mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-22 23:07:04 +00:00
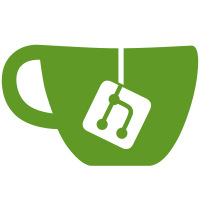
* [NOD-1551] Make UTXO-Diff implemented fully in utils/utxo * [NOD-1551] Fixes everywhere except database * [NOD-1551] Fix database * [NOD-1551] Add comments * [NOD-1551] Partial commit * [NOD-1551] Comlete making UTXOEntry immutable + don't clone it in UTXOCollectionClone * [NOD-1551] Rename ToUnmutable -> ToImmutable * [NOD-1551] Track immutable references generated from mutable UTXODiff, and invalidate them if the mutable one changed * [NOD-1551] Clone scriptPubKey in NewUTXOEntry * [NOD-1551] Remove redundant code * [NOD-1551] Remove redundant call for .CloneMutable and then .ToImmutable * [NOD-1551] Make utxoEntry pointert-receiver + clone ScriptPubKey in getter
34 lines
1.0 KiB
Go
34 lines
1.0 KiB
Go
package model
|
|
|
|
import "github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
|
|
// UTXOCollection represents a collection of UTXO entries, indexed by their outpoint
|
|
type UTXOCollection interface {
|
|
Iterator() ReadOnlyUTXOSetIterator
|
|
Get(outpoint *externalapi.DomainOutpoint) (externalapi.UTXOEntry, bool)
|
|
Contains(outpoint *externalapi.DomainOutpoint) bool
|
|
Len() int
|
|
}
|
|
|
|
// UTXODiff represents the diff between two UTXO sets
|
|
type UTXODiff interface {
|
|
ToAdd() UTXOCollection
|
|
ToRemove() UTXOCollection
|
|
WithDiff(other UTXODiff) (UTXODiff, error)
|
|
DiffFrom(other UTXODiff) (UTXODiff, error)
|
|
CloneMutable() MutableUTXODiff
|
|
}
|
|
|
|
// MutableUTXODiff represents a UTXO-Diff that can be mutated
|
|
type MutableUTXODiff interface {
|
|
ToImmutable() UTXODiff
|
|
|
|
WithDiff(other UTXODiff) (UTXODiff, error)
|
|
DiffFrom(other UTXODiff) (UTXODiff, error)
|
|
ToAdd() UTXOCollection
|
|
ToRemove() UTXOCollection
|
|
|
|
WithDiffInPlace(other UTXODiff) error
|
|
AddTransaction(transaction *externalapi.DomainTransaction, blockBlueScore uint64) error
|
|
}
|