|
|
func (x *GetSyncStatusResponseMessage) GetIsSynced() bool {
|
|
if x != nil {
|
|
return x.IsSynced
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetSyncStatusResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetDaaScoreTimestampEstimateRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
DaaScores []uint64 `protobuf:"varint,1,rep,packed,name=daaScores,proto3" json:"daaScores,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetDaaScoreTimestampEstimateRequestMessage) Reset() {
|
|
*x = GetDaaScoreTimestampEstimateRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[126]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetDaaScoreTimestampEstimateRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetDaaScoreTimestampEstimateRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetDaaScoreTimestampEstimateRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[126]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetDaaScoreTimestampEstimateRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetDaaScoreTimestampEstimateRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{126}
|
|
}
|
|
|
|
func (x *GetDaaScoreTimestampEstimateRequestMessage) GetDaaScores() []uint64 {
|
|
if x != nil {
|
|
return x.DaaScores
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetDaaScoreTimestampEstimateResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Timestamps []uint64 `protobuf:"varint,1,rep,packed,name=timestamps,proto3" json:"timestamps,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetDaaScoreTimestampEstimateResponseMessage) Reset() {
|
|
*x = GetDaaScoreTimestampEstimateResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[127]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetDaaScoreTimestampEstimateResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetDaaScoreTimestampEstimateResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetDaaScoreTimestampEstimateResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[127]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetDaaScoreTimestampEstimateResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetDaaScoreTimestampEstimateResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{127}
|
|
}
|
|
|
|
func (x *GetDaaScoreTimestampEstimateResponseMessage) GetTimestamps() []uint64 {
|
|
if x != nil {
|
|
return x.Timestamps
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetDaaScoreTimestampEstimateResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RpcFeerateBucket struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
// Fee/mass of a transaction in `sompi/gram` units
|
|
Feerate float64 `protobuf:"fixed64,1,opt,name=feerate,proto3" json:"feerate,omitempty"`
|
|
EstimatedSeconds float64 `protobuf:"fixed64,2,opt,name=estimatedSeconds,proto3" json:"estimatedSeconds,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcFeerateBucket) Reset() {
|
|
*x = RpcFeerateBucket{}
|
|
mi := &file_rpc_proto_msgTypes[128]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcFeerateBucket) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcFeerateBucket) ProtoMessage() {}
|
|
|
|
func (x *RpcFeerateBucket) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[128]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcFeerateBucket.ProtoReflect.Descriptor instead.
|
|
func (*RpcFeerateBucket) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{128}
|
|
}
|
|
|
|
func (x *RpcFeerateBucket) GetFeerate() float64 {
|
|
if x != nil {
|
|
return x.Feerate
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcFeerateBucket) GetEstimatedSeconds() float64 {
|
|
if x != nil {
|
|
return x.EstimatedSeconds
|
|
}
|
|
return 0
|
|
}
|
|
|
|
// Data required for making fee estimates.
|
|
//
|
|
// Feerate values represent fee/mass of a transaction in `sompi/gram` units.
|
|
// Given a feerate value recommendation, calculate the required fee by
|
|
// taking the transaction mass and multiplying it by feerate: `fee = feerate *
|
|
// mass(tx)`
|
|
type RpcFeeEstimate struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
// Top-priority feerate bucket. Provides an estimation of the feerate required
|
|
// for sub-second DAG inclusion.
|
|
PriorityBucket *RpcFeerateBucket `protobuf:"bytes,1,opt,name=priority_bucket,json=priorityBucket,proto3" json:"priority_bucket,omitempty"`
|
|
// A vector of *normal* priority feerate values. The first value of this
|
|
// vector is guaranteed to exist and provide an estimation for sub-*minute*
|
|
// DAG inclusion. All other values will have shorter estimation times than all
|
|
// `lowBuckets` values. Therefor by chaining `[priority] | normal | low` and
|
|
// interpolating between them, one can compose a complete feerate function on
|
|
// the client side. The API makes an effort to sample enough "interesting"
|
|
// points on the feerate-to-time curve, so that the interpolation is
|
|
// meaningful.
|
|
NormalBuckets []*RpcFeerateBucket `protobuf:"bytes,2,rep,name=normalBuckets,proto3" json:"normalBuckets,omitempty"`
|
|
// A vector of *low* priority feerate values. The first value of this vector
|
|
// is guaranteed to exist and provide an estimation for sub-*hour* DAG
|
|
// inclusion.
|
|
LowBuckets []*RpcFeerateBucket `protobuf:"bytes,3,rep,name=lowBuckets,proto3" json:"lowBuckets,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcFeeEstimate) Reset() {
|
|
*x = RpcFeeEstimate{}
|
|
mi := &file_rpc_proto_msgTypes[129]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcFeeEstimate) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcFeeEstimate) ProtoMessage() {}
|
|
|
|
func (x *RpcFeeEstimate) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[129]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcFeeEstimate.ProtoReflect.Descriptor instead.
|
|
func (*RpcFeeEstimate) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{129}
|
|
}
|
|
|
|
func (x *RpcFeeEstimate) GetPriorityBucket() *RpcFeerateBucket {
|
|
if x != nil {
|
|
return x.PriorityBucket
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcFeeEstimate) GetNormalBuckets() []*RpcFeerateBucket {
|
|
if x != nil {
|
|
return x.NormalBuckets
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *RpcFeeEstimate) GetLowBuckets() []*RpcFeerateBucket {
|
|
if x != nil {
|
|
return x.LowBuckets
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RpcFeeEstimateVerboseExperimentalData struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
MempoolReadyTransactionsCount uint64 `protobuf:"varint,1,opt,name=mempoolReadyTransactionsCount,proto3" json:"mempoolReadyTransactionsCount,omitempty"`
|
|
MempoolReadyTransactionsTotalMass uint64 `protobuf:"varint,2,opt,name=mempoolReadyTransactionsTotalMass,proto3" json:"mempoolReadyTransactionsTotalMass,omitempty"`
|
|
NetworkMassPerSecond uint64 `protobuf:"varint,3,opt,name=networkMassPerSecond,proto3" json:"networkMassPerSecond,omitempty"`
|
|
NextBlockTemplateFeerateMin float64 `protobuf:"fixed64,11,opt,name=nextBlockTemplateFeerateMin,proto3" json:"nextBlockTemplateFeerateMin,omitempty"`
|
|
NextBlockTemplateFeerateMedian float64 `protobuf:"fixed64,12,opt,name=nextBlockTemplateFeerateMedian,proto3" json:"nextBlockTemplateFeerateMedian,omitempty"`
|
|
NextBlockTemplateFeerateMax float64 `protobuf:"fixed64,13,opt,name=nextBlockTemplateFeerateMax,proto3" json:"nextBlockTemplateFeerateMax,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *RpcFeeEstimateVerboseExperimentalData) Reset() {
|
|
*x = RpcFeeEstimateVerboseExperimentalData{}
|
|
mi := &file_rpc_proto_msgTypes[130]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *RpcFeeEstimateVerboseExperimentalData) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*RpcFeeEstimateVerboseExperimentalData) ProtoMessage() {}
|
|
|
|
func (x *RpcFeeEstimateVerboseExperimentalData) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[130]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use RpcFeeEstimateVerboseExperimentalData.ProtoReflect.Descriptor instead.
|
|
func (*RpcFeeEstimateVerboseExperimentalData) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{130}
|
|
}
|
|
|
|
func (x *RpcFeeEstimateVerboseExperimentalData) GetMempoolReadyTransactionsCount() uint64 {
|
|
if x != nil {
|
|
return x.MempoolReadyTransactionsCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcFeeEstimateVerboseExperimentalData) GetMempoolReadyTransactionsTotalMass() uint64 {
|
|
if x != nil {
|
|
return x.MempoolReadyTransactionsTotalMass
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcFeeEstimateVerboseExperimentalData) GetNetworkMassPerSecond() uint64 {
|
|
if x != nil {
|
|
return x.NetworkMassPerSecond
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcFeeEstimateVerboseExperimentalData) GetNextBlockTemplateFeerateMin() float64 {
|
|
if x != nil {
|
|
return x.NextBlockTemplateFeerateMin
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcFeeEstimateVerboseExperimentalData) GetNextBlockTemplateFeerateMedian() float64 {
|
|
if x != nil {
|
|
return x.NextBlockTemplateFeerateMedian
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *RpcFeeEstimateVerboseExperimentalData) GetNextBlockTemplateFeerateMax() float64 {
|
|
if x != nil {
|
|
return x.NextBlockTemplateFeerateMax
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type GetFeeEstimateRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetFeeEstimateRequestMessage) Reset() {
|
|
*x = GetFeeEstimateRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[131]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetFeeEstimateRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetFeeEstimateRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetFeeEstimateRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[131]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetFeeEstimateRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetFeeEstimateRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{131}
|
|
}
|
|
|
|
type GetFeeEstimateResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Estimate *RpcFeeEstimate `protobuf:"bytes,1,opt,name=estimate,proto3" json:"estimate,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetFeeEstimateResponseMessage) Reset() {
|
|
*x = GetFeeEstimateResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[132]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetFeeEstimateResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetFeeEstimateResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetFeeEstimateResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[132]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetFeeEstimateResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetFeeEstimateResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{132}
|
|
}
|
|
|
|
func (x *GetFeeEstimateResponseMessage) GetEstimate() *RpcFeeEstimate {
|
|
if x != nil {
|
|
return x.Estimate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetFeeEstimateResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetFeeEstimateExperimentalRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Verbose bool `protobuf:"varint,1,opt,name=verbose,proto3" json:"verbose,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetFeeEstimateExperimentalRequestMessage) Reset() {
|
|
*x = GetFeeEstimateExperimentalRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[133]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetFeeEstimateExperimentalRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetFeeEstimateExperimentalRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetFeeEstimateExperimentalRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[133]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetFeeEstimateExperimentalRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetFeeEstimateExperimentalRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{133}
|
|
}
|
|
|
|
func (x *GetFeeEstimateExperimentalRequestMessage) GetVerbose() bool {
|
|
if x != nil {
|
|
return x.Verbose
|
|
}
|
|
return false
|
|
}
|
|
|
|
type GetFeeEstimateExperimentalResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Estimate *RpcFeeEstimate `protobuf:"bytes,1,opt,name=estimate,proto3" json:"estimate,omitempty"`
|
|
Verbose *RpcFeeEstimateVerboseExperimentalData `protobuf:"bytes,2,opt,name=verbose,proto3" json:"verbose,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetFeeEstimateExperimentalResponseMessage) Reset() {
|
|
*x = GetFeeEstimateExperimentalResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[134]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetFeeEstimateExperimentalResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetFeeEstimateExperimentalResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetFeeEstimateExperimentalResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[134]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetFeeEstimateExperimentalResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetFeeEstimateExperimentalResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{134}
|
|
}
|
|
|
|
func (x *GetFeeEstimateExperimentalResponseMessage) GetEstimate() *RpcFeeEstimate {
|
|
if x != nil {
|
|
return x.Estimate
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetFeeEstimateExperimentalResponseMessage) GetVerbose() *RpcFeeEstimateVerboseExperimentalData {
|
|
if x != nil {
|
|
return x.Verbose
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *GetFeeEstimateExperimentalResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type GetCurrentBlockColorRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Hash string `protobuf:"bytes,1,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetCurrentBlockColorRequestMessage) Reset() {
|
|
*x = GetCurrentBlockColorRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[135]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetCurrentBlockColorRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetCurrentBlockColorRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *GetCurrentBlockColorRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[135]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetCurrentBlockColorRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetCurrentBlockColorRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{135}
|
|
}
|
|
|
|
func (x *GetCurrentBlockColorRequestMessage) GetHash() string {
|
|
if x != nil {
|
|
return x.Hash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type GetCurrentBlockColorResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Blue bool `protobuf:"varint,1,opt,name=blue,proto3" json:"blue,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *GetCurrentBlockColorResponseMessage) Reset() {
|
|
*x = GetCurrentBlockColorResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[136]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *GetCurrentBlockColorResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetCurrentBlockColorResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *GetCurrentBlockColorResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[136]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetCurrentBlockColorResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*GetCurrentBlockColorResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{136}
|
|
}
|
|
|
|
func (x *GetCurrentBlockColorResponseMessage) GetBlue() bool {
|
|
if x != nil {
|
|
return x.Blue
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *GetCurrentBlockColorResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// SubmitTransactionReplacementRequestMessage submits a transaction to the
|
|
// mempool, applying a mandatory Replace by Fee policy
|
|
type SubmitTransactionReplacementRequestMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
Transaction *RpcTransaction `protobuf:"bytes,1,opt,name=transaction,proto3" json:"transaction,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *SubmitTransactionReplacementRequestMessage) Reset() {
|
|
*x = SubmitTransactionReplacementRequestMessage{}
|
|
mi := &file_rpc_proto_msgTypes[137]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *SubmitTransactionReplacementRequestMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubmitTransactionReplacementRequestMessage) ProtoMessage() {}
|
|
|
|
func (x *SubmitTransactionReplacementRequestMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[137]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubmitTransactionReplacementRequestMessage.ProtoReflect.Descriptor instead.
|
|
func (*SubmitTransactionReplacementRequestMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{137}
|
|
}
|
|
|
|
func (x *SubmitTransactionReplacementRequestMessage) GetTransaction() *RpcTransaction {
|
|
if x != nil {
|
|
return x.Transaction
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type SubmitTransactionReplacementResponseMessage struct {
|
|
state protoimpl.MessageState `protogen:"open.v1"`
|
|
// The transaction ID of the submitted transaction
|
|
TransactionId string `protobuf:"bytes,1,opt,name=transactionId,proto3" json:"transactionId,omitempty"`
|
|
// The previous transaction replaced in the mempool by the newly submitted one
|
|
ReplacedTransaction *RpcTransaction `protobuf:"bytes,2,opt,name=replacedTransaction,proto3" json:"replacedTransaction,omitempty"`
|
|
Error *RPCError `protobuf:"bytes,1000,opt,name=error,proto3" json:"error,omitempty"`
|
|
unknownFields protoimpl.UnknownFields
|
|
sizeCache protoimpl.SizeCache
|
|
}
|
|
|
|
func (x *SubmitTransactionReplacementResponseMessage) Reset() {
|
|
*x = SubmitTransactionReplacementResponseMessage{}
|
|
mi := &file_rpc_proto_msgTypes[138]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
|
|
func (x *SubmitTransactionReplacementResponseMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubmitTransactionReplacementResponseMessage) ProtoMessage() {}
|
|
|
|
func (x *SubmitTransactionReplacementResponseMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_rpc_proto_msgTypes[138]
|
|
if x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubmitTransactionReplacementResponseMessage.ProtoReflect.Descriptor instead.
