mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-07-04 03:42:30 +00:00
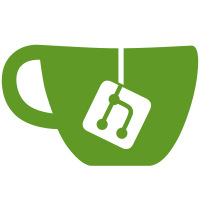
* [NOD-510] Change coinbase flags to kaspad. * [NOD-510] Removed superfluous spaces after periods in comments. * [NOD-510] Rename btcd -> kaspad in the root folder. * [NOD-510] Rename BtcEncode -> KaspaEncode and BtcDecode -> KaspaDecode. * [NOD-510] Rename BtcEncode -> KaspaEncode and BtcDecode -> KaspaDecode. * [NOD-510] Continue renaming btcd -> kaspad. * [NOD-510] Rename btcjson -> kaspajson. * [NOD-510] Rename file names inside kaspajson. * [NOD-510] Rename kaspajson -> jsonrpc. * [NOD-510] Finish renaming in addrmgr. * [NOD-510] Rename package btcec to ecc. * [NOD-510] Finish renaming stuff in blockdag. * [NOD-510] Rename stuff in cmd. * [NOD-510] Rename stuff in config. * [NOD-510] Rename stuff in connmgr. * [NOD-510] Rename stuff in dagconfig. * [NOD-510] Rename stuff in database. * [NOD-510] Rename stuff in docker. * [NOD-510] Rename stuff in integration. * [NOD-510] Rename jsonrpc to rpcmodel. * [NOD-510] Rename stuff in limits. * [NOD-510] Rename stuff in logger. * [NOD-510] Rename stuff in mempool. * [NOD-510] Rename stuff in mining. * [NOD-510] Rename stuff in netsync. * [NOD-510] Rename stuff in peer. * [NOD-510] Rename stuff in release. * [NOD-510] Rename stuff in rpcclient. * [NOD-510] Rename stuff in server. * [NOD-510] Rename stuff in signal. * [NOD-510] Rename stuff in txscript. * [NOD-510] Rename stuff in util. * [NOD-510] Rename stuff in wire. * [NOD-510] Fix failing tests. * [NOD-510] Fix merge errors. * [NOD-510] Fix go vet errors. * [NOD-510] Remove merged file that's no longer relevant. * [NOD-510] Add a comment above Op0. * [NOD-510] Fix some comments referencing Bitcoin Core. * [NOD-510] Fix some more comments referencing Bitcoin Core. * [NOD-510] Fix bitcoin -> kaspa. * [NOD-510] Fix more bitcoin -> kaspa. * [NOD-510] Fix comments, remove DisconnectBlock in addrindex. * [NOD-510] Rename KSPD to KASD. * [NOD-510] Fix comments and user agent.
88 lines
2.5 KiB
Go
88 lines
2.5 KiB
Go
// Copyright (c) 2014 The btcsuite developers
|
|
// Use of this source code is governed by an ISC
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package ecc_test
|
|
|
|
import (
|
|
"encoding/hex"
|
|
"fmt"
|
|
"github.com/kaspanet/kaspad/util/daghash"
|
|
|
|
"github.com/kaspanet/kaspad/ecc"
|
|
)
|
|
|
|
// This example demonstrates signing a message with a secp256k1 private key that
|
|
// is first parsed form raw bytes and serializing the generated signature.
|
|
func Example_signMessage() {
|
|
// Decode a hex-encoded private key.
|
|
pkBytes, err := hex.DecodeString("22a47fa09a223f2aa079edf85a7c2d4f87" +
|
|
"20ee63e502ee2869afab7de234b80c")
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
privKey, pubKey := ecc.PrivKeyFromBytes(ecc.S256(), pkBytes)
|
|
|
|
// Sign a message using the private key.
|
|
message := "test message"
|
|
messageHash := daghash.DoubleHashB([]byte(message))
|
|
signature, err := privKey.Sign(messageHash)
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
|
|
// Serialize and display the signature.
|
|
fmt.Printf("Serialized Signature: %x\n", signature.Serialize())
|
|
|
|
// Verify the signature for the message using the public key.
|
|
verified := signature.Verify(messageHash, pubKey)
|
|
fmt.Printf("Signature Verified? %v\n", verified)
|
|
|
|
// Output:
|
|
// Serialized Signature: 275d1c73a01b023377633cd1eaa6460e8cd0542ba089ad50450a8197246b3ef6d6836ef5a18226132c305e2b2060a699529cadc816fc98d63bc7d05771acec4d
|
|
// Signature Verified? true
|
|
}
|
|
|
|
// This example demonstrates verifying a secp256k1 signature against a public
|
|
// key that is first parsed from raw bytes. The signature is also parsed from
|
|
// raw bytes.
|
|
func Example_verifySignature() {
|
|
// Decode hex-encoded serialized public key.
|
|
pubKeyBytes, err := hex.DecodeString("02a673638cb9587cb68ea08dbef685c" +
|
|
"6f2d2a751a8b3c6f2a7e9a4999e6e4bfaf5")
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
pubKey, err := ecc.ParsePubKey(pubKeyBytes, ecc.S256())
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
|
|
// Decode hex-encoded serialized signature.
|
|
sigBytes, err := hex.DecodeString("275d1c73a01b023377633cd1eaa6460e8cd0542ba" +
|
|
"089ad50450a8197246b3ef6d6836ef5a18226132c305e2b2060a699529cadc816fc98d63bc7d05771acec4d")
|
|
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
signature, err := ecc.ParseSignature(sigBytes)
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
return
|
|
}
|
|
|
|
// Verify the signature for the message using the public key.
|
|
message := "test message"
|
|
messageHash := daghash.DoubleHashB([]byte(message))
|
|
verified := signature.Verify(messageHash, pubKey)
|
|
fmt.Println("Signature Verified?", verified)
|
|
|
|
// Output:
|
|
// Signature Verified? true
|
|
}
|