mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-09 06:12:32 +00:00
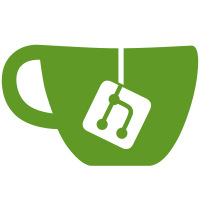
* [NOD-532] Change chain to DAG in the root package. * [NOD-532] Change chain to DAG in checkpoints.go. * [NOD-532] Change chain to DAG in blockdag. * [NOD-532] Change chain to DAG in cmd. * [NOD-532] Change chain to DAG in dagconfig. * [NOD-532] Change chain to DAG in database. * [NOD-532] Change chain to DAG in mempool. * [NOD-532] Change chain to DAG in mempool. * [NOD-532] Change chain to DAG in netsync. * [NOD-532] Change chain to DAG in rpcclient. * [NOD-532] Change chain to DAG in server. * [NOD-532] Change chain to DAG in txscript. * [NOD-532] Change chain to DAG in util. * [NOD-532] Change chain to DAG in wire. * [NOD-532] Remove block heights in dagio.go examples. * [NOD-532] Rename fakeChain to fakeDAG. * [NOD-532] Fix comments, remove unused EnableBCInfoHacks flag. * [NOD-532] Fix comments and variable names. * [NOD-532] Fix comments. * [NOD-532] Fix merge errors. * [NOD-532] Formatted project.
111 lines
2.6 KiB
Go
111 lines
2.6 KiB
Go
package util_test
|
|
|
|
import (
|
|
"fmt"
|
|
"math"
|
|
"math/big"
|
|
|
|
"github.com/kaspanet/kaspad/util"
|
|
)
|
|
|
|
func ExampleAmount() {
|
|
|
|
a := util.Amount(0)
|
|
fmt.Println("Zero Sompi:", a)
|
|
|
|
a = util.Amount(1e8)
|
|
fmt.Println("100,000,000 Sompi:", a)
|
|
|
|
a = util.Amount(1e5)
|
|
fmt.Println("100,000 Sompi:", a)
|
|
// Output:
|
|
// Zero Sompi: 0 KAS
|
|
// 100,000,000 Sompi: 1 KAS
|
|
// 100,000 Sompi: 0.001 KAS
|
|
}
|
|
|
|
func ExampleNewAmount() {
|
|
amountOne, err := util.NewAmount(1)
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
fmt.Println(amountOne) //Output 1
|
|
|
|
amountFraction, err := util.NewAmount(0.01234567)
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
fmt.Println(amountFraction) //Output 2
|
|
|
|
amountZero, err := util.NewAmount(0)
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
fmt.Println(amountZero) //Output 3
|
|
|
|
amountNaN, err := util.NewAmount(math.NaN())
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
fmt.Println(amountNaN) //Output 4
|
|
|
|
// Output: 1 KAS
|
|
// 0.01234567 KAS
|
|
// 0 KAS
|
|
// invalid kaspa amount
|
|
}
|
|
|
|
func ExampleAmount_unitConversions() {
|
|
amount := util.Amount(44433322211100)
|
|
|
|
fmt.Println("Sompi to kKAS:", amount.Format(util.AmountKiloKAS))
|
|
fmt.Println("Sompi to KAS:", amount)
|
|
fmt.Println("Sompi to MilliKAS:", amount.Format(util.AmountMilliKAS))
|
|
fmt.Println("Sompi to MicroKAS:", amount.Format(util.AmountMicroKAS))
|
|
fmt.Println("Sompi to Sompi:", amount.Format(util.AmountSompi))
|
|
|
|
// Output:
|
|
// Sompi to kKAS: 444.333222111 kKAS
|
|
// Sompi to KAS: 444333.222111 KAS
|
|
// Sompi to MilliKAS: 444333222.111 mKAS
|
|
// Sompi to MicroKAS: 444333222111 μKAS
|
|
// Sompi to Sompi: 44433322211100 Sompi
|
|
}
|
|
|
|
// This example demonstrates how to convert the compact "bits" in a block header
|
|
// which represent the target difficulty to a big integer and display it using
|
|
// the typical hex notation.
|
|
func ExampleCompactToBig() {
|
|
bits := uint32(419465580)
|
|
targetDifficulty := util.CompactToBig(bits)
|
|
|
|
// Display it in hex.
|
|
fmt.Printf("%064x\n", targetDifficulty.Bytes())
|
|
|
|
// Output:
|
|
// 0000000000000000896c00000000000000000000000000000000000000000000
|
|
}
|
|
|
|
// This example demonstrates how to convert a target difficulty into the compact
|
|
// "bits" in a block header which represent that target difficulty .
|
|
func ExampleBigToCompact() {
|
|
// Convert the target difficulty from block 300000 in the bitcoin
|
|
// main chain to compact form.
|
|
t := "0000000000000000896c00000000000000000000000000000000000000000000"
|
|
targetDifficulty, success := new(big.Int).SetString(t, 16)
|
|
if !success {
|
|
fmt.Println("invalid target difficulty")
|
|
return
|
|
}
|
|
bits := util.BigToCompact(targetDifficulty)
|
|
|
|
fmt.Println(bits)
|
|
|
|
// Output:
|
|
// 419465580
|
|
}
|