|
|
func (*SubmitTransactionReplacementResponseMessage) Descriptor() ([]byte, []int) {
|
|
return file_rpc_proto_rawDescGZIP(), []int{138}
|
|
}
|
|
|
|
func (x *SubmitTransactionReplacementResponseMessage) GetTransactionId() string {
|
|
if x != nil {
|
|
return x.TransactionId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *SubmitTransactionReplacementResponseMessage) GetReplacedTransaction() *RpcTransaction {
|
|
if x != nil {
|
|
return x.ReplacedTransaction
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SubmitTransactionReplacementResponseMessage) GetError() *RPCError {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
var File_rpc_proto protoreflect.FileDescriptor
|
|
|
|
var file_rpc_proto_rawDesc = []byte{
|
|
0x0a, 0x09, 0x72, 0x70, 0x63, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x12, 0x09, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x22, 0x24, 0x0a, 0x08, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72,
|
|
0x6f, 0x72, 0x12, 0x18, 0x0a, 0x07, 0x6d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x07, 0x6d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0xbe, 0x01, 0x0a,
|
|
0x08, 0x52, 0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x12, 0x31, 0x0a, 0x06, 0x68, 0x65, 0x61,
|
|
0x64, 0x65, 0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65,
|
|
0x61, 0x64, 0x65, 0x72, 0x52, 0x06, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x12, 0x3d, 0x0a, 0x0c,
|
|
0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x02, 0x20, 0x03,
|
|
0x28, 0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52,
|
|
0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x0c, 0x74,
|
|
0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x40, 0x0a, 0x0b, 0x76,
|
|
0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x44, 0x61, 0x74, 0x61, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x1e, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63,
|
|
0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x56, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x44, 0x61, 0x74, 0x61,
|
|
0x52, 0x0b, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x44, 0x61, 0x74, 0x61, 0x22, 0xab, 0x03,
|
|
0x0a, 0x0e, 0x52, 0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72,
|
|
0x12, 0x18, 0x0a, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x0d, 0x52, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x39, 0x0a, 0x07, 0x70, 0x61,
|
|
0x72, 0x65, 0x6e, 0x74, 0x73, 0x18, 0x0c, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1f, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x4c, 0x65, 0x76, 0x65, 0x6c, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x73, 0x52, 0x07, 0x70, 0x61,
|
|
0x72, 0x65, 0x6e, 0x74, 0x73, 0x12, 0x26, 0x0a, 0x0e, 0x68, 0x61, 0x73, 0x68, 0x4d, 0x65, 0x72,
|
|
0x6b, 0x6c, 0x65, 0x52, 0x6f, 0x6f, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0e, 0x68,
|
|
0x61, 0x73, 0x68, 0x4d, 0x65, 0x72, 0x6b, 0x6c, 0x65, 0x52, 0x6f, 0x6f, 0x74, 0x12, 0x32, 0x0a,
|
|
0x14, 0x61, 0x63, 0x63, 0x65, 0x70, 0x74, 0x65, 0x64, 0x49, 0x64, 0x4d, 0x65, 0x72, 0x6b, 0x6c,
|
|
0x65, 0x52, 0x6f, 0x6f, 0x74, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x14, 0x61, 0x63, 0x63,
|
|
0x65, 0x70, 0x74, 0x65, 0x64, 0x49, 0x64, 0x4d, 0x65, 0x72, 0x6b, 0x6c, 0x65, 0x52, 0x6f, 0x6f,
|
|
0x74, 0x12, 0x26, 0x0a, 0x0e, 0x75, 0x74, 0x78, 0x6f, 0x43, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x6d,
|
|
0x65, 0x6e, 0x74, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0e, 0x75, 0x74, 0x78, 0x6f, 0x43,
|
|
0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x6d, 0x65, 0x6e, 0x74, 0x12, 0x1c, 0x0a, 0x09, 0x74, 0x69, 0x6d,
|
|
0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x18, 0x06, 0x20, 0x01, 0x28, 0x03, 0x52, 0x09, 0x74, 0x69,
|
|
0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x12, 0x12, 0x0a, 0x04, 0x62, 0x69, 0x74, 0x73, 0x18,
|
|
0x07, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x04, 0x62, 0x69, 0x74, 0x73, 0x12, 0x14, 0x0a, 0x05, 0x6e,
|
|
0x6f, 0x6e, 0x63, 0x65, 0x18, 0x08, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05, 0x6e, 0x6f, 0x6e, 0x63,
|
|
0x65, 0x12, 0x1a, 0x0a, 0x08, 0x64, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x09, 0x20,
|
|
0x01, 0x28, 0x04, 0x52, 0x08, 0x64, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x1a, 0x0a,
|
|
0x08, 0x62, 0x6c, 0x75, 0x65, 0x57, 0x6f, 0x72, 0x6b, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x08, 0x62, 0x6c, 0x75, 0x65, 0x57, 0x6f, 0x72, 0x6b, 0x12, 0x22, 0x0a, 0x0c, 0x70, 0x72, 0x75,
|
|
0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x18, 0x0e, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x0c, 0x70, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x12, 0x1c, 0x0a,
|
|
0x09, 0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x0d, 0x20, 0x01, 0x28, 0x04,
|
|
0x52, 0x09, 0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x22, 0x3a, 0x0a, 0x14, 0x52,
|
|
0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x4c, 0x65, 0x76, 0x65, 0x6c, 0x50, 0x61, 0x72, 0x65,
|
|
0x6e, 0x74, 0x73, 0x12, 0x22, 0x0a, 0x0c, 0x70, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x48, 0x61, 0x73,
|
|
0x68, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0c, 0x70, 0x61, 0x72, 0x65, 0x6e,
|
|
0x74, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x22, 0x91, 0x03, 0x0a, 0x13, 0x52, 0x70, 0x63, 0x42,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x56, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x44, 0x61, 0x74, 0x61, 0x12,
|
|
0x12, 0x0a, 0x04, 0x68, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x68,
|
|
0x61, 0x73, 0x68, 0x12, 0x1e, 0x0a, 0x0a, 0x64, 0x69, 0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74,
|
|
0x79, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x01, 0x52, 0x0a, 0x64, 0x69, 0x66, 0x66, 0x69, 0x63, 0x75,
|
|
0x6c, 0x74, 0x79, 0x12, 0x2e, 0x0a, 0x12, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50,
|
|
0x61, 0x72, 0x65, 0x6e, 0x74, 0x48, 0x61, 0x73, 0x68, 0x18, 0x0d, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x12, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x48,
|
|
0x61, 0x73, 0x68, 0x12, 0x26, 0x0a, 0x0e, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x49, 0x64, 0x73, 0x18, 0x0e, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0e, 0x74, 0x72, 0x61,
|
|
0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x73, 0x12, 0x22, 0x0a, 0x0c, 0x69,
|
|
0x73, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x4f, 0x6e, 0x6c, 0x79, 0x18, 0x0f, 0x20, 0x01, 0x28,
|
|
0x08, 0x52, 0x0c, 0x69, 0x73, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x4f, 0x6e, 0x6c, 0x79, 0x12,
|
|
0x1c, 0x0a, 0x09, 0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x10, 0x20, 0x01,
|
|
0x28, 0x04, 0x52, 0x09, 0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x26, 0x0a,
|
|
0x0e, 0x63, 0x68, 0x69, 0x6c, 0x64, 0x72, 0x65, 0x6e, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18,
|
|
0x11, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0e, 0x63, 0x68, 0x69, 0x6c, 0x64, 0x72, 0x65, 0x6e, 0x48,
|
|
0x61, 0x73, 0x68, 0x65, 0x73, 0x12, 0x30, 0x0a, 0x13, 0x6d, 0x65, 0x72, 0x67, 0x65, 0x53, 0x65,
|
|
0x74, 0x42, 0x6c, 0x75, 0x65, 0x73, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18, 0x12, 0x20, 0x03,
|
|
0x28, 0x09, 0x52, 0x13, 0x6d, 0x65, 0x72, 0x67, 0x65, 0x53, 0x65, 0x74, 0x42, 0x6c, 0x75, 0x65,
|
|
0x73, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x12, 0x2e, 0x0a, 0x12, 0x6d, 0x65, 0x72, 0x67, 0x65,
|
|
0x53, 0x65, 0x74, 0x52, 0x65, 0x64, 0x73, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18, 0x13, 0x20,
|
|
0x03, 0x28, 0x09, 0x52, 0x12, 0x6d, 0x65, 0x72, 0x67, 0x65, 0x53, 0x65, 0x74, 0x52, 0x65, 0x64,
|
|
0x73, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x12, 0x22, 0x0a, 0x0c, 0x69, 0x73, 0x43, 0x68, 0x61,
|
|
0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x18, 0x14, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0c, 0x69,
|
|
0x73, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x22, 0xe5, 0x02, 0x0a, 0x0e,
|
|
0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x18,
|
|
0x0a, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52,
|
|
0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x36, 0x0a, 0x06, 0x69, 0x6e, 0x70, 0x75,
|
|
0x74, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1e, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x52, 0x06, 0x69, 0x6e, 0x70, 0x75, 0x74, 0x73,
|
|
0x12, 0x39, 0x0a, 0x07, 0x6f, 0x75, 0x74, 0x70, 0x75, 0x74, 0x73, 0x18, 0x03, 0x20, 0x03, 0x28,
|
|
0x0b, 0x32, 0x1f, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70,
|
|
0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4f, 0x75, 0x74, 0x70,
|
|
0x75, 0x74, 0x52, 0x07, 0x6f, 0x75, 0x74, 0x70, 0x75, 0x74, 0x73, 0x12, 0x1a, 0x0a, 0x08, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x54, 0x69, 0x6d, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x54, 0x69, 0x6d, 0x65, 0x12, 0x22, 0x0a, 0x0c, 0x73, 0x75, 0x62, 0x6e, 0x65,
|
|
0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0c, 0x73,
|
|
0x75, 0x62, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x12, 0x10, 0x0a, 0x03, 0x67,
|
|
0x61, 0x73, 0x18, 0x06, 0x20, 0x01, 0x28, 0x04, 0x52, 0x03, 0x67, 0x61, 0x73, 0x12, 0x18, 0x0a,
|
|
0x07, 0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x18, 0x08, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07,
|
|
0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x12, 0x46, 0x0a, 0x0b, 0x76, 0x65, 0x72, 0x62, 0x6f,
|
|
0x73, 0x65, 0x44, 0x61, 0x74, 0x61, 0x18, 0x09, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x24, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e,
|
|
0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x56, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x44, 0x61,
|
|
0x74, 0x61, 0x52, 0x0b, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x44, 0x61, 0x74, 0x61, 0x12,
|
|
0x12, 0x0a, 0x04, 0x6d, 0x61, 0x73, 0x73, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x04, 0x52, 0x04, 0x6d,
|
|
0x61, 0x73, 0x73, 0x22, 0x8c, 0x02, 0x0a, 0x13, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73,
|
|
0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x12, 0x42, 0x0a, 0x10, 0x70,
|
|
0x72, 0x65, 0x76, 0x69, 0x6f, 0x75, 0x73, 0x4f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x16, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x52, 0x70, 0x63, 0x4f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x52, 0x10, 0x70,
|
|
0x72, 0x65, 0x76, 0x69, 0x6f, 0x75, 0x73, 0x4f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x12,
|
|
0x28, 0x0a, 0x0f, 0x73, 0x69, 0x67, 0x6e, 0x61, 0x74, 0x75, 0x72, 0x65, 0x53, 0x63, 0x72, 0x69,
|
|
0x70, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0f, 0x73, 0x69, 0x67, 0x6e, 0x61, 0x74,
|
|
0x75, 0x72, 0x65, 0x53, 0x63, 0x72, 0x69, 0x70, 0x74, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65, 0x71,
|
|
0x75, 0x65, 0x6e, 0x63, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x73, 0x65, 0x71,
|
|
0x75, 0x65, 0x6e, 0x63, 0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x73, 0x69, 0x67, 0x4f, 0x70, 0x43, 0x6f,
|
|
0x75, 0x6e, 0x74, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0a, 0x73, 0x69, 0x67, 0x4f, 0x70,
|
|
0x43, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x4b, 0x0a, 0x0b, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65,
|
|
0x44, 0x61, 0x74, 0x61, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x29, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61,
|
|
0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x56, 0x65, 0x72, 0x62, 0x6f, 0x73,
|
|
0x65, 0x44, 0x61, 0x74, 0x61, 0x52, 0x0b, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x44, 0x61,
|
|
0x74, 0x61, 0x22, 0x58, 0x0a, 0x12, 0x52, 0x70, 0x63, 0x53, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50,
|
|
0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x12, 0x18, 0x0a, 0x07, 0x76, 0x65, 0x72, 0x73,
|
|
0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69,
|
|
0x6f, 0x6e, 0x12, 0x28, 0x0a, 0x0f, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c,
|
|
0x69, 0x63, 0x4b, 0x65, 0x79, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0f, 0x73, 0x63, 0x72,
|
|
0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x22, 0xc5, 0x01, 0x0a,
|
|
0x14, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4f,
|
|
0x75, 0x74, 0x70, 0x75, 0x74, 0x12, 0x16, 0x0a, 0x06, 0x61, 0x6d, 0x6f, 0x75, 0x6e, 0x74, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x06, 0x61, 0x6d, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x47, 0x0a,
|
|
0x0f, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1d, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x53, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c,
|
|
0x69, 0x63, 0x4b, 0x65, 0x79, 0x52, 0x0f, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62,
|
|
0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x12, 0x4c, 0x0a, 0x0b, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73,
|
|
0x65, 0x44, 0x61, 0x74, 0x61, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x2a, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73,
|
|
0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4f, 0x75, 0x74, 0x70, 0x75, 0x74, 0x56, 0x65, 0x72, 0x62,
|
|
0x6f, 0x73, 0x65, 0x44, 0x61, 0x74, 0x61, 0x52, 0x0b, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65,
|
|
0x44, 0x61, 0x74, 0x61, 0x22, 0x49, 0x0a, 0x0b, 0x52, 0x70, 0x63, 0x4f, 0x75, 0x74, 0x70, 0x6f,
|
|
0x69, 0x6e, 0x74, 0x12, 0x24, 0x0a, 0x0d, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x49, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0d, 0x74, 0x72, 0x61, 0x6e,
|
|
0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x12, 0x14, 0x0a, 0x05, 0x69, 0x6e, 0x64,
|
|
0x65, 0x78, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x05, 0x69, 0x6e, 0x64, 0x65, 0x78, 0x22,
|
|
0xb5, 0x01, 0x0a, 0x0c, 0x52, 0x70, 0x63, 0x55, 0x74, 0x78, 0x6f, 0x45, 0x6e, 0x74, 0x72, 0x79,
|
|
0x12, 0x16, 0x0a, 0x06, 0x61, 0x6d, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04,
|
|
0x52, 0x06, 0x61, 0x6d, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x47, 0x0a, 0x0f, 0x73, 0x63, 0x72, 0x69,
|
|
0x70, 0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x18, 0x02, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1d, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70,
|
|
0x63, 0x53, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79,
|
|
0x52, 0x0f, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65,
|
|
0x79, 0x12, 0x24, 0x0a, 0x0d, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f,
|
|
0x72, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0d, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x44,
|
|
0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x69, 0x73, 0x43, 0x6f, 0x69,
|
|
0x6e, 0x62, 0x61, 0x73, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0a, 0x69, 0x73, 0x43,
|
|
0x6f, 0x69, 0x6e, 0x62, 0x61, 0x73, 0x65, 0x22, 0xa5, 0x01, 0x0a, 0x19, 0x52, 0x70, 0x63, 0x54,
|
|
0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x56, 0x65, 0x72, 0x62, 0x6f, 0x73,
|
|
0x65, 0x44, 0x61, 0x74, 0x61, 0x12, 0x24, 0x0a, 0x0d, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0d, 0x74, 0x72,
|
|
0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x12, 0x12, 0x0a, 0x04, 0x68,
|
|
0x61, 0x73, 0x68, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x68, 0x61, 0x73, 0x68, 0x12,
|
|
0x12, 0x0a, 0x04, 0x6d, 0x61, 0x73, 0x73, 0x18, 0x04, 0x20, 0x01, 0x28, 0x04, 0x52, 0x04, 0x6d,
|
|
0x61, 0x73, 0x73, 0x12, 0x1c, 0x0a, 0x09, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68,
|
|
0x18, 0x0c, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73,
|
|
0x68, 0x12, 0x1c, 0x0a, 0x09, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x69, 0x6d, 0x65, 0x18, 0x0e,
|
|
0x20, 0x01, 0x28, 0x04, 0x52, 0x09, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x69, 0x6d, 0x65, 0x22,
|
|
0x20, 0x0a, 0x1e, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x56, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x44, 0x61, 0x74,
|
|
0x61, 0x22, 0x8b, 0x01, 0x0a, 0x1f, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x4f, 0x75, 0x74, 0x70, 0x75, 0x74, 0x56, 0x65, 0x72, 0x62, 0x6f, 0x73,
|
|
0x65, 0x44, 0x61, 0x74, 0x61, 0x12, 0x30, 0x0a, 0x13, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50,
|
|
0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x54, 0x79, 0x70, 0x65, 0x18, 0x05, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x13, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63,
|
|
0x4b, 0x65, 0x79, 0x54, 0x79, 0x70, 0x65, 0x12, 0x36, 0x0a, 0x16, 0x73, 0x63, 0x72, 0x69, 0x70,
|
|
0x74, 0x50, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73,
|
|
0x73, 0x18, 0x06, 0x20, 0x01, 0x28, 0x09, 0x52, 0x16, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x50,
|
|
0x75, 0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x22,
|
|
0x21, 0x0a, 0x1f, 0x47, 0x65, 0x74, 0x43, 0x75, 0x72, 0x72, 0x65, 0x6e, 0x74, 0x4e, 0x65, 0x74,
|
|
0x77, 0x6f, 0x72, 0x6b, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61,
|
|
0x67, 0x65, 0x22, 0x76, 0x0a, 0x20, 0x47, 0x65, 0x74, 0x43, 0x75, 0x72, 0x72, 0x65, 0x6e, 0x74,
|
|
0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x26, 0x0a, 0x0e, 0x63, 0x75, 0x72, 0x72, 0x65, 0x6e,
|
|
0x74, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0e,
|
|
0x63, 0x75, 0x72, 0x72, 0x65, 0x6e, 0x74, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x12, 0x2a,
|
|
0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72,
|
|
0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x74, 0x0a, 0x19, 0x53, 0x75,
