mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-03-30 15:08:33 +00:00
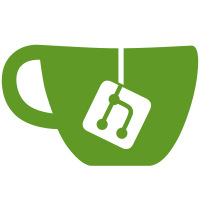
* [NOD-1125] Write a skeleton for starting IBD. * [NOD-1125] Add WaitForIBDStart to Peer. * [NOD-1125] Move functions around. * [NOD-1125] Fix merge errors. * [NOD-1125] Fix a comment. * [NOD-1125] Implement sendGetBlockLocator. * [NOD-1125] Begin implementing findIBDLowHash. * [NOD-1125] Finish implementing findIBDLowHash. * [NOD-1125] Rename findIBDLowHash to findHighestSharedBlockHash. * [NOD-1125] Implement downloadBlocks. * [NOD-1125] Implement msgIBDBlock. * [NOD-1125] Implement msgIBDBlock. * [NOD-1125] Fix message types for HandleIBD. * [NOD-1125] Write a skeleton for requesting selected tip hashes. * [NOD-1125] Write a skeleton for the rest of the IBD requests. * [NOD-1125] Implement HandleGetBlockLocator. * [NOD-1125] Fix wrong timeout. * [NOD-1125] Fix compilation error. * [NOD-1125] Implement HandleGetBlocks. * [NOD-1125] Fix compilation errors. * [NOD-1125] Fix merge errors. * [NOD-1125] Implement selectPeerForIBD. * [NOD-1125] Implement RequestSelectedTip. * [NOD-1125] Implement HandleGetSelectedTip. * [NOD-1125] Make go lint happy. * [NOD-1125] Add minGetSelectedTipInterval. * [NOD-1125] Call StartIBDIfRequired where needed. * [NOD-1125] Fix merge errors. * [NOD-1125] Remove a redundant line. * [NOD-1125] Rename shouldContinue to shouldStop. * [NOD-1125] Lowercasify an error message. * [NOD-1125] Shuffle statements around in findHighestSharedBlockHash. * [NOD-1125] Rename hasRecentlyReceivedBlock to isDAGTimeCurrent. * [NOD-1125] Scope minGetSelectedTipInterval. * [NOD-1125] Handle an unhandled error. * [NOD-1125] Use AddUint32 instead of LoadUint32 + StoreUint32. * [NOD-1125] Use AddUint32 instead of LoadUint32 + StoreUint32. * [NOD-1125] Use SwapUint32 instead of AddUint32. * [NOD-1125] Remove error from requestSelectedTips. * [NOD-1125] Actually stop IBD when it should stop. * [NOD-1125] Actually stop RequestSelectedTip when it should stop. * [NOD-1125] Don't ban peers that send us delayed blocks during IBD. * [NOD-1125] Make unexpected message type messages nicer. * [NOD-1125] Remove Peer.ready and make HandleHandshake return it to guarantee we never operate on a non-initialized peer. * [NOD-1125] Remove errors associated with Peer.ready. * [NOD-1125] Extract maxHashesInMsgIBDBlocks to a const. * [NOD-1125] Move the ibd package into flows. * [NOD-1125] Start IBD if required after getting an unknown block inv. * [NOD-1125] Don't request blocks during relay if we're in the middle of IBD. * [NOD-1125] Remove AddBlockLocatorHash. * [NOD-1125] Extract runIBD to a seperate function. * [NOD-1125] Extract runSelectedTipRequest to a seperate function. * [NOD-1125] Remove EnqueueWithTimeout. * [NOD-1125] Increase the capacity of the outgoingRoute. * [NOD-1125] Fix some bad names. * [NOD-1125] Fix a comment. * [NOD-1125] Simplify a comment. * [NOD-1125] Move WaitFor... functions into their respective run... functions. * [NOD-1125] Return default values in case of error. * [NOD-1125] Use CmdXXX in error messages. * [NOD-1125] Use MaxInvPerMsg in outgoingRouteMaxMessages instead of MaxBlockLocatorsPerMsg. * [NOD-1125] Fix a comment. * [NOD-1125] Disconnect a peer that sends us a delayed block during IBD. * [NOD-1125] Use StoreUint32 instead of SwapUint32. * [NOD-1125] Add a comment. * [NOD-1125] Don't ban peers that send us delayed blocks.
