mirror of
https://github.com/kaspanet/kaspad.git
synced 2025-05-22 14:56:44 +00:00
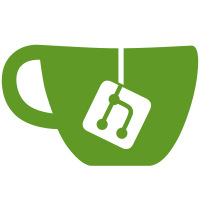
* Mine JSON * [Reindex tests] add test_params and validate_mining flag to test_consensus * Rename file and extend tests * Ignore local test datasets * Use spaces over tabs * Reindex algorithm - full algorithm, initial commit, some tests fail * Reindex algorithm - a few critical fixes * Reindex algorithm - move reindex struct and all related operations to new file * Reindex algorithm - added a validateIntervals method and modified tests to use it (instead of exact comparisons) * Reindex algorithm - modified reindexIntervals to receive the new child as argument and fixed an important related bug * Reindex attack tests - move logic to helper function and add stretch test * Reindex algorithm - variable names and some comments * Reindex algorithm - minor changes * Reindex algorithm - minor changes 2 * Reindex algorithm - extended stretch test * Reindex algorithm - small fix to validate function * Reindex tests - move tests and add DAG files * go format fixes * TestParams doc comment * Reindex tests - exact comparisons are not needed * Update to version 0.8.6 * Remove TestParams and use AddUTXOInvalidHeader instead * Use gzipeed test files * This unintended change somehow slipped in through branch merges * Rename test * Move interval increase/decrease methods to reachability interval file * Addressing a bunch of minor review comments * Addressed a few more minor review comments * Make code of offsetSiblingsBefore and offsetSiblingsAfter symmetric * Optimize reindex logic in cases where reorg occurs + reorg test * Do not change reindex root too fast (on reorg) * Some comments * A few more comments * Addressing review comments * Remove TestNoAttackAlternateReorg and assert chain attack * Minor Co-authored-by: Elichai Turkel <elichai.turkel@gmail.com> Co-authored-by: Mike Zak <feanorr@gmail.com> Co-authored-by: Ori Newman <orinewman1@gmail.com>
334 lines
11 KiB
Go
334 lines
11 KiB
Go
package reachabilitymanager_test
|
|
|
|
import (
|
|
"math"
|
|
"testing"
|
|
|
|
"github.com/kaspanet/kaspad/domain/consensus"
|
|
"github.com/kaspanet/kaspad/domain/consensus/model/externalapi"
|
|
"github.com/kaspanet/kaspad/domain/consensus/utils/testutils"
|
|
"github.com/kaspanet/kaspad/domain/dagconfig"
|
|
)
|
|
|
|
func TestAddChildThatPointsDirectlyToTheSelectedParentChainBelowReindexRoot(t *testing.T) {
|
|
reachabilityReindexWindow := uint64(10)
|
|
testutils.ForAllNets(t, true, func(t *testing.T, params *dagconfig.Params) {
|
|
factory := consensus.NewFactory()
|
|
tc, tearDown, err := factory.NewTestConsensus(params, false,
|
|
"TestAddChildThatPointsDirectlyToTheSelectedParentChainBelowReindexRoot")
|
|
if err != nil {
|
|
t.Fatalf("NewTestConsensus: %+v", err)
|
|
}
|
|
defer tearDown(false)
|
|
|
|
tc.ReachabilityManager().SetReachabilityReindexWindow(reachabilityReindexWindow)
|
|
|
|
reindexRoot, err := tc.ReachabilityDataStore().ReachabilityReindexRoot(tc.DatabaseContext())
|
|
if err != nil {
|
|
t.Fatalf("ReachabilityReindexRoot: %s", err)
|
|
}
|
|
|
|
if !reindexRoot.Equal(params.GenesisHash) {
|
|
t.Fatalf("reindex root is expected to initially be genesis")
|
|
}
|
|
|
|
// Add a block on top of the genesis block
|
|
chainRootBlock, _, err := tc.AddBlock([]*externalapi.DomainHash{params.GenesisHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
// Add chain of reachabilityReindexWindow blocks above chainRootBlock.