|
|
0x62, 0x6d, 0x69, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x29, 0x0a, 0x05, 0x62, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x05, 0x62, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x12, 0x2c, 0x0a, 0x11, 0x61, 0x6c, 0x6c, 0x6f, 0x77, 0x4e, 0x6f, 0x6e, 0x44, 0x41,
|
|
0x41, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x11, 0x61,
|
|
0x6c, 0x6c, 0x6f, 0x77, 0x4e, 0x6f, 0x6e, 0x44, 0x41, 0x41, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x73,
|
|
0x22, 0xdc, 0x01, 0x0a, 0x1a, 0x53, 0x75, 0x62, 0x6d, 0x69, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x56, 0x0a, 0x0c, 0x72, 0x65, 0x6a, 0x65, 0x63, 0x74, 0x52, 0x65, 0x61, 0x73, 0x6f, 0x6e, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x32, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x53, 0x75, 0x62, 0x6d, 0x69, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x2e, 0x52, 0x65, 0x6a,
|
|
0x65, 0x63, 0x74, 0x52, 0x65, 0x61, 0x73, 0x6f, 0x6e, 0x52, 0x0c, 0x72, 0x65, 0x6a, 0x65, 0x63,
|
|
0x74, 0x52, 0x65, 0x61, 0x73, 0x6f, 0x6e, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72,
|
|
0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72,
|
|
0x72, 0x6f, 0x72, 0x22, 0x3a, 0x0a, 0x0c, 0x52, 0x65, 0x6a, 0x65, 0x63, 0x74, 0x52, 0x65, 0x61,
|
|
0x73, 0x6f, 0x6e, 0x12, 0x08, 0x0a, 0x04, 0x4e, 0x4f, 0x4e, 0x45, 0x10, 0x00, 0x12, 0x11, 0x0a,
|
|
0x0d, 0x42, 0x4c, 0x4f, 0x43, 0x4b, 0x5f, 0x49, 0x4e, 0x56, 0x41, 0x4c, 0x49, 0x44, 0x10, 0x01,
|
|
0x12, 0x0d, 0x0a, 0x09, 0x49, 0x53, 0x5f, 0x49, 0x4e, 0x5f, 0x49, 0x42, 0x44, 0x10, 0x02, 0x22,
|
|
0x5e, 0x0a, 0x1e, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x65, 0x6d, 0x70, 0x6c,
|
|
0x61, 0x74, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x70, 0x61, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0a, 0x70, 0x61, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73,
|
|
0x73, 0x12, 0x1c, 0x0a, 0x09, 0x65, 0x78, 0x74, 0x72, 0x61, 0x44, 0x61, 0x74, 0x61, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x65, 0x78, 0x74, 0x72, 0x61, 0x44, 0x61, 0x74, 0x61, 0x22,
|
|
0x94, 0x01, 0x0a, 0x1f, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x65, 0x6d, 0x70,
|
|
0x6c, 0x61, 0x74, 0x65, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x12, 0x29, 0x0a, 0x05, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x18, 0x03, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52,
|
|
0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x05, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x12, 0x1a,
|
|
0x0a, 0x08, 0x69, 0x73, 0x53, 0x79, 0x6e, 0x63, 0x65, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08,
|
|
0x52, 0x08, 0x69, 0x73, 0x53, 0x79, 0x6e, 0x63, 0x65, 0x64, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72,
|
|
0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52,
|
|
0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x20, 0x0a, 0x1e, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79,
|
|
0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x41, 0x64, 0x64, 0x65, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
|
0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x4d, 0x0a, 0x1f, 0x4e, 0x6f, 0x74, 0x69,
|
|
0x66, 0x79, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x41, 0x64, 0x64, 0x65, 0x64, 0x52, 0x65, 0x73, 0x70,
|
|
0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65,
|
|
0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72,
|
|
0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x4a, 0x0a, 0x1d, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x41, 0x64, 0x64, 0x65, 0x64, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x29, 0x0a, 0x05, 0x62, 0x6c, 0x6f, 0x63,
|
|
0x6b, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x05, 0x62, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x22, 0x20, 0x0a, 0x1e, 0x47, 0x65, 0x74, 0x50, 0x65, 0x65, 0x72, 0x41, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0xf5, 0x01, 0x0a, 0x1f, 0x47, 0x65, 0x74, 0x50, 0x65, 0x65,
|
|
0x72, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e,
|
|
0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x4c, 0x0a, 0x09, 0x61, 0x64, 0x64,
|
|
0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x2e, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x47, 0x65, 0x74, 0x50, 0x65, 0x65, 0x72,
|
|
0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x4b, 0x6e, 0x6f, 0x77, 0x6e, 0x41, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x52, 0x09, 0x61, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x12, 0x58, 0x0a, 0x0f, 0x62, 0x61, 0x6e, 0x6e, 0x65,
|
|
0x64, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b,
|
|
0x32, 0x2e, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x47, 0x65, 0x74,
|
|
0x50, 0x65, 0x65, 0x72, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x4b, 0x6e, 0x6f,
|
|
0x77, 0x6e, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x52, 0x0f, 0x62, 0x61, 0x6e, 0x6e, 0x65, 0x64, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65,
|
|
0x73, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50,
|
|
0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x39, 0x0a,
|
|
0x23, 0x47, 0x65, 0x74, 0x50, 0x65, 0x65, 0x72, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65,
|
|
0x73, 0x4b, 0x6e, 0x6f, 0x77, 0x6e, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x4d, 0x65, 0x73,
|
|
0x73, 0x61, 0x67, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x41, 0x64, 0x64, 0x72, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x04, 0x41, 0x64, 0x64, 0x72, 0x22, 0x22, 0x0a, 0x20, 0x47, 0x65, 0x74, 0x53,
|
|
0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x54, 0x69, 0x70, 0x48, 0x61, 0x73, 0x68, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x79, 0x0a, 0x21,
|
|
0x47, 0x65, 0x74, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x54, 0x69, 0x70, 0x48, 0x61,
|
|
0x73, 0x68, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x28, 0x0a, 0x0f, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x54, 0x69, 0x70,
|
|
0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0f, 0x73, 0x65, 0x6c, 0x65,
|
|
0x63, 0x74, 0x65, 0x64, 0x54, 0x69, 0x70, 0x48, 0x61, 0x73, 0x68, 0x12, 0x2a, 0x0a, 0x05, 0x65,
|
|
0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72,
|
|
0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x97, 0x01, 0x0a, 0x1d, 0x47, 0x65, 0x74, 0x4d,
|
|
0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x74, 0x78, 0x49,
|
|
0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x74, 0x78, 0x49, 0x64, 0x12, 0x2c, 0x0a,
|
|
0x11, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x4f, 0x72, 0x70, 0x68, 0x61, 0x6e, 0x50, 0x6f,
|
|
0x6f, 0x6c, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x11, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64,
|
|
0x65, 0x4f, 0x72, 0x70, 0x68, 0x61, 0x6e, 0x50, 0x6f, 0x6f, 0x6c, 0x12, 0x34, 0x0a, 0x15, 0x66,
|
|
0x69, 0x6c, 0x74, 0x65, 0x72, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x50, 0x6f, 0x6f, 0x6c, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x15, 0x66, 0x69, 0x6c, 0x74,
|
|
0x65, 0x72, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x50, 0x6f, 0x6f,
|
|
0x6c, 0x22, 0x7b, 0x0a, 0x1e, 0x47, 0x65, 0x74, 0x4d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45,
|
|
0x6e, 0x74, 0x72, 0x79, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x12, 0x2d, 0x0a, 0x05, 0x65, 0x6e, 0x74, 0x72, 0x79, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x4d,
|
|
0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x05, 0x65, 0x6e, 0x74,
|
|
0x72, 0x79, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52,
|
|
0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x85,
|
|
0x01, 0x0a, 0x1f, 0x47, 0x65, 0x74, 0x4d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45, 0x6e, 0x74,
|
|
0x72, 0x69, 0x65, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61,
|
|
0x67, 0x65, 0x12, 0x2c, 0x0a, 0x11, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x4f, 0x72, 0x70,
|
|
0x68, 0x61, 0x6e, 0x50, 0x6f, 0x6f, 0x6c, 0x18, 0x01, 0x20, 0x01, 0x28, 0x08, 0x52, 0x11, 0x69,
|
|
0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x4f, 0x72, 0x70, 0x68, 0x61, 0x6e, 0x50, 0x6f, 0x6f, 0x6c,
|
|
0x12, 0x34, 0x0a, 0x15, 0x66, 0x69, 0x6c, 0x74, 0x65, 0x72, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61,
|
|
0x63, 0x74, 0x69, 0x6f, 0x6e, 0x50, 0x6f, 0x6f, 0x6c, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52,
|
|
0x15, 0x66, 0x69, 0x6c, 0x74, 0x65, 0x72, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x50, 0x6f, 0x6f, 0x6c, 0x22, 0x81, 0x01, 0x0a, 0x20, 0x47, 0x65, 0x74, 0x4d, 0x65,
|
|
0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45, 0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x52, 0x65, 0x73, 0x70,
|
|
0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x31, 0x0a, 0x07, 0x65,
|
|
0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x4d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x07, 0x65, 0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x12, 0x2a,
|
|
0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72,
|
|
0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x79, 0x0a, 0x0c, 0x4d, 0x65,
|
|
0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x66, 0x65,
|
|
0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x03, 0x66, 0x65, 0x65, 0x12, 0x3b, 0x0a, 0x0b,
|
|
0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x03, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70,
|
|
0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x0b, 0x74, 0x72,
|
|
0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x1a, 0x0a, 0x08, 0x69, 0x73, 0x4f,
|
|
0x72, 0x70, 0x68, 0x61, 0x6e, 0x18, 0x04, 0x20, 0x01, 0x28, 0x08, 0x52, 0x08, 0x69, 0x73, 0x4f,
|
|
0x72, 0x70, 0x68, 0x61, 0x6e, 0x22, 0x24, 0x0a, 0x22, 0x47, 0x65, 0x74, 0x43, 0x6f, 0x6e, 0x6e,
|
|
0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71,
|
|
0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x8f, 0x01, 0x0a, 0x23,
|
|
0x47, 0x65, 0x74, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x65, 0x65, 0x72,
|
|
0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x12, 0x3c, 0x0a, 0x05, 0x69, 0x6e, 0x66, 0x6f, 0x73, 0x18, 0x01, 0x20, 0x03,
|
|
0x28, 0x0b, 0x32, 0x26, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x47,
|
|
0x65, 0x74, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x65, 0x65, 0x72, 0x49,
|
|
0x6e, 0x66, 0x6f, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x52, 0x05, 0x69, 0x6e, 0x66, 0x6f,
|
|
0x73, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50,
|
|
0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0xd3, 0x02,
|
|
0x0a, 0x1b, 0x47, 0x65, 0x74, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x65,
|
|
0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x0e, 0x0a,
|
|
0x02, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x64, 0x12, 0x18, 0x0a,
|
|
0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07,
|
|
0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x2a, 0x0a, 0x10, 0x6c, 0x61, 0x73, 0x74, 0x50,
|
|
0x69, 0x6e, 0x67, 0x44, 0x75, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x03, 0x20, 0x01, 0x28,
|
|
0x03, 0x52, 0x10, 0x6c, 0x61, 0x73, 0x74, 0x50, 0x69, 0x6e, 0x67, 0x44, 0x75, 0x72, 0x61, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x12, 0x1e, 0x0a, 0x0a, 0x69, 0x73, 0x4f, 0x75, 0x74, 0x62, 0x6f, 0x75, 0x6e,
|
|
0x64, 0x18, 0x06, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0a, 0x69, 0x73, 0x4f, 0x75, 0x74, 0x62, 0x6f,
|
|
0x75, 0x6e, 0x64, 0x12, 0x1e, 0x0a, 0x0a, 0x74, 0x69, 0x6d, 0x65, 0x4f, 0x66, 0x66, 0x73, 0x65,
|
|
0x74, 0x18, 0x07, 0x20, 0x01, 0x28, 0x03, 0x52, 0x0a, 0x74, 0x69, 0x6d, 0x65, 0x4f, 0x66, 0x66,
|
|
0x73, 0x65, 0x74, 0x12, 0x1c, 0x0a, 0x09, 0x75, 0x73, 0x65, 0x72, 0x41, 0x67, 0x65, 0x6e, 0x74,
|
|
0x18, 0x08, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x75, 0x73, 0x65, 0x72, 0x41, 0x67, 0x65, 0x6e,
|
|
0x74, 0x12, 0x3c, 0x0a, 0x19, 0x61, 0x64, 0x76, 0x65, 0x72, 0x74, 0x69, 0x73, 0x65, 0x64, 0x50,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x09,
|
|
0x20, 0x01, 0x28, 0x0d, 0x52, 0x19, 0x61, 0x64, 0x76, 0x65, 0x72, 0x74, 0x69, 0x73, 0x65, 0x64,
|
|
0x50, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12,
|
|
0x24, 0x0a, 0x0d, 0x74, 0x69, 0x6d, 0x65, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x65, 0x64,
|
|
0x18, 0x0a, 0x20, 0x01, 0x28, 0x03, 0x52, 0x0d, 0x74, 0x69, 0x6d, 0x65, 0x43, 0x6f, 0x6e, 0x6e,
|
|
0x65, 0x63, 0x74, 0x65, 0x64, 0x12, 0x1c, 0x0a, 0x09, 0x69, 0x73, 0x49, 0x62, 0x64, 0x50, 0x65,
|
|
0x65, 0x72, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x08, 0x52, 0x09, 0x69, 0x73, 0x49, 0x62, 0x64, 0x50,
|
|
0x65, 0x65, 0x72, 0x22, 0x53, 0x0a, 0x15, 0x41, 0x64, 0x64, 0x50, 0x65, 0x65, 0x72, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x18, 0x0a, 0x07,
|
|
0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x61,
|
|
0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x20, 0x0a, 0x0b, 0x69, 0x73, 0x50, 0x65, 0x72, 0x6d,
|
|
0x61, 0x6e, 0x65, 0x6e, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0b, 0x69, 0x73, 0x50,
|
|
0x65, 0x72, 0x6d, 0x61, 0x6e, 0x65, 0x6e, 0x74, 0x22, 0x44, 0x0a, 0x16, 0x41, 0x64, 0x64, 0x50,
|
|
0x65, 0x65, 0x72, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61,
|
|
0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52,
|
|
0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x80,
|
|
0x01, 0x0a, 0x1f, 0x53, 0x75, 0x62, 0x6d, 0x69, 0x74, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61,
|
|
0x67, 0x65, 0x12, 0x3b, 0x0a, 0x0b, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x52, 0x0b, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12,
|
|
0x20, 0x0a, 0x0b, 0x61, 0x6c, 0x6c, 0x6f, 0x77, 0x4f, 0x72, 0x70, 0x68, 0x61, 0x6e, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x08, 0x52, 0x0b, 0x61, 0x6c, 0x6c, 0x6f, 0x77, 0x4f, 0x72, 0x70, 0x68, 0x61,
|
|
0x6e, 0x22, 0x74, 0x0a, 0x20, 0x53, 0x75, 0x62, 0x6d, 0x69, 0x74, 0x54, 0x72, 0x61, 0x6e, 0x73,
|
|
0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x24, 0x0a, 0x0d, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0d, 0x74, 0x72,
|
|
0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x12, 0x2a, 0x0a, 0x05, 0x65,
|
|
0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72,
|
|
0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x7d, 0x0a, 0x35, 0x4e, 0x6f, 0x74, 0x69, 0x66,
|
|
0x79, 0x56, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64,
|
|
0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x43, 0x68, 0x61, 0x6e, 0x67,
|
|
0x65, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x12, 0x44, 0x0a, 0x1d, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x41, 0x63, 0x63, 0x65, 0x70,
|
|
0x74, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64,
|
|
0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x08, 0x52, 0x1d, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65,
|
|
0x41, 0x63, 0x63, 0x65, 0x70, 0x74, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x49, 0x64, 0x73, 0x22, 0x64, 0x0a, 0x36, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79,
|
|
0x56, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50,
|
|
0x61, 0x72, 0x65, 0x6e, 0x74, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65,
|
|
0x64, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43,
|
|
0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x81, 0x02, 0x0a,
|
|
0x34, 0x56, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64,
|
|
0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x43, 0x68, 0x61, 0x6e, 0x67,
|
|
0x65, 0x64, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x38, 0x0a, 0x17, 0x72, 0x65, 0x6d, 0x6f, 0x76, 0x65, 0x64,
|
|
0x43, 0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73,
|
|
0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x17, 0x72, 0x65, 0x6d, 0x6f, 0x76, 0x65, 0x64, 0x43,
|
|
0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x12,
|
|
0x34, 0x0a, 0x15, 0x61, 0x64, 0x64, 0x65, 0x64, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18, 0x03, 0x20, 0x03, 0x28, 0x09, 0x52, 0x15,
|
|
0x61, 0x64, 0x64, 0x65, 0x64, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48,
|
|
0x61, 0x73, 0x68, 0x65, 0x73, 0x12, 0x59, 0x0a, 0x16, 0x61, 0x63, 0x63, 0x65, 0x70, 0x74, 0x65,
|
|
0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x73, 0x18,
|
|
0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x41, 0x63, 0x63, 0x65, 0x70, 0x74, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61,
|
|
0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x73, 0x52, 0x16, 0x61, 0x63, 0x63, 0x65, 0x70, 0x74,
|
|
0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x73,
|
|
0x22, 0x5e, 0x0a, 0x16, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x68, 0x61,
|
|
0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x68, 0x61, 0x73, 0x68, 0x12, 0x30,
|
|
0x0a, 0x13, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x13, 0x69, 0x6e, 0x63,
|
|
0x6c, 0x75, 0x64, 0x65, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73,
|
|
0x22, 0x70, 0x0a, 0x17, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x65, 0x73, 0x70,
|
|
0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x29, 0x0a, 0x05, 0x62,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52,
|
|
0x05, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18,
|
|
0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72,
|
|
0x6f, 0x72, 0x22, 0x41, 0x0a, 0x1b, 0x47, 0x65, 0x74, 0x53, 0x75, 0x62, 0x6e, 0x65, 0x74, 0x77,
|
|
0x6f, 0x72, 0x6b, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x22, 0x0a, 0x0c, 0x73, 0x75, 0x62, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49,