118 lines
3.4 KiB
Go
118 lines
3.4 KiB
Go
// Copyright (c) 2013-2016 The btcsuite developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package wire
|
|
|
|
import (
|
|
"bytes"
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/davecgh/go-spew/spew"
|
|
)
|
|
|
|
// TestIBDBlock tests the MsgIBDBlock API.
|
|
func TestIBDBlock(t *testing.T) {
|
|
pver := ProtocolVersion
|
|
|
|
// Block 1 header.
|
|
parentHashes := blockOne.Header.ParentHashes
|
|
hashMerkleRoot := blockOne.Header.HashMerkleRoot
|
|
acceptedIDMerkleRoot := blockOne.Header.AcceptedIDMerkleRoot
|
|
utxoCommitment := blockOne.Header.UTXOCommitment
|
|
bits := blockOne.Header.Bits
|
|
nonce := blockOne.Header.Nonce
|
|
bh := NewBlockHeader(1, parentHashes, hashMerkleRoot, acceptedIDMerkleRoot, utxoCommitment, bits, nonce)
|
|
|
|
// Ensure the command is expected value.
|
|
wantCmd := MessageCommand(25)
|
|
msg := NewMsgIBDBlock(NewMsgBlock(bh))
|
|
if cmd := msg.Command(); cmd != wantCmd {
|
|
t.Errorf("NewMsgIBDBlock: wrong command - got %v want %v",
|
|
cmd, wantCmd)
|
|
}
|
|
|
|
// Ensure max payload is expected value for latest protocol version.
|
|
wantPayload := uint32(1024 * 1024 * 32)
|
|
maxPayload := msg.MaxPayloadLength(pver)
|
|
if maxPayload != wantPayload {
|
|
t.Errorf("MaxPayloadLength: wrong max payload length for "+
|
|
"protocol version %d - got %v, want %v", pver,
|
|
maxPayload, wantPayload)
|
|
}
|
|
|
|
// Ensure we get the same block header data back out.
|
|
if !reflect.DeepEqual(&msg.Header, bh) {
|
|
t.Errorf("NewMsgIBDBlock: wrong block header - got %v, want %v",
|
|
spew.Sdump(&msg.Header), spew.Sdump(bh))
|
|
}
|
|
|
|
// Ensure transactions are added properly.
|
|
tx := blockOne.Transactions[0].Copy()
|
|
msg.AddTransaction(tx)
|
|
if !reflect.DeepEqual(msg.Transactions, blockOne.Transactions) {
|
|
t.Errorf("AddTransaction: wrong transactions - got %v, want %v",
|
|
spew.Sdump(msg.Transactions),
|
|
spew.Sdump(blockOne.Transactions))
|
|
}
|
|
|
|
// Ensure transactions are properly cleared.
|
|
msg.ClearTransactions()
|
|
if len(msg.Transactions) != 0 {
|
|
t.Errorf("ClearTransactions: wrong transactions - got %v, want %v",
|
|
len(msg.Transactions), 0)
|
|
}
|
|
}
|
|
|
|
// TestIBDBlockWire tests the MsgIBDBlock wire encode and decode for various numbers
|
|
// of transaction inputs and outputs and protocol versions.
|
|
func TestIBDBlockWire(t *testing.T) {
|
|
tests := []struct {
|
|
in *MsgIBDBlock // Message to encode
|
|
out *MsgIBDBlock // Expected decoded message
|
|
buf []byte // Wire encoding
|
|
txLocs []TxLoc // Expected transaction locations
|
|
pver uint32 // Protocol version for wire encoding
|
|
}{
|
|
// Latest protocol version.
|
|
{
|
|
&MsgIBDBlock{blockOne},
|
|
&MsgIBDBlock{blockOne},
|
|
blockOneBytes,
|
|
blockOneTxLocs,
|
|
ProtocolVersion,
|
|
},
|
|
}
|
|
|
|
t.Logf("Running %d tests", len(tests))
|
|
for i, test := range tests {
|
|
// Encode the message to wire format.
|
|
var buf bytes.Buffer
|
|
err := test.in.KaspaEncode(&buf, test.pver)
|
|
if err != nil {
|
|
t.Errorf("KaspaEncode #%d error %v", i, err)
|
|
continue
|
|
}
|
|
if !bytes.Equal(buf.Bytes(), test.buf) {
|
|
t.Errorf("KaspaEncode #%d\n got: %s want: %s", i,
|
|
spew.Sdump(buf.Bytes()), spew.Sdump(test.buf))
|
|
continue
|
|
}
|
|
|
|
// Decode the message from wire format.
|
|
var msg MsgIBDBlock
|
|
rbuf := bytes.NewReader(test.buf)
|
|
err = msg.KaspaDecode(rbuf, test.pver)
|
|
if err != nil {
|
|
t.Errorf("KaspaDecode #%d error %v", i, err)
|
|
continue
|
|
}
|
|
if !reflect.DeepEqual(&msg, test.out) {
|
|
t.Errorf("KaspaDecode #%d\n got: %s want: %s", i,
|
|
spew.Sdump(&msg), spew.Sdump(test.out))
|
|
continue
|
|
}
|
|
}
|
|
}
|