mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-04 03:42:30 +00:00
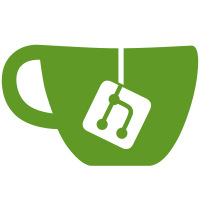
* [NOD-641] Upgrade to github.com/pkg/errors v0.9.1 and use errors.As where needed * [NOD-641] Fix find and replace error * [NOD-641] Use errors.As for error type checking * [NOD-641] Fix errors.As for pointer types * [NOD-641] Use errors.As where needed * [NOD-641] Rename rErr->ruleErr * [NOD-641] Rename derr->dbErr * [NOD-641] e->flagsErr where necessary * [NOD-641] change jerr to more appropriate name * [NOD-641] Rename cerr->bdRuleErr * [NOD-641] Rename serr->scriptErr * [NOD-641] Use errors.Is instead of testutil.AreErrorsEqual in TestNewHashFromStr * [NOD-641] Rename bdRuleErr->dagRuleErr * [NOD-641] Rename mErr->msgErr * [NOD-641] Rename dErr->deserializeErr
114 lines
2.8 KiB
Go
114 lines
2.8 KiB
Go
package main
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/hex"
|
|
"github.com/kaspanet/kaspad/rpcclient"
|
|
"github.com/kaspanet/kaspad/rpcmodel"
|
|
"github.com/kaspanet/kaspad/util"
|
|
"github.com/kaspanet/kaspad/wire"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
const (
|
|
resultsCount = 1000
|
|
minConfirmations = 10
|
|
)
|
|
|
|
func findUnspentTXO(cfg *ConfigFlags, client *rpcclient.Client, addrPubKeyHash *util.AddressPubKeyHash) (*wire.Outpoint, *wire.MsgTx, error) {
|
|
txs, err := collectTransactions(client, addrPubKeyHash)
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
utxos := buildUTXOs(txs)
|
|
for outpoint, tx := range utxos {
|
|
// Skip TXOs that can't pay for registration
|
|
if tx.TxOut[outpoint.Index].Value < cfg.RegistryTxFee {
|
|
continue
|
|
}
|
|
|
|
return &outpoint, tx, nil
|
|
}
|
|
|
|
return nil, nil, nil
|
|
}
|
|
|
|
func collectTransactions(client *rpcclient.Client, addrPubKeyHash *util.AddressPubKeyHash) ([]*wire.MsgTx, error) {
|
|
txs := make([]*wire.MsgTx, 0)
|
|
skip := 0
|
|
for {
|
|
results, err := client.SearchRawTransactionsVerbose(addrPubKeyHash, skip, resultsCount, true, false, nil)
|
|
if err != nil {
|
|
// Break when there are no further txs
|
|
var rpcError *rpcmodel.RPCError
|
|
if ok := errors.As(err, &rpcError); ok && rpcError.Code == rpcmodel.ErrRPCNoTxInfo {
|
|
break
|
|
}
|
|
|
|
return nil, err
|
|
}
|
|
|
|
for _, result := range results {
|
|
// Mempool transactions bring about unnecessary complexity, so
|
|
// simply don't bother processing them
|
|
if result.IsInMempool {
|
|
continue
|
|
}
|
|
|
|
tx, err := parseRawTransactionResult(result)
|
|
if err != nil {
|
|
return nil, errors.Errorf("failed to process SearchRawTransactionResult: %s", err)
|
|
}
|
|
if tx == nil {
|
|
continue
|
|
}
|
|
if !isTxMatured(tx, *result.Confirmations) {
|
|
continue
|
|
}
|
|
|
|
txs = append(txs, tx)
|
|
}
|
|
|
|
skip += resultsCount
|
|
}
|
|
return txs, nil
|
|
}
|
|
|
|
func parseRawTransactionResult(result *rpcmodel.SearchRawTransactionsResult) (*wire.MsgTx, error) {
|
|
txBytes, err := hex.DecodeString(result.Hex)
|
|
if err != nil {
|
|
return nil, errors.Errorf("failed to decode transaction bytes: %s", err)
|
|
}
|
|
var tx wire.MsgTx
|
|
reader := bytes.NewReader(txBytes)
|
|
err = tx.Deserialize(reader)
|
|
if err != nil {
|
|
return nil, errors.Errorf("failed to deserialize transaction: %s", err)
|
|
}
|
|
return &tx, nil
|
|
}
|
|
|
|
func isTxMatured(tx *wire.MsgTx, confirmations uint64) bool {
|
|
if !tx.IsCoinBase() {
|
|
return confirmations >= minConfirmations
|
|
}
|
|
return confirmations >= ActiveConfig().NetParams().BlockCoinbaseMaturity
|
|
}
|
|
|
|
func buildUTXOs(txs []*wire.MsgTx) map[wire.Outpoint]*wire.MsgTx {
|
|
utxos := make(map[wire.Outpoint]*wire.MsgTx)
|
|
for _, tx := range txs {
|
|
for i := range tx.TxOut {
|
|
outpoint := wire.NewOutpoint(tx.TxID(), uint32(i))
|
|
utxos[*outpoint] = tx
|
|
}
|
|
}
|
|
for _, tx := range txs {
|
|
for _, input := range tx.TxIn {
|
|
delete(utxos, input.PreviousOutpoint)
|
|
}
|
|
}
|
|
return utxos
|
|
}
|