|
|
0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0c, 0x73, 0x75, 0x62, 0x6e, 0x65, 0x74, 0x77,
|
|
0x6f, 0x72, 0x6b, 0x49, 0x64, 0x22, 0x66, 0x0a, 0x1c, 0x47, 0x65, 0x74, 0x53, 0x75, 0x62, 0x6e,
|
|
0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1a, 0x0a, 0x08, 0x67, 0x61, 0x73, 0x4c, 0x69, 0x6d, 0x69,
|
|
0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x67, 0x61, 0x73, 0x4c, 0x69, 0x6d, 0x69,
|
|
0x74, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50,
|
|
0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x9a, 0x01,
|
|
0x0a, 0x34, 0x47, 0x65, 0x74, 0x56, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65,
|
|
0x63, 0x74, 0x65, 0x64, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x46,
|
|
0x72, 0x6f, 0x6d, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1c, 0x0a, 0x09, 0x73, 0x74, 0x61, 0x72, 0x74, 0x48,
|
|
0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x73, 0x74, 0x61, 0x72, 0x74,
|
|
0x48, 0x61, 0x73, 0x68, 0x12, 0x44, 0x0a, 0x1d, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x41,
|
|
0x63, 0x63, 0x65, 0x70, 0x74, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x49, 0x64, 0x73, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x1d, 0x69, 0x6e, 0x63,
|
|
0x6c, 0x75, 0x64, 0x65, 0x41, 0x63, 0x63, 0x65, 0x70, 0x74, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e,
|
|
0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x73, 0x22, 0x80, 0x01, 0x0a, 0x16, 0x41,
|
|
0x63, 0x63, 0x65, 0x70, 0x74, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x49, 0x64, 0x73, 0x12, 0x2e, 0x0a, 0x12, 0x61, 0x63, 0x63, 0x65, 0x70, 0x74, 0x69,
|
|
0x6e, 0x67, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x12, 0x61, 0x63, 0x63, 0x65, 0x70, 0x74, 0x69, 0x6e, 0x67, 0x42, 0x6c, 0x6f, 0x63,
|
|
0x6b, 0x48, 0x61, 0x73, 0x68, 0x12, 0x36, 0x0a, 0x16, 0x61, 0x63, 0x63, 0x65, 0x70, 0x74, 0x65,
|
|
0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x73, 0x18,
|
|
0x02, 0x20, 0x03, 0x28, 0x09, 0x52, 0x16, 0x61, 0x63, 0x63, 0x65, 0x70, 0x74, 0x65, 0x64, 0x54,
|
|
0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x73, 0x22, 0xae, 0x02,
|
|
0x0a, 0x35, 0x47, 0x65, 0x74, 0x56, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65,
|
|
0x63, 0x74, 0x65, 0x64, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x46,
|
|
0x72, 0x6f, 0x6d, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x38, 0x0a, 0x17, 0x72, 0x65, 0x6d, 0x6f, 0x76,
|
|
0x65, 0x64, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68,
|
|
0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x17, 0x72, 0x65, 0x6d, 0x6f, 0x76, 0x65,
|
|
0x64, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x65,
|
|
0x73, 0x12, 0x34, 0x0a, 0x15, 0x61, 0x64, 0x64, 0x65, 0x64, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x42,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18, 0x03, 0x20, 0x03, 0x28, 0x09,
|
|
0x52, 0x15, 0x61, 0x64, 0x64, 0x65, 0x64, 0x43, 0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63,
|
|
0x6b, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x12, 0x59, 0x0a, 0x16, 0x61, 0x63, 0x63, 0x65, 0x70,
|
|
0x74, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64,
|
|
0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x41, 0x63, 0x63, 0x65, 0x70, 0x74, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e,
|
|
0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x73, 0x52, 0x16, 0x61, 0x63, 0x63, 0x65,
|
|
0x70, 0x74, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49,
|
|
0x64, 0x73, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52,
|
|
0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x8b,
|
|
0x01, 0x0a, 0x17, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x18, 0x0a, 0x07, 0x6c, 0x6f,
|
|
0x77, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x6c, 0x6f, 0x77,
|
|
0x48, 0x61, 0x73, 0x68, 0x12, 0x24, 0x0a, 0x0d, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x42,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0d, 0x69, 0x6e, 0x63,
|
|
0x6c, 0x75, 0x64, 0x65, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x12, 0x30, 0x0a, 0x13, 0x69, 0x6e,
|
|
0x63, 0x6c, 0x75, 0x64, 0x65, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x13, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65,
|
|
0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x22, 0x95, 0x01, 0x0a,
|
|
0x18, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e,
|
|
0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x20, 0x0a, 0x0b, 0x62, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0b,
|
|
0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x12, 0x2b, 0x0a, 0x06, 0x62,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x18, 0x03, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x52, 0x06, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f,
|
|
0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65,
|
|
0x72, 0x72, 0x6f, 0x72, 0x22, 0x1d, 0x0a, 0x1b, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x43, 0x6f, 0x75, 0x6e, 0x74, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x22, 0x8c, 0x01, 0x0a, 0x1c, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x43, 0x6f, 0x75, 0x6e, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73,
|
|
0x73, 0x61, 0x67, 0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x43, 0x6f, 0x75,
|
|
0x6e, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0a, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x43,
|
|
0x6f, 0x75, 0x6e, 0x74, 0x12, 0x20, 0x0a, 0x0b, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x43, 0x6f,
|
|
0x75, 0x6e, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b, 0x68, 0x65, 0x61, 0x64, 0x65,
|
|
0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18,
|
|
0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72,
|
|
0x6f, 0x72, 0x22, 0x1f, 0x0a, 0x1d, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x44, 0x61,
|
|
0x67, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x22, 0x9e, 0x03, 0x0a, 0x1e, 0x47, 0x65, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x44, 0x61, 0x67, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x20, 0x0a, 0x0b, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72,
|
|
0x6b, 0x4e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x6e, 0x65, 0x74,
|
|
0x77, 0x6f, 0x72, 0x6b, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x62, 0x6c, 0x6f, 0x63,
|
|
0x6b, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0a, 0x62, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x20, 0x0a, 0x0b, 0x68, 0x65, 0x61, 0x64,
|
|
0x65, 0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b, 0x68,
|
|
0x65, 0x61, 0x64, 0x65, 0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x1c, 0x0a, 0x09, 0x74, 0x69,
|
|
0x70, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x09, 0x52, 0x09, 0x74,
|
|
0x69, 0x70, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x12, 0x1e, 0x0a, 0x0a, 0x64, 0x69, 0x66, 0x66,
|
|
0x69, 0x63, 0x75, 0x6c, 0x74, 0x79, 0x18, 0x05, 0x20, 0x01, 0x28, 0x01, 0x52, 0x0a, 0x64, 0x69,
|
|
0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74, 0x79, 0x12, 0x26, 0x0a, 0x0e, 0x70, 0x61, 0x73, 0x74,
|
|
0x4d, 0x65, 0x64, 0x69, 0x61, 0x6e, 0x54, 0x69, 0x6d, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28, 0x03,
|
|
0x52, 0x0e, 0x70, 0x61, 0x73, 0x74, 0x4d, 0x65, 0x64, 0x69, 0x61, 0x6e, 0x54, 0x69, 0x6d, 0x65,
|
|
0x12, 0x30, 0x0a, 0x13, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x50, 0x61, 0x72, 0x65, 0x6e,
|
|
0x74, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x18, 0x07, 0x20, 0x03, 0x28, 0x09, 0x52, 0x13, 0x76,
|
|
0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x48, 0x61, 0x73, 0x68,
|
|
0x65, 0x73, 0x12, 0x2a, 0x0a, 0x10, 0x70, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69,
|
|
0x6e, 0x74, 0x48, 0x61, 0x73, 0x68, 0x18, 0x08, 0x20, 0x01, 0x28, 0x09, 0x52, 0x10, 0x70, 0x72,
|
|
0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x48, 0x61, 0x73, 0x68, 0x12, 0x28,
|
|
0x0a, 0x0f, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72,
|
|
0x65, 0x18, 0x09, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0f, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c,
|
|
0x44, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f,
|
|
0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65,
|
|
0x72, 0x72, 0x6f, 0x72, 0x22, 0x55, 0x0a, 0x25, 0x52, 0x65, 0x73, 0x6f, 0x6c, 0x76, 0x65, 0x46,
|
|
0x69, 0x6e, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x43, 0x6f, 0x6e, 0x66, 0x6c, 0x69, 0x63, 0x74, 0x52,
|
|
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2c, 0x0a,
|
|
0x11, 0x66, 0x69, 0x6e, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61,
|
|
0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x11, 0x66, 0x69, 0x6e, 0x61, 0x6c, 0x69,
|
|
0x74, 0x79, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x22, 0x54, 0x0a, 0x26, 0x52,
|
|
0x65, 0x73, 0x6f, 0x6c, 0x76, 0x65, 0x46, 0x69, 0x6e, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x43, 0x6f,
|
|
0x6e, 0x66, 0x6c, 0x69, 0x63, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8,
|
|
0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f,
|
|
0x72, 0x22, 0x27, 0x0a, 0x25, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x46, 0x69, 0x6e, 0x61, 0x6c,
|
|
0x69, 0x74, 0x79, 0x43, 0x6f, 0x6e, 0x66, 0x6c, 0x69, 0x63, 0x74, 0x73, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x54, 0x0a, 0x26, 0x4e, 0x6f,
|
|
0x74, 0x69, 0x66, 0x79, 0x46, 0x69, 0x6e, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x43, 0x6f, 0x6e, 0x66,
|
|
0x6c, 0x69, 0x63, 0x74, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73,
|
|
0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65,
|
|
0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72,
|
|
0x22, 0x55, 0x0a, 0x23, 0x46, 0x69, 0x6e, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x43, 0x6f, 0x6e, 0x66,
|
|
0x6c, 0x69, 0x63, 0x74, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2e, 0x0a, 0x12, 0x76, 0x69, 0x6f, 0x6c, 0x61,
|
|
0x74, 0x69, 0x6e, 0x67, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x12, 0x76, 0x69, 0x6f, 0x6c, 0x61, 0x74, 0x69, 0x6e, 0x67, 0x42, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x22, 0x5b, 0x0a, 0x2b, 0x46, 0x69, 0x6e, 0x61, 0x6c,
|
|
0x69, 0x74, 0x79, 0x43, 0x6f, 0x6e, 0x66, 0x6c, 0x69, 0x63, 0x74, 0x52, 0x65, 0x73, 0x6f, 0x6c,
|
|
0x76, 0x65, 0x64, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2c, 0x0a, 0x11, 0x66, 0x69, 0x6e, 0x61, 0x6c, 0x69,
|
|
0x74, 0x79, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x11, 0x66, 0x69, 0x6e, 0x61, 0x6c, 0x69, 0x74, 0x79, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x48, 0x61, 0x73, 0x68, 0x22, 0x18, 0x0a, 0x16, 0x53, 0x68, 0x75, 0x74, 0x44, 0x6f, 0x77, 0x6e,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x45,
|
|
0x0a, 0x17, 0x53, 0x68, 0x75, 0x74, 0x44, 0x6f, 0x77, 0x6e, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e,
|
|
0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72,
|
|
0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05,
|
|
0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x70, 0x0a, 0x18, 0x47, 0x65, 0x74, 0x48, 0x65, 0x61, 0x64,
|
|
0x65, 0x72, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x1c, 0x0a, 0x09, 0x73, 0x74, 0x61, 0x72, 0x74, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x73, 0x74, 0x61, 0x72, 0x74, 0x48, 0x61, 0x73, 0x68, 0x12,
|
|
0x14, 0x0a, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x05,
|
|
0x6c, 0x69, 0x6d, 0x69, 0x74, 0x12, 0x20, 0x0a, 0x0b, 0x69, 0x73, 0x41, 0x73, 0x63, 0x65, 0x6e,
|
|
0x64, 0x69, 0x6e, 0x67, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0b, 0x69, 0x73, 0x41, 0x73,
|
|
0x63, 0x65, 0x6e, 0x64, 0x69, 0x6e, 0x67, 0x22, 0x61, 0x0a, 0x19, 0x47, 0x65, 0x74, 0x48, 0x65,
|
|
0x61, 0x64, 0x65, 0x72, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73,
|
|
0x73, 0x61, 0x67, 0x65, 0x12, 0x18, 0x0a, 0x07, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x73, 0x18,
|
|
0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x07, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x73, 0x12, 0x2a,
|
|
0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72,
|
|
0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x40, 0x0a, 0x20, 0x4e, 0x6f,
|
|
0x74, 0x69, 0x66, 0x79, 0x55, 0x74, 0x78, 0x6f, 0x73, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x64,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1c,
|
|
0x0a, 0x09, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28,
|
|
0x09, 0x52, 0x09, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x22, 0x4f, 0x0a, 0x21,
|
|
0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x55, 0x74, 0x78, 0x6f, 0x73, 0x43, 0x68, 0x61, 0x6e, 0x67,
|
|
0x65, 0x64, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50,
|
|
0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x95, 0x01,
|
|
0x0a, 0x1f, 0x55, 0x74, 0x78, 0x6f, 0x73, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x64, 0x4e, 0x6f,
|
|
0x74, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x36, 0x0a, 0x05, 0x61, 0x64, 0x64, 0x65, 0x64, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b,
|
|
0x32, 0x20, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x55, 0x74, 0x78,
|
|
0x6f, 0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x45, 0x6e, 0x74,
|
|
0x72, 0x79, 0x52, 0x05, 0x61, 0x64, 0x64, 0x65, 0x64, 0x12, 0x3a, 0x0a, 0x07, 0x72, 0x65, 0x6d,
|
|
0x6f, 0x76, 0x65, 0x64, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x20, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x55, 0x74, 0x78, 0x6f, 0x73, 0x42, 0x79, 0x41, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x07, 0x72, 0x65,
|
|
0x6d, 0x6f, 0x76, 0x65, 0x64, 0x22, 0x9c, 0x01, 0x0a, 0x15, 0x55, 0x74, 0x78, 0x6f, 0x73, 0x42,
|
|
0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12,
|
|
0x18, 0x0a, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x32, 0x0a, 0x08, 0x6f, 0x75, 0x74,
|
|
0x70, 0x6f, 0x69, 0x6e, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x16, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x4f, 0x75, 0x74, 0x70, 0x6f,
|
|
0x69, 0x6e, 0x74, 0x52, 0x08, 0x6f, 0x75, 0x74, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x12, 0x35, 0x0a,
|
|
0x09, 0x75, 0x74, 0x78, 0x6f, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63,
|
|
0x55, 0x74, 0x78, 0x6f, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x09, 0x75, 0x74, 0x78, 0x6f, 0x45,
|
|
0x6e, 0x74, 0x72, 0x79, 0x22, 0x47, 0x0a, 0x27, 0x53, 0x74, 0x6f, 0x70, 0x4e, 0x6f, 0x74, 0x69,
|
|
0x66, 0x79, 0x69, 0x6e, 0x67, 0x55, 0x74, 0x78, 0x6f, 0x73, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65,
|
|
0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x1c, 0x0a, 0x09, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03,
|
|
0x28, 0x09, 0x52, 0x09, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x22, 0x56, 0x0a,
|
|
0x28, 0x53, 0x74, 0x6f, 0x70, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x69, 0x6e, 0x67, 0x55, 0x74,
|
|
0x78, 0x6f, 0x73, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x64, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e,
|
|
0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72,
|
|
0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05,
|
|
0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x41, 0x0a, 0x21, 0x47, 0x65, 0x74, 0x55, 0x74, 0x78, 0x6f,
|
|
0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1c, 0x0a, 0x09, 0x61, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x09, 0x61,
|
|
0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x22, 0x8c, 0x01, 0x0a, 0x22, 0x47, 0x65, 0x74,
|
|
0x55, 0x74, 0x78, 0x6f, 0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73,
|
|
0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x3a, 0x0a, 0x07, 0x65, 0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b,
|
|
0x32, 0x20, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x55, 0x74, 0x78,
|
|
0x6f, 0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x45, 0x6e, 0x74,
|
|
0x72, 0x79, 0x52, 0x07, 0x65, 0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x12, 0x2a, 0x0a, 0x05, 0x65,
|
|
0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72,
|
|
0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x3d, 0x0a, 0x21, 0x47, 0x65, 0x74, 0x42, 0x61,
|
|
0x6c, 0x61, 0x6e, 0x63, 0x65, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x18, 0x0a, 0x07,
|
|
0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x61,
|
|
0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x22, 0x6a, 0x0a, 0x22, 0x47, 0x65, 0x74, 0x42, 0x61, 0x6c,
|
|
0x61, 0x6e, 0x63, 0x65, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x18, 0x0a, 0x07,
|
|
0x62, 0x61, 0x6c, 0x61, 0x6e, 0x63, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x07, 0x62,
|
|
0x61, 0x6c, 0x61, 0x6e, 0x63, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18,
|
|
0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72,
|
|
0x6f, 0x72, 0x22, 0x44, 0x0a, 0x24, 0x47, 0x65, 0x74, 0x42, 0x61, 0x6c, 0x61, 0x6e, 0x63, 0x65,
|
|
0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1c, 0x0a, 0x09, 0x61, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x09, 0x61,
|
|
0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x22, 0x78, 0x0a, 0x16, 0x42, 0x61, 0x6c, 0x61,
|
|
0x6e, 0x63, 0x65, 0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x45, 0x6e, 0x74,
|
|
0x72, 0x79, 0x12, 0x18, 0x0a, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x18, 0x0a, 0x07,
|
|
0x62, 0x61, 0x6c, 0x61, 0x6e, 0x63, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x07, 0x62,
|
|
0x61, 0x6c, 0x61, 0x6e, 0x63, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18,