|
|
// This should move the reindex root
|
|
chainRootBlockTipHash := chainRootBlock
|
|
for i := uint64(0); i < reachabilityReindexWindow; i++ {
|
|
chainBlock, _, err := tc.AddBlock([]*externalapi.DomainHash{chainRootBlockTipHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
chainRootBlockTipHash = chainBlock
|
|
}
|
|
|
|
newReindexRoot, err := tc.ReachabilityDataStore().ReachabilityReindexRoot(tc.DatabaseContext())
|
|
if err != nil {
|
|
t.Fatalf("ReachabilityReindexRoot: %s", err)
|
|
}
|
|
|
|
if newReindexRoot.Equal(reindexRoot) {
|
|
t.Fatalf("reindex root is expected to change")
|
|
}
|
|
|
|
// Add enough blocks over genesis to test also the case where the first
|
|
// level (genesis in this case) runs out of slack
|
|
slackSize := tc.ReachabilityManager().ReachabilityReindexSlack()
|
|
blocksToAdd := uint64(math.Log2(float64(slackSize))) + 2
|
|
for i := uint64(0); i < blocksToAdd; i++ {
|
|
_, _, err = tc.AddBlock([]*externalapi.DomainHash{params.GenesisHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
}
|
|
|
|
err = tc.ReachabilityManager().ValidateIntervals(params.GenesisHash)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
})
|
|
}
|
|
|
|
func TestUpdateReindexRoot(t *testing.T) {
|
|
reachabilityReindexWindow := uint64(10)
|
|
testutils.ForAllNets(t, true, func(t *testing.T, params *dagconfig.Params) {
|
|
factory := consensus.NewFactory()
|
|
tc, tearDown, err := factory.NewTestConsensus(params, false, "TestUpdateReindexRoot")
|
|
if err != nil {
|
|
t.Fatalf("NewTestConsensus: %s", err)
|
|
}
|
|
defer tearDown(false)
|
|
|
|
tc.ReachabilityManager().SetReachabilityReindexWindow(reachabilityReindexWindow)
|
|
|
|
intervalSize := func(hash *externalapi.DomainHash) uint64 {
|
|
data, err := tc.ReachabilityDataStore().ReachabilityData(tc.DatabaseContext(), hash)
|
|
if err != nil {
|
|
t.Fatalf("ReachabilityData: %s", err)
|
|
}
|
|
return data.Interval().End - data.Interval().Start + 1
|
|
}
|
|
|
|
// Add two blocks on top of the genesis block
|
|
chain1RootBlock, _, err := tc.AddBlock([]*externalapi.DomainHash{params.GenesisHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
chain2RootBlock, _, err := tc.AddBlock([]*externalapi.DomainHash{params.GenesisHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
// Make two chains of size reachabilityReindexWindow and check that the reindex root is not changed.