|
|
0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69,
|
|
0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72,
|
|
0x6f, 0x72, 0x22, 0x90, 0x01, 0x0a, 0x25, 0x47, 0x65, 0x74, 0x42, 0x61, 0x6c, 0x61, 0x6e, 0x63,
|
|
0x65, 0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x3b, 0x0a, 0x07,
|
|
0x65, 0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x21, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x42, 0x61, 0x6c, 0x61, 0x6e, 0x63,
|
|
0x65, 0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79,
|
|
0x52, 0x07, 0x65, 0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72,
|
|
0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05,
|
|
0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x31, 0x0a, 0x2f, 0x47, 0x65, 0x74, 0x56, 0x69, 0x72, 0x74,
|
|
0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x61, 0x72, 0x65, 0x6e,
|
|
0x74, 0x42, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
|
0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x7c, 0x0a, 0x30, 0x47, 0x65, 0x74, 0x56,
|
|
0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x61,
|
|
0x72, 0x65, 0x6e, 0x74, 0x42, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1c, 0x0a, 0x09,
|
|
0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x09, 0x62, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72,
|
|
0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52,
|
|
0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x3b, 0x0a, 0x39, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79,
|
|
0x56, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50,
|
|
0x61, 0x72, 0x65, 0x6e, 0x74, 0x42, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x43, 0x68,
|
|
0x61, 0x6e, 0x67, 0x65, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x22, 0x68, 0x0a, 0x3a, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x56, 0x69, 0x72,
|
|
0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x61, 0x72, 0x65,
|
|
0x6e, 0x74, 0x42, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x43, 0x68, 0x61, 0x6e, 0x67,
|
|
0x65, 0x64, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50,
|
|
0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x82, 0x01,
|
|
0x0a, 0x38, 0x56, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65,
|
|
0x64, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x42, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65,
|
|
0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x64, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x46, 0x0a, 0x1e, 0x76, 0x69,
|
|
0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x65, 0x64, 0x50, 0x61, 0x72,
|
|
0x65, 0x6e, 0x74, 0x42, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x04, 0x52, 0x1e, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x53, 0x65, 0x6c, 0x65, 0x63,
|
|
0x74, 0x65, 0x64, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x42, 0x6c, 0x75, 0x65, 0x53, 0x63, 0x6f,
|
|
0x72, 0x65, 0x22, 0x2c, 0x0a, 0x2a, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x56, 0x69, 0x72, 0x74,
|
|
0x75, 0x61, 0x6c, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x43, 0x68, 0x61, 0x6e, 0x67,
|
|
0x65, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x22, 0x59, 0x0a, 0x2b, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x56, 0x69, 0x72, 0x74, 0x75, 0x61,
|
|
0x6c, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x43, 0x68, 0x61, 0x6e, 0x67, 0x65, 0x64,
|
|
0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45,
|
|
0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x55, 0x0a, 0x29, 0x56,
|
|
0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x43, 0x68,
|
|
0x61, 0x6e, 0x67, 0x65, 0x64, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x28, 0x0a, 0x0f, 0x76, 0x69, 0x72, 0x74,
|
|
0x75, 0x61, 0x6c, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x0f, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f,
|
|
0x72, 0x65, 0x22, 0x31, 0x0a, 0x2f, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x50, 0x72, 0x75, 0x6e,
|
|
0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x55, 0x54, 0x58, 0x4f, 0x53, 0x65, 0x74, 0x4f,
|
|
0x76, 0x65, 0x72, 0x72, 0x69, 0x64, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x5e, 0x0a, 0x30, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x50,
|
|
0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x55, 0x54, 0x58, 0x4f, 0x53,
|
|
0x65, 0x74, 0x4f, 0x76, 0x65, 0x72, 0x72, 0x69, 0x64, 0x65, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e,
|
|
0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72,
|
|
0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05,
|
|
0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x30, 0x0a, 0x2e, 0x50, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67,
|
|
0x50, 0x6f, 0x69, 0x6e, 0x74, 0x55, 0x54, 0x58, 0x4f, 0x53, 0x65, 0x74, 0x4f, 0x76, 0x65, 0x72,
|
|
0x72, 0x69, 0x64, 0x65, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x38, 0x0a, 0x36, 0x53, 0x74, 0x6f, 0x70, 0x4e,
|
|
0x6f, 0x74, 0x69, 0x66, 0x79, 0x69, 0x6e, 0x67, 0x50, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50,
|
|
0x6f, 0x69, 0x6e, 0x74, 0x55, 0x54, 0x58, 0x4f, 0x53, 0x65, 0x74, 0x4f, 0x76, 0x65, 0x72, 0x72,
|
|
0x69, 0x64, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x22, 0x65, 0x0a, 0x37, 0x53, 0x74, 0x6f, 0x70, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x69,
|
|
0x6e, 0x67, 0x50, 0x72, 0x75, 0x6e, 0x69, 0x6e, 0x67, 0x50, 0x6f, 0x69, 0x6e, 0x74, 0x55, 0x54,
|
|
0x58, 0x4f, 0x53, 0x65, 0x74, 0x4f, 0x76, 0x65, 0x72, 0x72, 0x69, 0x64, 0x65, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05,
|
|
0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f,
|
|
0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x23, 0x0a, 0x11, 0x42, 0x61, 0x6e, 0x52,
|
|
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x0e, 0x0a,
|
|
0x02, 0x69, 0x70, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x69, 0x70, 0x22, 0x40, 0x0a,
|
|
0x12, 0x42, 0x61, 0x6e, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e,
|
|
0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22,
|
|
0x25, 0x0a, 0x13, 0x55, 0x6e, 0x62, 0x61, 0x6e, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x70, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x02, 0x69, 0x70, 0x22, 0x42, 0x0a, 0x14, 0x55, 0x6e, 0x62, 0x61, 0x6e, 0x52,
|
|
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a,
|
|
0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72,
|
|
0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x17, 0x0a, 0x15, 0x47, 0x65,
|
|
0x74, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x22, 0xe4, 0x01, 0x0a, 0x16, 0x47, 0x65, 0x74, 0x49, 0x6e, 0x66, 0x6f, 0x52,
|
|
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x14,
|
|
0x0a, 0x05, 0x70, 0x32, 0x70, 0x49, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x70,
|
|
0x32, 0x70, 0x49, 0x64, 0x12, 0x20, 0x0a, 0x0b, 0x6d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x53,
|
|
0x69, 0x7a, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b, 0x6d, 0x65, 0x6d, 0x70, 0x6f,
|
|
0x6f, 0x6c, 0x53, 0x69, 0x7a, 0x65, 0x12, 0x24, 0x0a, 0x0d, 0x73, 0x65, 0x72, 0x76, 0x65, 0x72,
|
|
0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0d, 0x73,
|
|
0x65, 0x72, 0x76, 0x65, 0x72, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x24, 0x0a, 0x0d,
|
|
0x69, 0x73, 0x55, 0x74, 0x78, 0x6f, 0x49, 0x6e, 0x64, 0x65, 0x78, 0x65, 0x64, 0x18, 0x04, 0x20,
|
|
0x01, 0x28, 0x08, 0x52, 0x0d, 0x69, 0x73, 0x55, 0x74, 0x78, 0x6f, 0x49, 0x6e, 0x64, 0x65, 0x78,
|
|
0x65, 0x64, 0x12, 0x1a, 0x0a, 0x08, 0x69, 0x73, 0x53, 0x79, 0x6e, 0x63, 0x65, 0x64, 0x18, 0x05,
|
|
0x20, 0x01, 0x28, 0x08, 0x52, 0x08, 0x69, 0x73, 0x53, 0x79, 0x6e, 0x63, 0x65, 0x64, 0x12, 0x2a,
|
|
0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72,
|
|
0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x6c, 0x0a, 0x2c, 0x45, 0x73,
|
|
0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x48, 0x61, 0x73,
|
|
0x68, 0x65, 0x73, 0x50, 0x65, 0x72, 0x53, 0x65, 0x63, 0x6f, 0x6e, 0x64, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x77, 0x69,
|
|
0x6e, 0x64, 0x6f, 0x77, 0x53, 0x69, 0x7a, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0a,
|
|
0x77, 0x69, 0x6e, 0x64, 0x6f, 0x77, 0x53, 0x69, 0x7a, 0x65, 0x12, 0x1c, 0x0a, 0x09, 0x73, 0x74,
|
|
0x61, 0x72, 0x74, 0x48, 0x61, 0x73, 0x68, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x73,
|
|
0x74, 0x61, 0x72, 0x74, 0x48, 0x61, 0x73, 0x68, 0x22, 0x93, 0x01, 0x0a, 0x2d, 0x45, 0x73, 0x74,
|
|
0x69, 0x6d, 0x61, 0x74, 0x65, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x48, 0x61, 0x73, 0x68,
|
|
0x65, 0x73, 0x50, 0x65, 0x72, 0x53, 0x65, 0x63, 0x6f, 0x6e, 0x64, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
|
0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x36, 0x0a, 0x16, 0x6e, 0x65,
|
|
0x74, 0x77, 0x6f, 0x72, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x50, 0x65, 0x72, 0x53, 0x65,
|
|
0x63, 0x6f, 0x6e, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x16, 0x6e, 0x65, 0x74, 0x77,
|
|
0x6f, 0x72, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x50, 0x65, 0x72, 0x53, 0x65, 0x63, 0x6f,
|
|
0x6e, 0x64, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52,
|
|
0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x26,
|
|
0x0a, 0x24, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79, 0x4e, 0x65, 0x77, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x54, 0x65, 0x6d, 0x70, 0x6c, 0x61, 0x74, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x53, 0x0a, 0x25, 0x4e, 0x6f, 0x74, 0x69, 0x66, 0x79,
|
|
0x4e, 0x65, 0x77, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x65, 0x6d, 0x70, 0x6c, 0x61, 0x74, 0x65,
|
|
0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45,
|
|
0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x25, 0x0a, 0x23, 0x4e,
|
|
0x65, 0x77, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x65, 0x6d, 0x70, 0x6c, 0x61, 0x74, 0x65, 0x4e,
|
|
0x6f, 0x74, 0x69, 0x66, 0x69, 0x63, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61,
|
|
0x67, 0x65, 0x22, 0x9b, 0x01, 0x0a, 0x15, 0x4d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45, 0x6e,
|
|
0x74, 0x72, 0x79, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x18, 0x0a, 0x07,
|
|
0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x61,
|
|
0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x31, 0x0a, 0x07, 0x73, 0x65, 0x6e, 0x64, 0x69, 0x6e,
|
|
0x67, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x4d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45, 0x6e, 0x74, 0x72, 0x79,
|
|
0x52, 0x07, 0x73, 0x65, 0x6e, 0x64, 0x69, 0x6e, 0x67, 0x12, 0x35, 0x0a, 0x09, 0x72, 0x65, 0x63,
|
|
0x65, 0x69, 0x76, 0x69, 0x6e, 0x67, 0x18, 0x03, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x17, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x4d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x09, 0x72, 0x65, 0x63, 0x65, 0x69, 0x76, 0x69, 0x6e, 0x67,
|
|
0x22, 0xae, 0x01, 0x0a, 0x2a, 0x47, 0x65, 0x74, 0x4d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45,
|
|
0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65,
|
|
0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x1c, 0x0a, 0x09, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03,
|
|
0x28, 0x09, 0x52, 0x09, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x12, 0x2c, 0x0a,
|
|
0x11, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x4f, 0x72, 0x70, 0x68, 0x61, 0x6e, 0x50, 0x6f,
|
|
0x6f, 0x6c, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x11, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64,
|
|
0x65, 0x4f, 0x72, 0x70, 0x68, 0x61, 0x6e, 0x50, 0x6f, 0x6f, 0x6c, 0x12, 0x34, 0x0a, 0x15, 0x66,
|
|
0x69, 0x6c, 0x74, 0x65, 0x72, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x50, 0x6f, 0x6f, 0x6c, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x15, 0x66, 0x69, 0x6c, 0x74,
|
|
0x65, 0x72, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x50, 0x6f, 0x6f,
|
|
0x6c, 0x22, 0x95, 0x01, 0x0a, 0x2b, 0x47, 0x65, 0x74, 0x4d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c,
|
|
0x45, 0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x42, 0x79, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73,
|
|
0x65, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x3a, 0x0a, 0x07, 0x65, 0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03,
|
|
0x28, 0x0b, 0x32, 0x20, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x4d,
|
|
0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x42, 0x79, 0x41, 0x64, 0x64,
|
|
0x72, 0x65, 0x73, 0x73, 0x52, 0x07, 0x65, 0x6e, 0x74, 0x72, 0x69, 0x65, 0x73, 0x12, 0x2a, 0x0a,
|
|
0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72,
|
|
0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x1d, 0x0a, 0x1b, 0x47, 0x65, 0x74,
|
|
0x43, 0x6f, 0x69, 0x6e, 0x53, 0x75, 0x70, 0x70, 0x6c, 0x79, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
|
0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x92, 0x01, 0x0a, 0x1c, 0x47, 0x65, 0x74,
|
|
0x43, 0x6f, 0x69, 0x6e, 0x53, 0x75, 0x70, 0x70, 0x6c, 0x79, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e,
|
|
0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1a, 0x0a, 0x08, 0x6d, 0x61, 0x78,
|
|
0x53, 0x6f, 0x6d, 0x70, 0x69, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x6d, 0x61, 0x78,
|
|
0x53, 0x6f, 0x6d, 0x70, 0x69, 0x12, 0x2a, 0x0a, 0x10, 0x63, 0x69, 0x72, 0x63, 0x75, 0x6c, 0x61,
|
|
0x74, 0x69, 0x6e, 0x67, 0x53, 0x6f, 0x6d, 0x70, 0x69, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x10, 0x63, 0x69, 0x72, 0x63, 0x75, 0x6c, 0x61, 0x74, 0x69, 0x6e, 0x67, 0x53, 0x6f, 0x6d, 0x70,
|
|
0x69, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50,
|
|
0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x14, 0x0a,
|
|
0x12, 0x50, 0x69, 0x6e, 0x67, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x22, 0x41, 0x0a, 0x13, 0x50, 0x69, 0x6e, 0x67, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
|
0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72,
|
|
0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52,
|
|
0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0xe4, 0x02, 0x0a, 0x0e, 0x50, 0x72, 0x6f, 0x63, 0x65,
|
|
0x73, 0x73, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x12, 0x28, 0x0a, 0x0f, 0x72, 0x65, 0x73,
|
|
0x69, 0x64, 0x65, 0x6e, 0x74, 0x53, 0x65, 0x74, 0x53, 0x69, 0x7a, 0x65, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x04, 0x52, 0x0f, 0x72, 0x65, 0x73, 0x69, 0x64, 0x65, 0x6e, 0x74, 0x53, 0x65, 0x74, 0x53,
|
|
0x69, 0x7a, 0x65, 0x12, 0x2c, 0x0a, 0x11, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x4d, 0x65,
|
|
0x6d, 0x6f, 0x72, 0x79, 0x53, 0x69, 0x7a, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x11,
|
|
0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x4d, 0x65, 0x6d, 0x6f, 0x72, 0x79, 0x53, 0x69, 0x7a,
|
|
0x65, 0x12, 0x18, 0x0a, 0x07, 0x63, 0x6f, 0x72, 0x65, 0x4e, 0x75, 0x6d, 0x18, 0x03, 0x20, 0x01,
|
|
0x28, 0x0d, 0x52, 0x07, 0x63, 0x6f, 0x72, 0x65, 0x4e, 0x75, 0x6d, 0x12, 0x1a, 0x0a, 0x08, 0x63,
|
|
0x70, 0x75, 0x55, 0x73, 0x61, 0x67, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x02, 0x52, 0x08, 0x63,
|
|
0x70, 0x75, 0x55, 0x73, 0x61, 0x67, 0x65, 0x12, 0x14, 0x0a, 0x05, 0x66, 0x64, 0x4e, 0x75, 0x6d,
|
|
0x18, 0x05, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x05, 0x66, 0x64, 0x4e, 0x75, 0x6d, 0x12, 0x28, 0x0a,
|
|
0x0f, 0x64, 0x69, 0x73, 0x6b, 0x49, 0x6f, 0x52, 0x65, 0x61, 0x64, 0x42, 0x79, 0x74, 0x65, 0x73,
|
|
0x18, 0x06, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0f, 0x64, 0x69, 0x73, 0x6b, 0x49, 0x6f, 0x52, 0x65,
|
|
0x61, 0x64, 0x42, 0x79, 0x74, 0x65, 0x73, 0x12, 0x2a, 0x0a, 0x10, 0x64, 0x69, 0x73, 0x6b, 0x49,
|
|
0x6f, 0x57, 0x72, 0x69, 0x74, 0x65, 0x42, 0x79, 0x74, 0x65, 0x73, 0x18, 0x07, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x10, 0x64, 0x69, 0x73, 0x6b, 0x49, 0x6f, 0x57, 0x72, 0x69, 0x74, 0x65, 0x42, 0x79,
|
|
0x74, 0x65, 0x73, 0x12, 0x2a, 0x0a, 0x10, 0x64, 0x69, 0x73, 0x6b, 0x49, 0x6f, 0x52, 0x65, 0x61,
|
|
0x64, 0x50, 0x65, 0x72, 0x53, 0x65, 0x63, 0x18, 0x08, 0x20, 0x01, 0x28, 0x02, 0x52, 0x10, 0x64,
|
|
0x69, 0x73, 0x6b, 0x49, 0x6f, 0x52, 0x65, 0x61, 0x64, 0x50, 0x65, 0x72, 0x53, 0x65, 0x63, 0x12,
|
|
0x2c, 0x0a, 0x11, 0x64, 0x69, 0x73, 0x6b, 0x49, 0x6f, 0x57, 0x72, 0x69, 0x74, 0x65, 0x50, 0x65,
|
|
0x72, 0x53, 0x65, 0x63, 0x18, 0x09, 0x20, 0x01, 0x28, 0x02, 0x52, 0x11, 0x64, 0x69, 0x73, 0x6b,
|
|
0x49, 0x6f, 0x57, 0x72, 0x69, 0x74, 0x65, 0x50, 0x65, 0x72, 0x53, 0x65, 0x63, 0x22, 0xfb, 0x02,
|
|
0x0a, 0x11, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x74, 0x72,
|
|
0x69, 0x63, 0x73, 0x12, 0x32, 0x0a, 0x14, 0x62, 0x6f, 0x72, 0x73, 0x68, 0x4c, 0x69, 0x76, 0x65,
|
|
0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x1f, 0x20, 0x01, 0x28,
|
|
0x0d, 0x52, 0x14, 0x62, 0x6f, 0x72, 0x73, 0x68, 0x4c, 0x69, 0x76, 0x65, 0x43, 0x6f, 0x6e, 0x6e,
|
|
0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x38, 0x0a, 0x17, 0x62, 0x6f, 0x72, 0x73, 0x68,
|
|
0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x41, 0x74, 0x74, 0x65, 0x6d, 0x70,
|
|
0x74, 0x73, 0x18, 0x20, 0x20, 0x01, 0x28, 0x04, 0x52, 0x17, 0x62, 0x6f, 0x72, 0x73, 0x68, 0x43,
|
|
0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x41, 0x74, 0x74, 0x65, 0x6d, 0x70, 0x74,
|
|
0x73, 0x12, 0x36, 0x0a, 0x16, 0x62, 0x6f, 0x72, 0x73, 0x68, 0x48, 0x61, 0x6e, 0x64, 0x73, 0x68,
|
|
0x61, 0x6b, 0x65, 0x46, 0x61, 0x69, 0x6c, 0x75, 0x72, 0x65, 0x73, 0x18, 0x21, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x16, 0x62, 0x6f, 0x72, 0x73, 0x68, 0x48, 0x61, 0x6e, 0x64, 0x73, 0x68, 0x61, 0x6b,
|
|