|
|
chain1Tip, chain2Tip := chain1RootBlock, chain2RootBlock
|
|
for i := uint64(0); i < reachabilityReindexWindow-1; i++ {
|
|
var err error
|
|
chain1Tip, _, err = tc.AddBlock([]*externalapi.DomainHash{chain1Tip}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
chain2Tip, _, err = tc.AddBlock([]*externalapi.DomainHash{chain2Tip}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
reindexRoot, err := tc.ReachabilityDataStore().ReachabilityReindexRoot(tc.DatabaseContext())
|
|
if err != nil {
|
|
t.Fatalf("ReachabilityReindexRoot: %s", err)
|
|
}
|
|
|
|
if !reindexRoot.Equal(params.GenesisHash) {
|
|
t.Fatalf("reindex root unexpectedly moved")
|
|
}
|
|
}
|
|
|
|
// Add another block over chain1. This will move the reindex root to chain1RootBlock
|
|
_, _, err = tc.AddBlock([]*externalapi.DomainHash{chain1Tip}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
// Make sure that chain1RootBlock is now the reindex root
|
|
reindexRoot, err := tc.ReachabilityDataStore().ReachabilityReindexRoot(tc.DatabaseContext())
|
|
if err != nil {
|
|
t.Fatalf("ReachabilityReindexRoot: %s", err)
|
|
}
|
|
|
|
if !reindexRoot.Equal(chain1RootBlock) {
|
|
t.Fatalf("chain1RootBlock is not the reindex root after reindex")
|
|
}
|
|
|
|
// Make sure that tight intervals have been applied to chain2. Since
|
|
// we added reachabilityReindexWindow-1 blocks to chain2, the size
|
|
// of the interval at its root should be equal to reachabilityReindexWindow
|
|
if intervalSize(chain2RootBlock) != reachabilityReindexWindow {
|
|
t.Fatalf("got unexpected chain2RootBlock interval. Want: %d, got: %d",
|
|
intervalSize(chain2RootBlock), reachabilityReindexWindow)
|
|
}
|
|
|
|
// Make sure that the rest of the interval has been allocated to
|
|
// chain1RootNode, minus slack from both sides
|
|
expectedChain1RootIntervalSize := intervalSize(params.GenesisHash) - 1 -
|
|
intervalSize(chain2RootBlock) - 2*tc.ReachabilityManager().ReachabilityReindexSlack()
|
|
if intervalSize(chain1RootBlock) != expectedChain1RootIntervalSize {
|
|
t.Fatalf("got unexpected chain1RootBlock interval. Want: %d, got: %d",
|
|
intervalSize(chain1RootBlock), expectedChain1RootIntervalSize)
|
|
}
|
|
|
|
err = tc.ReachabilityManager().ValidateIntervals(params.GenesisHash)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
})
|
|
}
|
|
|
|
func TestReindexIntervalsEarlierThanReindexRoot(t *testing.T) {
|
|
reachabilityReindexWindow := uint64(10)
|
|
testutils.ForAllNets(t, true, func(t *testing.T, params *dagconfig.Params) {
|
|
factory := consensus.NewFactory()
|
|
tc, tearDown, err := factory.NewTestConsensus(params, false, "TestUpdateReindexRoot")
|
|
if err != nil {
|
|
t.Fatalf("NewTestConsensus: %+v", err)
|
|
}
|
|
defer tearDown(false)
|
|
|
|
tc.ReachabilityManager().SetReachabilityReindexWindow(reachabilityReindexWindow)
|
|
|
|
intervalSize := func(hash *externalapi.DomainHash) uint64 {
|
|
data, err := tc.ReachabilityDataStore().ReachabilityData(tc.DatabaseContext(), hash)
|
|
if err != nil {
|
|
t.Fatalf("ReachabilityData: %s", err)
|
|
}
|
|
return data.Interval().End - data.Interval().Start + 1
|
|
}
|
|
|
|
// Add three children to the genesis: leftBlock, centerBlock, rightBlock
|
|
leftBlock, _, err := tc.AddBlock([]*externalapi.DomainHash{params.GenesisHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
centerBlock, _, err := tc.AddBlock([]*externalapi.DomainHash{params.GenesisHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
rightBlock, _, err := tc.AddBlock([]*externalapi.DomainHash{params.GenesisHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
// Add a chain of reachabilityReindexWindow blocks above centerBlock.
|
|
// This will move the reindex root to centerBlock
|
|
centerTipHash := centerBlock
|
|
for i := uint64(0); i < reachabilityReindexWindow; i++ {
|
|
var err error
|
|
centerTipHash, _, err = tc.AddBlock([]*externalapi.DomainHash{centerTipHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
}
|
|
|
|
// Make sure that centerBlock is now the reindex root
|
|
reindexRoot, err := tc.ReachabilityDataStore().ReachabilityReindexRoot(tc.DatabaseContext())
|
|
if err != nil {
|
|
t.Fatalf("ReachabilityReindexRoot: %s", err)
|
|
}
|
|
|
|
if !reindexRoot.Equal(centerBlock) {
|
|
t.Fatalf("centerBlock is not the reindex root after reindex")
|
|
}
|
|
|
|
// Get the current interval for leftBlock. The reindex should have
|
|
// resulted in a tight interval there
|
|
if intervalSize(leftBlock) != 1 {
|
|
t.Fatalf("leftBlock interval not tight after reindex")
|
|
}
|
|
|
|
// Get the current interval for rightBlock. The reindex should have
|
|
// resulted in a tight interval there
|
|
if intervalSize(rightBlock) != 1 {
|
|
t.Fatalf("rightBlock interval not tight after reindex")
|
|
}
|
|
|
|
err = tc.ReachabilityManager().ValidateIntervals(params.GenesisHash)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
// Add a chain of reachabilityReindexWindow - 1 blocks above leftBlock.
|
|
// Each addition will trigger a low-than-reindex-root reindex. We
|
|
// expect the centerInterval to shrink by 1 each time, but its child
|
|
// to remain unaffected
|
|
|
|
leftTipHash := leftBlock
|
|
for i := uint64(0); i < reachabilityReindexWindow-1; i++ {
|
|
var err error
|
|
leftTipHash, _, err = tc.AddBlock([]*externalapi.DomainHash{leftTipHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
err = tc.ReachabilityManager().ValidateIntervals(params.GenesisHash)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
}
|
|
|
|
// Add a chain of reachabilityReindexWindow - 1 blocks above rightBlock.
|
|
// Each addition will trigger a low-than-reindex-root reindex. We
|
|
// expect the centerInterval to shrink by 1 each time, but its child
|
|
// to remain unaffected
|
|
rightTipHash := rightBlock
|
|
for i := uint64(0); i < reachabilityReindexWindow-1; i++ {
|
|
var err error
|
|
rightTipHash, _, err = tc.AddBlock([]*externalapi.DomainHash{rightTipHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
err = tc.ReachabilityManager().ValidateIntervals(params.GenesisHash)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
}
|
|
|
|
err = tc.ReachabilityManager().ValidateIntervals(params.GenesisHash)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
})
|
|
}
|
|
|
|
func TestTipsAfterReindexIntervalsEarlierThanReindexRoot(t *testing.T) {
|
|
reachabilityReindexWindow := uint64(10)
|
|
testutils.ForAllNets(t, true, func(t *testing.T, params *dagconfig.Params) {
|
|
factory := consensus.NewFactory()
|
|
tc, tearDown, err := factory.NewTestConsensus(params, false, "TestUpdateReindexRoot")
|
|
if err != nil {
|
|
t.Fatalf("NewTestConsensus: %s", err)
|
|
}
|
|
defer tearDown(false)
|
|
|
|
tc.ReachabilityManager().SetReachabilityReindexWindow(reachabilityReindexWindow)
|
|
|
|
// Add a chain of reachabilityReindexWindow + 1 blocks above the genesis.
|
|
// This will set the reindex root to the child of genesis
|
|
chainTipHash := params.GenesisHash
|
|
for i := uint64(0); i < reachabilityReindexWindow+1; i++ {
|
|
chainTipHash, _, err = tc.AddBlock([]*externalapi.DomainHash{chainTipHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
}
|
|
|
|
// Add another block above the genesis block. This will trigger an
|
|
// earlier-than-reindex-root reindex
|
|
sideBlock, _, err := tc.AddBlock([]*externalapi.DomainHash{params.GenesisHash}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
// Add a block whose parents are the chain tip and the side block.
|
|
// We expect this not to fail
|
|
_, _, err = tc.AddBlock([]*externalapi.DomainHash{sideBlock}, nil, nil)
|
|
if err != nil {
|
|
t.Fatalf("AddBlock: %+v", err)
|
|
}
|
|
|
|
err = tc.ReachabilityManager().ValidateIntervals(params.GenesisHash)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
})
|
|
}
|