0x65, 0x46, 0x61, 0x69, 0x6c, 0x75, 0x72, 0x65, 0x73, 0x12, 0x30, 0x0a, 0x13, 0x6a, 0x73, 0x6f,
|
|
0x6e, 0x4c, 0x69, 0x76, 0x65, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73,
|
|
0x18, 0x29, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x13, 0x6a, 0x73, 0x6f, 0x6e, 0x4c, 0x69, 0x76, 0x65,
|
|
0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x36, 0x0a, 0x16, 0x6a,
|
|
0x73, 0x6f, 0x6e, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x41, 0x74, 0x74,
|
|
0x65, 0x6d, 0x70, 0x74, 0x73, 0x18, 0x2a, 0x20, 0x01, 0x28, 0x04, 0x52, 0x16, 0x6a, 0x73, 0x6f,
|
|
0x6e, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x41, 0x74, 0x74, 0x65, 0x6d,
|
|
0x70, 0x74, 0x73, 0x12, 0x34, 0x0a, 0x15, 0x6a, 0x73, 0x6f, 0x6e, 0x48, 0x61, 0x6e, 0x64, 0x73,
|
|
0x68, 0x61, 0x6b, 0x65, 0x46, 0x61, 0x69, 0x6c, 0x75, 0x72, 0x65, 0x73, 0x18, 0x2b, 0x20, 0x01,
|
|
0x28, 0x04, 0x52, 0x15, 0x6a, 0x73, 0x6f, 0x6e, 0x48, 0x61, 0x6e, 0x64, 0x73, 0x68, 0x61, 0x6b,
|
|
0x65, 0x46, 0x61, 0x69, 0x6c, 0x75, 0x72, 0x65, 0x73, 0x12, 0x20, 0x0a, 0x0b, 0x61, 0x63, 0x74,
|
|
0x69, 0x76, 0x65, 0x50, 0x65, 0x65, 0x72, 0x73, 0x18, 0x33, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0b,
|
|
0x61, 0x63, 0x74, 0x69, 0x76, 0x65, 0x50, 0x65, 0x65, 0x72, 0x73, 0x22, 0xc2, 0x02, 0x0a, 0x10,
|
|
0x42, 0x61, 0x6e, 0x64, 0x77, 0x69, 0x64, 0x74, 0x68, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73,
|
|
0x12, 0x22, 0x0a, 0x0c, 0x62, 0x6f, 0x72, 0x73, 0x68, 0x42, 0x79, 0x74, 0x65, 0x73, 0x54, 0x78,
|
|
0x18, 0x3d, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0c, 0x62, 0x6f, 0x72, 0x73, 0x68, 0x42, 0x79, 0x74,
|
|
0x65, 0x73, 0x54, 0x78, 0x12, 0x22, 0x0a, 0x0c, 0x62, 0x6f, 0x72, 0x73, 0x68, 0x42, 0x79, 0x74,
|
|
0x65, 0x73, 0x52, 0x78, 0x18, 0x3e, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0c, 0x62, 0x6f, 0x72, 0x73,
|
|
0x68, 0x42, 0x79, 0x74, 0x65, 0x73, 0x52, 0x78, 0x12, 0x20, 0x0a, 0x0b, 0x6a, 0x73, 0x6f, 0x6e,
|
|
0x42, 0x79, 0x74, 0x65, 0x73, 0x54, 0x78, 0x18, 0x3f, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b, 0x6a,
|
|
0x73, 0x6f, 0x6e, 0x42, 0x79, 0x74, 0x65, 0x73, 0x54, 0x78, 0x12, 0x20, 0x0a, 0x0b, 0x6a, 0x73,
|
|
0x6f, 0x6e, 0x42, 0x79, 0x74, 0x65, 0x73, 0x52, 0x78, 0x18, 0x40, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x0b, 0x6a, 0x73, 0x6f, 0x6e, 0x42, 0x79, 0x74, 0x65, 0x73, 0x52, 0x78, 0x12, 0x26, 0x0a, 0x0e,
|
|
0x67, 0x72, 0x70, 0x63, 0x50, 0x32, 0x70, 0x42, 0x79, 0x74, 0x65, 0x73, 0x54, 0x78, 0x18, 0x41,
|
|
0x20, 0x01, 0x28, 0x04, 0x52, 0x0e, 0x67, 0x72, 0x70, 0x63, 0x50, 0x32, 0x70, 0x42, 0x79, 0x74,
|
|
0x65, 0x73, 0x54, 0x78, 0x12, 0x26, 0x0a, 0x0e, 0x67, 0x72, 0x70, 0x63, 0x50, 0x32, 0x70, 0x42,
|
|
0x79, 0x74, 0x65, 0x73, 0x52, 0x78, 0x18, 0x42, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0e, 0x67, 0x72,
|
|
0x70, 0x63, 0x50, 0x32, 0x70, 0x42, 0x79, 0x74, 0x65, 0x73, 0x52, 0x78, 0x12, 0x28, 0x0a, 0x0f,
|
|
0x67, 0x72, 0x70, 0x63, 0x55, 0x73, 0x65, 0x72, 0x42, 0x79, 0x74, 0x65, 0x73, 0x54, 0x78, 0x18,
|
|
0x43, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0f, 0x67, 0x72, 0x70, 0x63, 0x55, 0x73, 0x65, 0x72, 0x42,
|
|
0x79, 0x74, 0x65, 0x73, 0x54, 0x78, 0x12, 0x28, 0x0a, 0x0f, 0x67, 0x72, 0x70, 0x63, 0x55, 0x73,
|
|
0x65, 0x72, 0x42, 0x79, 0x74, 0x65, 0x73, 0x52, 0x78, 0x18, 0x44, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x0f, 0x67, 0x72, 0x70, 0x63, 0x55, 0x73, 0x65, 0x72, 0x42, 0x79, 0x74, 0x65, 0x73, 0x52, 0x78,
|
|
0x22, 0xc2, 0x04, 0x0a, 0x10, 0x43, 0x6f, 0x6e, 0x73, 0x65, 0x6e, 0x73, 0x75, 0x73, 0x4d, 0x65,
|
|
0x74, 0x72, 0x69, 0x63, 0x73, 0x12, 0x28, 0x0a, 0x0f, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x53,
|
|
0x75, 0x62, 0x6d, 0x69, 0x74, 0x74, 0x65, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0f,
|
|
0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x73, 0x53, 0x75, 0x62, 0x6d, 0x69, 0x74, 0x74, 0x65, 0x64, 0x12,
|
|
0x22, 0x0a, 0x0c, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x73, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0c, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x43, 0x6f, 0x75,
|
|
0x6e, 0x74, 0x73, 0x12, 0x1c, 0x0a, 0x09, 0x64, 0x65, 0x70, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x73,
|
|
0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x09, 0x64, 0x65, 0x70, 0x43, 0x6f, 0x75, 0x6e, 0x74,
|
|
0x73, 0x12, 0x1e, 0x0a, 0x0a, 0x62, 0x6f, 0x64, 0x79, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x73, 0x18,
|
|
0x04, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0a, 0x62, 0x6f, 0x64, 0x79, 0x43, 0x6f, 0x75, 0x6e, 0x74,
|
|
0x73, 0x12, 0x1c, 0x0a, 0x09, 0x74, 0x78, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x73, 0x18, 0x05,
|
|
0x20, 0x01, 0x28, 0x04, 0x52, 0x09, 0x74, 0x78, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x73, 0x12,
|
|
0x2a, 0x0a, 0x10, 0x63, 0x68, 0x61, 0x69, 0x6e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x43, 0x6f, 0x75,
|
|
0x6e, 0x74, 0x73, 0x18, 0x06, 0x20, 0x01, 0x28, 0x04, 0x52, 0x10, 0x63, 0x68, 0x61, 0x69, 0x6e,
|
|
0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x73, 0x12, 0x1e, 0x0a, 0x0a, 0x6d,
|
|
0x61, 0x73, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x73, 0x18, 0x07, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x0a, 0x6d, 0x61, 0x73, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x73, 0x12, 0x1e, 0x0a, 0x0a, 0x62,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x0a, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x20, 0x0a, 0x0b, 0x68,
|
|
0x65, 0x61, 0x64, 0x65, 0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x0c, 0x20, 0x01, 0x28, 0x04,
|
|
0x52, 0x0b, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x20, 0x0a,
|
|
0x0b, 0x6d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x53, 0x69, 0x7a, 0x65, 0x18, 0x0d, 0x20, 0x01,
|
|
0x28, 0x04, 0x52, 0x0b, 0x6d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x53, 0x69, 0x7a, 0x65, 0x12,
|
|
0x26, 0x0a, 0x0e, 0x74, 0x69, 0x70, 0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x43, 0x6f, 0x75, 0x6e,
|
|
0x74, 0x18, 0x0e, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0e, 0x74, 0x69, 0x70, 0x48, 0x61, 0x73, 0x68,
|
|
0x65, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x1e, 0x0a, 0x0a, 0x64, 0x69, 0x66, 0x66, 0x69,
|
|
0x63, 0x75, 0x6c, 0x74, 0x79, 0x18, 0x0f, 0x20, 0x01, 0x28, 0x01, 0x52, 0x0a, 0x64, 0x69, 0x66,
|
|
0x66, 0x69, 0x63, 0x75, 0x6c, 0x74, 0x79, 0x12, 0x26, 0x0a, 0x0e, 0x70, 0x61, 0x73, 0x74, 0x4d,
|
|
0x65, 0x64, 0x69, 0x61, 0x6e, 0x54, 0x69, 0x6d, 0x65, 0x18, 0x10, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x0e, 0x70, 0x61, 0x73, 0x74, 0x4d, 0x65, 0x64, 0x69, 0x61, 0x6e, 0x54, 0x69, 0x6d, 0x65, 0x12,
|
|
0x3a, 0x0a, 0x18, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74,
|
|
0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x11, 0x20, 0x01, 0x28,
|
|
0x0d, 0x52, 0x18, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74,
|
|
0x48, 0x61, 0x73, 0x68, 0x65, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x28, 0x0a, 0x0f, 0x76,
|
|
0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x12,
|
|
0x20, 0x01, 0x28, 0x04, 0x52, 0x0f, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x44, 0x61, 0x61,
|
|
0x53, 0x63, 0x6f, 0x72, 0x65, 0x22, 0x3c, 0x0a, 0x0e, 0x53, 0x74, 0x6f, 0x72, 0x61, 0x67, 0x65,
|
|
0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x12, 0x2a, 0x0a, 0x10, 0x73, 0x74, 0x6f, 0x72, 0x61,
|
|
0x67, 0x65, 0x53, 0x69, 0x7a, 0x65, 0x42, 0x79, 0x74, 0x65, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x10, 0x73, 0x74, 0x6f, 0x72, 0x61, 0x67, 0x65, 0x53, 0x69, 0x7a, 0x65, 0x42, 0x79,
|
|
0x74, 0x65, 0x73, 0x22, 0x4e, 0x0a, 0x1c, 0x47, 0x65, 0x74, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73,
|
|
0x61, 0x67, 0x65, 0x12, 0x2e, 0x0a, 0x12, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x50, 0x72,
|
|
0x6f, 0x66, 0x69, 0x6c, 0x65, 0x44, 0x61, 0x74, 0x61, 0x18, 0x01, 0x20, 0x01, 0x28, 0x08, 0x52,
|
|
0x12, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x50, 0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x44,
|
|
0x61, 0x74, 0x61, 0x22, 0x56, 0x0a, 0x16, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x73, 0x50, 0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x44, 0x61, 0x74, 0x61, 0x12, 0x1a, 0x0a,
|
|
0x08, 0x63, 0x70, 0x75, 0x55, 0x73, 0x61, 0x67, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x01, 0x52,
|
|
0x08, 0x63, 0x70, 0x75, 0x55, 0x73, 0x61, 0x67, 0x65, 0x12, 0x20, 0x0a, 0x0b, 0x6d, 0x65, 0x6d,
|
|
0x6f, 0x72, 0x79, 0x55, 0x73, 0x61, 0x67, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b,
|
|
0x6d, 0x65, 0x6d, 0x6f, 0x72, 0x79, 0x55, 0x73, 0x61, 0x67, 0x65, 0x22, 0xc0, 0x01, 0x0a, 0x1d,
|
|
0x47, 0x65, 0x74, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x52, 0x65,
|
|
0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x18, 0x0a,
|
|
0x07, 0x63, 0x6c, 0x69, 0x65, 0x6e, 0x74, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x07,
|
|
0x63, 0x6c, 0x69, 0x65, 0x6e, 0x74, 0x73, 0x12, 0x14, 0x0a, 0x05, 0x70, 0x65, 0x65, 0x72, 0x73,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x05, 0x70, 0x65, 0x65, 0x72, 0x73, 0x12, 0x43, 0x0a,
|
|
0x0b, 0x70, 0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x44, 0x61, 0x74, 0x61, 0x18, 0x03, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x21, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x43,
|
|
0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x50, 0x72, 0x6f, 0x66, 0x69, 0x6c,
|
|
0x65, 0x44, 0x61, 0x74, 0x61, 0x52, 0x0b, 0x70, 0x72, 0x6f, 0x66, 0x69, 0x6c, 0x65, 0x44, 0x61,
|
|
0x74, 0x61, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52,
|
|
0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x1d,
|
|
0x0a, 0x1b, 0x47, 0x65, 0x74, 0x53, 0x79, 0x73, 0x74, 0x65, 0x6d, 0x49, 0x6e, 0x66, 0x6f, 0x52,
|
|
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0xf0, 0x01,
|
|
0x0a, 0x1c, 0x47, 0x65, 0x74, 0x53, 0x79, 0x73, 0x74, 0x65, 0x6d, 0x49, 0x6e, 0x66, 0x6f, 0x52,
|
|
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x18,
|
|
0x0a, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x79, 0x73, 0x74,
|
|
0x65, 0x6d, 0x49, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x73, 0x79, 0x73, 0x74,
|
|
0x65, 0x6d, 0x49, 0x64, 0x12, 0x18, 0x0a, 0x07, 0x67, 0x69, 0x74, 0x48, 0x61, 0x73, 0x68, 0x18,
|
|
0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x67, 0x69, 0x74, 0x48, 0x61, 0x73, 0x68, 0x12, 0x18,
|
|
0x0a, 0x07, 0x63, 0x6f, 0x72, 0x65, 0x4e, 0x75, 0x6d, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0d, 0x52,
|
|
0x07, 0x63, 0x6f, 0x72, 0x65, 0x4e, 0x75, 0x6d, 0x12, 0x20, 0x0a, 0x0b, 0x74, 0x6f, 0x74, 0x61,
|
|
0x6c, 0x4d, 0x65, 0x6d, 0x6f, 0x72, 0x79, 0x18, 0x05, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b, 0x74,
|
|
0x6f, 0x74, 0x61, 0x6c, 0x4d, 0x65, 0x6d, 0x6f, 0x72, 0x79, 0x12, 0x18, 0x0a, 0x07, 0x66, 0x64,
|
|
0x4c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x07, 0x66, 0x64, 0x4c,
|
|
0x69, 0x6d, 0x69, 0x74, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65,
|
|
0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72,
|
|
0x22, 0x96, 0x02, 0x0a, 0x18, 0x47, 0x65, 0x74, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x52,
|
|
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x26, 0x0a,
|
|
0x0e, 0x70, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0e, 0x70, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x4d, 0x65,
|
|
0x74, 0x72, 0x69, 0x63, 0x73, 0x12, 0x2c, 0x0a, 0x11, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08,
|
|
0x52, 0x11, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x74, 0x72,
|
|
0x69, 0x63, 0x73, 0x12, 0x2a, 0x0a, 0x10, 0x62, 0x61, 0x6e, 0x64, 0x77, 0x69, 0x64, 0x74, 0x68,
|
|
0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x10, 0x62,
|
|
0x61, 0x6e, 0x64, 0x77, 0x69, 0x64, 0x74, 0x68, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x12,
|
|
0x2a, 0x0a, 0x10, 0x63, 0x6f, 0x6e, 0x73, 0x65, 0x6e, 0x73, 0x75, 0x73, 0x4d, 0x65, 0x74, 0x72,
|
|
0x69, 0x63, 0x73, 0x18, 0x04, 0x20, 0x01, 0x28, 0x08, 0x52, 0x10, 0x63, 0x6f, 0x6e, 0x73, 0x65,
|
|
0x6e, 0x73, 0x75, 0x73, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x12, 0x26, 0x0a, 0x0e, 0x73,
|
|
0x74, 0x6f, 0x72, 0x61, 0x67, 0x65, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x18, 0x05, 0x20,
|
|
0x01, 0x28, 0x08, 0x52, 0x0e, 0x73, 0x74, 0x6f, 0x72, 0x61, 0x67, 0x65, 0x4d, 0x65, 0x74, 0x72,
|
|
0x69, 0x63, 0x73, 0x12, 0x24, 0x0a, 0x0d, 0x63, 0x75, 0x73, 0x74, 0x6f, 0x6d, 0x4d, 0x65, 0x74,
|
|
0x72, 0x69, 0x63, 0x73, 0x18, 0x06, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0d, 0x63, 0x75, 0x73, 0x74,
|
|
0x6f, 0x6d, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x22, 0xcb, 0x03, 0x0a, 0x19, 0x47, 0x65,
|
|
0x74, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x73, 0x65, 0x72, 0x76, 0x65,
|
|
0x72, 0x54, 0x69, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0a, 0x73, 0x65, 0x72,
|
|
0x76, 0x65, 0x72, 0x54, 0x69, 0x6d, 0x65, 0x12, 0x41, 0x0a, 0x0e, 0x70, 0x72, 0x6f, 0x63, 0x65,
|
|
0x73, 0x73, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x50, 0x72, 0x6f, 0x63,
|
|
0x65, 0x73, 0x73, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x52, 0x0e, 0x70, 0x72, 0x6f, 0x63,
|
|
0x65, 0x73, 0x73, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x12, 0x4a, 0x0a, 0x11, 0x63, 0x6f,
|
|
0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x18,
|
|
0x0c, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x43, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x74, 0x72,
|
|
0x69, 0x63, 0x73, 0x52, 0x11, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x4d,
|
|
0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x12, 0x47, 0x0a, 0x10, 0x62, 0x61, 0x6e, 0x64, 0x77, 0x69,
|
|
0x64, 0x74, 0x68, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x18, 0x0d, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x1b, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x42, 0x61, 0x6e,
|
|
0x64, 0x77, 0x69, 0x64, 0x74, 0x68, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x52, 0x10, 0x62,
|
|
0x61, 0x6e, 0x64, 0x77, 0x69, 0x64, 0x74, 0x68, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x12,
|
|
0x47, 0x0a, 0x10, 0x63, 0x6f, 0x6e, 0x73, 0x65, 0x6e, 0x73, 0x75, 0x73, 0x4d, 0x65, 0x74, 0x72,
|
|
0x69, 0x63, 0x73, 0x18, 0x0e, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x43, 0x6f, 0x6e, 0x73, 0x65, 0x6e, 0x73, 0x75, 0x73, 0x4d,
|
|
0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x52, 0x10, 0x63, 0x6f, 0x6e, 0x73, 0x65, 0x6e, 0x73, 0x75,
|
|
0x73, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x12, 0x41, 0x0a, 0x0e, 0x73, 0x74, 0x6f, 0x72,
|
|
0x61, 0x67, 0x65, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x18, 0x0f, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x53, 0x74, 0x6f,
|
|
0x72, 0x61, 0x67, 0x65, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x52, 0x0e, 0x73, 0x74, 0x6f,
|
|
0x72, 0x61, 0x67, 0x65, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x73, 0x12, 0x2a, 0x0a, 0x05, 0x65,
|
|
0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72,
|
|
0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x1d, 0x0a, 0x1b, 0x47, 0x65, 0x74, 0x53, 0x65,
|
|
0x72, 0x76, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0xc6, 0x02, 0x0a, 0x1c, 0x47, 0x65, 0x74, 0x53, 0x65,
|
|
0x72, 0x76, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x24, 0x0a, 0x0d, 0x72, 0x70, 0x63, 0x41, 0x70,
|
|
0x69, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0d,
|
|
0x72, 0x70, 0x63, 0x41, 0x70, 0x69, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x26, 0x0a,
|
|
0x0e, 0x72, 0x70, 0x63, 0x41, 0x70, 0x69, 0x52, 0x65, 0x76, 0x69, 0x73, 0x69, 0x6f, 0x6e, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0e, 0x72, 0x70, 0x63, 0x41, 0x70, 0x69, 0x52, 0x65, 0x76,
|
|
0x69, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x24, 0x0a, 0x0d, 0x73, 0x65, 0x72, 0x76, 0x65, 0x72, 0x56,
|
|
0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0d, 0x73, 0x65,
|
|
0x72, 0x76, 0x65, 0x72, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x1c, 0x0a, 0x09, 0x6e,
|
|
0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09,
|
|
0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x64, 0x12, 0x22, 0x0a, 0x0c, 0x68, 0x61, 0x73,
|
|
0x55, 0x74, 0x78, 0x6f, 0x49, 0x6e, 0x64, 0x65, 0x78, 0x18, 0x05, 0x20, 0x01, 0x28, 0x08, 0x52,
|
|
0x0c, 0x68, 0x61, 0x73, 0x55, 0x74, 0x78, 0x6f, 0x49, 0x6e, 0x64, 0x65, 0x78, 0x12, 0x1a, 0x0a,
|
|
0x08, 0x69, 0x73, 0x53, 0x79, 0x6e, 0x63, 0x65, 0x64, 0x18, 0x06, 0x20, 0x01, 0x28, 0x08, 0x52,
|
|
0x08, 0x69, 0x73, 0x53, 0x79, 0x6e, 0x63, 0x65, 0x64, 0x12, 0x28, 0x0a, 0x0f, 0x76, 0x69, 0x72,
|
|
0x74, 0x75, 0x61, 0x6c, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x07, 0x20, 0x01,
|
|
0x28, 0x04, 0x52, 0x0f, 0x76, 0x69, 0x72, 0x74, 0x75, 0x61, 0x6c, 0x44, 0x61, 0x61, 0x53, 0x63,
|
|
0x6f, 0x72, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e,
|
|
0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22,
|
|
0x1d, 0x0a, 0x1b, 0x47, 0x65, 0x74, 0x53, 0x79, 0x6e, 0x63, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x66,
|
|
0x0a, 0x1c, 0x47, 0x65, 0x74, 0x53, 0x79, 0x6e, 0x63, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x52,
|
|
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x1a,
|
|
0x0a, 0x08, 0x69, 0x73, 0x53, 0x79, 0x6e, 0x63, 0x65, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x08,
|
|
0x52, 0x08, 0x69, 0x73, 0x53, 0x79, 0x6e, 0x63, 0x65, 0x64, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72,
|
|
0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52,
|
|
0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x4a, 0x0a, 0x2a, 0x47, 0x65, 0x74, 0x44, 0x61, 0x61,
|
|
0x53, 0x63, 0x6f, 0x72, 0x65, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x45, 0x73,
|
|
0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73,
|
|
0x73, 0x61, 0x67, 0x65, 0x12, 0x1c, 0x0a, 0x09, 0x64, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72, 0x65,
|
|
0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x04, 0x52, 0x09, 0x64, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72,
|
|
0x65, 0x73, 0x22, 0x79, 0x0a, 0x2b, 0x47, 0x65, 0x74, 0x44, 0x61, 0x61, 0x53, 0x63, 0x6f, 0x72,
|
|
0x65, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x45, 0x73, 0x74, 0x69, 0x6d, 0x61,
|
|
0x74, 0x65, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67,
|
|
0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x73, 0x18,
|
|
0x01, 0x20, 0x03, 0x28, 0x04, 0x52, 0x0a, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70,
|
|
0x73, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50,
|
|
0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x58, 0x0a,
|
|
0x10, 0x52, 0x70, 0x63, 0x46, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x42, 0x75, 0x63, 0x6b, 0x65,
|
|
0x74, 0x12, 0x18, 0x0a, 0x07, 0x66, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x01, 0x52, 0x07, 0x66, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x12, 0x2a, 0x0a, 0x10, 0x65,
|
|
0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x64, 0x53, 0x65, 0x63, 0x6f, 0x6e, 0x64, 0x73, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x01, 0x52, 0x10, 0x65, 0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x64,
|
|
0x53, 0x65, 0x63, 0x6f, 0x6e, 0x64, 0x73, 0x22, 0xd6, 0x01, 0x0a, 0x0e, 0x52, 0x70, 0x63, 0x46,
|
|
0x65, 0x65, 0x45, 0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x12, 0x44, 0x0a, 0x0f, 0x70, 0x72,
|
|
0x69, 0x6f, 0x72, 0x69, 0x74, 0x79, 0x5f, 0x62, 0x75, 0x63, 0x6b, 0x65, 0x74, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e,
|
|
0x52, 0x70, 0x63, 0x46, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x42, 0x75, 0x63, 0x6b, 0x65, 0x74,
|
|
0x52, 0x0e, 0x70, 0x72, 0x69, 0x6f, 0x72, 0x69, 0x74, 0x79, 0x42, 0x75, 0x63, 0x6b, 0x65, 0x74,
|
|
0x12, 0x41, 0x0a, 0x0d, 0x6e, 0x6f, 0x72, 0x6d, 0x61, 0x6c, 0x42, 0x75, 0x63, 0x6b, 0x65, 0x74,
|
|
0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x46, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x42, 0x75,
|
|
0x63, 0x6b, 0x65, 0x74, 0x52, 0x0d, 0x6e, 0x6f, 0x72, 0x6d, 0x61, 0x6c, 0x42, 0x75, 0x63, 0x6b,
|
|
0x65, 0x74, 0x73, 0x12, 0x3b, 0x0a, 0x0a, 0x6c, 0x6f, 0x77, 0x42, 0x75, 0x63, 0x6b, 0x65, 0x74,
|
|
0x73, 0x18, 0x03, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x46, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x42, 0x75,
|
|
0x63, 0x6b, 0x65, 0x74, 0x52, 0x0a, 0x6c, 0x6f, 0x77, 0x42, 0x75, 0x63, 0x6b, 0x65, 0x74, 0x73,
|
|
0x22, 0xbb, 0x03, 0x0a, 0x25, 0x52, 0x70, 0x63, 0x46, 0x65, 0x65, 0x45, 0x73, 0x74, 0x69, 0x6d,
|
|
0x61, 0x74, 0x65, 0x56, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x45, 0x78, 0x70, 0x65, 0x72, 0x69,
|
|
0x6d, 0x65, 0x6e, 0x74, 0x61, 0x6c, 0x44, 0x61, 0x74, 0x61, 0x12, 0x44, 0x0a, 0x1d, 0x6d, 0x65,
|
|
0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x52, 0x65, 0x61, 0x64, 0x79, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61,
|
|
0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x1d, 0x6d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x52, 0x65, 0x61, 0x64, 0x79, 0x54,
|
|
0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x43, 0x6f, 0x75, 0x6e, 0x74,
|
|
0x12, 0x4c, 0x0a, 0x21, 0x6d, 0x65, 0x6d, 0x70, 0x6f, 0x6f, 0x6c, 0x52, 0x65, 0x61, 0x64, 0x79,
|
|
0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x54, 0x6f, 0x74, 0x61,
|
|
0x6c, 0x4d, 0x61, 0x73, 0x73, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x21, 0x6d, 0x65, 0x6d,
|
|
0x70, 0x6f, 0x6f, 0x6c, 0x52, 0x65, 0x61, 0x64, 0x79, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x73, 0x54, 0x6f, 0x74, 0x61, 0x6c, 0x4d, 0x61, 0x73, 0x73, 0x12, 0x32,
|
|
0x0a, 0x14, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x4d, 0x61, 0x73, 0x73, 0x50, 0x65, 0x72,
|
|
0x53, 0x65, 0x63, 0x6f, 0x6e, 0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x14, 0x6e, 0x65,
|
|
0x74, 0x77, 0x6f, 0x72, 0x6b, 0x4d, 0x61, 0x73, 0x73, 0x50, 0x65, 0x72, 0x53, 0x65, 0x63, 0x6f,
|
|
0x6e, 0x64, 0x12, 0x40, 0x0a, 0x1b, 0x6e, 0x65, 0x78, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x54,
|
|
0x65, 0x6d, 0x70, 0x6c, 0x61, 0x74, 0x65, 0x46, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x4d, 0x69,
|
|
0x6e, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x01, 0x52, 0x1b, 0x6e, 0x65, 0x78, 0x74, 0x42, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x54, 0x65, 0x6d, 0x70, 0x6c, 0x61, 0x74, 0x65, 0x46, 0x65, 0x65, 0x72, 0x61, 0x74,
|
|
0x65, 0x4d, 0x69, 0x6e, 0x12, 0x46, 0x0a, 0x1e, 0x6e, 0x65, 0x78, 0x74, 0x42, 0x6c, 0x6f, 0x63,
|
|
0x6b, 0x54, 0x65, 0x6d, 0x70, 0x6c, 0x61, 0x74, 0x65, 0x46, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65,
|
|
0x4d, 0x65, 0x64, 0x69, 0x61, 0x6e, 0x18, 0x0c, 0x20, 0x01, 0x28, 0x01, 0x52, 0x1e, 0x6e, 0x65,
|
|
0x78, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x65, 0x6d, 0x70, 0x6c, 0x61, 0x74, 0x65, 0x46,
|
|
0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x4d, 0x65, 0x64, 0x69, 0x61, 0x6e, 0x12, 0x40, 0x0a, 0x1b,
|
|
0x6e, 0x65, 0x78, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x65, 0x6d, 0x70, 0x6c, 0x61, 0x74,
|
|
0x65, 0x46, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x4d, 0x61, 0x78, 0x18, 0x0d, 0x20, 0x01, 0x28,
|
|
0x01, 0x52, 0x1b, 0x6e, 0x65, 0x78, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x54, 0x65, 0x6d, 0x70,
|
|
0x6c, 0x61, 0x74, 0x65, 0x46, 0x65, 0x65, 0x72, 0x61, 0x74, 0x65, 0x4d, 0x61, 0x78, 0x22, 0x1e,
|
|
0x0a, 0x1c, 0x47, 0x65, 0x74, 0x46, 0x65, 0x65, 0x45, 0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x22, 0x82,
|
|
0x01, 0x0a, 0x1d, 0x47, 0x65, 0x74, 0x46, 0x65, 0x65, 0x45, 0x73, 0x74, 0x69, 0x6d, 0x61, 0x74,
|
|
0x65, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x12, 0x35, 0x0a, 0x08, 0x65, 0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52,
|
|
0x70, 0x63, 0x46, 0x65, 0x65, 0x45, 0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x52, 0x08, 0x65,
|
|
0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72,
|
|
0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77,
|
|
0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72,
|
|
0x72, 0x6f, 0x72, 0x22, 0x44, 0x0a, 0x28, 0x47, 0x65, 0x74, 0x46, 0x65, 0x65, 0x45, 0x73, 0x74,
|
|
0x69, 0x6d, 0x61, 0x74, 0x65, 0x45, 0x78, 0x70, 0x65, 0x72, 0x69, 0x6d, 0x65, 0x6e, 0x74, 0x61,
|
|
0x6c, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x18, 0x0a, 0x07, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x08,
|
|
0x52, 0x07, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x22, 0xda, 0x01, 0x0a, 0x29, 0x47, 0x65,
|
|
0x74, 0x46, 0x65, 0x65, 0x45, 0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x45, 0x78, 0x70, 0x65,
|
|
0x72, 0x69, 0x6d, 0x65, 0x6e, 0x74, 0x61, 0x6c, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x35, 0x0a, 0x08, 0x65, 0x73, 0x74, 0x69, 0x6d,
|
|
0x61, 0x74, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x46, 0x65, 0x65, 0x45, 0x73, 0x74, 0x69,
|
|
0x6d, 0x61, 0x74, 0x65, 0x52, 0x08, 0x65, 0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x12, 0x4a,
|
|
0x0a, 0x07, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x30, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x46,
|
|
0x65, 0x65, 0x45, 0x73, 0x74, 0x69, 0x6d, 0x61, 0x74, 0x65, 0x56, 0x65, 0x72, 0x62, 0x6f, 0x73,
|
|
0x65, 0x45, 0x78, 0x70, 0x65, 0x72, 0x69, 0x6d, 0x65, 0x6e, 0x74, 0x61, 0x6c, 0x44, 0x61, 0x74,
|
|
0x61, 0x52, 0x07, 0x76, 0x65, 0x72, 0x62, 0x6f, 0x73, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72,
|
|
0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52,
|
|
0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x38, 0x0a, 0x22, 0x47, 0x65, 0x74, 0x43, 0x75, 0x72,
|
|
0x72, 0x65, 0x6e, 0x74, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x43, 0x6f, 0x6c, 0x6f, 0x72, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x12, 0x0a, 0x04,
|
|
0x68, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x68, 0x61, 0x73, 0x68,
|
|
0x22, 0x65, 0x0a, 0x23, 0x47, 0x65, 0x74, 0x43, 0x75, 0x72, 0x72, 0x65, 0x6e, 0x74, 0x42, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x43, 0x6f, 0x6c, 0x6f, 0x72, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x62, 0x6c, 0x75, 0x65, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x08, 0x52, 0x04, 0x62, 0x6c, 0x75, 0x65, 0x12, 0x2a, 0x0a, 0x05, 0x65,
|
|
0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72,
|
|
0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x22, 0x69, 0x0a, 0x2a, 0x53, 0x75, 0x62, 0x6d, 0x69,
|
|
0x74, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x70, 0x6c,
|
|
0x61, 0x63, 0x65, 0x6d, 0x65, 0x6e, 0x74, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x3b, 0x0a, 0x0b, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x2e, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61,
|
|
0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x0b, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x22, 0xcc, 0x01, 0x0a, 0x2b, 0x53, 0x75, 0x62, 0x6d, 0x69, 0x74, 0x54, 0x72, 0x61,
|
|
0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x70, 0x6c, 0x61, 0x63, 0x65, 0x6d,
|
|
0x65, 0x6e, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61,
|
|
0x67, 0x65, 0x12, 0x24, 0x0a, 0x0d, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x49, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0d, 0x74, 0x72, 0x61, 0x6e, 0x73,
|
|
0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x64, 0x12, 0x4b, 0x0a, 0x13, 0x72, 0x65, 0x70, 0x6c,
|
|
0x61, 0x63, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x52, 0x70, 0x63, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x52, 0x13, 0x72, 0x65, 0x70, 0x6c, 0x61, 0x63, 0x65, 0x64, 0x54, 0x72, 0x61, 0x6e, 0x73, 0x61,
|
|
0x63, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x2a, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0xe8,
|
|
0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x13, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72,
|
|
0x65, 0x2e, 0x52, 0x50, 0x43, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f,
|
|
0x72, 0x42, 0x26, 0x5a, 0x24, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f,
|
|
0x6b, 0x61, 0x73, 0x70, 0x61, 0x6e, 0x65, 0x74, 0x2f, 0x6b, 0x61, 0x73, 0x70, 0x61, 0x64, 0x2f,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x77, 0x69, 0x72, 0x65, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x33,
|
|
}
|
|
|
|
var (
|
|
file_rpc_proto_rawDescOnce sync.Once
|
|
file_rpc_proto_rawDescData = file_rpc_proto_rawDesc
|
|
)
|
|
|
|
func file_rpc_proto_rawDescGZIP() []byte {
|
|
file_rpc_proto_rawDescOnce.Do(func() {
|
|
file_rpc_proto_rawDescData = protoimpl.X.CompressGZIP(file_rpc_proto_rawDescData)
|
|
})
|
|
return file_rpc_proto_rawDescData
|
|
}
|
|
|
|
var file_rpc_proto_enumTypes = make([]protoimpl.EnumInfo, 1)
|
|
var file_rpc_proto_msgTypes = make([]protoimpl.MessageInfo, 139)
|
|
var file_rpc_proto_goTypes = []any{
|
|
(SubmitBlockResponseMessage_RejectReason)(0), // 0: protowire.SubmitBlockResponseMessage.RejectReason
|
|
(*RPCError)(nil), // 1: protowire.RPCError
|
|
(*RpcBlock)(nil), // 2: protowire.RpcBlock
|
|
(*RpcBlockHeader)(nil), // 3: protowire.RpcBlockHeader
|
|
(*RpcBlockLevelParents)(nil), // 4: protowire.RpcBlockLevelParents
|
|
(*RpcBlockVerboseData)(nil), // 5: protowire.RpcBlockVerboseData
|
|
(*RpcTransaction)(nil), // 6: protowire.RpcTransaction
|
|
(*RpcTransactionInput)(nil), // 7: protowire.RpcTransactionInput
|
|
(*RpcScriptPublicKey)(nil), // 8: protowire.RpcScriptPublicKey
|
|
(*RpcTransactionOutput)(nil), // 9: protowire.RpcTransactionOutput
|
|
(*RpcOutpoint)(nil), // 10: protowire.RpcOutpoint
|
|
(*RpcUtxoEntry)(nil), // 11: protowire.RpcUtxoEntry
|
|
(*RpcTransactionVerboseData)(nil), // 12: protowire.RpcTransactionVerboseData
|
|
(*RpcTransactionInputVerboseData)(nil), // 13: protowire.RpcTransactionInputVerboseData
|
|
(*RpcTransactionOutputVerboseData)(nil), // 14: protowire.RpcTransactionOutputVerboseData
|
|
(*GetCurrentNetworkRequestMessage)(nil), // 15: protowire.GetCurrentNetworkRequestMessage
|
|
(*GetCurrentNetworkResponseMessage)(nil), // 16: protowire.GetCurrentNetworkResponseMessage
|
|
(*SubmitBlockRequestMessage)(nil), // 17: protowire.SubmitBlockRequestMessage
|
|
(*SubmitBlockResponseMessage)(nil), // 18: protowire.SubmitBlockResponseMessage
|
|
(*GetBlockTemplateRequestMessage)(nil), // 19: protowire.GetBlockTemplateRequestMessage
|
|
(*GetBlockTemplateResponseMessage)(nil), // 20: protowire.GetBlockTemplateResponseMessage
|
|
(*NotifyBlockAddedRequestMessage)(nil), // 21: protowire.NotifyBlockAddedRequestMessage
|
|
(*NotifyBlockAddedResponseMessage)(nil), // 22: protowire.NotifyBlockAddedResponseMessage
|
|
(*BlockAddedNotificationMessage)(nil), // 23: protowire.BlockAddedNotificationMessage
|
|
(*GetPeerAddressesRequestMessage)(nil), // 24: protowire.GetPeerAddressesRequestMessage
|
|
(*GetPeerAddressesResponseMessage)(nil), // 25: protowire.GetPeerAddressesResponseMessage
|
|
(*GetPeerAddressesKnownAddressMessage)(nil), // 26: protowire.GetPeerAddressesKnownAddressMessage
|
|
(*GetSelectedTipHashRequestMessage)(nil), // 27: protowire.GetSelectedTipHashRequestMessage
|
|
(*GetSelectedTipHashResponseMessage)(nil), // 28: protowire.GetSelectedTipHashResponseMessage
|
|
(*GetMempoolEntryRequestMessage)(nil), // 29: protowire.GetMempoolEntryRequestMessage
|
|
(*GetMempoolEntryResponseMessage)(nil), // 30: protowire.GetMempoolEntryResponseMessage
|
|
(*GetMempoolEntriesRequestMessage)(nil), // 31: protowire.GetMempoolEntriesRequestMessage
|
|
(*GetMempoolEntriesResponseMessage)(nil), // 32: protowire.GetMempoolEntriesResponseMessage
|
|
(*MempoolEntry)(nil), // 33: protowire.MempoolEntry
|
|
(*GetConnectedPeerInfoRequestMessage)(nil), // 34: protowire.GetConnectedPeerInfoRequestMessage
|
|
(*GetConnectedPeerInfoResponseMessage)(nil), // 35: protowire.GetConnectedPeerInfoResponseMessage
|
|
(*GetConnectedPeerInfoMessage)(nil), // 36: protowire.GetConnectedPeerInfoMessage
|
|
(*AddPeerRequestMessage)(nil), // 37: protowire.AddPeerRequestMessage
|
|
(*AddPeerResponseMessage)(nil), // 38: protowire.AddPeerResponseMessage
|
|
(*SubmitTransactionRequestMessage)(nil), // 39: protowire.SubmitTransactionRequestMessage
|
|
(*SubmitTransactionResponseMessage)(nil), // 40: protowire.SubmitTransactionResponseMessage
|
|
(*NotifyVirtualSelectedParentChainChangedRequestMessage)(nil), // 41: protowire.NotifyVirtualSelectedParentChainChangedRequestMessage
|
|
(*NotifyVirtualSelectedParentChainChangedResponseMessage)(nil), // 42: protowire.NotifyVirtualSelectedParentChainChangedResponseMessage
|
|
(*VirtualSelectedParentChainChangedNotificationMessage)(nil), // 43: protowire.VirtualSelectedParentChainChangedNotificationMessage
|
|
(*GetBlockRequestMessage)(nil), // 44: protowire.GetBlockRequestMessage
|
|
(*GetBlockResponseMessage)(nil), // 45: protowire.GetBlockResponseMessage
|
|
(*GetSubnetworkRequestMessage)(nil), // 46: protowire.GetSubnetworkRequestMessage
|
|
(*GetSubnetworkResponseMessage)(nil), // 47: protowire.GetSubnetworkResponseMessage
|
|
(*GetVirtualSelectedParentChainFromBlockRequestMessage)(nil), // 48: protowire.GetVirtualSelectedParentChainFromBlockRequestMessage
|
|
(*AcceptedTransactionIds)(nil), // 49: protowire.AcceptedTransactionIds
|
|
(*GetVirtualSelectedParentChainFromBlockResponseMessage)(nil), // 50: protowire.GetVirtualSelectedParentChainFromBlockResponseMessage
|
|
(*GetBlocksRequestMessage)(nil), // 51: protowire.GetBlocksRequestMessage
|
|
(*GetBlocksResponseMessage)(nil), // 52: protowire.GetBlocksResponseMessage
|
|
(*GetBlockCountRequestMessage)(nil), // 53: protowire.GetBlockCountRequestMessage
|
|
(*GetBlockCountResponseMessage)(nil), // 54: protowire.GetBlockCountResponseMessage
|
|
(*GetBlockDagInfoRequestMessage)(nil), // 55: protowire.GetBlockDagInfoRequestMessage
|
|
(*GetBlockDagInfoResponseMessage)(nil), // 56: protowire.GetBlockDagInfoResponseMessage
|
|
(*ResolveFinalityConflictRequestMessage)(nil), // 57: protowire.ResolveFinalityConflictRequestMessage
|
|
(*ResolveFinalityConflictResponseMessage)(nil), // 58: protowire.ResolveFinalityConflictResponseMessage
|
|
(*NotifyFinalityConflictsRequestMessage)(nil), // 59: protowire.NotifyFinalityConflictsRequestMessage
|
|
(*NotifyFinalityConflictsResponseMessage)(nil), // 60: protowire.NotifyFinalityConflictsResponseMessage
|
|
(*FinalityConflictNotificationMessage)(nil), // 61: protowire.FinalityConflictNotificationMessage
|
|
(*FinalityConflictResolvedNotificationMessage)(nil), // 62: protowire.FinalityConflictResolvedNotificationMessage
|
|
(*ShutDownRequestMessage)(nil), // 63: protowire.ShutDownRequestMessage
|
|
(*ShutDownResponseMessage)(nil), // 64: protowire.ShutDownResponseMessage
|
|
(*GetHeadersRequestMessage)(nil), // 65: protowire.GetHeadersRequestMessage
|
|
(*GetHeadersResponseMessage)(nil), // 66: protowire.GetHeadersResponseMessage
|
|
(*NotifyUtxosChangedRequestMessage)(nil), // 67: protowire.NotifyUtxosChangedRequestMessage
|
|
(*NotifyUtxosChangedResponseMessage)(nil), // 68: protowire.NotifyUtxosChangedResponseMessage
|
|
(*UtxosChangedNotificationMessage)(nil), // 69: protowire.UtxosChangedNotificationMessage
|
|
(*UtxosByAddressesEntry)(nil), // 70: protowire.UtxosByAddressesEntry
|
|
(*StopNotifyingUtxosChangedRequestMessage)(nil), // 71: protowire.StopNotifyingUtxosChangedRequestMessage
|
|
(*StopNotifyingUtxosChangedResponseMessage)(nil), // 72: protowire.StopNotifyingUtxosChangedResponseMessage
|
|
(*GetUtxosByAddressesRequestMessage)(nil), // 73: protowire.GetUtxosByAddressesRequestMessage
|
|
(*GetUtxosByAddressesResponseMessage)(nil), // 74: protowire.GetUtxosByAddressesResponseMessage
|
|
(*GetBalanceByAddressRequestMessage)(nil), // 75: protowire.GetBalanceByAddressRequestMessage
|
|
(*GetBalanceByAddressResponseMessage)(nil), // 76: protowire.GetBalanceByAddressResponseMessage
|
|
(*GetBalancesByAddressesRequestMessage)(nil), // 77: protowire.GetBalancesByAddressesRequestMessage
|
|
(*BalancesByAddressEntry)(nil), // 78: protowire.BalancesByAddressEntry
|
|
(*GetBalancesByAddressesResponseMessage)(nil), // 79: protowire.GetBalancesByAddressesResponseMessage
|
|
(*GetVirtualSelectedParentBlueScoreRequestMessage)(nil), // 80: protowire.GetVirtualSelectedParentBlueScoreRequestMessage
|
|
(*GetVirtualSelectedParentBlueScoreResponseMessage)(nil), // 81: protowire.GetVirtualSelectedParentBlueScoreResponseMessage
|
|
(*NotifyVirtualSelectedParentBlueScoreChangedRequestMessage)(nil), // 82: protowire.NotifyVirtualSelectedParentBlueScoreChangedRequestMessage
|
|
(*NotifyVirtualSelectedParentBlueScoreChangedResponseMessage)(nil), // 83: protowire.NotifyVirtualSelectedParentBlueScoreChangedResponseMessage
|
|
(*VirtualSelectedParentBlueScoreChangedNotificationMessage)(nil), // 84: protowire.VirtualSelectedParentBlueScoreChangedNotificationMessage
|
|
(*NotifyVirtualDaaScoreChangedRequestMessage)(nil), // 85: protowire.NotifyVirtualDaaScoreChangedRequestMessage
|
|
(*NotifyVirtualDaaScoreChangedResponseMessage)(nil), // 86: protowire.NotifyVirtualDaaScoreChangedResponseMessage
|
|
(*VirtualDaaScoreChangedNotificationMessage)(nil), // 87: protowire.VirtualDaaScoreChangedNotificationMessage
|
|
(*NotifyPruningPointUTXOSetOverrideRequestMessage)(nil), // 88: protowire.NotifyPruningPointUTXOSetOverrideRequestMessage
|
|
(*NotifyPruningPointUTXOSetOverrideResponseMessage)(nil), // 89: protowire.NotifyPruningPointUTXOSetOverrideResponseMessage
|
|
(*PruningPointUTXOSetOverrideNotificationMessage)(nil), // 90: protowire.PruningPointUTXOSetOverrideNotificationMessage
|
|
(*StopNotifyingPruningPointUTXOSetOverrideRequestMessage)(nil), // 91: protowire.StopNotifyingPruningPointUTXOSetOverrideRequestMessage
|
|
(*StopNotifyingPruningPointUTXOSetOverrideResponseMessage)(nil), // 92: protowire.StopNotifyingPruningPointUTXOSetOverrideResponseMessage
|
|
(*BanRequestMessage)(nil), // 93: protowire.BanRequestMessage
|
|
(*BanResponseMessage)(nil), // 94: protowire.BanResponseMessage
|
|
(*UnbanRequestMessage)(nil), // 95: protowire.UnbanRequestMessage
|
|
(*UnbanResponseMessage)(nil), // 96: protowire.UnbanResponseMessage
|
|
(*GetInfoRequestMessage)(nil), // 97: protowire.GetInfoRequestMessage
|
|
(*GetInfoResponseMessage)(nil), // 98: protowire.GetInfoResponseMessage
|
|
(*EstimateNetworkHashesPerSecondRequestMessage)(nil), // 99: protowire.EstimateNetworkHashesPerSecondRequestMessage
|
|
(*EstimateNetworkHashesPerSecondResponseMessage)(nil), // 100: protowire.EstimateNetworkHashesPerSecondResponseMessage
|
|
(*NotifyNewBlockTemplateRequestMessage)(nil), // 101: protowire.NotifyNewBlockTemplateRequestMessage
|
|
(*NotifyNewBlockTemplateResponseMessage)(nil), // 102: protowire.NotifyNewBlockTemplateResponseMessage
|
|
(*NewBlockTemplateNotificationMessage)(nil), // 103: protowire.NewBlockTemplateNotificationMessage
|
|
(*MempoolEntryByAddress)(nil), // 104: protowire.MempoolEntryByAddress
|
|
(*GetMempoolEntriesByAddressesRequestMessage)(nil), // 105: protowire.GetMempoolEntriesByAddressesRequestMessage
|
|
(*GetMempoolEntriesByAddressesResponseMessage)(nil), // 106: protowire.GetMempoolEntriesByAddressesResponseMessage
|
|
(*GetCoinSupplyRequestMessage)(nil), // 107: protowire.GetCoinSupplyRequestMessage
|
|
(*GetCoinSupplyResponseMessage)(nil), // 108: protowire.GetCoinSupplyResponseMessage
|
|
(*PingRequestMessage)(nil), // 109: protowire.PingRequestMessage
|
|
(*PingResponseMessage)(nil), // 110: protowire.PingResponseMessage
|
|
(*ProcessMetrics)(nil), // 111: protowire.ProcessMetrics
|
|
(*ConnectionMetrics)(nil), // 112: protowire.ConnectionMetrics
|
|
(*BandwidthMetrics)(nil), // 113: protowire.BandwidthMetrics
|
|
(*ConsensusMetrics)(nil), // 114: protowire.ConsensusMetrics
|
|
(*StorageMetrics)(nil), // 115: protowire.StorageMetrics
|
|
(*GetConnectionsRequestMessage)(nil), // 116: protowire.GetConnectionsRequestMessage
|
|
(*ConnectionsProfileData)(nil), // 117: protowire.ConnectionsProfileData
|
|
(*GetConnectionsResponseMessage)(nil), // 118: protowire.GetConnectionsResponseMessage
|
|
(*GetSystemInfoRequestMessage)(nil), // 119: protowire.GetSystemInfoRequestMessage
|
|
(*GetSystemInfoResponseMessage)(nil), // 120: protowire.GetSystemInfoResponseMessage
|
|
(*GetMetricsRequestMessage)(nil), // 121: protowire.GetMetricsRequestMessage
|
|
(*GetMetricsResponseMessage)(nil), // 122: protowire.GetMetricsResponseMessage
|
|
(*GetServerInfoRequestMessage)(nil), // 123: protowire.GetServerInfoRequestMessage
|
|
(*GetServerInfoResponseMessage)(nil), // 124: protowire.GetServerInfoResponseMessage
|
|
(*GetSyncStatusRequestMessage)(nil), // 125: protowire.GetSyncStatusRequestMessage
|
|
(*GetSyncStatusResponseMessage)(nil), // 126: protowire.GetSyncStatusResponseMessage
|
|
(*GetDaaScoreTimestampEstimateRequestMessage)(nil), // 127: protowire.GetDaaScoreTimestampEstimateRequestMessage
|
|
(*GetDaaScoreTimestampEstimateResponseMessage)(nil), // 128: protowire.GetDaaScoreTimestampEstimateResponseMessage
|
|
(*RpcFeerateBucket)(nil), // 129: protowire.RpcFeerateBucket
|
|
(*RpcFeeEstimate)(nil), // 130: protowire.RpcFeeEstimate
|
|
(*RpcFeeEstimateVerboseExperimentalData)(nil), // 131: protowire.RpcFeeEstimateVerboseExperimentalData
|
|
(*GetFeeEstimateRequestMessage)(nil), // 132: protowire.GetFeeEstimateRequestMessage
|
|
(*GetFeeEstimateResponseMessage)(nil), // 133: protowire.GetFeeEstimateResponseMessage
|
|
(*GetFeeEstimateExperimentalRequestMessage)(nil), // 134: protowire.GetFeeEstimateExperimentalRequestMessage
|
|
(*GetFeeEstimateExperimentalResponseMessage)(nil), // 135: protowire.GetFeeEstimateExperimentalResponseMessage
|
|
(*GetCurrentBlockColorRequestMessage)(nil), // 136: protowire.GetCurrentBlockColorRequestMessage
|
|
(*GetCurrentBlockColorResponseMessage)(nil), // 137: protowire.GetCurrentBlockColorResponseMessage
|
|
(*SubmitTransactionReplacementRequestMessage)(nil), // 138: protowire.SubmitTransactionReplacementRequestMessage
|
|
(*SubmitTransactionReplacementResponseMessage)(nil), // 139: protowire.SubmitTransactionReplacementResponseMessage
|
|
}
|
|
var file_rpc_proto_depIdxs = []int32{
|
|
3, // 0: protowire.RpcBlock.header:type_name -> protowire.RpcBlockHeader
|
|
6, // 1: protowire.RpcBlock.transactions:type_name -> protowire.RpcTransaction
|
|
5, // 2: protowire.RpcBlock.verboseData:type_name -> protowire.RpcBlockVerboseData
|
|
4, // 3: protowire.RpcBlockHeader.parents:type_name -> protowire.RpcBlockLevelParents
|
|
7, // 4: protowire.RpcTransaction.inputs:type_name -> protowire.RpcTransactionInput
|
|
9, // 5: protowire.RpcTransaction.outputs:type_name -> protowire.RpcTransactionOutput
|
|
12, // 6: protowire.RpcTransaction.verboseData:type_name -> protowire.RpcTransactionVerboseData
|
|
10, // 7: protowire.RpcTransactionInput.previousOutpoint:type_name -> protowire.RpcOutpoint
|
|
13, // 8: protowire.RpcTransactionInput.verboseData:type_name -> protowire.RpcTransactionInputVerboseData
|
|
8, // 9: protowire.RpcTransactionOutput.scriptPublicKey:type_name -> protowire.RpcScriptPublicKey
|
|
14, // 10: protowire.RpcTransactionOutput.verboseData:type_name -> protowire.RpcTransactionOutputVerboseData
|
|
8, // 11: protowire.RpcUtxoEntry.scriptPublicKey:type_name -> protowire.RpcScriptPublicKey
|
|
1, // 12: protowire.GetCurrentNetworkResponseMessage.error:type_name -> protowire.RPCError
|
|
2, // 13: protowire.SubmitBlockRequestMessage.block:type_name -> protowire.RpcBlock
|
|
0, // 14: protowire.SubmitBlockResponseMessage.rejectReason:type_name -> protowire.SubmitBlockResponseMessage.RejectReason
|
|
1, // 15: protowire.SubmitBlockResponseMessage.error:type_name -> protowire.RPCError
|
|
2, // 16: protowire.GetBlockTemplateResponseMessage.block:type_name -> protowire.RpcBlock
|
|
1, // 17: protowire.GetBlockTemplateResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 18: protowire.NotifyBlockAddedResponseMessage.error:type_name -> protowire.RPCError
|
|
2, // 19: protowire.BlockAddedNotificationMessage.block:type_name -> protowire.RpcBlock
|
|
26, // 20: protowire.GetPeerAddressesResponseMessage.addresses:type_name -> protowire.GetPeerAddressesKnownAddressMessage
|
|
26, // 21: protowire.GetPeerAddressesResponseMessage.bannedAddresses:type_name -> protowire.GetPeerAddressesKnownAddressMessage
|
|
1, // 22: protowire.GetPeerAddressesResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 23: protowire.GetSelectedTipHashResponseMessage.error:type_name -> protowire.RPCError
|
|
33, // 24: protowire.GetMempoolEntryResponseMessage.entry:type_name -> protowire.MempoolEntry
|
|
1, // 25: protowire.GetMempoolEntryResponseMessage.error:type_name -> protowire.RPCError
|
|
33, // 26: protowire.GetMempoolEntriesResponseMessage.entries:type_name -> protowire.MempoolEntry
|
|
1, // 27: protowire.GetMempoolEntriesResponseMessage.error:type_name -> protowire.RPCError
|
|
6, // 28: protowire.MempoolEntry.transaction:type_name -> protowire.RpcTransaction
|
|
36, // 29: protowire.GetConnectedPeerInfoResponseMessage.infos:type_name -> protowire.GetConnectedPeerInfoMessage
|
|
1, // 30: protowire.GetConnectedPeerInfoResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 31: protowire.AddPeerResponseMessage.error:type_name -> protowire.RPCError
|
|
6, // 32: protowire.SubmitTransactionRequestMessage.transaction:type_name -> protowire.RpcTransaction
|
|
1, // 33: protowire.SubmitTransactionResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 34: protowire.NotifyVirtualSelectedParentChainChangedResponseMessage.error:type_name -> protowire.RPCError
|
|
49, // 35: protowire.VirtualSelectedParentChainChangedNotificationMessage.acceptedTransactionIds:type_name -> protowire.AcceptedTransactionIds
|
|
2, // 36: protowire.GetBlockResponseMessage.block:type_name -> protowire.RpcBlock
|
|
1, // 37: protowire.GetBlockResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 38: protowire.GetSubnetworkResponseMessage.error:type_name -> protowire.RPCError
|
|
49, // 39: protowire.GetVirtualSelectedParentChainFromBlockResponseMessage.acceptedTransactionIds:type_name -> protowire.AcceptedTransactionIds
|
|
1, // 40: protowire.GetVirtualSelectedParentChainFromBlockResponseMessage.error:type_name -> protowire.RPCError
|
|
2, // 41: protowire.GetBlocksResponseMessage.blocks:type_name -> protowire.RpcBlock
|
|
1, // 42: protowire.GetBlocksResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 43: protowire.GetBlockCountResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 44: protowire.GetBlockDagInfoResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 45: protowire.ResolveFinalityConflictResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 46: protowire.NotifyFinalityConflictsResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 47: protowire.ShutDownResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 48: protowire.GetHeadersResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 49: protowire.NotifyUtxosChangedResponseMessage.error:type_name -> protowire.RPCError
|
|
70, // 50: protowire.UtxosChangedNotificationMessage.added:type_name -> protowire.UtxosByAddressesEntry
|
|
70, // 51: protowire.UtxosChangedNotificationMessage.removed:type_name -> protowire.UtxosByAddressesEntry
|
|
10, // 52: protowire.UtxosByAddressesEntry.outpoint:type_name -> protowire.RpcOutpoint
|
|
11, // 53: protowire.UtxosByAddressesEntry.utxoEntry:type_name -> protowire.RpcUtxoEntry
|
|
1, // 54: protowire.StopNotifyingUtxosChangedResponseMessage.error:type_name -> protowire.RPCError
|
|
70, // 55: protowire.GetUtxosByAddressesResponseMessage.entries:type_name -> protowire.UtxosByAddressesEntry
|
|
1, // 56: protowire.GetUtxosByAddressesResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 57: protowire.GetBalanceByAddressResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 58: protowire.BalancesByAddressEntry.error:type_name -> protowire.RPCError
|
|
78, // 59: protowire.GetBalancesByAddressesResponseMessage.entries:type_name -> protowire.BalancesByAddressEntry
|
|
1, // 60: protowire.GetBalancesByAddressesResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 61: protowire.GetVirtualSelectedParentBlueScoreResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 62: protowire.NotifyVirtualSelectedParentBlueScoreChangedResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 63: protowire.NotifyVirtualDaaScoreChangedResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 64: protowire.NotifyPruningPointUTXOSetOverrideResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 65: protowire.StopNotifyingPruningPointUTXOSetOverrideResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 66: protowire.BanResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 67: protowire.UnbanResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 68: protowire.GetInfoResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 69: protowire.EstimateNetworkHashesPerSecondResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 70: protowire.NotifyNewBlockTemplateResponseMessage.error:type_name -> protowire.RPCError
|
|
33, // 71: protowire.MempoolEntryByAddress.sending:type_name -> protowire.MempoolEntry
|
|
33, // 72: protowire.MempoolEntryByAddress.receiving:type_name -> protowire.MempoolEntry
|
|
104, // 73: protowire.GetMempoolEntriesByAddressesResponseMessage.entries:type_name -> protowire.MempoolEntryByAddress
|
|
1, // 74: protowire.GetMempoolEntriesByAddressesResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 75: protowire.GetCoinSupplyResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 76: protowire.PingResponseMessage.error:type_name -> protowire.RPCError
|
|
117, // 77: protowire.GetConnectionsResponseMessage.profileData:type_name -> protowire.ConnectionsProfileData
|
|
1, // 78: protowire.GetConnectionsResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 79: protowire.GetSystemInfoResponseMessage.error:type_name -> protowire.RPCError
|
|
111, // 80: protowire.GetMetricsResponseMessage.processMetrics:type_name -> protowire.ProcessMetrics
|
|
112, // 81: protowire.GetMetricsResponseMessage.connectionMetrics:type_name -> protowire.ConnectionMetrics
|
|
113, // 82: protowire.GetMetricsResponseMessage.bandwidthMetrics:type_name -> protowire.BandwidthMetrics
|
|
114, // 83: protowire.GetMetricsResponseMessage.consensusMetrics:type_name -> protowire.ConsensusMetrics
|
|
115, // 84: protowire.GetMetricsResponseMessage.storageMetrics:type_name -> protowire.StorageMetrics
|
|
1, // 85: protowire.GetMetricsResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 86: protowire.GetServerInfoResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 87: protowire.GetSyncStatusResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 88: protowire.GetDaaScoreTimestampEstimateResponseMessage.error:type_name -> protowire.RPCError
|
|
129, // 89: protowire.RpcFeeEstimate.priority_bucket:type_name -> protowire.RpcFeerateBucket
|
|
129, // 90: protowire.RpcFeeEstimate.normalBuckets:type_name -> protowire.RpcFeerateBucket
|
|
129, // 91: protowire.RpcFeeEstimate.lowBuckets:type_name -> protowire.RpcFeerateBucket
|
|
130, // 92: protowire.GetFeeEstimateResponseMessage.estimate:type_name -> protowire.RpcFeeEstimate
|
|
1, // 93: protowire.GetFeeEstimateResponseMessage.error:type_name -> protowire.RPCError
|
|
130, // 94: protowire.GetFeeEstimateExperimentalResponseMessage.estimate:type_name -> protowire.RpcFeeEstimate
|
|
131, // 95: protowire.GetFeeEstimateExperimentalResponseMessage.verbose:type_name -> protowire.RpcFeeEstimateVerboseExperimentalData
|
|
1, // 96: protowire.GetFeeEstimateExperimentalResponseMessage.error:type_name -> protowire.RPCError
|
|
1, // 97: protowire.GetCurrentBlockColorResponseMessage.error:type_name -> protowire.RPCError
|
|
6, // 98: protowire.SubmitTransactionReplacementRequestMessage.transaction:type_name -> protowire.RpcTransaction
|
|
6, // 99: protowire.SubmitTransactionReplacementResponseMessage.replacedTransaction:type_name -> protowire.RpcTransaction
|
|
1, // 100: protowire.SubmitTransactionReplacementResponseMessage.error:type_name -> protowire.RPCError
|
|
101, // [101:101] is the sub-list for method output_type
|
|
101, // [101:101] is the sub-list for method input_type
|
|
101, // [101:101] is the sub-list for extension type_name
|
|
101, // [101:101] is the sub-list for extension extendee
|
|
0, // [0:101] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_rpc_proto_init() }
|
|
func file_rpc_proto_init() {
|
|
if File_rpc_proto != nil {
|
|
return
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_rpc_proto_rawDesc,
|
|
NumEnums: 1,
|
|
NumMessages: 139,
|
|
NumExtensions: 0,
|
|
NumServices: 0,
|
|
},
|
|
GoTypes: file_rpc_proto_goTypes,
|
|
DependencyIndexes: file_rpc_proto_depIdxs,
|
|
EnumInfos: file_rpc_proto_enumTypes,
|
|
MessageInfos: file_rpc_proto_msgTypes,
|
|
}.Build()
|
|
File_rpc_proto = out.File
|
|
file_rpc_proto_rawDesc = nil
|
|
file_rpc_proto_goTypes = nil
|
|
file_rpc_proto_depIdxs = nil
|
|
}
|